ProductPromotion
GitHub - hishamkaram/gismanager: Publish Your GIS Data(Vector Data) to PostGIS and GeoserverPublish Your GIS Data(Vector Data) to PostGIS and Geoserver - hishamkaram/gismanager
Visit Site
GitHub - hishamkaram/gismanager: Publish Your GIS Data(Vector Data) to PostGIS and Geoserver
GISManager
Publish Your GIS Data(Vector Data) to PostGIS and Geoserver
- How to install:
go get -v github.com/hishamkaram/gismanager
- Usage:
testdata
folder content:./testdata/ ├── neighborhood_names_gis.geojson ├── nested │ └── nyc_wi-fi_hotspot_locations.geojson ├── sample.gpkg
- create
ManagerConfig
instance:manager:= gismanager.ManagerConfig{ Geoserver: gismanager.GeoserverConfig{WorkspaceName: "golang", Username: "admin", Password: "geoserver", ServerURL: "http://localhost:8080/geoserver"}, Datastore: gismanager.DatastoreConfig{Host: "localhost", Port: 5432, DBName: "gis", DBUser: "golang", DBPass: "golang", Name: "gismanager_data"}, Source: gismanager.SourceConfig{Path: "./testdata"}, logger: gismanager.GetLogger(), }
- get Supported GIS Files:
files, _ := gismanager.GetGISFiles(manager.Source.Path) for _, file := range files { fmt.Println(file) }
- output:
<full_path>/testdata/neighborhood_names_gis.geojson <full_path>/testdata/nested/nyc_wi-fi_hotspot_locations.geojson <full_path>/testdata/sample.gpkg
- output:
- read files and get layers Schema:
for _, file := range files { source, ok := manager.OpenSource(file, 0) if ok { for index := 0; index < source.LayerCount(); index++ { layer := source.LayerByIndex(index) gLayer := gismanager.GdalLayer{ Layer: &layer, } fmt.Println(layer.Name()) for _, f := range gLayer.GetLayerSchema() { fmt.Printf("\n%+v\n", *f) } } } }
- output sample:
neighborhood_names_gis {Name:geom Type:POINT} {Name:stacked Type:String} {Name:name Type:String} {Name:annoline1 Type:String} {Name:annoline3 Type:String} {Name:objectid Type:String} {Name:annoangle Type:String} {Name:annoline2 Type:String} {Name:borough Type:String} ...
- output sample:
- add your gis data to your database:
for _, file := range files { source, ok := manager.OpenSource(file, 0) targetSource, targetOK := manager.OpenSource(manager.Datastore.BuildConnectionString(), 1) if ok && targetOK { for index := 0; index < source.LayerCount(); index++ { layer := source.LayerByIndex(index) gLayer := gismanager.GdalLayer{ Layer: &layer, } newLayer, postgisErr := gLayer.LayerToPostgis(targetSource, manager, true) if postgisErr != nil { panic(postgisErr) } logger.Infof("Layer: %s added to you database", newLayer.Name()) } } }
- output:
INFO[14-10-2018 17:28:37] Layer: neighborhood_names_gis added to you database INFO[14-10-2018 17:28:38] Layer: nyc_wi_fi_hotspot_locations added to you database INFO[14-10-2018 17:28:38] Layer: hwy_patrol added to you database
- output:
- update the previous code to publish your postgis layers to geoserver
for _, file := range files { source, ok := manager.OpenSource(file, 0) targetSource, targetOK := manager.OpenSource(manager.Datastore.BuildConnectionString(), 1) if ok && targetOK { for index := 0; index < source.LayerCount(); index++ { layer := source.LayerByIndex(index) gLayer := gismanager.GdalLayer{ Layer: &layer, } if newLayer, postgisErr := gLayer.LayerToPostgis(targetSource, manager, true); newLayer.Layer != nil || postgisErr != nil { ok, pubErr := manager.PublishGeoserverLayer(newLayer) if pubErr != nil { logger.Error(pubErr) } if !ok { logger.Error("Failed to Publish") } else { logger.Info("published") } } } } }
- output:
INFO[14-10-2018 17:37:07] url:http://localhost:8080/geoserver/rest/workspaces/golang Status=404 ERRO[14-10-2018 17:37:07] No such workspace: 'golang' found INFO[14-10-2018 17:37:07] url:http://localhost:8080/geoserver/rest/workspaces Status=201 INFO[14-10-2018 17:37:07] url:http://localhost:8080/geoserver/rest/workspaces/golang/datastores/gis?quietOnNotFound=true Status=404 INFO[14-10-2018 17:37:07] url:http://localhost:8080/geoserver/rest/workspaces/golang/datastores Status=201 ERRO[14-10-2018 17:37:07] {"featureType":{"name":"neighborhood_names_gis","nativeName":"neighborhood_names_gis"}} INFO[14-10-2018 17:37:07] url:http://localhost:8080/geoserver/rest/workspaces/golang/datastores/gis/featuretypes Status=201 INFO[14-10-2018 17:37:07] published INFO[14-10-2018 17:37:08] url:http://localhost:8080/geoserver/rest/workspaces/golang Status=200 INFO[14-10-2018 17:37:08] url:http://localhost:8080/geoserver/rest/workspaces/golang/datastores/gis?quietOnNotFound=true Status=200 ERRO[14-10-2018 17:37:08] {"featureType":{"name":"nyc_wi_fi_hotspot_locations","nativeName":"nyc_wi_fi_hotspot_locations"}} INFO[14-10-2018 17:37:08] url:http://localhost:8080/geoserver/rest/workspaces/golang/datastores/gis/featuretypes Status=201 INFO[14-10-2018 17:37:08] published INFO[14-10-2018 17:37:08] url:http://localhost:8080/geoserver/rest/workspaces/golang Status=200 INFO[14-10-2018 17:37:08] url:http://localhost:8080/geoserver/rest/workspaces/golang/datastores/gis?quietOnNotFound=true Status=200 ERRO[14-10-2018 17:37:08] {"featureType":{"name":"hwy_patrol","nativeName":"hwy_patrol"}} INFO[14-10-2018 17:37:08] url:http://localhost:8080/geoserver/rest/workspaces/golang/datastores/gis/featuretypes Status=201 INFO[14-10-2018 17:37:08] published
- done check you geoserver or via geoserver rest api url http://localhost:8080/geoserver/rest/layers.json :
{ "layers": { "layer": [..., { "name": "golang:hwy_patrol", "href": "http:\/\/localhost:8080\/geoserver\/rest\/layers\/golang%3Ahwy_patrol.json" }, { "name": "golang:neighborhood_names_gis", "href": "http:\/\/localhost:8080\/geoserver\/rest\/layers\/golang%3Aneighborhood_names_gis.json" }, { "name": "golang:nyc_wi_fi_hotspot_locations", "href": "http:\/\/localhost:8080\/geoserver\/rest\/layers\/golang%3Anyc_wi_fi_hotspot_locations.json" }] } }
Todo:
- backup postgis as geopackage
Articlesto learn more about the golang concepts.
- 1Go Language Basics
- 2Top 10 Go Interview Questions and Answers for Beginners
- 3Understanding Go Goroutines and Concurrency
- 4Error Handling in Go
- 5Building RESTful APIs with Go: A Step-by-Step Guide
- 6Creating a Web Server in Go
- 7Building a Command-Line Tool with Go: A Step-by-Step Tutorial
- 8Real-Time Web Applications with Go: WebSockets
- 9Go Modules: Managing Dependencies in Your Go Projects
- 10Optimizing Go Code for Performance
Resourceswhich are currently available to browse on.
mail [email protected] to add your project or resources here 🔥.
- 1Task automation Go library
https://github.com/goyek/goyek
Task automation Go library. Contribute to goyek/goyek development by creating an account on GitHub.
- 2gommon/color at master · labstack/gommon
https://github.com/labstack/gommon/tree/master/color
Common packages for Go. Contribute to labstack/gommon development by creating an account on GitHub.
- 3A modern text/numeric/geo-spatial/vector indexing library for go
https://github.com/blevesearch/bleve
A modern text/numeric/geo-spatial/vector indexing library for go - blevesearch/bleve
- 4A really basic thread-safe progress bar for Golang applications
https://github.com/schollz/progressbar
A really basic thread-safe progress bar for Golang applications - schollz/progressbar
- 5Highly concurrent drop-in replacement for bufio.Writer
https://github.com/free/concurrent-writer
Highly concurrent drop-in replacement for bufio.Writer - free/concurrent-writer
- 6mattn/go-colorable
https://github.com/mattn/go-colorable
Contribute to mattn/go-colorable development by creating an account on GitHub.
- 7A simple Set data structure implementation in Go (Golang) using LinkedHashMap.
https://github.com/StudioSol/set
A simple Set data structure implementation in Go (Golang) using LinkedHashMap. - StudioSol/set
- 8Authenticated encrypted API tokens (IETF XChaCha20-Poly1305 AEAD) for Golang
https://github.com/essentialkaos/branca
Authenticated encrypted API tokens (IETF XChaCha20-Poly1305 AEAD) for Golang - essentialkaos/branca
- 9Cloud Native Computing Foundation
https://www.cncf.io/.
CNCF is the vendor-neutral hub of cloud native computing, dedicated to making cloud native ubiquitous.
- 10Genv is a library for Go (golang) that makes it easy to read and use environment variables in your projects. It also allows environment variables to be loaded from the .env file.
https://github.com/sakirsensoy/genv
Genv is a library for Go (golang) that makes it easy to read and use environment variables in your projects. It also allows environment variables to be loaded from the .env file. - sakirsensoy/genv
- 11Load configuration in cascade from multiple backends into a struct
https://github.com/heetch/confita
Load configuration in cascade from multiple backends into a struct - heetch/confita
- 12Simple JWT Golang
https://github.com/brianvoe/sjwt
Simple JWT Golang. Contribute to brianvoe/sjwt development by creating an account on GitHub.
- 13Go package that encodes and decodes INI-files
https://github.com/subpop/go-ini
Go package that encodes and decodes INI-files. Contribute to subpop/go-ini development by creating an account on GitHub.
- 14Go tools for audio processing & creation 🎶
https://github.com/DylanMeeus/GoAudio
Go tools for audio processing & creation 🎶. Contribute to DylanMeeus/GoAudio development by creating an account on GitHub.
- 15Change the color of console text.
https://github.com/daviddengcn/go-colortext
Change the color of console text. Contribute to daviddengcn/go-colortext development by creating an account on GitHub.
- 16[Unmaintained] Open source two-factor authentication for Android
https://github.com/andOTP/andOTP
[Unmaintained] Open source two-factor authentication for Android - andOTP/andOTP
- 17Dynamic configuration file templating tool for kubernetes manifest or general configuration files
https://github.com/alfiankan/crab-config-files-templating
Dynamic configuration file templating tool for kubernetes manifest or general configuration files - alfiankan/crab-config-files-templating
- 18A flexible multi-layer Go caching library to deal with in-memory and shared cache by adopting Cache-Aside pattern.
https://github.com/viney-shih/go-cache
A flexible multi-layer Go caching library to deal with in-memory and shared cache by adopting Cache-Aside pattern. - viney-shih/go-cache
- 19An ANSI colour terminal package for Go
https://github.com/TreyBastian/colourize
An ANSI colour terminal package for Go. Contribute to TreyBastian/colourize development by creating an account on GitHub.
- 20A customizable, interactive table component for the Bubble Tea framework
https://github.com/Evertras/bubble-table
A customizable, interactive table component for the Bubble Tea framework - Evertras/bubble-table
- 21A Go package that read or set data from map, slice or json
https://github.com/deatil/go-array
A Go package that read or set data from map, slice or json - deatil/go-array
- 22CometBFT (fork of Tendermint Core): A distributed, Byzantine fault-tolerant, deterministic state machine replication engine
https://github.com/cometbft/cometbft
CometBFT (fork of Tendermint Core): A distributed, Byzantine fault-tolerant, deterministic state machine replication engine - cometbft/cometbft
- 23Go session management for web servers (including support for Google App Engine - GAE).
https://github.com/icza/session
Go session management for web servers (including support for Google App Engine - GAE). - icza/session
- 24Safe, simple and fast JSON Web Tokens for Go
https://github.com/cristalhq/jwt
Safe, simple and fast JSON Web Tokens for Go. Contribute to cristalhq/jwt development by creating an account on GitHub.
- 25a thread-safe concurrent map for go
https://github.com/lrita/cmap
a thread-safe concurrent map for go. Contribute to lrita/cmap development by creating an account on GitHub.
- 26Console Text Colors - The non-invasive cross-platform terminal color library does not need to modify the Print method
https://github.com/wzshiming/ctc
Console Text Colors - The non-invasive cross-platform terminal color library does not need to modify the Print method - wzshiming/ctc
- 27map library using Go generics that offers a standard interface, go routine synchronization, and sorting
https://github.com/goradd/maps
map library using Go generics that offers a standard interface, go routine synchronization, and sorting - goradd/maps
- 28Telegram Bot Exec Terminal Command
https://github.com/alfiankan/teleterm
Telegram Bot Exec Terminal Command. Contribute to alfiankan/teleterm development by creating an account on GitHub.
- 2912 factor configuration as a typesafe struct in as little as two function calls
https://github.com/JeremyLoy/config
12 factor configuration as a typesafe struct in as little as two function calls - JeremyLoy/config
- 30Layer based configuration for golang
https://github.com/goraz/onion
Layer based configuration for golang. Contribute to goraz/onion development by creating an account on GitHub.
- 31Authenticated and encrypted API tokens using modern crypto
https://github.com/tuupola/branca-spec
Authenticated and encrypted API tokens using modern crypto - tuupola/branca-spec
- 32A RESTful caching micro-service in Go backed by Couchbase
https://github.com/codingsince1985/couchcache
A RESTful caching micro-service in Go backed by Couchbase - codingsince1985/couchcache
- 33Golang Framework for writing Slack bots
https://github.com/sbstjn/hanu
Golang Framework for writing Slack bots. Contribute to sbstjn/hanu development by creating an account on GitHub.
- 34Go bindings for the PortAudio audio I/O library
https://github.com/gordonklaus/portaudio
Go bindings for the PortAudio audio I/O library. Contribute to gordonklaus/portaudio development by creating an account on GitHub.
- 35Middleware for keeping track of users, login states and permissions
https://github.com/xyproto/permissions2
:closed_lock_with_key: Middleware for keeping track of users, login states and permissions - GitHub - xyproto/permissions2: Middleware for keeping track of users, login states and permissions
- 36:art: Contextual fmt inspired by bootstrap color classes
https://github.com/mingrammer/cfmt
:art: Contextual fmt inspired by bootstrap color classes - mingrammer/cfmt
- 37Straightforward HTTP session management
https://github.com/swithek/sessionup
Straightforward HTTP session management. Contribute to swithek/sessionup development by creating an account on GitHub.
- 38Package goth provides a simple, clean, and idiomatic way to write authentication packages for Go web applications.
https://github.com/markbates/goth
Package goth provides a simple, clean, and idiomatic way to write authentication packages for Go web applications. - markbates/goth
- 39Intuitive package for prettifying terminal/console output. http://godoc.org/github.com/ttacon/chalk
https://github.com/ttacon/chalk
Intuitive package for prettifying terminal/console output. http://godoc.org/github.com/ttacon/chalk - ttacon/chalk
- 40HTTP Session Management for Go
https://github.com/alexedwards/scs
HTTP Session Management for Go. Contribute to alexedwards/scs development by creating an account on GitHub.
- 41:handbag: Cache arbitrary data with an expiration time.
https://github.com/akyoto/cache
:handbag: Cache arbitrary data with an expiration time. - akyoto/cache
- 42Go Bindings for libsamplerate
https://github.com/dh1tw/gosamplerate
Go Bindings for libsamplerate. Contribute to dh1tw/gosamplerate development by creating an account on GitHub.
- 43Ordered-concurrently a library for concurrent processing with ordered output in Go. Process work concurrently and returns output in a channel in the order of input. It is useful in concurrently processing items in a queue, and get output in the order provided by the queue.
https://github.com/tejzpr/ordered-concurrently
Ordered-concurrently a library for concurrent processing with ordered output in Go. Process work concurrently and returns output in a channel in the order of input. It is useful in concurrently pro...
- 44A minimal but powerful cli library for Go
https://github.com/jxskiss/mcli
A minimal but powerful cli library for Go. Contribute to jxskiss/mcli development by creating an account on GitHub.
- 45Go package to make lightweight ASCII line graph ╭┈╯ in command line apps with no other dependencies.
https://github.com/guptarohit/asciigraph
Go package to make lightweight ASCII line graph ╭┈╯ in command line apps with no other dependencies. - guptarohit/asciigraph
- 46The Tenyks IRC bot.
https://github.com/kyleterry/tenyks
The Tenyks IRC bot. Contribute to kyleterry/tenyks development by creating an account on GitHub.
- 47Advanced ANSI style & color support for your terminal applications
https://github.com/muesli/termenv
Advanced ANSI style & color support for your terminal applications - muesli/termenv
- 48cookiestxt implement parser of cookies txt format
https://github.com/mengzhuo/cookiestxt
cookiestxt implement parser of cookies txt format. Contribute to mengzhuo/cookiestxt development by creating an account on GitHub.
- 49A dead simple, highly performant, highly customizable sessions middleware for go http servers.
https://github.com/adam-hanna/sessions
A dead simple, highly performant, highly customizable sessions middleware for go http servers. - adam-hanna/sessions
- 50:crystal_ball: Simple application watcher
https://github.com/GuilhermeCaruso/anko
:crystal_ball: Simple application watcher. Contribute to GuilhermeCaruso/anko development by creating an account on GitHub.
- 51golang persistent immutable treap sorted sets
https://github.com/perdata/treap
golang persistent immutable treap sorted sets. Contribute to perdata/treap development by creating an account on GitHub.
- 52Easy to use OpenID Connect client and server library written for Go and certified by the OpenID Foundation
https://github.com/zitadel/oidc
Easy to use OpenID Connect client and server library written for Go and certified by the OpenID Foundation - zitadel/oidc
- 53a Make/rake-like dev tool using Go
https://github.com/magefile/mage
a Make/rake-like dev tool using Go. Contribute to magefile/mage development by creating an account on GitHub.
- 54Small library for simple and convenient formatted stylized output to the console.
https://github.com/i582/cfmt
Small library for simple and convenient formatted stylized output to the console. - i582/cfmt
- 55Terminal based dashboard.
https://github.com/mum4k/termdash
Terminal based dashboard. Contribute to mum4k/termdash development by creating an account on GitHub.
- 56CONTRIBUTIONS ONLY: A Go (golang) command line and flag parser
https://github.com/alecthomas/kingpin
CONTRIBUTIONS ONLY: A Go (golang) command line and flag parser - alecthomas/kingpin
- 57Bencher - Continuous Benchmarking
https://bencher.dev/
Catch performance regressions in CI with continuous benchmarking
- 58Library to generate TOTP/HOTP codes
https://github.com/grijul/otpgen
Library to generate TOTP/HOTP codes. Contribute to RijulGulati/otpgen development by creating an account on GitHub.
- 59Data structure and algorithm library for go, designed to provide functions similar to C++ STL
https://github.com/liyue201/gostl
Data structure and algorithm library for go, designed to provide functions similar to C++ STL - liyue201/gostl
- 60A Go library for implementing command-line interfaces.
https://github.com/mitchellh/cli
A Go library for implementing command-line interfaces. - mitchellh/cli
- 61Argparse for golang. Just because `flag` sucks
https://github.com/akamensky/argparse
Argparse for golang. Just because `flag` sucks. Contribute to akamensky/argparse development by creating an account on GitHub.
- 62Eventually consistent distributed in-memory cache Go library
https://github.com/iwanbk/bcache
Eventually consistent distributed in-memory cache Go library - iwanbk/bcache
- 63A Discord bot for managing ephemeral roles based upon voice channel member presence.
https://github.com/ewohltman/ephemeral-roles
A Discord bot for managing ephemeral roles based upon voice channel member presence. - ewohltman/ephemeral-roles
- 64Package flac provides access to FLAC (Free Lossless Audio Codec) streams.
https://github.com/mewkiz/flac
Package flac provides access to FLAC (Free Lossless Audio Codec) streams. - mewkiz/flac
- 65Go-Guardian is a golang library that provides a simple, clean, and idiomatic way to create powerful modern API and web authentication.
https://github.com/shaj13/go-guardian
Go-Guardian is a golang library that provides a simple, clean, and idiomatic way to create powerful modern API and web authentication. - shaj13/go-guardian
- 66Decode mp3 base on https://github.com/lieff/minimp3
https://github.com/tosone/minimp3
Decode mp3 base on https://github.com/lieff/minimp3 - tosone/minimp3
- 67Platform-Agnostic Security Tokens implementation in GO (Golang)
https://github.com/o1egl/paseto
Platform-Agnostic Security Tokens implementation in GO (Golang) - o1egl/paseto
- 68goRBAC provides a lightweight role-based access control (RBAC) implementation in Golang.
https://github.com/mikespook/gorbac
goRBAC provides a lightweight role-based access control (RBAC) implementation in Golang. - mikespook/gorbac
- 69Golang telegram bot API wrapper, session-based router and middleware
https://github.com/olebedev/go-tgbot
Golang telegram bot API wrapper, session-based router and middleware - olebedev/go-tgbot
- 70🍍Jeff provides the simplest way to manage web sessions in Go.
https://github.com/abraithwaite/jeff
🍍Jeff provides the simplest way to manage web sessions in Go. - abraithwaite/jeff
- 71Decorate your terminals using Palette 🎨
https://github.com/abusomani/go-palette
Decorate your terminals using Palette 🎨. Contribute to abusomani/go-palette development by creating an account on GitHub.
- 72JOB, make your short-term command as a long-term job. 将命令行规划成任务的工具
https://github.com/liujianping/job
JOB, make your short-term command as a long-term job. 将命令行规划成任务的工具 - liujianping/job
- 73Generic sorted map for Go with red-black tree under the hood
https://github.com/igrmk/treemap
Generic sorted map for Go with red-black tree under the hood - igrmk/treemap
- 74A task runner / simpler Make alternative written in Go
https://github.com/go-task/task
A task runner / simpler Make alternative written in Go - go-task/task
- 75JSON Web Token library
https://github.com/pascaldekloe/jwt
JSON Web Token library. Contribute to pascaldekloe/jwt development by creating an account on GitHub.
- 76Go OAuth2
https://github.com/golang/oauth2
Go OAuth2. Contribute to golang/oauth2 development by creating an account on GitHub.
- 77Client lib for Telegram bot api
https://github.com/onrik/micha
Client lib for Telegram bot api. Contribute to onrik/micha development by creating an account on GitHub.
- 78🖍️ Marker is the easiest way to match and mark strings for colorful terminal outputs!
https://github.com/cyucelen/marker
🖍️ Marker is the easiest way to match and mark strings for colorful terminal outputs! - cyucelen/marker
- 79A go library for easy configure and run command chains. Such like pipelining in unix shells.
https://github.com/rainu/go-command-chain
A go library for easy configure and run command chains. Such like pipelining in unix shells. - rainu/go-command-chain
- 80Go-based framework for building Polkadot/Substrate-compatible runtimes. 🧱
https://github.com/LimeChain/gosemble
Go-based framework for building Polkadot/Substrate-compatible runtimes. 🧱 - LimeChain/gosemble
- 81Builds and restarts a Go project when it crashes or some watched file changes
https://github.com/maxcnunes/gaper
Builds and restarts a Go project when it crashes or some watched file changes - maxcnunes/gaper
- 82A Simple and Clear CLI library. Dependency free.
https://github.com/leaanthony/clir
A Simple and Clear CLI library. Dependency free. Contribute to leaanthony/clir development by creating an account on GitHub.
- 83A Go port of Ruby's dotenv library (Loads environment variables from .env files)
https://github.com/joho/godotenv
A Go port of Ruby's dotenv library (Loads environment variables from .env files) - joho/godotenv
- 84Tools for parse JSON-like logs for collecting unique fields and events
https://github.com/MonaxGT/parsefields
Tools for parse JSON-like logs for collecting unique fields and events - MonaxGT/parsefields
- 85Finite State Machine package in Go
https://github.com/cocoonspace/fsm
Finite State Machine package in Go. Contribute to cocoonspace/fsm development by creating an account on GitHub.
- 86Minimalist Go package aimed at creating Console User Interfaces.
https://github.com/jroimartin/gocui
Minimalist Go package aimed at creating Console User Interfaces. - jroimartin/gocui
- 87The open source high performance ELT framework powered by Apache Arrow
http://github.com/cloudquery/cloudquery
The open source high performance ELT framework powered by Apache Arrow - cloudquery/cloudquery
- 88Telegram Bot API Go framework
https://github.com/go-telegram/bot
Telegram Bot API Go framework. Contribute to go-telegram/bot development by creating an account on GitHub.
- 89Fast, zero-allocation, lexicographic-order-preserving packing/unpacking of native Go types to bytes.
https://github.com/iancmcc/bingo
Fast, zero-allocation, lexicographic-order-preserving packing/unpacking of native Go types to bytes. - iancmcc/bingo
- 90Tabular simplifies printing ASCII tables from command line utilities
https://github.com/InVisionApp/tabular
Tabular simplifies printing ASCII tables from command line utilities - InVisionApp/tabular
- 91mattn/go-isatty
https://github.com/mattn/go-isatty
Contribute to mattn/go-isatty development by creating an account on GitHub.
- 92multi progress bar for Go cli applications
https://github.com/vbauerster/mpb
multi progress bar for Go cli applications. Contribute to vbauerster/mpb development by creating an account on GitHub.
- 93Slack Bot Framework
https://github.com/shomali11/slacker
Slack Bot Framework. Contribute to shomali11/slacker development by creating an account on GitHub.
- 94⟁ Tendermint Core (BFT Consensus) in Go
https://github.com/tendermint/tendermint
⟁ Tendermint Core (BFT Consensus) in Go. Contribute to tendermint/tendermint development by creating an account on GitHub.
- 95Golang OAuth2 server library
https://github.com/openshift/osin
Golang OAuth2 server library. Contribute to openshift/osin development by creating an account on GitHub.
- 96Go login handlers for authentication providers (OAuth1, OAuth2)
https://github.com/dghubble/gologin
Go login handlers for authentication providers (OAuth1, OAuth2) - dghubble/gologin
- 97TUI components for Bubble Tea 🫧
https://github.com/charmbracelet/bubbles
TUI components for Bubble Tea 🫧. Contribute to charmbracelet/bubbles development by creating an account on GitHub.
- 98A versatile library for building CLI applications in Go
https://github.com/jawher/mow.cli
A versatile library for building CLI applications in Go - jawher/mow.cli
- 99Another Text Attribute Manupulator
https://github.com/workanator/go-ataman
Another Text Attribute Manupulator. Contribute to workanator/go-ataman development by creating an account on GitHub.
- 100Markdown defined task runner.
https://github.com/joerdav/xc
Markdown defined task runner. Contribute to joerdav/xc development by creating an account on GitHub.
- 101Simple, useful and opinionated CLI package in Go.
https://github.com/cristalhq/acmd
Simple, useful and opinionated CLI package in Go. Contribute to cristalhq/acmd development by creating an account on GitHub.
- 102Time-Based One-Time Password (TOTP) and HMAC-Based One-Time Password (HOTP) library for Go.
https://github.com/jltorresm/otpgo
Time-Based One-Time Password (TOTP) and HMAC-Based One-Time Password (HOTP) library for Go. - jltorresm/otpgo
- 103A go library to improve readability in terminal apps using tabular data
https://github.com/gosuri/uitable
A go library to improve readability in terminal apps using tabular data - gosuri/uitable
- 104A tiny library for super simple Golang tables
https://github.com/cheynewallace/tabby
A tiny library for super simple Golang tables. Contribute to cheynewallace/tabby development by creating an account on GitHub.
- 105Library for easy configuration of a golang service
https://github.com/ThomasObenaus/go-conf
Library for easy configuration of a golang service - ThomasObenaus/go-conf
- 106A golang package for parsing ini-style configuration files
https://github.com/sasbury/mini
A golang package for parsing ini-style configuration files - sasbury/mini
- 107Pipelines using goroutines
https://github.com/hyfather/pipeline
Pipelines using goroutines. Contribute to hyfather/pipeline development by creating an account on GitHub.
- 108A golang implementation of a console-based trading bot for cryptocurrency exchanges
https://github.com/saniales/golang-crypto-trading-bot
A golang implementation of a console-based trading bot for cryptocurrency exchanges - saniales/golang-crypto-trading-bot
- 109go irc client for twitch.tv
https://github.com/gempir/go-twitch-irc
go irc client for twitch.tv. Contribute to gempir/go-twitch-irc development by creating an account on GitHub.
- 110Golang library for managing configuration data from environment variables
https://github.com/kelseyhightower/envconfig
Golang library for managing configuration data from environment variables - kelseyhightower/envconfig
- 111GAAD (Go Advanced Audio Decoder)
https://github.com/Comcast/gaad
GAAD (Go Advanced Audio Decoder). Contribute to Comcast/gaad development by creating an account on GitHub.
- 112IRC, Slack, Telegram and RocketChat bot written in go
https://github.com/go-chat-bot/bot
IRC, Slack, Telegram and RocketChat bot written in go - go-chat-bot/bot
- 113hiboot/pkg/app/cli at master · hidevopsio/hiboot
https://github.com/hidevopsio/hiboot/tree/master/pkg/app/cli
hiboot is a high performance web and cli application framework with dependency injection support - hidevopsio/hiboot
- 114This package provides json web token (jwt) middleware for goLang http servers
https://github.com/adam-hanna/jwt-auth
This package provides json web token (jwt) middleware for goLang http servers - adam-hanna/jwt-auth
- 115Data structure and relevant algorithms for extremely fast prefix/fuzzy string searching.
https://github.com/derekparker/trie
Data structure and relevant algorithms for extremely fast prefix/fuzzy string searching. - derekparker/trie
- 116skiplist for golang
https://github.com/gansidui/skiplist
skiplist for golang. Contribute to gansidui/skiplist development by creating an account on GitHub.
- 117Mini audio library
https://github.com/gen2brain/malgo
Mini audio library. Contribute to gen2brain/malgo development by creating an account on GitHub.
- 118Go implementation of JSON Web Tokens (JWT).
https://github.com/golang-jwt/jwt
Go implementation of JSON Web Tokens (JWT). Contribute to golang-jwt/jwt development by creating an account on GitHub.
- 119Declarative configuration for Go
https://github.com/num30/config
Declarative configuration for Go. Contribute to num30/config development by creating an account on GitHub.
- 120Go library for Telegram Bot API
https://github.com/yanzay/tbot
Go library for Telegram Bot API. Contribute to yanzay/tbot development by creating an account on GitHub.
- 121Nullable Go types that can be marshalled/unmarshalled to/from JSON.
https://github.com/emvi/null
Nullable Go types that can be marshalled/unmarshalled to/from JSON. - emvi/null
- 122Larry 🐦 is a bot generator that post content from different providers to one or multiple publishers
https://github.com/ezeoleaf/larry
Larry 🐦 is a bot generator that post content from different providers to one or multiple publishers - ezeoleaf/larry
- 123Make Highly Customized Boxes for CLI
https://github.com/Delta456/box-cli-maker
Make Highly Customized Boxes for CLI. Contribute to Delta456/box-cli-maker development by creating an account on GitHub.
- 124Small library to read your configuration from environment variables
https://github.com/vrischmann/envconfig
Small library to read your configuration from environment variables - vrischmann/envconfig
- 125Modern Make
https://github.com/tj/mmake
Modern Make . Contribute to tj/mmake development by creating an account on GitHub.
- 126LangChain for Go, the easiest way to write LLM-based programs in Go
https://github.com/tmc/langchaingo
LangChain for Go, the easiest way to write LLM-based programs in Go - tmc/langchaingo
- 127An implementation of JOSE standards (JWE, JWS, JWT) in Go
https://github.com/go-jose/go-jose
An implementation of JOSE standards (JWE, JWS, JWT) in Go - go-jose/go-jose
- 128Fast, secure and efficient secure cookie encoder/decoder
https://github.com/chmike/securecookie
Fast, secure and efficient secure cookie encoder/decoder - GitHub - chmike/securecookie: Fast, secure and efficient secure cookie encoder/decoder
- 129Go implementation of the Ethereum protocol
https://github.com/ethereum/go-ethereum
Go implementation of the Ethereum protocol. Contribute to ethereum/go-ethereum development by creating an account on GitHub.
- 130Cuckoo Filter: Practically Better Than Bloom
https://github.com/seiflotfy/cuckoofilter
Cuckoo Filter: Practically Better Than Bloom. Contribute to seiflotfy/cuckoofilter development by creating an account on GitHub.
- 131A high performance and flexible authorization/permission engine built for developers and inspired by Google Zanzibar
https://github.com/openfga/openfga
A high performance and flexible authorization/permission engine built for developers and inspired by Google Zanzibar - openfga/openfga
- 132A minimal continuous integration system. Uses docker to run jobs concurrently in stages.
https://github.com/opnlabs/dot
A minimal continuous integration system. Uses docker to run jobs concurrently in stages. - opnlabs/dot
- 133Levenshtein distance and similarity metrics with customizable edit costs and Winkler-like bonus for common prefix.
https://github.com/agext/levenshtein
Levenshtein distance and similarity metrics with customizable edit costs and Winkler-like bonus for common prefix. - agext/levenshtein
- 134VK bot package for Go
https://github.com/nikepan/govkbot
VK bot package for Go. Contribute to nikepan/govkbot development by creating an account on GitHub.
- 135Library for setting values to structs' fields from env, flags, files or default tag
https://github.com/BoRuDar/configuration
Library for setting values to structs' fields from env, flags, files or default tag - BoRuDar/configuration
- 136Building powerful interactive prompts in Go, inspired by python-prompt-toolkit.
https://github.com/c-bata/go-prompt
Building powerful interactive prompts in Go, inspired by python-prompt-toolkit. - c-bata/go-prompt
- 137Concurrent task runner, developer's routine tasks automation toolkit. Simple modern alternative to GNU Make 🧰
https://github.com/taskctl/taskctl
Concurrent task runner, developer's routine tasks automation toolkit. Simple modern alternative to GNU Make 🧰 - taskctl/taskctl
- 138🎵 ID3 decoding and encoding library for Go
https://github.com/bogem/id3v2
🎵 ID3 decoding and encoding library for Go. Contribute to n10v/id3v2 development by creating an account on GitHub.
- 139A simple, battle-tested and generic set type for the Go language. Trusted by Docker, 1Password, Ethereum and Hashicorp.
https://github.com/deckarep/golang-set
A simple, battle-tested and generic set type for the Go language. Trusted by Docker, 1Password, Ethereum and Hashicorp. - deckarep/golang-set
- 140Fancy stream processing made operationally mundane
https://github.com/benthosdev/benthos
Fancy stream processing made operationally mundane - redpanda-data/connect
- 141Structured data format to config struct decoder library for Go
https://github.com/greencoda/confiq
Structured data format to config struct decoder library for Go - greencoda/confiq
- 142A collection of CLI argument types for the Go `flag` package.
https://github.com/sgreben/flagvar
A collection of CLI argument types for the Go `flag` package. - sgreben/flagvar
- 143Disjoint Set data structure implementation in Go
https://github.com/ihebu/dsu
Disjoint Set data structure implementation in Go. Contribute to ihebu/dsu development by creating an account on GitHub.
- 144Telegram Bot Framework for Go
https://github.com/zhulik/margelet
Telegram Bot Framework for Go. Contribute to zhulik/margelet development by creating an account on GitHub.
- 145go implementation of lightbend's HOCON configuration library https://github.com/lightbend/config
https://github.com/gurkankaymak/hocon
go implementation of lightbend's HOCON configuration library https://github.com/lightbend/config - gurkankaymak/hocon
- 146pretty colorfull tables in go with less effort
https://github.com/tomlazar/table
pretty colorfull tables in go with less effort. Contribute to tomlazar/table development by creating an account on GitHub.
- 147Go helpers to manage environment variables
https://github.com/antham/envh
Go helpers to manage environment variables. Contribute to antham/envh development by creating an account on GitHub.
- 148:robot: The free, Open Source alternative to OpenAI, Claude and others. Self-hosted and local-first. Drop-in replacement for OpenAI, running on consumer-grade hardware. No GPU required. Runs gguf, transformers, diffusers and many more models architectures. Features: Generate Text, Audio, Video, Images, Voice Cloning, Distributed inference
https://github.com/mudler/LocalAI
:robot: The free, Open Source alternative to OpenAI, Claude and others. Self-hosted and local-first. Drop-in replacement for OpenAI, running on consumer-grade hardware. No GPU required. Runs gguf,...
- 149mattn/goveralls
https://github.com/mattn/goveralls
Contribute to mattn/goveralls development by creating an account on GitHub.
- 150⚙️ Golang config manager. Control your configurations using tags, unmarshal to structs, implement and inject your own value providers and parsers.
https://github.com/Jagerente/gocfg
⚙️ Golang config manager. Control your configurations using tags, unmarshal to structs, implement and inject your own value providers and parsers. - Jagerente/gocfg
- 151A blazingly fast JSON serializing & deserializing library
https://github.com/bytedance/sonic
A blazingly fast JSON serializing & deserializing library - bytedance/sonic
- 152A simple, zero-dependencies library to parse environment variables into structs
https://github.com/caarlos0/env
A simple, zero-dependencies library to parse environment variables into structs - caarlos0/env
- 153CLI program to encrypt/decrypt andOTP files
https://github.com/grijul/go-andotp
CLI program to encrypt/decrypt andOTP files. Contribute to RijulGulati/go-andotp development by creating an account on GitHub.
- 154command argument completion generator for spf13/cobra
https://github.com/rsteube/carapace
command argument completion generator for spf13/cobra - carapace-sh/carapace
- 155Golang ultimate ANSI-colors that supports Printf/Sprintf methods
https://github.com/logrusorgru/aurora
Golang ultimate ANSI-colors that supports Printf/Sprintf methods - logrusorgru/aurora
- 156Paralleling pipeline
https://github.com/nazar256/parapipe
Paralleling pipeline. Contribute to nazar256/parapipe development by creating an account on GitHub.
- 157Strumt is a library to create prompt chain
https://github.com/antham/strumt
Strumt is a library to create prompt chain. Contribute to antham/strumt development by creating an account on GitHub.
- 158A go library to render progress bars in terminal applications
https://github.com/gosuri/uiprogress
A go library to render progress bars in terminal applications - gosuri/uiprogress
- 159Simple dense bitmap index in Go with binary operators
https://github.com/kelindar/bitmap
Simple dense bitmap index in Go with binary operators - kelindar/bitmap
- 160Fast ring-buffer deque (double-ended queue)
https://github.com/gammazero/deque
Fast ring-buffer deque (double-ended queue). Contribute to gammazero/deque development by creating an account on GitHub.
- 161🛠 Viper wrapper with config inheritance and key generation
https://github.com/Yiling-J/piper
🛠 Viper wrapper with config inheritance and key generation - Yiling-J/piper
- 162A zero-dependency generic in-memory cache Go library
https://github.com/erni27/imcache
A zero-dependency generic in-memory cache Go library - erni27/imcache
- 163Harvest configuration, watch and notify subscriber
https://github.com/beatlabs/harvester
Harvest configuration, watch and notify subscriber - beatlabs/harvester
- 164Cache Slow Database Queries
https://github.com/rocketlaunchr/remember-go
Cache Slow Database Queries. Contribute to rocketlaunchr/remember-go development by creating an account on GitHub.
- 165timestamp convert & compare tool. 时间戳转换与对比工具
https://github.com/liujianping/ts
timestamp convert & compare tool. 时间戳转换与对比工具. Contribute to liujianping/ts development by creating an account on GitHub.
- 166gone/jconf at master · One-com/gone
https://github.com/One-com/gone/tree/master/jconf
Golang packages for writing small daemons and servers. - One-com/gone
- 167high performance in-memory cache
https://github.com/Yiling-J/theine-go
high performance in-memory cache. Contribute to Yiling-J/theine-go development by creating an account on GitHub.
- 168Easily Manage OAuth2 Scopes In Go
https://github.com/SonicRoshan/scope
Easily Manage OAuth2 Scopes In Go. Contribute to ThundR67/scope development by creating an account on GitHub.
- 169Go (golang) package with 90 configurable terminal spinner/progress indicators.
https://github.com/briandowns/spinner
Go (golang) package with 90 configurable terminal spinner/progress indicators. - briandowns/spinner
- 170A tool for testing, building, signing, and publishing binaries.
https://github.com/nikogura/gomason
A tool for testing, building, signing, and publishing binaries. - nikogura/gomason
- 171Build software better, together
https://github.com/jonathanslenders/python-prompt-toolkit.
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 172Configuration loader to use in Go projects
https://github.com/crgimenes/goConfig
Configuration loader to use in Go projects. Contribute to crgimenes/config development by creating an account on GitHub.
- 173An authorization library that supports access control models like ACL, RBAC, ABAC in Golang: https://discord.gg/S5UjpzGZjN
https://github.com/hsluoyz/casbin
An authorization library that supports access control models like ACL, RBAC, ABAC in Golang: https://discord.gg/S5UjpzGZjN - casbin/casbin
- 174An in-memory cache with item expiration and generics
https://github.com/jellydator/ttlcache
An in-memory cache with item expiration and generics - jellydator/ttlcache
- 175GoDS (Go Data Structures) - Sets, Lists, Stacks, Maps, Trees, Queues, and much more
https://github.com/emirpasic/gods
GoDS (Go Data Structures) - Sets, Lists, Stacks, Maps, Trees, Queues, and much more - emirpasic/gods
- 176cosiner/argv
https://github.com/cosiner/argv
Contribute to cosiner/argv development by creating an account on GitHub.
- 177A powerful little TUI framework 🏗
https://github.com/charmbracelet/bubbletea
A powerful little TUI framework 🏗. Contribute to charmbracelet/bubbletea development by creating an account on GitHub.
- 178A minimalist Go configuration library
https://github.com/kkyr/fig
A minimalist Go configuration library. Contribute to kkyr/fig development by creating an account on GitHub.
- 179Go package for mapping values to and from space-filling curves, such as Hilbert and Peano curves.
https://github.com/google/hilbert
Go package for mapping values to and from space-filling curves, such as Hilbert and Peano curves. - google/hilbert
- 180Merkle hash trees
https://github.com/bobg/merkle
Merkle hash trees. Contribute to bobg/merkle development by creating an account on GitHub.
- 181A Go type to prevent internal numeric IDs from being exposed to clients using HashIDs and JSON.
https://github.com/emvi/hide
A Go type to prevent internal numeric IDs from being exposed to clients using HashIDs and JSON. - emvi/hide
- 182Go program designed to automate semantic versioning of Git repository by analyzing their formatted commit history and tagging them with the right semver.
https://github.com/s0ders/go-semver-release
Go program designed to automate semantic versioning of Git repository by analyzing their formatted commit history and tagging them with the right semver. - s0ders/go-semver-release
- 183A thread safe map which has expiring key-value pairs.
https://github.com/zekroTJA/timedmap
A thread safe map which has expiring key-value pairs. - zekroTJA/timedmap
- 184Drop-in replacement for Go's flag package, implementing POSIX/GNU-style --flags.
https://github.com/spf13/pflag
Drop-in replacement for Go's flag package, implementing POSIX/GNU-style --flags. - spf13/pflag
- 185Struct-based argument parsing in Go
https://github.com/alexflint/go-arg
Struct-based argument parsing in Go. Contribute to alexflint/go-arg development by creating an account on GitHub.
- 186GC-less, fast and generic LRU hashmap library for Go
https://github.com/elastic/go-freelru
GC-less, fast and generic LRU hashmap library for Go - elastic/go-freelru
- 187JWT login microservice with plugable backends such as OAuth2, Google, Github, htpasswd, osiam, ..
https://github.com/tarent/loginsrv
JWT login microservice with plugable backends such as OAuth2, Google, Github, htpasswd, osiam, .. - qvest-digital/loginsrv
- 188♪ A low-level library to play sound on multiple platforms ♪
https://github.com/hajimehoshi/oto
♪ A low-level library to play sound on multiple platforms ♪ - ebitengine/oto
- 189✨ #PTerm is a modern Go module to easily beautify console output. Featuring charts, progressbars, tables, trees, text input, select menus and much more 🚀 It's completely configurable and 100% cross-platform compatible.
https://github.com/pterm/pterm
✨ #PTerm is a modern Go module to easily beautify console output. Featuring charts, progressbars, tables, trees, text input, select menus and much more 🚀 It's completely configurable and 100% c...
- 190Go minimalist typed environment variables library
https://github.com/diegomarangoni/typenv
Go minimalist typed environment variables library. Contribute to diegomarangoni/typenv development by creating an account on GitHub.
- 191Go package implementing Bloom filters, used by Milvus and Beego.
https://github.com/bits-and-blooms/bloom
Go package implementing Bloom filters, used by Milvus and Beego. - bits-and-blooms/bloom
- 192Simple in-memory key-value storage with TTL for each record.
https://github.com/cheshir/ttlcache
Simple in-memory key-value storage with TTL for each record. - cheshir/ttlcache
- 193bobg/go-generics
https://github.com/bobg/go-generics
Contribute to bobg/go-generics development by creating an account on GitHub.
- 194A Go caching framework that supports multiple data source drivers
https://github.com/no-src/nscache
A Go caching framework that supports multiple data source drivers - no-src/nscache
- 195Automatically sets up command line flags based on struct fields and tags.
https://github.com/jaffee/commandeer
Automatically sets up command line flags based on struct fields and tags. - jaffee/commandeer
- 196An easy to use menu structure for cli applications that prompts users to make choices.
https://github.com/dixonwille/wmenu
An easy to use menu structure for cli applications that prompts users to make choices. - dixonwille/wmenu
- 197Fast thread-safe inmemory cache for big number of entries in Go. Minimizes GC overhead
https://github.com/VictoriaMetrics/fastcache
Fast thread-safe inmemory cache for big number of entries in Go. Minimizes GC overhead - VictoriaMetrics/fastcache
- 198Basic Configuration Language.
https://github.com/wkhere/bcl
Basic Configuration Language. Contribute to wkhere/bcl development by creating an account on GitHub.
- 199🧬 Pure DNA of your CLI!
https://github.com/mszostok/version
🧬 Pure DNA of your CLI! Contribute to mszostok/version development by creating an account on GitHub.
- 200This is quick session for net/http in golang. This package is perhaps the best implementation of the session mechanism, at least it tries to become one.
http://github.com/Kwynto/gosession
This is quick session for net/http in golang. This package is perhaps the best implementation of the session mechanism, at least it tries to become one. - Kwynto/gosession
- 201:pushpin: State of the art point location and neighbour finding algorithms for region quadtrees, in Go
https://github.com/aurelien-rainone/go-rquad
:pushpin: State of the art point location and neighbour finding algorithms for region quadtrees, in Go - arl/go-rquad
- 202:chains: A Framework for Building High Value Public Blockchains :sparkles:
https://github.com/cosmos/cosmos-sdk
:chains: A Framework for Building High Value Public Blockchains :sparkles: - cosmos/cosmos-sdk
- 203A Go implementation of the Elias-Fano encoding
https://github.com/amallia/go-ef
A Go implementation of the Elias-Fano encoding. Contribute to amallia/go-ef development by creating an account on GitHub.
- 204bash completion written in go + bash completion for go command
https://github.com/posener/complete
bash completion written in go + bash completion for go command - posener/complete
- 205POSIX-compliant command-line UI (CLI) parser and Hierarchical-configuration operations
https://github.com/hedzr/cmdr
POSIX-compliant command-line UI (CLI) parser and Hierarchical-configuration operations - hedzr/cmdr
- 206Adaptive Radix Trees implemented in Go
https://github.com/plar/go-adaptive-radix-tree
Adaptive Radix Trees implemented in Go. Contribute to plar/go-adaptive-radix-tree development by creating an account on GitHub.
- 207:jeans:Multi-Package go project coverprofile for tools like goveralls
https://github.com/go-playground/overalls
:jeans:Multi-Package go project coverprofile for tools like goveralls - go-playground/overalls
- 208The Coherence Go Client allows native Go applications to act as cache clients to a Coherence cluster using gRPC for the network transport.
https://github.com/oracle/coherence-go-client
The Coherence Go Client allows native Go applications to act as cache clients to a Coherence cluster using gRPC for the network transport. - oracle/coherence-go-client
- 209Pure Go line editor with history, inspired by linenoise
https://github.com/peterh/liner
Pure Go line editor with history, inspired by linenoise - peterh/liner
- 210✨Clean and minimalistic environment configuration reader for Golang
https://github.com/ilyakaznacheev/cleanenv
✨Clean and minimalistic environment configuration reader for Golang - ilyakaznacheev/cleanenv
- 211Lightweight, zero-dependency, and extendable configuration management library for Go
https://github.com/omeid/uconfig
Lightweight, zero-dependency, and extendable configuration management library for Go - omeid/uconfig
- 212Go implementation to calculate Levenshtein Distance.
https://github.com/agnivade/levenshtein
Go implementation to calculate Levenshtein Distance. - agnivade/levenshtein
- 213A simple command line notebook for programmers
https://github.com/dnote/dnote
A simple command line notebook for programmers. Contribute to dnote/dnote development by creating an account on GitHub.
- 214daichi-m/go18ds
https://github.com/daichi-m/go18ds
Contribute to daichi-m/go18ds development by creating an account on GitHub.
- 215Another CLI framework for Go. It works on my machine.
https://github.com/ukautz/clif
Another CLI framework for Go. It works on my machine. - ukautz/clif
- 216Bloom filters implemented in Go.
https://github.com/zhenjl/bloom
Bloom filters implemented in Go. Contribute to zentures/bloom development by creating an account on GitHub.
- 217Powerful scripting language & versatile interactive shell
https://github.com/elves/elvish
Powerful scripting language & versatile interactive shell - elves/elvish
- 218An archiving tool with an IM-style interface that prioritizes privacy and accessibility, integrated with various archival services including Internet Archive, archive.today, IPFS, Telegraph, and file systems.
https://github.com/wabarc/wayback
An archiving tool with an IM-style interface that prioritizes privacy and accessibility, integrated with various archival services including Internet Archive, archive.today, IPFS, Telegraph, and fi...
- 219Simple yet customizable bot framework written in Go.
https://github.com/oklahomer/go-sarah
Simple yet customizable bot framework written in Go. - oklahomer/go-sarah
- 220Slack bot core/framework written in Go with support for reactions to message updates/deletes
https://github.com/alexandre-normand/slackscot
Slack bot core/framework written in Go with support for reactions to message updates/deletes - alexandre-normand/slackscot
- 221A Go library for building command line applications.
https://github.com/devfacet/gocmd
A Go library for building command line applications. - devfacet/gocmd
- 222Lazy iterator implementation for Golang
https://github.com/yaa110/goterator
Lazy iterator implementation for Golang. Contribute to yaa110/goterator development by creating an account on GitHub.
- 223The standard library flag package with its missing features
https://github.com/posener/cmd
The standard library flag package with its missing features - posener/cmd
- 224Functions that operate on slices. Similar to functions from package strings or package bytes that have been adapted to work with slices.
https://github.com/srfrog/slices
Functions that operate on slices. Similar to functions from package strings or package bytes that have been adapted to work with slices. - srfrog/slices
- 225Go utility for loading configuration parameters from AWS SSM (Parameter Store)
https://github.com/ianlopshire/go-ssm-config
Go utility for loading configuration parameters from AWS SSM (Parameter Store) - ianlopshire/go-ssm-config
- 226Go library to simplify CLI workflow
https://github.com/yitsushi/go-commander
Go library to simplify CLI workflow. Contribute to yitsushi/go-commander development by creating an account on GitHub.
- 227Fast in-memory key:value store/cache with TTL
https://github.com/OrlovEvgeny/go-mcache
Fast in-memory key:value store/cache with TTL. Contribute to OrlovEvgeny/go-mcache development by creating an account on GitHub.
- 228omniparser: a native Golang ETL streaming parser and transform library for CSV, JSON, XML, EDI, text, etc.
https://github.com/jf-tech/omniparser
omniparser: a native Golang ETL streaming parser and transform library for CSV, JSON, XML, EDI, text, etc. - jf-tech/omniparser
- 229Go concurrent-safe, goroutine-safe, thread-safe queue
https://github.com/enriquebris/goconcurrentqueue
Go concurrent-safe, goroutine-safe, thread-safe queue - enriquebris/goconcurrentqueue
- 230A binary stream packer and unpacker
https://github.com/zhuangsirui/binpacker
A binary stream packer and unpacker. Contribute to zhuangsirui/binpacker development by creating an account on GitHub.
- 231GitHub Action for Go 1.18 fuzz testing
https://github.com/jidicula/go-fuzz-action
GitHub Action for Go 1.18 fuzz testing. Contribute to jidicula/go-fuzz-action development by creating an account on GitHub.
- 232Go 1.18+ generic tuple
https://github.com/barweiss/go-tuple
Go 1.18+ generic tuple. Contribute to barweiss/go-tuple development by creating an account on GitHub.
- 233Roaring bitmaps in Go (golang), used by InfluxDB, Bleve, DataDog
https://github.com/RoaringBitmap/roaring
Roaring bitmaps in Go (golang), used by InfluxDB, Bleve, DataDog - RoaringBitmap/roaring
- 234Composable, observable and performant config handling for Go for the distributed processing era
https://github.com/lalamove/konfig
Composable, observable and performant config handling for Go for the distributed processing era - lalamove/konfig
- 235A golang library for building interactive and accessible prompts with full support for windows and posix terminals.
https://github.com/go-survey/survey
A golang library for building interactive and accessible prompts with full support for windows and posix terminals. - AlecAivazis/survey
- 236Gota: DataFrames and data wrangling in Go (Golang)
https://github.com/kniren/gota
Gota: DataFrames and data wrangling in Go (Golang) - go-gota/gota
- 237Go SDK library and RPC client for the Solana Blockchain
https://github.com/gagliardetto/solana-go
Go SDK library and RPC client for the Solana Blockchain - gagliardetto/solana-go
- 238Go 1.18+ polymorphic generic containers and functions.
https://github.com/nwillc/genfuncs
Go 1.18+ polymorphic generic containers and functions. - nwillc/genfuncs
- 239A simple, fast, and fun package for building command line apps in Go
https://github.com/urfave/cli
A simple, fast, and fun package for building command line apps in Go - urfave/cli
- 240Go package that interfaces with AWS System Manager
https://github.com/PaddleHQ/go-aws-ssm
Go package that interfaces with AWS System Manager - PaddleHQ/go-aws-ssm
- 241A cross platform package that follows the XDG Standard
https://github.com/OpenPeeDeeP/xdg
A cross platform package that follows the XDG Standard - OpenPeeDeeP/xdg
- 242A Go library for an efficient implementation of a skip list: https://godoc.org/github.com/MauriceGit/skiplist
https://github.com/MauriceGit/skiplist
A Go library for an efficient implementation of a skip list: https://godoc.org/github.com/MauriceGit/skiplist - MauriceGit/skiplist
- 243The boss of http auth.
https://github.com/volatiletech/authboss
The boss of http auth. Contribute to volatiletech/authboss development by creating an account on GitHub.
- 244A Commander for modern Go CLI interactions
https://github.com/spf13/cobra
A Commander for modern Go CLI interactions. Contribute to spf13/cobra development by creating an account on GitHub.
- 245Yet another Bloomfilter implementation in Go, compatible with Java's Guava library
https://github.com/OldPanda/bloomfilter
Yet another Bloomfilter implementation in Go, compatible with Java's Guava library - OldPanda/bloomfilter
- 246Style definitions for nice terminal layouts 👄
https://github.com/charmbracelet/lipgloss
Style definitions for nice terminal layouts 👄. Contribute to charmbracelet/lipgloss development by creating an account on GitHub.
- 247go-test-coverage is tool and github action which reports issues when test coverage is below set threshold
https://github.com/vladopajic/go-test-coverage
go-test-coverage is tool and github action which reports issues when test coverage is below set threshold - GitHub - vladopajic/go-test-coverage: go-test-coverage is tool and github action which r...
- 248multi-shell multi-command argument completer
https://github.com/rsteube/carapace-bin
multi-shell multi-command argument completer. Contribute to carapace-sh/carapace-bin development by creating an account on GitHub.
- 249Go configuration made easy!
https://github.com/ian-kent/gofigure
Go configuration made easy! Contribute to ian-kent/gofigure development by creating an account on GitHub.
- 250Configure is a Go package that gives you easy configuration of your project through redundancy
https://github.com/paked/configure
Configure is a Go package that gives you easy configuration of your project through redundancy - paked/configure
- 251Package for creating interpreters
https://github.com/Zaba505/sand
Package for creating interpreters. Contribute to Zaba505/sand development by creating an account on GitHub.
- 252go command line option parser
https://github.com/jessevdk/go-flags
go command line option parser. Contribute to jessevdk/go-flags development by creating an account on GitHub.
- 253A simple logging interface that supports cross-platform color and concurrency.
https://github.com/dixonwille/wlog
A simple logging interface that supports cross-platform color and concurrency. - dixonwille/wlog
- 254HyperLogLog with lots of sugar (Sparse, LogLog-Beta bias correction and TailCut space reduction) brought to you by Axiom
https://github.com/axiomhq/hyperloglog
HyperLogLog with lots of sugar (Sparse, LogLog-Beta bias correction and TailCut space reduction) brought to you by Axiom - axiomhq/hyperloglog
- 255A Kafka log inspired in-memory and append-only data structure
https://github.com/embano1/memlog
A Kafka log inspired in-memory and append-only data structure - embano1/memlog
- 256Golang Get Environment Variables Package
https://github.com/nasermirzaei89/env
Golang Get Environment Variables Package. Contribute to nasermirzaei89/env development by creating an account on GitHub.
- 257An IPFS implementation in Go
https://github.com/ipfs/kubo
An IPFS implementation in Go. Contribute to ipfs/kubo development by creating an account on GitHub.
- 258An in-memory cache library for golang. It supports multiple eviction policies: LRU, LFU, ARC
https://github.com/bluele/gcache
An in-memory cache library for golang. It supports multiple eviction policies: LRU, LFU, ARC - bluele/gcache
- 259Pure Go CSS Preprocessor
https://github.com/yosssi/gcss
Pure Go CSS Preprocessor. Contribute to yosssi/gcss development by creating an account on GitHub.
- 260An opinionated configuration loading framework for Containerized and Cloud-Native applications.
https://github.com/sherifabdlnaby/configuro
An opinionated configuration loading framework for Containerized and Cloud-Native applications. - sherifabdlnaby/configuro
- 261Ready to use Slack bot for lazy developers: start Jenkins jobs, watch Jira tickets, watch pull requests with AI support...
https://github.com/innogames/slack-bot
Ready to use Slack bot for lazy developers: start Jenkins jobs, watch Jira tickets, watch pull requests with AI support... - innogames/slack-bot
- 262The magic YAML configuration framework for Go
https://github.com/romshark/yamagiconf
The magic YAML configuration framework for Go. Contribute to romshark/yamagiconf development by creating an account on GitHub.
- 263CLRS study. Codes are written with golang.
https://github.com/shady831213/algorithms
CLRS study. Codes are written with golang. Contribute to shady831213/algorithms development by creating an account on GitHub.
- 264Gitness is an Open Source developer platform with Source Control management, Continuous Integration and Continuous Delivery.
https://github.com/drone/drone
Gitness is an Open Source developer platform with Source Control management, Continuous Integration and Continuous Delivery. - harness/gitness
- 265Multi-String Pattern Matching Algorithm Using TrieNode
https://github.com/BlackRabbitt/mspm
Multi-String Pattern Matching Algorithm Using TrieNode - BlackRabbitt/mspm
- 266Climax is an alternative CLI with the human face
https://github.com/tucnak/climax
Climax is an alternative CLI with the human face. Contribute to tucnak/climax development by creating an account on GitHub.
- 267Pure Go termbox implementation
https://github.com/nsf/termbox-go
Pure Go termbox implementation. Contribute to nsf/termbox-go development by creating an account on GitHub.
- 268Shell library with powerful and modern UI, large feature set, and `.inputrc` support
https://github.com/reeflective/readline
Shell library with powerful and modern UI, large feature set, and `.inputrc` support - reeflective/readline
- 269A GitHub Action to track code coverage in your pull requests, with a beautiful HTML preview, for free.
https://github.com/gha-common/go-beautiful-html-coverage
A GitHub Action to track code coverage in your pull requests, with a beautiful HTML preview, for free. - gha-common/go-beautiful-html-coverage
- 270Simple, useful and opinionated config loader.
https://github.com/cristalhq/aconfig
Simple, useful and opinionated config loader. Contribute to cristalhq/aconfig development by creating an account on GitHub.
- 271Go native library for fast point tracking and K-Nearest queries
https://github.com/hailocab/go-geoindex
Go native library for fast point tracking and K-Nearest queries - hailocab/go-geoindex
- 272Efficient cache for gigabytes of data written in Go.
https://github.com/allegro/bigcache
Efficient cache for gigabytes of data written in Go. - allegro/bigcache
- 273A prefix tree implementation in go
https://github.com/viant/ptrie
A prefix tree implementation in go . Contribute to viant/ptrie development by creating an account on GitHub.
- 274floatdrop/2q
https://github.com/floatdrop/2q
Contribute to floatdrop/2q development by creating an account on GitHub.
- 275fractional api base on golang . golang math tools fractional molecular denominator 分数计算 分子 分母 运算
https://github.com/xxjwxc/gofal
fractional api base on golang . golang math tools fractional molecular denominator 分数计算 分子 分母 运算 - xxjwxc/gofal
- 276A caching library with advanced concurrency features designed to make I/O heavy applications robust and highly performant
https://github.com/creativecreature/sturdyc
A caching library with advanced concurrency features designed to make I/O heavy applications robust and highly performant - creativecreature/sturdyc
- 277Kelp is a free and open-source trading bot for the Stellar DEX and 100+ centralized exchanges
https://github.com/stellar/kelp
Kelp is a free and open-source trading bot for the Stellar DEX and 100+ centralized exchanges - stellar-deprecated/kelp
- 278A high performance cache for Go
https://github.com/maypok86/otter
A high performance cache for Go. Contribute to maypok86/otter development by creating an account on GitHub.
- 279gdcache is a pure non-intrusive cache library implemented by golang, you can use it to implement your own cache.
https://github.com/ulovecode/gdcache
gdcache is a pure non-intrusive cache library implemented by golang, you can use it to implement your own cache. - ulovecode/gdcache
- 280Open Source (Go) implementation of "Zanzibar: Google's Consistent, Global Authorization System". Ships gRPC, REST APIs, newSQL, and an easy and granular permission language. Supports ACL, RBAC, and other access models.
https://github.com/ory/keto
Open Source (Go) implementation of "Zanzibar: Google's Consistent, Global Authorization System". Ships gRPC, REST APIs, newSQL, and an easy and granular permission language. Supports ...
- 281A small flexible merge library in go
https://github.com/InVisionApp/conjungo
A small flexible merge library in go. Contribute to InVisionApp/conjungo development by creating an account on GitHub.
- 282Golang bindings for the Telegram Bot API
https://github.com/Syfaro/telegram-bot-api
Golang bindings for the Telegram Bot API. Contribute to go-telegram-bot-api/telegram-bot-api development by creating an account on GitHub.
- 283Go package implementing bitsets
https://github.com/bits-and-blooms/bitset
Go package implementing bitsets. Contribute to bits-and-blooms/bitset development by creating an account on GitHub.
- 284A comprehensive, reusable and efficient concurrent-safe generics utility functions and data structures library.
https://github.com/esimov/gogu
A comprehensive, reusable and efficient concurrent-safe generics utility functions and data structures library. - esimov/gogu
- 285A High Performance, Generic, thread-safe, zero-dependency, key-value, in-memory cache
https://github.com/mdaliyan/icache
A High Performance, Generic, thread-safe, zero-dependency, key-value, in-memory cache - mdaliyan/icache
- 286Bitset data structure
https://github.com/yourbasic/bit
Bitset data structure. Contribute to yourbasic/bit development by creating an account on GitHub.
- 287Idiomatic Go input parsing with subcommands, positional values, and flags at any position. No required project or package layout and no external dependencies.
https://github.com/integrii/flaggy
Idiomatic Go input parsing with subcommands, positional values, and flags at any position. No required project or package layout and no external dependencies. - integrii/flaggy
- 288Golang struct-tag based configfile and flag parsing
https://github.com/muir/nfigure
Golang struct-tag based configfile and flag parsing - muir/nfigure
- 289💁♀️Your new best friend powered by an artificial neural network
https://github.com/olivia-ai/olivia
💁♀️Your new best friend powered by an artificial neural network - olivia-ai/olivia
- 290Package ini provides INI file read and write functionality in Go
https://github.com/go-ini/ini
Package ini provides INI file read and write functionality in Go - go-ini/ini
- 291☁️ Live reload for Go apps
https://github.com/cosmtrek/air
☁️ Live reload for Go apps. Contribute to air-verse/air development by creating an account on GitHub.
- 292Go implementation of the XDG Base Directory Specification and XDG user directories
https://github.com/adrg/xdg
Go implementation of the XDG Base Directory Specification and XDG user directories - adrg/xdg
- 293Telegram Bot API library for Go
https://github.com/mymmrac/telego
Telegram Bot API library for Go. Contribute to mymmrac/telego development by creating an account on GitHub.
- 294golang bigcache with clustering as a library.
https://github.com/oaStuff/clusteredBigCache
golang bigcache with clustering as a library. Contribute to oaStuff/clusteredBigCache development by creating an account on GitHub.
- 295Flag is a simple but powerful command line option parsing library for Go support infinite level subcommand
https://github.com/cosiner/flag
Flag is a simple but powerful command line option parsing library for Go support infinite level subcommand - cosiner/flag
- 296Tag-based environment configuration for structs
https://github.com/codingconcepts/env
Tag-based environment configuration for structs. Contribute to codingconcepts/env development by creating an account on GitHub.
- 297Null Types, Safe primitive type conversion and fetching value from complex structures.
https://github.com/gurukami/typ
Null Types, Safe primitive type conversion and fetching value from complex structures. - gurukami/typ
- 298ini parser for golang
https://github.com/wlevene/ini
ini parser for golang. Contribute to wlevene/ini development by creating an account on GitHub.
- 299Library providing routines to merge and validate JSON, YAML and/or TOML files
https://github.com/the4thamigo-uk/conflate
Library providing routines to merge and validate JSON, YAML and/or TOML files - the4thamigo-uk/conflate
- 300Fully featured Go (golang) command line option parser with built-in auto-completion support.
https://github.com/DavidGamba/go-getoptions
Fully featured Go (golang) command line option parser with built-in auto-completion support. - DavidGamba/go-getoptions
- 301Build software better, together
https://github.com/yaronn/blessed-contrib.
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 302Build software better, together
https://github.com/gizak/termui.
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 303Package ring provides a high performance and thread safe Go implementation of a bloom filter.
https://github.com/TheTannerRyan/ring
Package ring provides a high performance and thread safe Go implementation of a bloom filter. - tannerryan/ring
- 304uilive is a go library for updating terminal output in realtime
https://github.com/gosuri/uilive
uilive is a go library for updating terminal output in realtime - gosuri/uilive
- 305🎨 Terminal color rendering library, support 8/16 colors, 256 colors, RGB color rendering output, support Print/Sprintf methods, compatible with Windows. GO CLI 控制台颜色渲染工具库,支持16色,256色,RGB色彩渲染输出,使用类似于 Print/Sprintf,兼容并支持 Windows 环境的色彩渲染
https://github.com/gookit/color
🎨 Terminal color rendering library, support 8/16 colors, 256 colors, RGB color rendering output, support Print/Sprintf methods, compatible with Windows. GO CLI 控制台颜色渲染工具库,支持16色,256色,RGB色彩渲染输出,使用类似于...
- 306Probabilistic set data structure
https://github.com/yourbasic/bloom
Probabilistic set data structure. Contribute to yourbasic/bloom development by creating an account on GitHub.
- 307An elegant and concurrent library for the Telegram bot API in Go.
https://github.com/NicoNex/echotron
An elegant and concurrent library for the Telegram bot API in Go. - NicoNex/echotron
- 308persistent storage for flags in go
https://github.com/schachmat/ingo
persistent storage for flags in go. Contribute to schachmat/ingo development by creating an account on GitHub.
- 309Golang terminal dashboard
https://github.com/gizak/termui
Golang terminal dashboard. Contribute to gizak/termui development by creating an account on GitHub.
- 310Hjson for Go
https://github.com/hjson/hjson-go
Hjson for Go. Contribute to hjson/hjson-go development by creating an account on GitHub.
- 311The simplest config loader for Go that reads/watches from file, env, flag and clouds (AWS, Azure, GCP).
https://github.com/nil-go/konf
The simplest config loader for Go that reads/watches from file, env, flag and clouds (AWS, Azure, GCP). - GitHub - nil-go/konf: The simplest config loader for Go that reads/watches from file, env,...
- 312bobg/subcmd
https://github.com/bobg/subcmd
Contribute to bobg/subcmd development by creating an account on GitHub.
- 313Instantiate/configure structs recursively, based on build environment. (YAML, TOML, JSON and env).
https://github.com/oblq/swap
Instantiate/configure structs recursively, based on build environment. (YAML, TOML, JSON and env). - oblq/swap
- 314Cuckoo Filter go implement, better than Bloom Filter, configurable and space optimized 布谷鸟过滤器的Go实现,优于布隆过滤器,可以定制化过滤器参数,并进行了空间优化
https://github.com/linvon/cuckoo-filter
Cuckoo Filter go implement, better than Bloom Filter, configurable and space optimized 布谷鸟过滤器的Go实现,优于布隆过滤器,可以定制化过滤器参数,并进行了空间优化 - linvon/cuckoo-filter
- 315Pure functions for slices.
https://github.com/twharmon/slices
Pure functions for slices. Contribute to twharmon/slices development by creating an account on GitHub.
- 316Woodpecker is a simple yet powerful CI/CD engine with great extensibility.
https://github.com/woodpecker-ci/woodpecker
Woodpecker is a simple yet powerful CI/CD engine with great extensibility. - woodpecker-ci/woodpecker
- 317go-up! A simple configuration library with recursive placeholders resolution and no magic.
https://github.com/ufoscout/go-up
go-up! A simple configuration library with recursive placeholders resolution and no magic. - ufoscout/go-up
- 318Embeddable and distributed in-memory alternative to Redis.
https://github.com/EchoVault/EchoVault
Embeddable and distributed in-memory alternative to Redis. - EchoVault/EchoVault
- 319Tag based configuration loader from different providers
https://github.com/milad-abbasi/gonfig
Tag based configuration loader from different providers - miladabc/gonfig
- 320Get up and running with Llama 3.1, Mistral, Gemma 2, and other large language models.
https://github.com/jmorganca/ollama
Get up and running with Llama 3.1, Mistral, Gemma 2, and other large language models. - ollama/ollama
- 321groupcache is a caching and cache-filling library, intended as a replacement for memcached in many cases.
https://github.com/golang/groupcache
groupcache is a caching and cache-filling library, intended as a replacement for memcached in many cases. - golang/groupcache
- 322Gocache is an in-memory key:value store/cache (similar to Memcached) library for Go, suitable for single-machine applications. it is safe with concorent go routine access.
https://github.com/yuseferi/gocache
Gocache is an in-memory key:value store/cache (similar to Memcached) library for Go, suitable for single-machine applications. it is safe with concorent go routine access. - yuseferi/gocache
- 323Frictionless way of managing project-specific commands
https://github.com/gopinath-langote/1build
Frictionless way of managing project-specific commands - gopinath-langote/1build
- 324Telebot is a Telegram bot framework in Go.
https://github.com/tucnak/telebot
Telebot is a Telegram bot framework in Go. Contribute to tucnak/telebot development by creating an account on GitHub.
- 325Simple and complete API for building command line applications in Go
https://github.com/teris-io/cli
Simple and complete API for building command line applications in Go - teris-io/cli
- 326Go implementation of C++ STL iterators and algorithms.
https://github.com/disksing/iter
Go implementation of C++ STL iterators and algorithms. - disksing/iter
- 327take bytes out of things easily ✨🍪
https://github.com/superwhiskers/crunch
take bytes out of things easily ✨🍪. Contribute to superwhiskers/crunch development by creating an account on GitHub.
- 328Go wrapper for libsass, the only Sass 3.5 compiler for Go
https://github.com/wellington/go-libsass
Go wrapper for libsass, the only Sass 3.5 compiler for Go - wellington/go-libsass
- 329Generate flags by parsing structures
https://github.com/octago/sflags
Generate flags by parsing structures. Contribute to octago/sflags development by creating an account on GitHub.
- 330Zero allocation Nullable structures in one library with handy conversion functions, marshallers and unmarshallers
https://github.com/kak-tus/nan
Zero allocation Nullable structures in one library with handy conversion functions, marshallers and unmarshallers - kak-tus/nan
- 331🤖 Go client library for accessing Telegram Bot API, with batteries for building complex bots included.
https://github.com/mr-linch/go-tg
🤖 Go client library for accessing Telegram Bot API, with batteries for building complex bots included. - mr-linch/go-tg
- 332📝 Go configuration manage(load,get,set,export). support JSON, YAML, TOML, Properties, INI, HCL, ENV and Flags. Multi file load, data override merge, parse ENV var. Go应用配置加载管理,支持多种格式,多文件加载,远程文件加载,支持数据合并,解析环境变量名
https://github.com/gookit/config
📝 Go configuration manage(load,get,set,export). support JSON, YAML, TOML, Properties, INI, HCL, ENV and Flags. Multi file load, data override merge, parse ENV var. Go应用配置加载管理,支持多种格式,多文件加载,远程文件加载,支持...
- 333☔️ A complete Go cache library that brings you multiple ways of managing your caches
https://github.com/eko/gocache
☔️ A complete Go cache library that brings you multiple ways of managing your caches - eko/gocache
- 334Probabilistic data structures for processing continuous, unbounded streams.
https://github.com/tylertreat/BoomFilters
Probabilistic data structures for processing continuous, unbounded streams. - tylertreat/BoomFilters
- 335Realize is the #1 Golang Task Runner which enhance your workflow by automating the most common tasks and using the best performing Golang live reloading.
https://github.com/tockins/realize
Realize is the #1 Golang Task Runner which enhance your workflow by automating the most common tasks and using the best performing Golang live reloading. - oxequa/realize
- 336define simple completions using a spec file
https://github.com/rsteube/carapace-spec
define simple completions using a spec file. Contribute to carapace-sh/carapace-spec development by creating an account on GitHub.
- 337The library provides a unified way to read configuration data from different sources, such as environment variables, command line flags, and configuration files.
https://github.com/dsbasko/go-cfg
The library provides a unified way to read configuration data from different sources, such as environment variables, command line flags, and configuration files. - dsbasko/go-cfg
- 338CLI - A package for building command line app with go
https://github.com/mkideal/cli
CLI - A package for building command line app with go - mkideal/cli
- 339A highly optimized double-ended queue
https://github.com/edwingeng/deque
A highly optimized double-ended queue. Contribute to edwingeng/deque development by creating an account on GitHub.
- 340Golang project boot
https://github.com/kcmvp/gob
Golang project boot. Contribute to kcmvp/gob development by creating an account on GitHub.
- 341Concurrency-safe Go caching library with expiration capabilities and access counters
https://github.com/muesli/cache2go
Concurrency-safe Go caching library with expiration capabilities and access counters - muesli/cache2go
- 342Yet Another CLi Spinner; providing over 80 easy to use and customizable terminal spinners for multiple OSes
https://github.com/theckman/yacspin
Yet Another CLi Spinner; providing over 80 easy to use and customizable terminal spinners for multiple OSes - theckman/yacspin
- 343A collection of useful, performant, and threadsafe Go datastructures.
https://github.com/Workiva/go-datastructures
A collection of useful, performant, and threadsafe Go datastructures. - Workiva/go-datastructures
- 344A lightweight yet powerful configuration manager for the Go programming language
https://github.com/golobby/config
A lightweight yet powerful configuration manager for the Go programming language - golobby/config
- 345Simple tables in terminal with Go
https://github.com/alexeyco/simpletable
Simple tables in terminal with Go. Contribute to alexeyco/simpletable development by creating an account on GitHub.
- 346An environment variable utility package
https://github.com/syntaqx/env
An environment variable utility package. Contribute to syntaqx/env development by creating an account on GitHub.
- 347Kong is a command-line parser for Go
https://github.com/alecthomas/kong
Kong is a command-line parser for Go. Contribute to alecthomas/kong development by creating an account on GitHub.
- 348go test output for humans
https://github.com/GoTestTools/gotestfmt
go test output for humans. Contribute to GoTestTools/gotestfmt development by creating an account on GitHub.
- 349🔍 Load environment variables into a config struct
https://github.com/junk1tm/env
🔍 Load environment variables into a config struct. Contribute to go-simpler/env development by creating an account on GitHub.
- 350Stellar | Blockchain Network for Smart Contracts, DeFi, Payments & Asset Tokenization
https://www.stellar.org/
Stellar Network: Discover an open-source blockchain platform equipped for DeFi with a secure smart contract platform, fast and affordable payments and enterprise-grade asset tokenization. Join our vibrant ecosystem of developers, entrepreneurs, and enterprises to pioneer the future of blockchain technology
- 351🛠 A configuration library for Go that parses environment variables, JSON files, and reloads automatically on SIGHUP.
https://github.com/joshbetz/config
🛠 A configuration library for Go that parses environment variables, JSON files, and reloads automatically on SIGHUP. - joshbetz/config
- 352🕸️ Go Implementation of the Polkadot Host
https://github.com/ChainSafe/gossamer
🕸️ Go Implementation of the Polkadot Host. Contribute to ChainSafe/gossamer development by creating an account on GitHub.
- 353Generic, zero-alloc, 100%-test covered Quadtree for golang
https://github.com/s0rg/quadtree
Generic, zero-alloc, 100%-test covered Quadtree for golang - s0rg/quadtree
- 354A Go recursive coverage testing tool
https://github.com/LawrenceWoodman/roveralls
A Go recursive coverage testing tool. Contribute to lawrencewoodman/roveralls development by creating an account on GitHub.
- 355Determine how intervals relate to each other.
https://github.com/francesconi/go-rampart
Determine how intervals relate to each other. Contribute to francesconi/go-rampart development by creating an account on GitHub.
- 356Set is a useful collection but there is no built-in implementation in Go lang.
https://github.com/zoumo/goset
Set is a useful collection but there is no built-in implementation in Go lang. - zoumo/goset
- 357A dead simple configuration manager for Go applications
https://github.com/tucnak/store
A dead simple configuration manager for Go applications - tucnak/store
- 358Go implementation of Count-Min-Log
https://github.com/seiflotfy/count-min-log
Go implementation of Count-Min-Log. Contribute to seiflotfy/count-min-log development by creating an account on GitHub.
- 359Python-like dictionaries for Go
https://github.com/srfrog/dict
Python-like dictionaries for Go. Contribute to srfrog/dict development by creating an account on GitHub.
- 360Enterprise-Grade Continuous Delivery & DevOps Automation Open Source Platform
https://github.com/ovh/cds
Enterprise-Grade Continuous Delivery & DevOps Automation Open Source Platform - ovh/cds
- 361⏪️ Go package providing multiple queue implementations. Developed in a thread-safe generic way.
https://github.com/adrianbrad/queue
⏪️ Go package providing multiple queue implementations. Developed in a thread-safe generic way. - adrianbrad/queue
- 362ops - build and run nanos unikernels
https://github.com/nanovms/ops
ops - build and run nanos unikernels. Contribute to nanovms/ops development by creating an account on GitHub.
- 363Golang Cache component - Multiple drivers
https://github.com/faabiosr/cachego
Golang Cache component - Multiple drivers. Contribute to faabiosr/cachego development by creating an account on GitHub.
- 364📚 String comparison and edit distance algorithms library, featuring : Levenshtein, LCS, Hamming, Damerau levenshtein (OSA and Adjacent transpositions algorithms), Jaro-Winkler, Cosine, etc...
https://github.com/hbollon/go-edlib
📚 String comparison and edit distance algorithms library, featuring : Levenshtein, LCS, Hamming, Damerau levenshtein (OSA and Adjacent transpositions algorithms), Jaro-Winkler, Cosine, etc... - hbo...
- 365bobg/hashsplit
http://github.com/bobg/hashsplit
Contribute to bobg/hashsplit development by creating an account on GitHub.
- 366Simple, extremely lightweight, extensible, configuration management library for Go. Support for JSON, TOML, YAML, env, command line, file, S3 etc. Alternative to viper.
https://github.com/knadh/koanf
Simple, extremely lightweight, extensible, configuration management library for Go. Support for JSON, TOML, YAML, env, command line, file, S3 etc. Alternative to viper. - knadh/koanf
- 367Go configuration with fangs
https://github.com/spf13/viper
Go configuration with fangs. Contribute to spf13/viper development by creating an account on GitHub.
- 368Build software better, together
https://github.com/shipt/plinko
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 369Collects many small inserts to ClickHouse and send in big inserts
https://github.com/nikepan/clickhouse-bulk
Collects many small inserts to ClickHouse and send in big inserts - nikepan/clickhouse-bulk
- 370[abandoned] Duktape JavaScript engine bindings for Go
https://github.com/olebedev/go-duktape
[abandoned] Duktape JavaScript engine bindings for Go - olebedev/go-duktape
- 371VictoriaMetrics: fast, cost-effective monitoring solution and time series database
https://github.com/VictoriaMetrics/VictoriaMetrics
VictoriaMetrics: fast, cost-effective monitoring solution and time series database - VictoriaMetrics/VictoriaMetrics
- 372GoCQL
https://gocql.github.io
GoCQL - Modern Cassandra client for the Go
- 373Transaction manager for GoLang
https://github.com/avito-tech/go-transaction-manager
Transaction manager for GoLang. Contribute to avito-tech/go-transaction-manager development by creating an account on GitHub.
- 374The lightweight, user-friendly, distributed relational database built on SQLite.
https://github.com/rqlite/rqlite
The lightweight, user-friendly, distributed relational database built on SQLite. - rqlite/rqlite
- 375Library for scanning data from a database into Go structs and more
https://github.com/georgysavva/scany
Library for scanning data from a database into Go structs and more - georgysavva/scany
- 376QOS Energy / squalus · GitLab
https://gitlab.com/qosenergy/squalus
A package to make performing SQL queries in Go easier and less error-prone.
- 377CLI-friendly package for pg migrations management.
https://github.com/lawzava/go-pg-migrate
CLI-friendly package for pg migrations management. - lawzava/go-pg-migrate
- 378Parquet
https://parquet.apache.org
The Apache Parquet Website
- 379pjrpc / pjrpc · GitLab
https://gitlab.com/pjrpc/pjrpc
Golang JSON RPC lib with Generator (Server + Client)
- 380Minimalistic database migration helper for Gorm ORM
https://github.com/go-gormigrate/gormigrate
Minimalistic database migration helper for Gorm ORM - go-gormigrate/gormigrate
- 381raylib
https://www.raylib.com/
raylib is a simple and easy-to-use library to enjoy videogames programming.
- 382🚀📦 Multi-tenancy support for GORM managed databases
https://github.com/bartventer/gorm-multitenancy
🚀📦 Multi-tenancy support for GORM managed databases - bartventer/gorm-multitenancy
- 383goal
https://codeberg.org/anaseto/goal
Goal, an embeddable scripting array language.
- 384Error handling library with readable stack traces and flexible formatting support 🎆
https://github.com/rotisserie/eris
Error handling library with readable stack traces and flexible formatting support 🎆 - rotisserie/eris
- 385Simple key-value store abstraction and implementations for Go (Redis, Consul, etcd, bbolt, BadgerDB, LevelDB, Memcached, DynamoDB, S3, PostgreSQL, MongoDB, CockroachDB and many more)
https://github.com/philippgille/gokv
Simple key-value store abstraction and implementations for Go (Redis, Consul, etcd, bbolt, BadgerDB, LevelDB, Memcached, DynamoDB, S3, PostgreSQL, MongoDB, CockroachDB and many more) - philippgille...
- 386Firebird RDBMS sql driver for Go (golang)
https://github.com/nakagami/firebirdsql
Firebird RDBMS sql driver for Go (golang). Contribute to nakagami/firebirdsql development by creating an account on GitHub.
- 387goworker is a Go-based background worker that runs 10 to 100,000* times faster than Ruby-based workers.
https://github.com/benmanns/goworker
goworker is a Go-based background worker that runs 10 to 100,000* times faster than Ruby-based workers. - benmanns/goworker
- 388A game server framework in Go (golang)
https://github.com/name5566/leaf
A game server framework in Go (golang). Contribute to name5566/leaf development by creating an account on GitHub.
- 389Inline styling for html mail in golang
https://github.com/vanng822/go-premailer
Inline styling for html mail in golang. Contribute to vanng822/go-premailer development by creating an account on GitHub.
- 390A Go microservices framework
https://github.com/micro/go-micro
A Go microservices framework. Contribute to micro/go-micro development by creating an account on GitHub.
- 391goriak - Go language driver for Riak KV
https://github.com/zegl/goriak
goriak - Go language driver for Riak KV. Contribute to zegl/goriak development by creating an account on GitHub.
- 392Type safe SQL builder with code generation and automatic query result data mapping
https://github.com/go-jet/jet
Type safe SQL builder with code generation and automatic query result data mapping - go-jet/jet
- 393ThreadLocal for Golang.
https://github.com/timandy/routine
ThreadLocal for Golang. Contribute to timandy/routine development by creating an account on GitHub.
- 394Limits the number of goroutines that are allowed to run concurrently
https://github.com/zenthangplus/goccm
Limits the number of goroutines that are allowed to run concurrently - zenthangplus/goccm
- 395A library that implements the outboxer pattern in go
https://github.com/italolelis/outboxer
A library that implements the outboxer pattern in go - italolelis/outboxer
- 396A Lua VM in Go
https://github.com/Shopify/go-lua
A Lua VM in Go. Contribute to Shopify/go-lua development by creating an account on GitHub.
- 397alcastle / dyndns · GitLab
https://gitlab.com/alcastle/dyndns
dyndns is s a simple app that updates dynamic DNS records for (one or many) domains, on a variety of providers via a simple config file. Works on...
- 398tozd / go / errors · GitLab
https://gitlab.com/tozd/go/errors
A Go package providing errors with a stack trace and structured details. https://pkg.go.dev/gitlab.com/tozd/go/errors
- 399Add a type for paths in Go.
https://github.com/jonchun/pathtype
Add a type for paths in Go. Contribute to jonchun/pathtype development by creating an account on GitHub.
- 400convert sql to elasticsearch DSL in golang(go)
https://github.com/cch123/elasticsql
convert sql to elasticsearch DSL in golang(go). Contribute to cch123/elasticsql development by creating an account on GitHub.
- 401A fast data generator that produces CSV files from generated relational data
https://github.com/codingconcepts/dg
A fast data generator that produces CSV files from generated relational data - codingconcepts/dg
- 402linkedin/goavro
https://github.com/linkedin/goavro
Contribute to linkedin/goavro development by creating an account on GitHub.
- 403Pure Go native and database/sql driver for YDB
https://github.com/ydb-platform/ydb-go-sdk
Pure Go native and database/sql driver for YDB. Contribute to ydb-platform/ydb-go-sdk development by creating an account on GitHub.
- 404Dead simple Go database migration library.
https://github.com/lopezator/migrator
Dead simple Go database migration library. Contribute to lopezator/migrator development by creating an account on GitHub.
- 405Cross-platform client for PostgreSQL databases
https://github.com/sosedoff/pgweb
Cross-platform client for PostgreSQL databases. Contribute to sosedoff/pgweb development by creating an account on GitHub.
- 406A tiny Redis server built with Golang, compatible with RESP protocols.
https://github.com/xgzlucario/rotom
A tiny Redis server built with Golang, compatible with RESP protocols. - xgzlucario/rotom
- 407Best microservices framework in Go, like alibaba Dubbo, but with more features, Scale easily. Try it. Test it. If you feel it's better, use it! 𝐉𝐚𝐯𝐚有𝐝𝐮𝐛𝐛𝐨, 𝐆𝐨𝐥𝐚𝐧𝐠有𝐫𝐩𝐜𝐱! build for cloud!
https://github.com/smallnest/rpcx
Best microservices framework in Go, like alibaba Dubbo, but with more features, Scale easily. Try it. Test it. If you feel it's better, use it! 𝐉𝐚𝐯𝐚有𝐝𝐮𝐛𝐛𝐨, 𝐆𝐨𝐥𝐚𝐧𝐠有𝐫𝐩𝐜𝐱! build for cloud! - small...
- 408A pool that spins up a given number of processes in advance and attaches stdin and stdout when needed. Very similar to FastCGI but works for any command.
https://github.com/hexdigest/execpool
A pool that spins up a given number of processes in advance and attaches stdin and stdout when needed. Very similar to FastCGI but works for any command. - hexdigest/execpool
- 409Easily build SQL queries in Go.
https://github.com/cristalhq/builq
Easily build SQL queries in Go. Contribute to cristalhq/builq development by creating an account on GitHub.
- 410🐝 A Highly Performant and easy to use goroutine pool for Go
https://github.com/loveleshsharma/gohive
🐝 A Highly Performant and easy to use goroutine pool for Go - loveleshsharma/gohive
- 411Dolt – Git for Data
https://github.com/dolthub/dolt
Dolt – Git for Data. Contribute to dolthub/dolt development by creating an account on GitHub.
- 412Golang client library for adding support for interacting and monitoring Celery workers, tasks and events.
https://github.com/svcavallar/celeriac.v1
Golang client library for adding support for interacting and monitoring Celery workers, tasks and events. - svcavallar/celeriac.v1
- 413Derives and generates mundane golang functions that you do not want to maintain yourself
https://github.com/awalterschulze/goderive
Derives and generates mundane golang functions that you do not want to maintain yourself - awalterschulze/goderive
- 414The only reasonable scripting engine for Go.
https://github.com/ichiban/prolog
The only reasonable scripting engine for Go. Contribute to ichiban/prolog development by creating an account on GitHub.
- 415Database schema evolution library for Go
https://github.com/GuiaBolso/darwin
Database schema evolution library for Go. Contribute to GuiaBolso/darwin development by creating an account on GitHub.
- 416LinDB is a scalable, high performance, high availability distributed time series database.
https://github.com/lindb/lindb
LinDB is a scalable, high performance, high availability distributed time series database. - lindb/lindb
- 417CockroachDB — the cloud native, distributed SQL database designed for high availability, effortless scale, and control over data placement.
https://github.com/cockroachdb/cockroach
CockroachDB — the cloud native, distributed SQL database designed for high availability, effortless scale, and control over data placement. - cockroachdb/cockroach
- 418Glow is an easy-to-use distributed computation system written in Go, similar to Hadoop Map Reduce, Spark, Flink, Storm, etc. I am also working on another similar pure Go system, https://github.com/chrislusf/gleam , which is more flexible and more performant.
https://github.com/chrislusf/glow
Glow is an easy-to-use distributed computation system written in Go, similar to Hadoop Map Reduce, Spark, Flink, Storm, etc. I am also working on another similar pure Go system, https://github.com/...
- 419Oracle driver for Go using database/sql
https://github.com/mattn/go-oci8
Oracle driver for Go using database/sql. Contribute to mattn/go-oci8 development by creating an account on GitHub.
- 420Golang source code parsing, usage like reflect package
https://github.com/wzshiming/gotype
Golang source code parsing, usage like reflect package - wzshiming/gotype
- 421A rudimentary implementation of a basic document (NoSQL) database in Go
https://github.com/HouzuoGuo/tiedot
A rudimentary implementation of a basic document (NoSQL) database in Go - HouzuoGuo/tiedot
- 422PHP bindings for the Go programming language (Golang)
https://github.com/deuill/go-php
PHP bindings for the Go programming language (Golang) - deuill/go-php
- 423A date and time parse and format library for Go.
https://github.com/deatil/go-datebin
A date and time parse and format library for Go. Contribute to deatil/go-datebin development by creating an account on GitHub.
- 424a db migration manager
https://github.com/rafaelespinoza/godfish
a db migration manager. Contribute to rafaelespinoza/godfish development by creating an account on GitHub.
- 425Scalable datastore for metrics, events, and real-time analytics
https://github.com/influxdb/influxdb
Scalable datastore for metrics, events, and real-time analytics - influxdata/influxdb
- 426Zero boilerplate database operations for Go
https://github.com/rocketlaunchr/dbq
Zero boilerplate database operations for Go. Contribute to rocketlaunchr/dbq development by creating an account on GitHub.
- 427Go library for the crypto NOWPayments API
https://github.com/matm/go-nowpayments
Go library for the crypto NOWPayments API. Contribute to matm/go-nowpayments development by creating an account on GitHub.
- 428Database migrations. CLI and Golang library.
https://github.com/golang-migrate/migrate
Database migrations. CLI and Golang library. Contribute to golang-migrate/migrate development by creating an account on GitHub.
- 429macOS Sleep/ Wake notifications in golang
https://github.com/prashantgupta24/mac-sleep-notifier
macOS Sleep/ Wake notifications in golang. Contribute to prashantgupta24/mac-sleep-notifier development by creating an account on GitHub.
- 430Matching Engine for Limit Order Book in Golang
https://github.com/i25959341/orderbook
Matching Engine for Limit Order Book in Golang. Contribute to i25959341/orderbook development by creating an account on GitHub.
- 431MySQL database migrator
https://github.com/larapulse/migrator
MySQL database migrator. Contribute to larapulse/migrator development by creating an account on GitHub.
- 432A Go package to help write migrations with go-pg/pg.
https://github.com/robinjoseph08/go-pg-migrations
A Go package to help write migrations with go-pg/pg. - robinjoseph08/go-pg-migrations
- 433Fluent SQL generation for golang
https://github.com/Masterminds/squirrel
Fluent SQL generation for golang. Contribute to Masterminds/squirrel development by creating an account on GitHub.
- 434RocksDB/LevelDB inspired key-value database in Go
https://github.com/cockroachdb/pebble
RocksDB/LevelDB inspired key-value database in Go. Contribute to cockroachdb/pebble development by creating an account on GitHub.
- 435Go copy directory recursively
https://github.com/otiai10/copy
Go copy directory recursively. Contribute to otiai10/copy development by creating an account on GitHub.
- 436A high performance NoSQL Database Server powered by Go
https://github.com/siddontang/ledisdb
A high performance NoSQL Database Server powered by Go - ledisdb/ledisdb
- 437Versatile Go code generator.
https://github.com/senselogic/GENERIS
Versatile Go code generator. Contribute to senselogic/GENERIS development by creating an account on GitHub.
- 438A tiny cross-platform Go library to hide/unhide files and directories
https://github.com/dastoori/higgs
A tiny cross-platform Go library to hide/unhide files and directories - dastoori/higgs
- 439html5tag generates html 5 tags
https://github.com/goradd/html5tag
html5tag generates html 5 tags. Contribute to goradd/html5tag development by creating an account on GitHub.
- 440Fast specialized time-series database for IoT, real-time internet connected devices and AI analytics.
https://github.com/unit-io/unitdb
Fast specialized time-series database for IoT, real-time internet connected devices and AI analytics. - unit-io/unitdb
- 441File system event notification library on steroids.
https://github.com/rjeczalik/notify
File system event notification library on steroids. - rjeczalik/notify
- 442🌧 BitTorrent client and library in Go
https://github.com/cenkalti/rain
🌧 BitTorrent client and library in Go. Contribute to cenkalti/rain development by creating an account on GitHub.
- 443Regatta is a distributed key-value store. It is Kubernetes friendly with emphasis on high read throughput and low operational cost.
https://github.com/jamf/regatta
Regatta is a distributed key-value store. It is Kubernetes friendly with emphasis on high read throughput and low operational cost. - jamf/regatta
- 444A Golang implemented Redis Server and Cluster. Go 语言实现的 Redis 服务器和分布式集群
https://github.com/hdt3213/godis
A Golang implemented Redis Server and Cluster. Go 语言实现的 Redis 服务器和分布式集群 - HDT3213/godis
- 445Go Memcached client library #golang
https://github.com/bradfitz/gomemcache/
Go Memcached client library #golang. Contribute to bradfitz/gomemcache development by creating an account on GitHub.
- 446A disk-backed key-value store.
https://github.com/peterbourgon/diskv
A disk-backed key-value store. Contribute to peterbourgon/diskv development by creating an account on GitHub.
- 447QR code generator (ASCII & PNG) for SEPA payments
https://github.com/jovandeginste/payme
QR code generator (ASCII & PNG) for SEPA payments. Contribute to jovandeginste/payme development by creating an account on GitHub.
- 448A database migration tool. Supports SQL migrations and Go functions.
https://github.com/pressly/goose
A database migration tool. Supports SQL migrations and Go functions. - GitHub - pressly/goose: A database migration tool. Supports SQL migrations and Go functions.
- 449Engo is an open-source 2D game engine written in Go.
https://github.com/EngoEngine/engo
Engo is an open-source 2D game engine written in Go. - EngoEngine/engo
- 450Go RPC framework with high-performance and strong-extensibility for building micro-services.
https://github.com/cloudwego/kitex
Go RPC framework with high-performance and strong-extensibility for building micro-services. - cloudwego/kitex
- 451Embedded schema migration package for Go
https://github.com/adlio/schema
Embedded schema migration package for Go. Contribute to adlio/schema development by creating an account on GitHub.
- 452LevelDB is a fast key-value storage library written at Google that provides an ordered mapping from string keys to string values.
https://github.com/google/leveldb
LevelDB is a fast key-value storage library written at Google that provides an ordered mapping from string keys to string values. - google/leveldb
- 453LevelDB key/value database in Go.
https://github.com/syndtr/goleveldb
LevelDB key/value database in Go. Contribute to syndtr/goleveldb development by creating an account on GitHub.
- 454Go library containing a collection of financial functions for time value of money (annuities), cash flow, interest rate conversions, bonds and depreciation calculations.
https://github.com/alpeb/go-finance
Go library containing a collection of financial functions for time value of money (annuities), cash flow, interest rate conversions, bonds and depreciation calculations. - alpeb/go-finance
- 455Go MySQL Driver is a MySQL driver for Go's (golang) database/sql package
https://github.com/go-sql-driver/mysql
Go MySQL Driver is a MySQL driver for Go's (golang) database/sql package - go-sql-driver/mysql
- 456:clock1: Date and Time - Golang Formatting Library
https://github.com/GuilhermeCaruso/kair
:clock1: Date and Time - Golang Formatting Library - GuilhermeCaruso/kair
- 457Embedded key-value store for read-heavy workloads written in Go
https://github.com/akrylysov/pogreb
Embedded key-value store for read-heavy workloads written in Go - akrylysov/pogreb
- 458A feature complete and high performance multi-group Raft library in Go.
https://github.com/lni/dragonboat
A feature complete and high performance multi-group Raft library in Go. - GitHub - lni/dragonboat: A feature complete and high performance multi-group Raft library in Go.
- 459Database wrapper that manage read write connections
https://github.com/andizzle/rwdb
Database wrapper that manage read write connections - andizzle/rwdb
- 460A Go distributed systems development framework
https://github.com/gmsec/micro
A Go distributed systems development framework. Contribute to gmsec/micro development by creating an account on GitHub.
- 461time range expression in cron style
https://github.com/1set/cronrange
time range expression in cron style. Contribute to 1set/cronrange development by creating an account on GitHub.
- 462Lightweight RESTful database engine based on stack data structures
https://github.com/fern4lvarez/piladb
Lightweight RESTful database engine based on stack data structures - fern4lvarez/piladb
- 463Fast disk usage analyzer with console interface written in Go
https://github.com/dundee/gdu
Fast disk usage analyzer with console interface written in Go - dundee/gdu
- 464Drop-in replacement for the standard library errors package and github.com/pkg/errors
https://github.com/emperror/errors
Drop-in replacement for the standard library errors package and github.com/pkg/errors - emperror/errors
- 465📥 An IMAP library for clients and servers
https://github.com/emersion/go-imap
📥 An IMAP library for clients and servers. Contribute to emersion/go-imap development by creating an account on GitHub.
- 466Microsoft ActiveX Object DataBase driver for go that using exp/sql
https://github.com/mattn/go-adodb
Microsoft ActiveX Object DataBase driver for go that using exp/sql - mattn/go-adodb
- 467SQL builder and query library for golang
https://github.com/doug-martin/goqu
SQL builder and query library for golang. Contribute to doug-martin/goqu development by creating an account on GitHub.
- 468create type dynamically in Golang
https://github.com/xiaoxin01/typeregistry
create type dynamically in Golang. Contribute to xiaoxin01/typeregistry development by creating an account on GitHub.
- 469PostgreSQL driver and toolkit for Go
https://github.com/jackc/pgx
PostgreSQL driver and toolkit for Go. Contribute to jackc/pgx development by creating an account on GitHub.
- 470Pure Go Postgres driver for database/sql
https://github.com/lib/pq
Pure Go Postgres driver for database/sql. Contribute to lib/pq development by creating an account on GitHub.
- 471The implementation of Persian (Solar Hijri) Calendar in Go
https://github.com/yaa110/go-persian-calendar
The implementation of Persian (Solar Hijri) Calendar in Go - yaa110/go-persian-calendar
- 472Open-Source ClickHouse http proxy and load balancer
https://github.com/Vertamedia/chproxy
Open-Source ClickHouse http proxy and load balancer - ContentSquare/chproxy
- 473Scalable, fault-tolerant application-layer sharding for Go applications
https://github.com/uber/ringpop-go
Scalable, fault-tolerant application-layer sharding for Go applications - uber/ringpop-go
- 474Key-value store for temporary items :memo:
https://github.com/rafaeljesus/tempdb
Key-value store for temporary items :memo:. Contribute to rafaeljesus/tempdb development by creating an account on GitHub.
- 475📧 Easy to use, yet comprehensive library for sending mails with Go
https://github.com/wneessen/go-mail
📧 Easy to use, yet comprehensive library for sending mails with Go - wneessen/go-mail
- 476Simple LISP in Go
https://github.com/jcla1/gisp
Simple LISP in Go. Contribute to jcla1/gisp development by creating an account on GitHub.
- 477The Prometheus monitoring system and time series database.
https://github.com/prometheus/prometheus
The Prometheus monitoring system and time series database. - prometheus/prometheus
- 478Take control of your data, connect with anything, and expose it anywhere through protocols such as HTTP, GraphQL, and gRPC.
https://github.com/jexia/semaphore
Take control of your data, connect with anything, and expose it anywhere through protocols such as HTTP, GraphQL, and gRPC. - jexia/semaphore
- 479Declarative pure-SQL schema management for MySQL and MariaDB
https://github.com/skeema/skeema
Declarative pure-SQL schema management for MySQL and MariaDB - skeema/skeema
- 480Adaptive Accrual Failure Detector
https://github.com/andy2046/failured
Adaptive Accrual Failure Detector. Contribute to andy2046/failured development by creating an account on GitHub.
- 481Hare is a nimble little database management system for Go.
https://github.com/jameycribbs/hare
Hare is a nimble little database management system for Go. - jameycribbs/hare
- 482🚀 A lightweight, framework-agnostic database migration tool.
https://github.com/amacneil/dbmate
🚀 A lightweight, framework-agnostic database migration tool. - amacneil/dbmate
- 483Effortlessly database dump with one command.
https://github.com/liweiyi88/onedump
Effortlessly database dump with one command. Contribute to liweiyi88/onedump development by creating an account on GitHub.
- 484Fast key-value DB in Go.
https://github.com/dgraph-io/badger
Fast key-value DB in Go. Contribute to dgraph-io/badger development by creating an account on GitHub.
- 485SQL schema migration tool for Go.
https://github.com/rubenv/sql-migrate
SQL schema migration tool for Go. Contribute to rubenv/sql-migrate development by creating an account on GitHub.
- 486A Go package for working with dates
https://github.com/rickb777/date
A Go package for working with dates. Contribute to rickb777/date development by creating an account on GitHub.
- 487An email and SMTP testing tool with API for developers
https://github.com/axllent/mailpit
An email and SMTP testing tool with API for developers - axllent/mailpit
- 488A hand-crafted 2D game library in Go
https://github.com/faiface/pixel
A hand-crafted 2D game library in Go. Contribute to faiface/pixel development by creating an account on GitHub.
- 489A probabilistic data structure service and storage
https://github.com/seiflotfy/skizze
A probabilistic data structure service and storage - seiflotfy/skizze
- 490go routine control, abstraction of the Main and some useful Executors.如果你不会管理Goroutine的话,用它
https://github.com/x-mod/routine
go routine control, abstraction of the Main and some useful Executors.如果你不会管理Goroutine的话,用它 - x-mod/routine
- 491Consistent hashing with bounded loads in Golang
https://github.com/buraksezer/consistent
Consistent hashing with bounded loads in Golang. Contribute to buraksezer/consistent development by creating an account on GitHub.
- 492A package to allow one to concurrently go through a filesystem with ease
https://github.com/dixonwille/skywalker
A package to allow one to concurrently go through a filesystem with ease - dixonwille/skywalker
- 493Database Abstraction Layer (dbal) for Go. Support SQL builder and get result easily (now only support mysql)
https://github.com/xujiajun/godbal
Database Abstraction Layer (dbal) for Go. Support SQL builder and get result easily (now only support mysql) - xujiajun/godbal
- 494An open-source graph database
https://github.com/google/cayley
An open-source graph database. Contribute to cayleygraph/cayley development by creating an account on GitHub.
- 495A distributed key-value store. On Disk. Able to grow or shrink without service interruption.
https://github.com/chrislusf/vasto
A distributed key-value store. On Disk. Able to grow or shrink without service interruption. - chrislusf/vasto
- 496RinkWorks fantasy name generator for golang
https://github.com/s0rg/fantasyname
RinkWorks fantasy name generator for golang. Contribute to s0rg/fantasyname development by creating an account on GitHub.
- 497Aerospike Client Go
https://github.com/aerospike/aerospike-client-go
Aerospike Client Go . Contribute to aerospike/aerospike-client-go development by creating an account on GitHub.
- 498🏋️ dbbench is a simple database benchmarking tool which supports several databases and own scripts
https://github.com/sj14/dbbench
🏋️ dbbench is a simple database benchmarking tool which supports several databases and own scripts - sj14/dbbench
- 499Immutable floating-point decimals for Go
https://github.com/govalues/decimal
Immutable floating-point decimals for Go. Contribute to govalues/decimal development by creating an account on GitHub.
- 500A cloud-native vector database, storage for next generation AI applications
https://github.com/milvus-io/milvus
A cloud-native vector database, storage for next generation AI applications - milvus-io/milvus
- 501sg: A simple standard SQL generator written in Go.
https://github.com/go-the-way/sg
sg: A simple standard SQL generator written in Go. - go-the-way/sg
- 502PostgreSQL ➕ REST, low-code, simplify and accelerate development, ⚡ instant, realtime, high-performance on any Postgres application, existing or new
https://github.com/prest/prest
PostgreSQL ➕ REST, low-code, simplify and accelerate development, ⚡ instant, realtime, high-performance on any Postgres application, existing or new - prest/prest
- 503Neo4j REST Client in golang
https://github.com/davemeehan/Neo4j-GO
Neo4j REST Client in golang. Contribute to davemeehan/Neo4j-GO development by creating an account on GitHub.
- 504Write your SQL queries in raw files with all benefits of modern IDEs, use them in an easy way inside your application with all the profit of compile time constants
https://github.com/HnH/qry
Write your SQL queries in raw files with all benefits of modern IDEs, use them in an easy way inside your application with all the profit of compile time constants - HnH/qry
- 505C99-compatible strftime formatter for use with Go time.Time instances.
https://github.com/awoodbeck/strftime
C99-compatible strftime formatter for use with Go time.Time instances. - awoodbeck/strftime
- 506Ruby-compatible strftime for golang
https://github.com/osteele/tuesday
Ruby-compatible strftime for golang. Contribute to osteele/tuesday development by creating an account on GitHub.
- 507Gentee - script programming language for automation. It uses VM and compiler written in Go (Golang).
https://github.com/gentee/gentee
Gentee - script programming language for automation. It uses VM and compiler written in Go (Golang). - gentee/gentee
- 508A Simple Yet Highly Powerful Package For Error Handling
https://github.com/SonicRoshan/falcon
A Simple Yet Highly Powerful Package For Error Handling - ThundR67/falcon
- 509Scriptable interpreter written in golang
https://github.com/mattn/anko
Scriptable interpreter written in golang. Contribute to mattn/anko development by creating an account on GitHub.
- 510A Go Mongo library based on the official MongoDB driver, featuring streamlined document operations, generic binding of structs to collections, built-in CRUD, aggregation, automated field updates, struct validation, hooks, and plugin-based programming.
https://github.com/chenmingyong0423/go-mongox
A Go Mongo library based on the official MongoDB driver, featuring streamlined document operations, generic binding of structs to collections, built-in CRUD, aggregation, automated field updates, s...
- 511Simplified distributed locking implementation using Redis
https://github.com/bsm/redislock
Simplified distributed locking implementation using Redis - bsm/redislock
- 512Fast and simple key/value store written using Go's standard library
https://github.com/recoilme/pudge
Fast and simple key/value store written using Go's standard library - recoilme/pudge
- 513A framework for building other frameworks
https://github.com/z5labs/bedrock
A framework for building other frameworks. Contribute to z5labs/bedrock development by creating an account on GitHub.
- 514Neo4j Rest API Client for Go lang
https://github.com/cihangir/neo4j
Neo4j Rest API Client for Go lang. Contribute to cihangir/neo4j development by creating an account on GitHub.
- 515Golang implementation of the Raft consensus protocol
https://github.com/hashicorp/raft
Golang implementation of the Raft consensus protocol - hashicorp/raft
- 516An unofficial Google Cloud Platform Go Datastore wrapper that adds caching using memcached. For App Engine Flexible, Compute Engine, Kubernetes Engine, and more.
https://github.com/defcronyke/godscache
An unofficial Google Cloud Platform Go Datastore wrapper that adds caching using memcached. For App Engine Flexible, Compute Engine, Kubernetes Engine, and more. - defcronyke/godscache
- 517levigo is a Go wrapper for LevelDB
https://github.com/jmhodges/levigo
levigo is a Go wrapper for LevelDB. Contribute to jmhodges/levigo development by creating an account on GitHub.
- 518Robust and flexible email library for Go
https://github.com/jordan-wright/email
Robust and flexible email library for Go. Contribute to jordan-wright/email development by creating an account on GitHub.
- 519Expression evaluation in golang
https://github.com/PaesslerAG/gval
Expression evaluation in golang. Contribute to PaesslerAG/gval development by creating an account on GitHub.
- 520💥 A lightweight DSL & ORM which helps you to write SQL in Go.
https://github.com/lqs/sqlingo
💥 A lightweight DSL & ORM which helps you to write SQL in Go. - lqs/sqlingo
- 521pop/soda at main · gobuffalo/pop
https://github.com/gobuffalo/pop/tree/master/soda
A Tasty Treat For All Your Database Needs. Contribute to gobuffalo/pop development by creating an account on GitHub.
- 522Helpfully Functional Go - A useful collection of Go utilities. Designed for programmer happiness.
https://github.com/tobyhede/go-underscore
Helpfully Functional Go - A useful collection of Go utilities. Designed for programmer happiness. - GitHub - tobyhede/go-underscore: Helpfully Functional Go - A useful collection of Go utilitie...
- 523Temporal Go SDK
https://github.com/temporalio/sdk-go
Temporal Go SDK. Contribute to temporalio/sdk-go development by creating an account on GitHub.
- 524A simple, fast, embeddable, persistent key/value store written in pure Go. It supports fully serializable transactions and many data structures such as list, set, sorted set.
https://github.com/xujiajun/nutsdb
A simple, fast, embeddable, persistent key/value store written in pure Go. It supports fully serializable transactions and many data structures such as list, set, sorted set. - nutsdb/nutsdb
- 525gosx-notifier is a Go framework for sending desktop notifications to OSX 10.8 or higher
https://github.com/deckarep/gosx-notifier
gosx-notifier is a Go framework for sending desktop notifications to OSX 10.8 or higher - deckarep/gosx-notifier
- 526Hotcoal - Secure your handcrafted SQL against injection
https://github.com/motrboat/hotcoal
Hotcoal - Secure your handcrafted SQL against injection - motrboat/hotcoal
- 527Go package for sharding databases ( Supports every ORM or raw SQL )
https://github.com/knocknote/octillery
Go package for sharding databases ( Supports every ORM or raw SQL ) - blastrain/octillery
- 528The official Go client for Elasticsearch
https://github.com/elastic/go-elasticsearch
The official Go client for Elasticsearch. Contribute to elastic/go-elasticsearch development by creating an account on GitHub.
- 529Energy is a framework developed by Go language based on CEF (Chromium Embedded Framework) for developing cross-platform desktop applications for Windows, Mac OS X, and Linux
https://github.com/energye/energy
Energy is a framework developed by Go language based on CEF (Chromium Embedded Framework) for developing cross-platform desktop applications for Windows, Mac OS X, and Linux - energye/energy
- 530Fluent SQL generation for golang
https://github.com/elgris/sqrl
Fluent SQL generation for golang. Contribute to elgris/sqrl development by creating an account on GitHub.
- 531Carbon for Golang, an extension for Time
https://github.com/uniplaces/carbon
Carbon for Golang, an extension for Time. Contribute to uniplaces/carbon development by creating an account on GitHub.
- 532LibraDB is a simple, persistent key/value store written in pure Go in less than 1000 lines for learning purposes.
https://github.com/amit-davidson/LibraDB
LibraDB is a simple, persistent key/value store written in pure Go in less than 1000 lines for learning purposes. - amit-davidson/LibraDB
- 533Lightweight package containing some ORM-like features and helpers for sqlite databases.
https://github.com/pupizoid/ormlite
Lightweight package containing some ORM-like features and helpers for sqlite databases. - pupizoid/ormlite
- 534copy files for humans
https://github.com/hugocarreira/go-decent-copy
copy files for humans. Contribute to hugocarreira/go-decent-copy development by creating an account on GitHub.
- 535Personal DDNS client with Digital Ocean Networking DNS as backend.
https://github.com/skibish/ddns
Personal DDNS client with Digital Ocean Networking DNS as backend. - skibish/ddns
- 536A library for reading and writing parquet files.
https://github.com/parsyl/parquet
A library for reading and writing parquet files. Contribute to parsyl/parquet development by creating an account on GitHub.
- 537✉️ A streaming Go library for the Internet Message Format and mail messages
https://github.com/emersion/go-message
✉️ A streaming Go library for the Internet Message Format and mail messages - emersion/go-message
- 538Hprose is a cross-language RPC. This project is Hprose for Golang.
https://github.com/hprose/hprose-golang
Hprose is a cross-language RPC. This project is Hprose for Golang. - hprose/hprose-golang
- 539Django style fixtures for Golang's excellent built-in database/sql library.
https://github.com/RichardKnop/go-fixtures
Django style fixtures for Golang's excellent built-in database/sql library. - RichardKnop/go-fixtures
- 540Simple and efficient error package
https://github.com/PumpkinSeed/errors
Simple and efficient error package . Contribute to PumpkinSeed/errors development by creating an account on GitHub.
- 541pg_timetable: Advanced scheduling for PostgreSQL
https://github.com/cybertec-postgresql/pg_timetable
pg_timetable: Advanced scheduling for PostgreSQL. Contribute to cybertec-postgresql/pg_timetable development by creating an account on GitHub.
- 542MetaCall: The ultimate polyglot programming experience.
https://github.com/metacall/core
MetaCall: The ultimate polyglot programming experience. - metacall/core
- 543SQL query builder for Go
https://github.com/twharmon/gosql
SQL query builder for Go. Contribute to twharmon/gosql development by creating an account on GitHub.
- 544Apache Calcite Go
https://github.com/apache/calcite-avatica-go
Apache Calcite Go. Contribute to apache/calcite-avatica-go development by creating an account on GitHub.
- 545Your ultimate Go microservices framework for the cloud-native era.
https://github.com/go-kratos/kratos
Your ultimate Go microservices framework for the cloud-native era. - go-kratos/kratos
- 546Golang wrapper for Exiftool : extract as much metadata as possible (EXIF, ...) from files (pictures, pdf, office documents, ...)
https://github.com/barasher/go-exiftool
Golang wrapper for Exiftool : extract as much metadata as possible (EXIF, ...) from files (pictures, pdf, office documents, ...) - barasher/go-exiftool
- 547Terminal stock ticker with live updates and position tracking
https://github.com/achannarasappa/ticker
Terminal stock ticker with live updates and position tracking - achannarasappa/ticker
- 548Read csv file from go using tags
https://github.com/artonge/go-csv-tag
Read csv file from go using tags. Contribute to artonge/go-csv-tag development by creating an account on GitHub.
- 549Simplify working with AWS DynamoDB.
https://github.com/twharmon/dynago
Simplify working with AWS DynamoDB. Contribute to twharmon/dynago development by creating an account on GitHub.
- 550Reflectionless data binding for Go's net/http (not actively maintained)
https://github.com/mholt/binding
Reflectionless data binding for Go's net/http (not actively maintained) - mholt/binding
- 551Embedded Go Database, the fast alternative to SQLite, gorm, etc.
https://github.com/objectbox/objectbox-go
Embedded Go Database, the fast alternative to SQLite, gorm, etc. - objectbox/objectbox-go
- 552A simple CSS parser and inliner in Go
https://github.com/aymerick/douceur
A simple CSS parser and inliner in Go. Contribute to aymerick/douceur development by creating an account on GitHub.
- 553database schema migrations on a per-library basis [Go]
https://github.com/muir/libschema
database schema migrations on a per-library basis [Go] - muir/libschema
- 554An embeddable implementation of the Ngaro Virtual Machine for Go programs
https://github.com/db47h/ngaro
An embeddable implementation of the Ngaro Virtual Machine for Go programs - db47h/ngaro
- 555Prep finds all SQL statements in a Go package and instruments db connection with prepared statements
https://github.com/hexdigest/prep
Prep finds all SQL statements in a Go package and instruments db connection with prepared statements - hexdigest/prep
- 556generate SQL migration schema from golang models and the current SQL schema.
https://github.com/sunary/sqlize
generate SQL migration schema from golang models and the current SQL schema. - sunary/sqlize
- 557The fully compliant, embeddable high-performance Go MQTT v5 server for IoT, smarthome, and pubsub
https://github.com/mochi-co/mqtt
The fully compliant, embeddable high-performance Go MQTT v5 server for IoT, smarthome, and pubsub - mochi-mqtt/server
- 558Datastore Connectivity for BigQuery in go
https://github.com/viant/bgc
Datastore Connectivity for BigQuery in go. Contribute to viant/bgc development by creating an account on GitHub.
- 559Fast SQL query builder for Go
https://github.com/leporo/sqlf
Fast SQL query builder for Go. Contribute to leporo/sqlf development by creating an account on GitHub.
- 560Open Sound Control (OSC) library for Golang. Implemented in pure Go.
https://github.com/hypebeast/go-osc
Open Sound Control (OSC) library for Golang. Implemented in pure Go. - hypebeast/go-osc
- 561The GitHub/GitLab for database DevOps. World's most advanced database DevOps and CI/CD for Developer, DBA and Platform Engineering teams.
https://github.com/bytebase/bytebase
The GitHub/GitLab for database DevOps. World's most advanced database DevOps and CI/CD for Developer, DBA and Platform Engineering teams. - bytebase/bytebase
- 562Golang package to manipulate time intervals.
https://github.com/SaidinWoT/timespan
Golang package to manipulate time intervals. Contribute to SaidinWoT/timespan development by creating an account on GitHub.
- 563Golang simple thread pool implementation
https://github.com/shettyh/threadpool
Golang simple thread pool implementation. Contribute to shettyh/threadpool development by creating an account on GitHub.
- 564A lightweight go library for parsing form data or json from an http.Request.
https://github.com/albrow/forms
A lightweight go library for parsing form data or json from an http.Request. - albrow/forms
- 565Sponge is a powerful Go development framework, it's easy to develop web and microservice projects.
https://github.com/zhufuyi/sponge
Sponge is a powerful Go development framework, it's easy to develop web and microservice projects. - zhufuyi/sponge
- 566Command line tool to generate idiomatic Go code for SQL databases supporting PostgreSQL, MySQL, SQLite, Oracle, and Microsoft SQL Server
https://github.com/knq/xo
Command line tool to generate idiomatic Go code for SQL databases supporting PostgreSQL, MySQL, SQLite, Oracle, and Microsoft SQL Server - xo/xo
- 567a cross platfrom Go library to place an icon and menu in the notification area
https://github.com/getlantern/systray
a cross platfrom Go library to place an icon and menu in the notification area - getlantern/systray
- 568Run functions in parallel :comet:
https://github.com/rafaeljesus/parallel-fn
Run functions in parallel :comet:. Contribute to rafaeljesus/parallel-fn development by creating an account on GitHub.
- 569A Go package to work with ISO 8601 week dates
https://github.com/stoewer/go-week
A Go package to work with ISO 8601 week dates. Contribute to stoewer/go-week development by creating an account on GitHub.
- 570Perl, but fluffy like a cat!
https://github.com/ian-kent/purl
Perl, but fluffy like a cat! Contribute to ian-kent/purl development by creating an account on GitHub.
- 571A lightweight document-oriented NoSQL database written in pure Golang.
https://github.com/ostafen/clover
A lightweight document-oriented NoSQL database written in pure Golang. - ostafen/clover
- 572go-jump: Jump consistent hashing
https://github.com/dgryski/go-jump
go-jump: Jump consistent hashing. Contribute to dgryski/go-jump development by creating an account on GitHub.
- 573A standard library for microservices.
https://github.com/go-kit/kit
A standard library for microservices. Contribute to go-kit/kit development by creating an account on GitHub.
- 574moss - a simple, fast, ordered, persistable, key-val storage library for golang
https://github.com/couchbase/moss
moss - a simple, fast, ordered, persistable, key-val storage library for golang - couchbase/moss
- 575Web and API based SMTP testing
https://github.com/mailhog/MailHog
Web and API based SMTP testing. Contribute to mailhog/MailHog development by creating an account on GitHub.
- 576Lightweight, fault-tolerant message streams.
https://github.com/liftbridge-io/liftbridge
Lightweight, fault-tolerant message streams. Contribute to liftbridge-io/liftbridge development by creating an account on GitHub.
- 577Manage your database schema as code
https://github.com/ariga/atlas
Manage your database schema as code. Contribute to ariga/atlas development by creating an account on GitHub.
- 578A currency computations package.
https://github.com/bnkamalesh/currency
A currency computations package. Contribute to bnkamalesh/currency development by creating an account on GitHub.
- 579🍱 yet another collection of go utilities & tools
https://github.com/1set/gut
🍱 yet another collection of go utilities & tools. Contribute to 1set/gut development by creating an account on GitHub.
- 580Embedded database for accounts transactions.
https://github.com/claygod/transaction
Embedded database for accounts transactions. Contribute to claygod/transaction development by creating an account on GitHub.
- 581High level go to Lua binder. Write less, do more.
https://github.com/alexeyco/binder
High level go to Lua binder. Write less, do more. Contribute to alexeyco/binder development by creating an account on GitHub.
- 582Lightweight Goroutine pool
https://github.com/ivpusic/grpool
Lightweight Goroutine pool. Contribute to ivpusic/grpool development by creating an account on GitHub.
- 583Attach hooks to any database/sql driver
https://github.com/qustavo/sqlhooks
Attach hooks to any database/sql driver. Contribute to qustavo/sqlhooks development by creating an account on GitHub.
- 584Draw a polygon on the map or paste a geoJSON and explore how the s2.RegionCoverer covers it with S2 cells depending on the min and max levels
https://github.com/pantrif/s2-geojson
Draw a polygon on the map or paste a geoJSON and explore how the s2.RegionCoverer covers it with S2 cells depending on the min and max levels - pantrif/s2-geojson
- 585robfig/bind
https://github.com/robfig/bind
Contribute to robfig/bind development by creating an account on GitHub.
- 586Go module for encoding structs into URL query parameters
https://github.com/sonh/qs
Go module for encoding structs into URL query parameters - sonh/qs
- 587Simple in-memory job queue for Golang using worker-based dispatching
https://github.com/borderstech/artifex
Simple in-memory job queue for Golang using worker-based dispatching - mborders/artifex
- 588:white_check_mark: A Go library for email verification without sending any emails.
https://github.com/AfterShip/email-verifier
:white_check_mark: A Go library for email verification without sending any emails. - AfterShip/email-verifier
- 589A Binary Memcached client for Go with support for sharding using consistent hashing, along with SASL.
https://github.com/aliexpressru/gomemcached
A Binary Memcached client for Go with support for sharding using consistent hashing, along with SASL. - aliexpressru/gomemcached
- 590Currency handling for Go.
https://github.com/bojanz/currency
Currency handling for Go. Contribute to bojanz/currency development by creating an account on GitHub.
- 591MailHog SMTP Protocol
https://github.com/mailhog/smtp
MailHog SMTP Protocol. Contribute to mailhog/smtp development by creating an account on GitHub.
- 592Dragonfly is an open source P2P-based file distribution and image acceleration system. It is hosted by the Cloud Native Computing Foundation (CNCF) as an Incubating Level Project.
https://github.com/dragonflyoss/Dragonfly2
Dragonfly is an open source P2P-based file distribution and image acceleration system. It is hosted by the Cloud Native Computing Foundation (CNCF) as an Incubating Level Project. - dragonflyoss/Dr...
- 593Build software better, together
https://github.com/todotxt/todo.txt.
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 594Make functions return a channel for parallel processing via go routines.
https://github.com/ddelizia/channelify
Make functions return a channel for parallel processing via go routines. - ddelizia/channelify
- 595🍡 HTTP Input for Go - HTTP Request from/to Go Struct (Bi-directional Data Binding between Go Struct and http.Request)
https://github.com/ggicci/httpin
🍡 HTTP Input for Go - HTTP Request from/to Go Struct (Bi-directional Data Binding between Go Struct and http.Request) - ggicci/httpin
- 596:clock8: Better time duration formatting in Go!
https://github.com/hako/durafmt
:clock8: Better time duration formatting in Go! . Contribute to hako/durafmt development by creating an account on GitHub.
- 597Golang errors with stack trace and source fragments.
https://github.com/ztrue/tracerr
Golang errors with stack trace and source fragments. - ztrue/tracerr
- 598Build your own Game-Engine based on the Entity Component System concept in Golang.
https://github.com/andygeiss/ecs
Build your own Game-Engine based on the Entity Component System concept in Golang. - andygeiss/ecs
- 599Golang implemented Redis RDB parser for secondary development and memory analysis
https://github.com/HDT3213/rdb
Golang implemented Redis RDB parser for secondary development and memory analysis - HDT3213/rdb
- 600TiDB is an open-source, cloud-native, distributed, MySQL-Compatible database for elastic scale and real-time analytics. Try AI-powered Chat2Query free at : https://www.pingcap.com/tidb-serverless/
https://github.com/pingcap/tidb
TiDB is an open-source, cloud-native, distributed, MySQL-Compatible database for elastic scale and real-time analytics. Try AI-powered Chat2Query free at : https://www.pingcap.com/tidb-serverless/ ...
- 601BQB is a lightweight and easy to use query builder that works with sqlite, mysql, mariadb, postgres, and others.
https://github.com/nullism/bqb
BQB is a lightweight and easy to use query builder that works with sqlite, mysql, mariadb, postgres, and others. - GitHub - nullism/bqb: BQB is a lightweight and easy to use query builder that wor...
- 602Lightweight, fast and reliable key/value storage engine based on Bitcask.
https://github.com/roseduan/rosedb
Lightweight, fast and reliable key/value storage engine based on Bitcask. - rosedblabs/rosedb
- 603kirillDanshin/nulltime
https://github.com/kirillDanshin/nulltime
Contribute to kirillDanshin/nulltime development by creating an account on GitHub.
- 604:electric_plug: Raspberry Pi GPIO library for go-lang
https://github.com/stianeikeland/go-rpio
:electric_plug: Raspberry Pi GPIO library for go-lang - stianeikeland/go-rpio
- 605a golang library for sql builder
https://github.com/didi/gendry
a golang library for sql builder. Contribute to didi/gendry development by creating an account on GitHub.
- 606A library that makes it easier to send email via SMTP.
https://github.com/valord577/mailx
A library that makes it easier to send email via SMTP. - valord577/mailx
- 607CSRF protection middleware for Go.
https://github.com/justinas/nosurf
CSRF protection middleware for Go. Contribute to justinas/nosurf development by creating an account on GitHub.
- 608A safe way to execute functions asynchronously, recovering them in case of panic. It also provides an error stack aiming to facilitate fail causes discovery.
https://github.com/studiosol/async
A safe way to execute functions asynchronously, recovering them in case of panic. It also provides an error stack aiming to facilitate fail causes discovery. - StudioSol/async
- 609The MongoDB driver for Go
https://github.com/globalsign/mgo
The MongoDB driver for Go. Contribute to globalsign/mgo development by creating an account on GitHub.
- 610Go package for dealing with EU VAT. Does VAT number validation & rates retrieval.
https://github.com/dannyvankooten/vat
Go package for dealing with EU VAT. Does VAT number validation & rates retrieval. - dannyvankooten/vat
- 611📧 Golang Email address validator
https://github.com/go-email-validator/go-email-validator
📧 Golang Email address validator. Contribute to go-email-validator/go-email-validator development by creating an account on GitHub.
- 612WeScale is a Modern MySQL proxy that supports read-write-split, read-after-write-consistency, load balancing and OnlineDDL.
https://github.com/wesql/wescale
WeScale is a Modern MySQL proxy that supports read-write-split, read-after-write-consistency, load balancing and OnlineDDL. - wesql/wescale
- 613Go client library for Pilosa
https://github.com/pilosa/go-pilosa
Go client library for Pilosa. Contribute to FeatureBaseDB/go-pilosa development by creating an account on GitHub.
- 614A goroutine pool for Go
https://github.com/Jeffail/tunny
A goroutine pool for Go. Contribute to Jeffail/tunny development by creating an account on GitHub.
- 615AsyncJob is an asynchronous queue job manager with light code, clear and speed. I hope so ! 😬
https://github.com/lab210-dev/async-job
AsyncJob is an asynchronous queue job manager with light code, clear and speed. I hope so ! 😬 - kitstack/async-job
- 616EliasDB a graph-based database.
https://github.com/krotik/eliasdb
EliasDB a graph-based database. Contribute to krotik/eliasdb development by creating an account on GitHub.
- 617Go bindings for Lua C API - in progress
https://github.com/aarzilli/golua
Go bindings for Lua C API - in progress. Contribute to aarzilli/golua development by creating an account on GitHub.
- 618MySQL Backed Locking Primitive
https://github.com/sanketplus/go-mysql-lock
MySQL Backed Locking Primitive. Contribute to sanketplus/go-mysql-lock development by creating an account on GitHub.
- 619Datastore Connectivity in go
https://github.com/viant/dsc
Datastore Connectivity in go. Contribute to viant/dsc development by creating an account on GitHub.
- 620A Go library for master-less peer-to-peer autodiscovery and RPC between HTTP services
https://github.com/ursiform/sleuth
A Go library for master-less peer-to-peer autodiscovery and RPC between HTTP services - ursiform/sleuth
- 621Golang client for redislabs' ReJSON module with support for multilple redis clients (redigo, go-redis)
https://github.com/nitishm/go-rejson
Golang client for redislabs' ReJSON module with support for multilple redis clients (redigo, go-redis) - nitishm/go-rejson
- 622A pluggable, high-performance RPC framework written in golang
https://github.com/trpc-group/trpc-go
A pluggable, high-performance RPC framework written in golang - trpc-group/trpc-go
- 623Starlark in Go: the Starlark configuration language, implemented in Go
https://github.com/google/starlark-go
Starlark in Go: the Starlark configuration language, implemented in Go - google/starlark-go
- 624Apache AVRO for go
https://github.com/khezen/avro
Apache AVRO for go. Contribute to khezen/avro development by creating an account on GitHub.
- 625A drop-in replacement for Go errors, with some added sugar! Unwrap user-friendly messages, HTTP status code, easy wrapping with multiple error types.
https://github.com/bnkamalesh/errors
A drop-in replacement for Go errors, with some added sugar! Unwrap user-friendly messages, HTTP status code, easy wrapping with multiple error types. - bnkamalesh/errors
- 626TryLock support on read-write lock for Golang
https://github.com/subchen/go-trylock
TryLock support on read-write lock for Golang. Contribute to subchen/go-trylock development by creating an account on GitHub.
- 627Structured Concurrency in Go
https://github.com/arunsworld/nursery
Structured Concurrency in Go. Contribute to arunsworld/nursery development by creating an account on GitHub.
- 628A concurrent toolkit to help execute funcs concurrently in an efficient and safe way. It supports specifying the overall timeout to avoid blocking.
https://github.com/ITcathyh/conexec
A concurrent toolkit to help execute funcs concurrently in an efficient and safe way. It supports specifying the overall timeout to avoid blocking. - ITcathyh/conexec
- 629Generate type-safe Go converters by simply defining an interface
https://github.com/jmattheis/goverter
Generate type-safe Go converters by simply defining an interface - jmattheis/goverter
- 630naive go bindings to the CPython2 C-API
https://github.com/sbinet/go-python
naive go bindings to the CPython2 C-API. Contribute to sbinet/go-python development by creating an account on GitHub.
- 631An PDU implementation in Go
https://github.com/pdupub/go-pdu
An PDU implementation in Go. Contribute to pdupub/go-pdu development by creating an account on GitHub.
- 632Synchronization and asynchronous computation package for Go
https://github.com/reugn/async
Synchronization and asynchronous computation package for Go - reugn/async
- 633Mongo Go Models (mgm) is a fast and simple MongoDB ODM for Go (based on official Mongo Go Driver)
https://github.com/kamva/mgm
Mongo Go Models (mgm) is a fast and simple MongoDB ODM for Go (based on official Mongo Go Driver) - Kamva/mgm
- 634Type safe SQL query builder and struct mapper for Go
https://github.com/bokwoon95/go-structured-query
Type safe SQL query builder and struct mapper for Go - bokwoon95/go-structured-query
- 635Compute message digest for large files in Go
https://github.com/codingsince1985/checksum
Compute message digest for large files in Go. Contribute to codingsince1985/checksum development by creating an account on GitHub.
- 636Finance related Go functions (e.g. exchange rates, VAT number checking, …)
https://github.com/pieterclaerhout/go-finance
Finance related Go functions (e.g. exchange rates, VAT number checking, …) - pieterclaerhout/go-finance
- 637Lightweight Golang driver for ArangoDB
https://github.com/solher/arangolite
Lightweight Golang driver for ArangoDB. Contribute to solher/arangolite development by creating an account on GitHub.
- 638Wa is the best Wasm language
https://github.com/wa-lang/wa
Wa is the best Wasm language. Contribute to wa-lang/wa development by creating an account on GitHub.
- 639Build software better, together
https://github.com/zeromq/libzmq.
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 640Cross-platform Go library to place an icon in the host operating system's taskbar.
https://github.com/shurcooL/trayhost
Cross-platform Go library to place an icon in the host operating system's taskbar. - shurcooL/trayhost
- 641Parser for todo.txt files in Go ✅
https://github.com/1set/todotxt
Parser for todo.txt files in Go ✅. Contribute to 1set/todotxt development by creating an account on GitHub.
- 642Arbitrary-precision fixed-point decimal numbers in Go
https://github.com/shopspring/decimal
Arbitrary-precision fixed-point decimal numbers in Go - shopspring/decimal
- 643Go WorkerPool aims to control heavy Go Routines
https://github.com/zenthangplus/go-workerpool
Go WorkerPool aims to control heavy Go Routines. Contribute to zenthangplus/go-workerpool development by creating an account on GitHub.
- 644Conversion utilities between H3 indexes and GeoJSON
https://github.com/mmadfox/go-geojson2h3
Conversion utilities between H3 indexes and GeoJSON - mmadfox/go-geojson2h3
- 645Go package for calculating the sunrise and sunset times for a given location
https://github.com/nathan-osman/go-sunrise
Go package for calculating the sunrise and sunset times for a given location - nathan-osman/go-sunrise
- 646A library to interact with Elasticsearch in Go!
https://github.com/OwnLocal/goes
A library to interact with Elasticsearch in Go! Contribute to OwnLocal/goes development by creating an account on GitHub.
- 647Golang package for send email. Support keep alive connection, TLS and SSL. Easy for bulk SMTP.
https://github.com/xhit/go-simple-mail
Golang package for send email. Support keep alive connection, TLS and SSL. Easy for bulk SMTP. - xhit/go-simple-mail
- 648Payment abstraction library - one interface for multiple payment processors ( inspired by Ruby's ActiveMerchant )
https://github.com/BoltApp/sleet
Payment abstraction library - one interface for multiple payment processors ( inspired by Ruby's ActiveMerchant ) - BoltApp/sleet
- 649Lightweight SMTP client written in Go
https://github.com/hectane/hectane
Lightweight SMTP client written in Go. Contribute to hectane/hectane development by creating an account on GitHub.
- 650A collection of tools for Golang
https://github.com/nikhilsaraf/go-tools
A collection of tools for Golang. Contribute to nikhilsaraf/go-tools development by creating an account on GitHub.
- 651Go bindings for ForestDB
https://github.com/couchbase/goforestdb
Go bindings for ForestDB. Contribute to couchbase/goforestdb development by creating an account on GitHub.
- 652A fast script language for Go
https://github.com/d5/tengo
A fast script language for Go. Contribute to d5/tengo development by creating an account on GitHub.
- 653Code generation tools for Go.
https://github.com/rjeczalik/interfaces
Code generation tools for Go. Contribute to rjeczalik/interfaces development by creating an account on GitHub.
- 654Trims, sanitizes & scrubs data based on struct tags (go, golang)
https://github.com/leebenson/conform
Trims, sanitizes & scrubs data based on struct tags (go, golang) - leebenson/conform
- 655A library built to provide support for defining service health for golang services. It allows you to register async health checks for your dependencies and the service itself, provides a health endpoint that exposes their status, and health metrics.
https://github.com/AppsFlyer/go-sundheit
A library built to provide support for defining service health for golang services. It allows you to register async health checks for your dependencies and the service itself, provides a health end...
- 656Generic Golang Key/Value trait for AWS storage services
https://github.com/fogfish/dynamo
Generic Golang Key/Value trait for AWS storage services - fogfish/dynamo
- 657Reduce debugging time. Use static & stack-trace analysis to identify the error immediately.
https://github.com/snwfdhmp/errlog
Reduce debugging time. Use static & stack-trace analysis to identify the error immediately. - snwfdhmp/errlog
- 658.NET LINQ capabilities in Go
https://github.com/ahmetalpbalkan/go-linq
.NET LINQ capabilities in Go. Contribute to ahmetb/go-linq development by creating an account on GitHub.
- 659A free and open source framework for building powerful, fast, elegant 2D and 3D apps that run on macOS, Windows, Linux, iOS, Android, and the web with a single Go codebase, allowing you to Code Once, Run Everywhere.
https://github.com/cogentcore/core
A free and open source framework for building powerful, fast, elegant 2D and 3D apps that run on macOS, Windows, Linux, iOS, Android, and the web with a single Go codebase, allowing you to Code Onc...
- 660gpool - a generic context-aware resizable goroutines pool to bound concurrency based on semaphore.
https://github.com/Sherifabdlnaby/gpool
gpool - a generic context-aware resizable goroutines pool to bound concurrency based on semaphore. - GitHub - sherifabdlnaby/gpool: gpool - a generic context-aware resizable goroutines pool to bou...
- 661dht is used by anacrolix/torrent, and is intended for use as a library in other projects both torrent related and otherwise
https://github.com/anacrolix/dht
dht is used by anacrolix/torrent, and is intended for use as a library in other projects both torrent related and otherwise - anacrolix/dht
- 662Hunch provides functions like: All, First, Retry, Waterfall etc., that makes asynchronous flow control more intuitive.
https://github.com/AaronJan/Hunch
Hunch provides functions like: All, First, Retry, Waterfall etc., that makes asynchronous flow control more intuitive. - AaronJan/Hunch
- 663✨ Yet another Go wrapper for Starlark that simplifies usage, offers data conversion and useful Starlark libraries
https://github.com/1set/starlet
✨ Yet another Go wrapper for Starlark that simplifies usage, offers data conversion and useful Starlark libraries - 1set/starlet
- 664SurrealDB SDK for Golang
https://github.com/surrealdb/surrealdb.go
SurrealDB SDK for Golang. Contribute to surrealdb/surrealdb.go development by creating an account on GitHub.
- 665Solution for accumulation of events and their subsequent processing.
https://github.com/nar10z/go-accumulator
Solution for accumulation of events and their subsequent processing. - nar10z/go-accumulator
- 666Publish Your GIS Data(Vector Data) to PostGIS and Geoserver
https://github.com/hishamkaram/gismanager
Publish Your GIS Data(Vector Data) to PostGIS and Geoserver - hishamkaram/gismanager
- 667Bigfile -- a file transfer system that supports http, rpc and ftp protocol https://bigfile.site
https://github.com/bigfile/bigfile
Bigfile -- a file transfer system that supports http, rpc and ftp protocol https://bigfile.site - GitHub - bigfile/bigfile: Bigfile -- a file transfer system that supports http, rpc and ftp pro...
- 668BuntDB is an embeddable, in-memory key/value database for Go with custom indexing and geospatial support
https://github.com/tidwall/buntdb
BuntDB is an embeddable, in-memory key/value database for Go with custom indexing and geospatial support - tidwall/buntdb
- 669Simple golang error handling with classification primitives.
https://github.com/neuronlabs/errors
Simple golang error handling with classification primitives. - neuronlabs/errors
- 670🚦 Semaphore pattern implementation with timeout of lock/unlock operations.
https://github.com/kamilsk/semaphore
🚦 Semaphore pattern implementation with timeout of lock/unlock operations. - kamilsk/semaphore
- 671Microsoft SQL server driver written in go language
https://github.com/denisenkom/go-mssqldb
Microsoft SQL server driver written in go language - denisenkom/go-mssqldb
- 672The Official Twilio SendGrid Golang API Library
https://github.com/sendgrid/sendgrid-go
The Official Twilio SendGrid Golang API Library. Contribute to sendgrid/sendgrid-go development by creating an account on GitHub.
- 673Simple pgx wrapper to execute and scan query results
https://github.com/alexeyco/pig
Simple pgx wrapper to execute and scan query results - alexeyco/pig
- 674Expression language and expression evaluation for Go
https://github.com/antonmedv/expr
Expression language and expression evaluation for Go - expr-lang/expr
- 675Unlimited job queue for go, using a pool of concurrent workers processing the job queue entries
https://github.com/dirkaholic/kyoo
Unlimited job queue for go, using a pool of concurrent workers processing the job queue entries - dirkaholic/kyoo
- 676Go bindings for GTK3
https://github.com/gotk3/gotk3
Go bindings for GTK3. Contribute to gotk3/gotk3 development by creating an account on GitHub.
- 677🧧 Fixed-Point Decimal Money
https://github.com/nikolaydubina/fpmoney
🧧 Fixed-Point Decimal Money. Contribute to nikolaydubina/fpmoney development by creating an account on GitHub.
- 678A revamped Google's jump consistent hash
https://github.com/edwingeng/doublejump
A revamped Google's jump consistent hash. Contribute to edwingeng/doublejump development by creating an account on GitHub.
- 679Simple 2D game prototyping framework.
https://github.com/gonutz/prototype
Simple 2D game prototyping framework. Contribute to gonutz/prototype development by creating an account on GitHub.
- 680Datastore Connectivity for Aerospike for go
https://github.com/viant/asc
Datastore Connectivity for Aerospike for go. Contribute to viant/asc development by creating an account on GitHub.
- 681DKIM package for golang
https://github.com/toorop/go-dkim
DKIM package for golang. Contribute to toorop/go-dkim development by creating an account on GitHub.
- 682Go errors but structured and composable. Fault provides an extensible yet ergonomic mechanism for wrapping errors.
https://github.com/Southclaws/fault
Go errors but structured and composable. Fault provides an extensible yet ergonomic mechanism for wrapping errors. - Southclaws/fault
- 683a tool for handling file uploads simple
https://github.com/xis/baraka
a tool for handling file uploads simple. Contribute to xis/baraka development by creating an account on GitHub.
- 684Scalable game server framework with clustering support and client libraries for iOS, Android, Unity and others through the C SDK.
https://github.com/topfreegames/pitaya
Scalable game server framework with clustering support and client libraries for iOS, Android, Unity and others through the C SDK. - topfreegames/pitaya
- 685Now is a time toolkit for golang
https://github.com/jinzhu/now
Now is a time toolkit for golang. Contribute to jinzhu/now development by creating an account on GitHub.
- 686Load GTFS files in golang
https://github.com/artonge/go-gtfs
Load GTFS files in golang. Contribute to artonge/go-gtfs development by creating an account on GitHub.
- 687A library to notify about any (pluggable) activity on your machine, and let you take action as needed
https://github.com/prashantgupta24/activity-tracker
A library to notify about any (pluggable) activity on your machine, and let you take action as needed - prashantgupta24/activity-tracker
- 688H3-geo distributed cells
https://github.com/mmadfox/go-h3geo-dist
H3-geo distributed cells. Contribute to mmadfox/go-h3geo-dist development by creating an account on GitHub.
- 689:steam_locomotive: Decodes url.Values into Go value(s) and Encodes Go value(s) into url.Values. Dual Array and Full map support.
https://github.com/go-playground/form
:steam_locomotive: Decodes url.Values into Go value(s) and Encodes Go value(s) into url.Values. Dual Array and Full map support. - go-playground/form
- 690A Go (golang) based Elasticsearch client library.
https://github.com/mattbaird/elastigo
A Go (golang) based Elasticsearch client library. Contribute to mattbaird/elastigo development by creating an account on GitHub.
- 691A PDF processor written in Go.
https://github.com/pdfcpu/pdfcpu
A PDF processor written in Go. Contribute to pdfcpu/pdfcpu development by creating an account on GitHub.
- 692Go bindings for raylib, a simple and easy-to-use library to enjoy videogames programming.
https://github.com/gen2brain/raylib-go
Go bindings for raylib, a simple and easy-to-use library to enjoy videogames programming. - gen2brain/raylib-go
- 693Fast resizable golang semaphore primitive
https://github.com/marusama/semaphore
Fast resizable golang semaphore primitive. Contribute to marusama/semaphore development by creating an account on GitHub.
- 694A tiny library for writing concurrent programs in Go using actor model
https://github.com/vladopajic/go-actor
A tiny library for writing concurrent programs in Go using actor model - vladopajic/go-actor
- 695A simple embeddable scripting language which supports concurrent event processing.
https://github.com/krotik/ecal
A simple embeddable scripting language which supports concurrent event processing. - krotik/ecal
- 696A performance oriented 2D/3D math package for Go
https://github.com/ungerik/go3d
A performance oriented 2D/3D math package for Go. Contribute to ungerik/go3d development by creating an account on GitHub.
- 697OpenStreetMap PBF golang parser
https://github.com/maguro/pbf
OpenStreetMap PBF golang parser. Contribute to maguro/pbf development by creating an account on GitHub.
- 698The Emperor takes care of all errors personally
https://github.com/emperror/emperror
The Emperor takes care of all errors personally. Contribute to emperror/emperror development by creating an account on GitHub.
- 699errgroup with goroutine worker limits
https://github.com/neilotoole/errgroup
errgroup with goroutine worker limits. Contribute to neilotoole/errgroup development by creating an account on GitHub.
- 700GoWrap is a command line tool for generating decorators for Go interfaces
https://github.com/hexdigest/gowrap
GoWrap is a command line tool for generating decorators for Go interfaces - hexdigest/gowrap
- 701A cloud-native Go microservices framework with cli tool for productivity.
https://github.com/tal-tech/go-zero
A cloud-native Go microservices framework with cli tool for productivity. - zeromicro/go-zero
- 702CyclicBarrier golang implementation
https://github.com/marusama/cyclicbarrier
CyclicBarrier golang implementation. Contribute to marusama/cyclicbarrier development by creating an account on GitHub.
- 703Go database query builder library for PostgreSQL
https://github.com/arthurkushman/buildsqlx
Go database query builder library for PostgreSQL. Contribute to arthurkushman/buildsqlx development by creating an account on GitHub.
- 704a package for decode form's values into struct in Go
https://github.com/monoculum/formam
a package for decode form's values into struct in Go - monoculum/formam
- 705Go implementation of Fowler's Money pattern
https://github.com/rhymond/go-money
Go implementation of Fowler's Money pattern. Contribute to Rhymond/go-money development by creating an account on GitHub.
- 706Simple, fast and scalable golang rpc library for high load
https://github.com/valyala/gorpc
Simple, fast and scalable golang rpc library for high load - valyala/gorpc
- 707Go package to easily convert a URL's query parameters/values into usable struct values of the correct types.
https://github.com/tomwright/queryparam
Go package to easily convert a URL's query parameters/values into usable struct values of the correct types. - TomWright/queryparam
- 708Golang bindings of Sciter: the Embeddable HTML/CSS/script engine for modern UI development
https://github.com/sciter-sdk/go-sciter
Golang bindings of Sciter: the Embeddable HTML/CSS/script engine for modern UI development - sciter-sdk/go-sciter
- 709Async is an asynchronous task package for Go
https://github.com/yaitoo/async
Async is an asynchronous task package for Go. Contribute to yaitoo/async development by creating an account on GitHub.
- 710Build software better, together
https://github.com/yuin/gopher-lua.
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 711A zero-dependency Go package for coordinate transformations.
https://github.com/wroge/wgs84
A zero-dependency Go package for coordinate transformations. - wroge/wgs84
- 712Azul3D - A 3D game engine written in Go!
https://github.com/azul3d/engine
Azul3D - A 3D game engine written in Go! Contribute to azul3d/engine development by creating an account on GitHub.
- 713Gowl is a process management and process monitoring tool at once. An infinite worker pool gives you the ability to control the pool and processes and monitor their status.
https://github.com/hamed-yousefi/gowl
Gowl is a process management and process monitoring tool at once. An infinite worker pool gives you the ability to control the pool and processes and monitor their status. - hmdsefi/gowl
- 714Software Transactional Locks
https://github.com/ssgreg/stl
Software Transactional Locks. Contribute to ssgreg/stl development by creating an account on GitHub.
- 715vivek-ng/concurrency-limiter
https://github.com/vivek-ng/concurrency-limiter
Contribute to vivek-ng/concurrency-limiter development by creating an account on GitHub.
- 716Dynatomic is a library for using dynamodb as an atomic counter
https://github.com/tylfin/dynatomic
Dynatomic is a library for using dynamodb as an atomic counter - tylfin/dynatomic
- 717Simple and easy to use client for stock market, forex and crypto data from finnhub.io written in Go. Access real-time financial market data from 60+ stock exchanges, 10 forex brokers, and 15+ crypto exchanges
https://github.com/m1/go-finnhub
Simple and easy to use client for stock market, forex and crypto data from finnhub.io written in Go. Access real-time financial market data from 60+ stock exchanges, 10 forex brokers, and 15+ crypt...
- 718Lightweight, facility, high performance golang based game server framework
https://github.com/lonng/nano
Lightweight, facility, high performance golang based game server framework - lonng/nano
- 719Fast, portable, non-Turing complete expression evaluation with gradual typing (Go)
https://github.com/google/cel-go
Fast, portable, non-Turing complete expression evaluation with gradual typing (Go) - google/cel-go
- 720The jsonrpc package helps implement of JSON-RPC 2.0
https://github.com/osamingo/jsonrpc
The jsonrpc package helps implement of JSON-RPC 2.0 - osamingo/jsonrpc
- 721S2 geometry library in Go
https://github.com/golang/geo
S2 geometry library in Go. Contribute to golang/geo development by creating an account on GitHub.
- 722React-like desktop GUI toolkit for Go
https://github.com/roblillack/spot
React-like desktop GUI toolkit for Go. Contribute to roblillack/spot development by creating an account on GitHub.
- 723Convert C to Go
https://github.com/goplus/c2go
Convert C to Go. Contribute to goplus/c2go development by creating an account on GitHub.
- 724Compute cluster (HPC) job submission library for Go (#golang) based on the open DRMAA standard.
https://github.com/dgruber/drmaa
Compute cluster (HPC) job submission library for Go (#golang) based on the open DRMAA standard. - dgruber/drmaa
- 725Concurrency limiting goroutine pool
https://github.com/gammazero/workerpool
Concurrency limiting goroutine pool. Contribute to gammazero/workerpool development by creating an account on GitHub.
- 726Scalable Distributed Game Server Engine with Hot Swapping in Golang
https://github.com/xiaonanln/goworld
Scalable Distributed Game Server Engine with Hot Swapping in Golang - xiaonanln/goworld
- 727Go database/sql driver for Azure Cosmos DB SQL API
https://github.com/btnguyen2k/gocosmos
Go database/sql driver for Azure Cosmos DB SQL API - btnguyen2k/gocosmos
- 728A Go project to explore the math to calculate and present data in a map using the `Web Mercator Projection`
https://github.com/jorelosorio/web-mercator-projection
A Go project to explore the math to calculate and present data in a map using the `Web Mercator Projection` - jorelosorio/web-mercator-projection
- 729:traffic_light: Go bindings for libappindicator3 C library
https://github.com/gopherlibs/appindicator
:traffic_light: Go bindings for libappindicator3 C library - gopherlibs/appindicator
- 730Go language driver for RethinkDB
https://github.com/dancannon/gorethink
Go language driver for RethinkDB. Contribute to rethinkdb/rethinkdb-go development by creating an account on GitHub.
- 731A go library for reading and creating ISO9660 images
https://github.com/kdomanski/iso9660
A go library for reading and creating ISO9660 images - kdomanski/iso9660
- 732Terminal-based game engine for Go, built on top of Termbox
https://github.com/JoelOtter/termloop
Terminal-based game engine for Go, built on top of Termbox - JoelOtter/termloop
- 733a powerful mysql toolset with Go
https://github.com/siddontang/go-mysql
a powerful mysql toolset with Go. Contribute to go-mysql-org/go-mysql development by creating an account on GitHub.
- 734High performance, distributed and low latency publish-subscribe platform.
https://github.com/emitter-io/emitter
High performance, distributed and low latency publish-subscribe platform. - emitter-io/emitter
- 735Go implementation of the Open Packaging Conventions (OPC)
https://github.com/qmuntal/opc
Go implementation of the Open Packaging Conventions (OPC) - qmuntal/opc
- 736immudb - immutable database based on zero trust, SQL/Key-Value/Document model, tamperproof, data change history
https://github.com/codenotary/immudb
immudb - immutable database based on zero trust, SQL/Key-Value/Document model, tamperproof, data change history - codenotary/immudb
- 737A decentralized, trusted, high performance, SQL database with blockchain features
https://github.com/CovenantSQL/CovenantSQL
A decentralized, trusted, high performance, SQL database with blockchain features - CovenantSQL/CovenantSQL
- 738A Go library that implements Consistent Hashing (+Block Partitioning)
https://github.com/mbrostami/consistenthash
A Go library that implements Consistent Hashing (+Block Partitioning) - mbrostami/consistenthash
- 739Simple Features is a pure Go Implementation of the OpenGIS Simple Feature Access Specification
https://github.com/peterstace/simplefeatures
Simple Features is a pure Go Implementation of the OpenGIS Simple Feature Access Specification - peterstace/simplefeatures
- 740The high-performance database for modern applications
https://github.com/dgraph-io/dgraph
The high-performance database for modern applications - dgraph-io/dgraph
- 741Go file operations library chasing GNU APIs.
https://github.com/homedepot/flop
Go file operations library chasing GNU APIs. Contribute to homedepot/flop development by creating an account on GitHub.
- 742Write as Functions, Deploy as a Monolith or Microservice with WebAssembly
https://github.com/tarmac-project/tarmac
Write as Functions, Deploy as a Monolith or Microservice with WebAssembly - tarmac-project/tarmac
- 743Go Sql Server database driver.
https://github.com/minus5/gofreetds
Go Sql Server database driver. Contribute to minus5/gofreetds development by creating an account on GitHub.
- 744Golang library for providing a canonical representation of email address.
https://github.com/dimuska139/go-email-normalizer
Golang library for providing a canonical representation of email address. - dimuska139/go-email-normalizer
- 745A library provides spatial data and geometric algorithms
https://github.com/spatial-go/geoos
A library provides spatial data and geometric algorithms - spatial-go/geoos
- 746Parse natural and standardized dates/times and ranges in Go without knowing the format in advance
https://github.com/ijt/go-anytime
Parse natural and standardized dates/times and ranges in Go without knowing the format in advance - ijt/go-anytime
- 747Go implementation of the A* search algorithm
https://github.com/beefsack/go-astar
Go implementation of the A* search algorithm. Contribute to beefsack/go-astar development by creating an account on GitHub.
- 748A Game Server Skeleton in golang.
https://github.com/xtaci/gonet
A Game Server Skeleton in golang. Contribute to xtaci/gonet development by creating an account on GitHub.
- 749Bind data to any Go value. Can use built-in and custom expression binding capabilities; supports data validation logic for Go values. // 将数据绑定到任何 Go 值。可使用内置和自定义表达式绑定能力;支持对Go值的数据校验逻辑.
https://github.com/bdjimmy/gbind
Bind data to any Go value. Can use built-in and custom expression binding capabilities; supports data validation logic for Go values. // 将数据绑定到任何 Go 值。可使用内置和自定义表达式绑定能力;支持对Go值的数据校验逻辑. - bdjimmy/gbind
- 750Go simple async worker pool
https://github.com/vardius/worker-pool
Go simple async worker pool. Contribute to vardius/worker-pool development by creating an account on GitHub.
- 751A Go (golang) package for representing a list of errors as a single error.
https://github.com/hashicorp/go-multierror
A Go (golang) package for representing a list of errors as a single error. - hashicorp/go-multierror
- 752Neo4j client for Golang
https://github.com/jmcvetta/neoism
Neo4j client for Golang. Contribute to jmcvetta/neoism development by creating an account on GitHub.
- 753HARFANG 3D source code public repository
https://github.com/harfang3d/harfang3d
HARFANG 3D source code public repository. Contribute to harfang3d/harfang3d development by creating an account on GitHub.
- 754Golang package that generates clean, responsive HTML e-mails for sending transactional mail
https://github.com/matcornic/hermes
Golang package that generates clean, responsive HTML e-mails for sending transactional mail - matcornic/hermes
- 755Package approx adds support for durations of days, weeks and years.
https://github.com/goschtalt/approx
Package approx adds support for durations of days, weeks and years. - goschtalt/approx
- 756🦄 Monads and popular FP abstractions, powered by Go 1.18+ Generics (Option, Result, Either...)
https://github.com/samber/mo
🦄 Monads and popular FP abstractions, powered by Go 1.18+ Generics (Option, Result, Either...) - samber/mo
- 757Build cross platform GUI apps with GO and HTML/JS/CSS (powered by nwjs)
https://github.com/dtylman/gowd
Build cross platform GUI apps with GO and HTML/JS/CSS (powered by nwjs) - dtylman/gowd
- 758High-performance, columnar, in-memory store with bitmap indexing in Go
https://github.com/kelindar/column
High-performance, columnar, in-memory store with bitmap indexing in Go - kelindar/column
- 759Tiny cross-platform webview library for C/C++. Uses WebKit (GTK/Cocoa) and Edge WebView2 (Windows).
https://github.com/zserge/webview
Tiny cross-platform webview library for C/C++. Uses WebKit (GTK/Cocoa) and Edge WebView2 (Windows). - webview/webview
- 760Functional Experiment in Golang
https://github.com/seborama/fuego
Functional Experiment in Golang. Contribute to seborama/fuego development by creating an account on GitHub.
- 761👷 Library for safely running groups of workers concurrently or consecutively that require input and output through channels
https://github.com/catmullet/go-workers
👷 Library for safely running groups of workers concurrently or consecutively that require input and output through channels - catmullet/go-workers
- 762Simply ACID* key-value database. At the medium or even low latency it tries to provide greater throughput without losing the ACID properties of the database. The database provides the ability to create record headers at own discretion and use them as transactions. The maximum size of stored data is limited by the size of the computer's RAM.
https://github.com/claygod/coffer
Simply ACID* key-value database. At the medium or even low latency it tries to provide greater throughput without losing the ACID properties of the database. The database provides the ability to cr...
- 763redis client implement by golang, inspired by jedis.
https://github.com/piaohao/godis
redis client implement by golang, inspired by jedis. - piaohao/godis
- 764High-performance framework for building redis-protocol compatible TCP servers/services
https://github.com/bsm/redeo
High-performance framework for building redis-protocol compatible TCP servers/services - bsm/redeo
- 765Go asynchronous simple function utilities, for managing execution of closures and callbacks
https://github.com/vardius/gollback
Go asynchronous simple function utilities, for managing execution of closures and callbacks - vardius/gollback
- 766A Windows GUI toolkit for the Go Programming Language
https://github.com/lxn/walk
A Windows GUI toolkit for the Go Programming Language - lxn/walk
- 767🛫 Fixed-Point Decimals
https://github.com/nikolaydubina/fpdecimal
🛫 Fixed-Point Decimals. Contribute to nikolaydubina/fpdecimal development by creating an account on GitHub.
- 768Qt binding for Go (Golang) with support for Windows / macOS / Linux / FreeBSD / Android / iOS / Sailfish OS / Raspberry Pi / AsteroidOS / Ubuntu Touch / JavaScript / WebAssembly
https://github.com/therecipe/qt
Qt binding for Go (Golang) with support for Windows / macOS / Linux / FreeBSD / Android / iOS / Sailfish OS / Raspberry Pi / AsteroidOS / Ubuntu Touch / JavaScript / WebAssembly - therecipe/qt
- 769Golang->Haxe->CPP/CSharp/Java/JavaScript transpiler
https://github.com/tardisgo/tardisgo
Golang->Haxe->CPP/CSharp/Java/JavaScript transpiler - GitHub - tardisgo/tardisgo: Golang->Haxe->CPP/CSharp/Java/JavaScript transpiler
- 770Package gorilla/csrf provides Cross Site Request Forgery (CSRF) prevention middleware for Go web applications & services 🔒
https://github.com/gorilla/csrf
Package gorilla/csrf provides Cross Site Request Forgery (CSRF) prevention middleware for Go web applications & services 🔒 - gorilla/csrf
- 771Generic 2D grid
https://github.com/s0rg/grid
Generic 2D grid. Contribute to s0rg/grid development by creating an account on GitHub.
- 772A Golang library for using SQL.
https://github.com/gchaincl/dotsql
A Golang library for using SQL. Contribute to qustavo/dotsql development by creating an account on GitHub.
- 773Real-time Geospatial and Geofencing
https://github.com/tidwall/tile38
Real-time Geospatial and Geofencing. Contribute to tidwall/tile38 development by creating an account on GitHub.
- 774A package to build progressive web apps with Go programming language and WebAssembly.
https://github.com/murlokswarm/app
A package to build progressive web apps with Go programming language and WebAssembly. - maxence-charriere/go-app
- 775🔘 Minimalistic and High-performance goroutine worker pool written in Go
https://github.com/alitto/pond
🔘 Minimalistic and High-performance goroutine worker pool written in Go - alitto/pond
- 776🚧 Flexible mechanism to make execution flow interruptible.
https://github.com/kamilsk/breaker
🚧 Flexible mechanism to make execution flow interruptible. - kamilsk/breaker
- 777Vitess is a database clustering system for horizontal scaling of MySQL.
https://github.com/youtube/vitess
Vitess is a database clustering system for horizontal scaling of MySQL. - vitessio/vitess
- 778golang wrapper for github.com/OSGEO/gdal
https://github.com/airbusgeo/godal
golang wrapper for github.com/OSGEO/gdal. Contribute to airbusgeo/godal development by creating an account on GitHub.
- 779Platform-native GUI library for Go.
https://github.com/andlabs/ui
Platform-native GUI library for Go. Contribute to andlabs/ui development by creating an account on GitHub.
- 780fp-go is a collection of Functional Programming helpers powered by Golang 1.18+ generics.
https://github.com/repeale/fp-go
fp-go is a collection of Functional Programming helpers powered by Golang 1.18+ generics. - repeale/fp-go
- 781Gesetzliche Feiertage und mehr in Deutschland und Österreich (Bank holidays/public holidays in Austria and Germany)
https://github.com/wlbr/feiertage
Gesetzliche Feiertage und mehr in Deutschland und Österreich (Bank holidays/public holidays in Austria and Germany) - wlbr/feiertage
- 782A compiler from Go to JavaScript for running Go code in a browser
https://github.com/gopherjs/gopherjs
A compiler from Go to JavaScript for running Go code in a browser - gopherjs/gopherjs
- 783Convert string to duration in golang
https://github.com/xhit/go-str2duration
Convert string to duration in golang. Contribute to xhit/go-str2duration development by creating an account on GitHub.
- 784Sysinfo is a Go library providing Linux OS / kernel / hardware system information.
https://github.com/zcalusic/sysinfo
Sysinfo is a Go library providing Linux OS / kernel / hardware system information. - zcalusic/sysinfo
- 785A comprehensive error handling library for Go
https://github.com/joomcode/errorx
A comprehensive error handling library for Go. Contribute to joomcode/errorx development by creating an account on GitHub.
- 786Golang library for querying and parsing OFX
https://github.com/aclindsa/ofxgo
Golang library for querying and parsing OFX. Contribute to aclindsa/ofxgo development by creating an account on GitHub.
- 787Generate type-safe code from SQL
https://github.com/kyleconroy/sqlc
Generate type-safe code from SQL. Contribute to sqlc-dev/sqlc development by creating an account on GitHub.
- 788Raft library for maintaining a replicated state machine
https://github.com/etcd-io/raft
Raft library for maintaining a replicated state machine - etcd-io/raft
- 789Monad, Functional Programming features for Golang
https://github.com/TeaEntityLab/fpGo
Monad, Functional Programming features for Golang. Contribute to TeaEntityLab/fpGo development by creating an account on GitHub.
- 790An API first development platform
https://github.com/micro/micro
An API first development platform . Contribute to micro/micro development by creating an account on GitHub.
- 791Go concurrency with channel transformations: a toolkit for streaming, batching, pipelines, and error handling
https://github.com/destel/rill
Go concurrency with channel transformations: a toolkit for streaming, batching, pipelines, and error handling - destel/rill
- 792🌟 Useful functional programming helpers for Go
https://github.com/rjNemo/underscore
🌟 Useful functional programming helpers for Go. Contribute to rjNemo/underscore development by creating an account on GitHub.
- 793Golang SQLite without cgo
https://github.com/cvilsmeier/sqinn-go
Golang SQLite without cgo. Contribute to cvilsmeier/sqinn-go development by creating an account on GitHub.
- 794Redis Go client
https://github.com/redis/go-redis
Redis Go client. Contribute to redis/go-redis development by creating an account on GitHub.
- 795✉️ Composable all-in-one mail server.
https://github.com/foxcpp/maddy
✉️ Composable all-in-one mail server. Contribute to foxcpp/maddy development by creating an account on GitHub.
- 796A pure Go game engine
https://github.com/oakmound/oak
A pure Go game engine. Contribute to oakmound/oak development by creating an account on GitHub.
- 797Transpiling C code to Go code
https://github.com/Konstantin8105/c4go
Transpiling C code to Go code. Contribute to Konstantin8105/c4go development by creating an account on GitHub.
- 798Technical Analysis Library for Golang
https://github.com/sdcoffey/techan
Technical Analysis Library for Golang. Contribute to sdcoffey/techan development by creating an account on GitHub.
- 799Simply way to control goroutines execution order based on dependencies
https://github.com/kamildrazkiewicz/go-flow
Simply way to control goroutines execution order based on dependencies - kamildrazkiewicz/go-flow
- 800timeutil - useful extensions (Timedelta, Strftime, ...) to the golang's time package
https://github.com/leekchan/timeutil
timeutil - useful extensions (Timedelta, Strftime, ...) to the golang's time package - leekchan/timeutil
- 801An embedded key/value database for Go.
https://github.com/etcd-io/bbolt
An embedded key/value database for Go. Contribute to etcd-io/bbolt development by creating an account on GitHub.
- 802The Official Golang driver for MongoDB
https://github.com/mongodb/mongo-go-driver
The Official Golang driver for MongoDB. Contribute to mongodb/mongo-go-driver development by creating an account on GitHub.
- 803High-Performance server for NATS.io, the cloud and edge native messaging system.
https://github.com/nats-io/gnatsd
High-Performance server for NATS.io, the cloud and edge native messaging system. - nats-io/nats-server
- 804Secure Vault for Customer PII/PHI/PCI/KYC Records
https://github.com/paranoidguy/databunker
Secure Vault for Customer PII/PHI/PCI/KYC Records. Contribute to securitybunker/databunker development by creating an account on GitHub.
- 805SDL2 binding for Go
https://github.com/veandco/go-sdl2
SDL2 binding for Go. Contribute to veandco/go-sdl2 development by creating an account on GitHub.
- 806:speedboat: a limited consumer goroutine or unlimited goroutine pool for easier goroutine handling and cancellation
https://github.com/go-playground/pool
:speedboat: a limited consumer goroutine or unlimited goroutine pool for easier goroutine handling and cancellation - go-playground/pool
- 807🚀 Configurable Golang 📨 email validator/verifier. Verify email via Regex, DNS, SMTP and even more. Be sure that email address valid and exists.
https://github.com/truemail-rb/truemail-go
🚀 Configurable Golang 📨 email validator/verifier. Verify email via Regex, DNS, SMTP and even more. Be sure that email address valid and exists. - truemail-rb/truemail-go
- 808The Go language implementation of gRPC. HTTP/2 based RPC
https://github.com/grpc/grpc-go
The Go language implementation of gRPC. HTTP/2 based RPC - grpc/grpc-go
- 809Structured concurrency made easy
https://github.com/carlmjohnson/flowmatic
Structured concurrency made easy. Contribute to earthboundkid/flowmatic development by creating an account on GitHub.
- 810General purpose library for reading, writing and working with OpenStreetMap data
https://github.com/paulmach/osm
General purpose library for reading, writing and working with OpenStreetMap data - paulmach/osm
- 811A super simple Lodash like utility library with essential functions that empowers the development in Go
https://github.com/rbrahul/gofp
A super simple Lodash like utility library with essential functions that empowers the development in Go - rbrahul/gofp
- 812An enum generator for go
https://github.com/abice/go-enum
An enum generator for go. Contribute to abice/go-enum development by creating an account on GitHub.
- 813Couchbase client in Go
https://github.com/couchbase/go-couchbase
Couchbase client in Go. Contribute to couchbase/go-couchbase development by creating an account on GitHub.
- 814Pluggable, extensible virtual file system for Go
https://github.com/C2FO/vfs
Pluggable, extensible virtual file system for Go. Contribute to C2FO/vfs development by creating an account on GitHub.
- 815Go option and result types that optionally contain a value
https://github.com/phelmkamp/valor
Go option and result types that optionally contain a value - phelmkamp/valor
- 816Go generator to copy values from type to type and fields from struct to struct (copier without reflection). Generate any code based on types.
https://github.com/switchupcb/copygen
Go generator to copy values from type to type and fields from struct to struct (copier without reflection). Generate any code based on types. - switchupcb/copygen
- 817A simple utility package for exception handling with try-catch in Golang
https://github.com/rbrahul/exception
A simple utility package for exception handling with try-catch in Golang - rbrahul/exception
- 818Go bindings for H3, a hierarchical hexagonal geospatial indexing system
https://github.com/uber/h3-go
Go bindings for H3, a hierarchical hexagonal geospatial indexing system - uber/h3-go
- 819money and currency formatting for golang
https://github.com/leekchan/accounting
money and currency formatting for golang. Contribute to leekchan/accounting development by creating an account on GitHub.
- 820Better structured concurrency for go
https://github.com/sourcegraph/conc
Better structured concurrency for go. Contribute to sourcegraph/conc development by creating an account on GitHub.
- 821Basic Go server for mbtiles
https://github.com/consbio/mbtileserver
Basic Go server for mbtiles. Contribute to consbio/mbtileserver development by creating an account on GitHub.
- 822Go Redis Client
https://github.com/shomali11/xredis
Go Redis Client. Contribute to shomali11/xredis development by creating an account on GitHub.
- 823Distributed batch data processing framework
https://github.com/capillariesio/capillaries
Distributed batch data processing framework. Contribute to capillariesio/capillaries development by creating an account on GitHub.
- 824Full-featured BitTorrent client package and utilities
https://github.com/anacrolix/torrent
Full-featured BitTorrent client package and utilities - anacrolix/torrent
- 825GO DRiver for ORacle DB
https://github.com/godror/godror
GO DRiver for ORacle DB. Contribute to godror/godror development by creating an account on GitHub.
- 826Ebitengine - A dead simple 2D game engine for Go
https://github.com/hajimehoshi/ebiten
Ebitengine - A dead simple 2D game engine for Go. Contribute to hajimehoshi/ebiten development by creating an account on GitHub.
- 827Cross platform GUI toolkit in Go inspired by Material Design
https://github.com/fyne-io/fyne
Cross platform GUI toolkit in Go inspired by Material Design - fyne-io/fyne
- 828A sync.WaitGroup with error handling and concurrency control
https://github.com/pieterclaerhout/go-waitgroup
A sync.WaitGroup with error handling and concurrency control - pieterclaerhout/go-waitgroup
- 829Floc: Orchestrate goroutines with ease.
https://github.com/workanator/go-floc
Floc: Orchestrate goroutines with ease. Contribute to workanator/go-floc development by creating an account on GitHub.
- 830Go library for sending mail with the Mailgun API.
https://github.com/mailgun/mailgun-go
Go library for sending mail with the Mailgun API. Contribute to mailgun/mailgun-go development by creating an account on GitHub.
- 831geoserver is a Go library for manipulating a GeoServer instance via the GeoServer REST API.
https://github.com/hishamkaram/geoserver
geoserver is a Go library for manipulating a GeoServer instance via the GeoServer REST API. - hishamkaram/geoserver
- 832golang worker pool , Concurrency limiting goroutine pool
https://github.com/xxjwxc/gowp
golang worker pool , Concurrency limiting goroutine pool - xxjwxc/gowp
- 833GoLang Parse many date strings without knowing format in advance.
https://github.com/araddon/dateparse
GoLang Parse many date strings without knowing format in advance. - araddon/dateparse
- 834Go Joystick API
https://github.com/0xcafed00d/joystick
Go Joystick API. Contribute to 0xcafed00d/joystick development by creating an account on GitHub.
- 835Go client for Redis
https://github.com/gomodule/redigo
Go client for Redis. Contribute to gomodule/redigo development by creating an account on GitHub.
- 836A Go (golang) package that enhances the standard database/sql package by providing powerful data retrieval methods as well as DB-agnostic query building capabilities.
https://github.com/go-ozzo/ozzo-dbx
A Go (golang) package that enhances the standard database/sql package by providing powerful data retrieval methods as well as DB-agnostic query building capabilities. - go-ozzo/ozzo-dbx
- 837A fast ISO8601 date parser for Go
https://github.com/relvacode/iso8601
A fast ISO8601 date parser for Go. Contribute to relvacode/iso8601 development by creating an account on GitHub.
- 838Golang driver for ClickHouse
https://github.com/ClickHouse/clickhouse-go/
Golang driver for ClickHouse. Contribute to ClickHouse/clickhouse-go development by creating an account on GitHub.
- 839Ultra performant API Gateway with middlewares. A project hosted at The Linux Foundation
https://github.com/luraproject/lura
Ultra performant API Gateway with middlewares. A project hosted at The Linux Foundation - luraproject/lura
- 840Gostradamus: Better DateTimes for Go 🕰️
https://github.com/bykof/gostradamus
Gostradamus: Better DateTimes for Go 🕰️. Contribute to bykof/gostradamus development by creating an account on GitHub.
- 841Checker is a Go library for validating user input through checker rules provided in struct tags.
https://github.com/cinar/checker
Checker is a Go library for validating user input through checker rules provided in struct tags. - cinar/checker
- 842Flowgraph package for scalable asynchronous system development
https://github.com/vectaport/flowgraph
Flowgraph package for scalable asynchronous system development - vectaport/flowgraph
- 843Evans: more expressive universal gRPC client
https://github.com/ktr0731/evans
Evans: more expressive universal gRPC client. Contribute to ktr0731/evans development by creating an account on GitHub.
- 844periph
https://periph.io/
Peripherals I/O in Go
- 845gomponents, HTML components in pure Go
https://www.gomponents.com
HTML components in pure Go, that render to HTML5.
- 846For parsing, creating and editing unknown or dynamic JSON in Go
https://github.com/Jeffail/gabs
For parsing, creating and editing unknown or dynamic JSON in Go - Jeffail/gabs
- 847Word Stemming in Go
https://github.com/agonopol/go-stem
Word Stemming in Go. Contribute to agonopol/go-stem development by creating an account on GitHub.
- 848Simple image color extractor written in Go with no external dependencies
https://github.com/marekm4/color-extractor
Simple image color extractor written in Go with no external dependencies - marekm4/color-extractor
- 849GreyXor / Slogor · GitLab
https://gitlab.com/greyxor/slogor
A colorful slog handler
- 850ipaddress — IPv4/IPv6 manipulation library
https://docs.python.org/3/library/ipaddress.html
Source code: Lib/ipaddress.py ipaddress provides the capabilities to create, manipulate and operate on IPv4 and IPv6 addresses and networks. The functions and classes in this module make it straigh...
- 851Python-like logging design in Golang
https://github.com/xybor-x/xylog
Python-like logging design in Golang. Contribute to xybor-x/xylog development by creating an account on GitHub.
- 852A simple, modern and secure encryption tool (and Go library) with small explicit keys, no config options, and UNIX-style composability.
https://github.com/FiloSottile/age
A simple, modern and secure encryption tool (and Go library) with small explicit keys, no config options, and UNIX-style composability. - FiloSottile/age
- 853ASCII transliterations of Unicode text.
https://github.com/mozillazg/go-unidecode
ASCII transliterations of Unicode text. Contribute to mozillazg/go-unidecode development by creating an account on GitHub.
- 854Log Analysis | Log Management by Loggly
https://www.loggly.com/.
Log Analysis / Log Management by Loggly: the world's most popular log analysis & monitoring in the cloud. Free trial. See why ⅓ of the Fortune 500 use us!
- 855Golang push server cluster
https://github.com/Terry-Mao/gopush-cluster
Golang push server cluster. Contribute to Terry-Mao/gopush-cluster development by creating an account on GitHub.
- 856Buckley / di · GitLab
https://gitlab.com/cosban/di
dependency injection in golang made easy through code generation
- 857Consul by HashiCorp
https://www.consul.io/
Consul is a service networking solution to automate network configurations, discover services, and enable secure connectivity across any cloud or runtime.
- 858TCP proxy, highjacks HTTP to allow CORS
https://github.com/aybabtme/portproxy
TCP proxy, highjacks HTTP to allow CORS. Contribute to aybabtme/portproxy development by creating an account on GitHub.
- 859Resiliency patterns for golang
https://github.com/eapache/go-resiliency
Resiliency patterns for golang. Contribute to eapache/go-resiliency development by creating an account on GitHub.
- 860🔍 Go tool for LSB steganography, capable of hiding any file within an image.
https://github.com/DimitarPetrov/stegify
🔍 Go tool for LSB steganography, capable of hiding any file within an image. - DimitarPetrov/stegify
- 861A simple OCR API server, seriously easy to be deployed by Docker, on Heroku as well
https://github.com/otiai10/ocrserver
A simple OCR API server, seriously easy to be deployed by Docker, on Heroku as well - otiai10/ocrserver
- 862End to end functional test and automation framework
https://github.com/viant/endly
End to end functional test and automation framework - viant/endly
- 863psutil for golang
https://github.com/shirou/gopsutil
psutil for golang. Contribute to shirou/gopsutil development by creating an account on GitHub.
- 864A Go package for quickly building tcp servers
https://github.com/gansidui/gotcp
A Go package for quickly building tcp servers. Contribute to gansidui/gotcp development by creating an account on GitHub.
- 865🔊Minimalist message bus implementation for internal communication with zero-allocation magic on Emit
https://github.com/mustafaturan/bus
🔊Minimalist message bus implementation for internal communication with zero-allocation magic on Emit - mustafaturan/bus
- 866A multilayer perceptron network implemented in Go, with training via backpropagation.
https://github.com/schuyler/neural-go
A multilayer perceptron network implemented in Go, with training via backpropagation. - schuyler/neural-go
- 867Go bingings for GD (http://www.boutell.com/gd/)
https://github.com/bolknote/go-gd
Go bingings for GD (http://www.boutell.com/gd/). Contribute to bolknote/go-gd development by creating an account on GitHub.
- 868variable length integer encoding using prefix code
https://github.com/chmike/varint
variable length integer encoding using prefix code - chmike/varint
- 869All you need with JSON
https://github.com/sinhashubham95/jsonic
All you need with JSON. Contribute to sinhashubham95/jsonic development by creating an account on GitHub.
- 870A simple wrapper around libpcap for the Go programming language
https://github.com/akrennmair/gopcap
A simple wrapper around libpcap for the Go programming language - akrennmair/gopcap
- 871The imghdr module determines the type of image contained in a file for go
https://github.com/corona10/goimghdr
The imghdr module determines the type of image contained in a file for go - corona10/goimghdr
- 872smartcrop finds good image crops for arbitrary crop sizes
https://github.com/muesli/smartcrop
smartcrop finds good image crops for arbitrary crop sizes - muesli/smartcrop
- 873A JSON diff utility
https://github.com/yazgazan/jaydiff
A JSON diff utility. Contribute to yazgazan/jaydiff development by creating an account on GitHub.
- 874Package to do Bradley-Terry Model pairwise compairsons
https://github.com/seanhagen/bradleyterry
Package to do Bradley-Terry Model pairwise compairsons - seanhagen/bradleyterry
- 875Go dependency management tool experiment (deprecated)
https://github.com/golang/dep
Go dependency management tool experiment (deprecated) - golang/dep
- 876Go binding to ImageMagick's MagickWand C API
https://github.com/gographics/imagick
Go binding to ImageMagick's MagickWand C API. Contribute to gographics/imagick development by creating an account on GitHub.
- 877Image resizing in pure Go and SIMD
https://github.com/bamiaux/rez
Image resizing in pure Go and SIMD. Contribute to bamiaux/rez development by creating an account on GitHub.
- 878A logger, for Go
https://github.com/ian-kent/go-log
A logger, for Go. Contribute to ian-kent/go-log development by creating an account on GitHub.
- 879go library for image programming (merge, crop, resize, watermark, animate, ease, transit)
https://github.com/noelyahan/mergi
go library for image programming (merge, crop, resize, watermark, animate, ease, transit) - noelyahan/mergi
- 880Structured logging package for Go.
https://github.com/apex/log
Structured logging package for Go. Contribute to apex/log development by creating an account on GitHub.
- 881Modern Go Application example
https://github.com/sagikazarmark/modern-go-application
Modern Go Application example. Contribute to sagikazarmark/modern-go-application development by creating an account on GitHub.
- 882Go library for encoding glTF 2.0 files
https://github.com/qmuntal/gltf
Go library for encoding glTF 2.0 files. Contribute to qmuntal/gltf development by creating an account on GitHub.
- 883A simple logging module for go, with a rotating file feature and console logging.
https://github.com/jbrodriguez/mlog
A simple logging module for go, with a rotating file feature and console logging. - jbrodriguez/mlog
- 884JPEG-MPO Decoder / Converter Library and CLI Tool
https://github.com/donatj/mpo
JPEG-MPO Decoder / Converter Library and CLI Tool. Contribute to donatj/mpo development by creating an account on GitHub.
- 885You had one job, or more then one, which can be done in steps
https://github.com/kilgaloon/leprechaun
You had one job, or more then one, which can be done in steps - kilgaloon/leprechaun
- 886Port of webcolors library from Python to Go
https://github.com/jyotiska/go-webcolors
Port of webcolors library from Python to Go. Contribute to jyotiska/go-webcolors development by creating an account on GitHub.
- 887:memo: Logging with multiple output targets.
https://github.com/aerogo/log
:memo: Logging with multiple output targets. Contribute to aerogo/log development by creating an account on GitHub.
- 888Probability distributions and associated methods in Go
https://github.com/e-dard/godist
Probability distributions and associated methods in Go - e-dard/godist
- 889Tymoteusz Blazejczyk / Go Formatter · GitLab
https://gitlab.com/tymonx/go-formatter
The Go Formatter library implements “replacement fields” surrounded by curly braces {} format strings.
- 890Build software better, together
https://github.com/jasonlvhit/gocron.
GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
- 891Suite of libraries for IoT devices (written in Go), experimental for x/exp/io
https://github.com/goiot/devices
Suite of libraries for IoT devices (written in Go), experimental for x/exp/io - goiot/devices
- 892A job scheduler for Go with the ability to fast-forward time.
https://github.com/romshark/sched
A job scheduler for Go with the ability to fast-forward time. - romshark/sched
- 893kit/log at master · go-kit/kit
https://github.com/go-kit/kit/tree/master/log
A standard library for microservices. Contribute to go-kit/kit development by creating an account on GitHub.
- 894Simple and fast webp library for golang
https://github.com/kolesa-team/go-webp
Simple and fast webp library for golang. Contribute to kolesa-team/go-webp development by creating an account on GitHub.
- 895Scalable real-time messaging server in a language-agnostic way. Self-hosted alternative to Pubnub, Pusher, Ably. Set up once and forever.
https://github.com/centrifugal/centrifugo
Scalable real-time messaging server in a language-agnostic way. Self-hosted alternative to Pubnub, Pusher, Ably. Set up once and forever. - centrifugal/centrifugo
- 896Job Scheduling Library
https://github.com/onatm/clockwerk
Job Scheduling Library. Contribute to onatm/clockwerk development by creating an account on GitHub.
- 897Abstract JSON for Golang with JSONPath support
https://github.com/spyzhov/ajson
Abstract JSON for Golang with JSONPath support . Contribute to spyzhov/ajson development by creating an account on GitHub.
- 898Pure Golang Library that allows LSB steganography on images using ZERO dependencies
https://github.com/auyer/steganography
Pure Golang Library that allows LSB steganography on images using ZERO dependencies - auyer/steganography
- 899libsvm go version
https://github.com/datastream/libsvm
libsvm go version. Contribute to datastream/libsvm development by creating an account on GitHub.
- 900Structured, composable logging for Go
https://github.com/inconshreveable/log15
Structured, composable logging for Go. Contribute to inconshreveable/log15 development by creating an account on GitHub.
- 901Go Perceptual image hashing package
https://github.com/corona10/goimagehash
Go Perceptual image hashing package. Contribute to corona10/goimagehash development by creating an account on GitHub.
- 902:foggy: Convert image to ASCII
https://github.com/qeesung/image2ascii
:foggy: Convert image to ASCII. Contribute to qeesung/image2ascii development by creating an account on GitHub.
- 903Wrapper package for Go's template/html to allow for easy file-based template inheritance.
https://github.com/dannyvankooten/extemplate
Wrapper package for Go's template/html to allow for easy file-based template inheritance. - dannyvankooten/extemplate
- 904gone/log at master · One-com/gone
https://github.com/One-com/gone/tree/master/log
Golang packages for writing small daemons and servers. - One-com/gone
- 905Framework for performing work asynchronously, outside of the request flow
https://github.com/bamzi/jobrunner
Framework for performing work asynchronously, outside of the request flow - bamzi/jobrunner
- 906Transliterate Cyrillic → Latin in every possible way
https://github.com/mehanizm/iuliia-go
Transliterate Cyrillic → Latin in every possible way - mehanizm/iuliia-go
- 907🔥 Fast, simple sklearn-like feature processing for Go
https://github.com/nikolaydubina/go-featureprocessing
🔥 Fast, simple sklearn-like feature processing for Go - nikolaydubina/go-featureprocessing
- 908Bayesian text classifier with flexible tokenizers and storage backends for Go
https://github.com/eaigner/shield
Bayesian text classifier with flexible tokenizers and storage backends for Go - eaigner/shield
- 909Cairo in Go: vector to raster, SVG, PDF, EPS, WASM, OpenGL, Gio, etc.
https://github.com/tdewolff/canvas
Cairo in Go: vector to raster, SVG, PDF, EPS, WASM, OpenGL, Gio, etc. - tdewolff/canvas
- 910Simple and minimal image server capable of storing, resizing, converting and caching images.
https://github.com/mehdipourfar/webp-server
Simple and minimal image server capable of storing, resizing, converting and caching images. - mehdipourfar/webp-server
- 911A Golang library to manipulate strings according to the word parsing rules of the UNIX Bourne shell.
https://github.com/Wing924/shellwords
A Golang library to manipulate strings according to the word parsing rules of the UNIX Bourne shell. - Wing924/shellwords
- 912Go client to reliable queues based on Redis Cluster Streams
https://github.com/kak-tus/ami
Go client to reliable queues based on Redis Cluster Streams - kak-tus/ami
- 913go.rice is a Go package that makes working with resources such as html,js,css,images,templates, etc very easy.
https://github.com/GeertJohan/go.rice
go.rice is a Go package that makes working with resources such as html,js,css,images,templates, etc very easy. - GeertJohan/go.rice
- 914Expressive end-to-end HTTP API testing made easy in Go
https://github.com/h2non/baloo
Expressive end-to-end HTTP API testing made easy in Go - h2non/baloo
- 915A dependency injection based application framework for Go.
https://github.com/uber-go/fx
A dependency injection based application framework for Go. - uber-go/fx
- 916gup - Update binaries installed by "go install" with goroutines.
https://github.com/nao1215/gup
gup - Update binaries installed by "go install" with goroutines. - nao1215/gup
- 917Simple leveled logging wrapper around standard log package
https://github.com/heartwilltell/log
Simple leveled logging wrapper around standard log package - heartwilltell/log
- 918A pure Go 3D math library.
https://github.com/go-gl/mathgl
A pure Go 3D math library. Contribute to go-gl/mathgl development by creating an account on GitHub.
- 919[UNMANTEINED] Extract values from strings and fill your structs with nlp.
https://github.com/Shixzie/nlp
[UNMANTEINED] Extract values from strings and fill your structs with nlp. - shixzie/nlp
- 920Create go type representation from json
https://github.com/m-zajac/json2go
Create go type representation from json. Contribute to m-zajac/json2go development by creating an account on GitHub.
- 921A Go HTTP client library for creating and sending API requests
https://github.com/dghubble/sling
A Go HTTP client library for creating and sending API requests - dghubble/sling
- 922Imaging is a simple image processing package for Go
https://github.com/disintegration/imaging
Imaging is a simple image processing package for Go - disintegration/imaging
- 923The implementation of the pattern observer
https://github.com/agoalofalife/event
The implementation of the pattern observer. Contribute to agoalofalife/event development by creating an account on GitHub.
- 924Leveled execution logs for Go
https://github.com/golang/glog
Leveled execution logs for Go. Contribute to golang/glog development by creating an account on GitHub.
- 925🫐 Rich rendering of JSON as HTML in Go
https://github.com/nikolaydubina/htmljson
🫐 Rich rendering of JSON as HTML in Go. Contribute to nikolaydubina/htmljson development by creating an account on GitHub.
- 926A simple Go package to make custom structs marshal into HAL compatible JSON responses.
https://github.com/RichardKnop/jsonhal
A simple Go package to make custom structs marshal into HAL compatible JSON responses. - RichardKnop/jsonhal
- 927Job scheduling made easy.
https://github.com/carlescere/scheduler
Job scheduling made easy. Contribute to carlescere/scheduler development by creating an account on GitHub.
- 928The "interpol" security string interpolation library and the "police" command line tool. Just moved here from https://bitbucket.org/vahidi/interpol
https://github.com/avahidi/interpol
The "interpol" security string interpolation library and the "police" command line tool. Just moved here from https://bitbucket.org/vahidi/interpol - avahidi/interpol
- 929Structured log interface
https://github.com/teris-io/log
Structured log interface. Contribute to teris-io/log development by creating an account on GitHub.
- 930Generate High Level Cloud Architecture diagrams using YAML syntax.
https://github.com/lucasepe/draft
Generate High Level Cloud Architecture diagrams using YAML syntax. - lucasepe/draft
- 931Testing API Handler written in Golang.
https://github.com/appleboy/gofight
Testing API Handler written in Golang. Contribute to appleboy/gofight development by creating an account on GitHub.
- 932Seelog is a native Go logging library that provides flexible asynchronous dispatching, filtering, and formatting.
https://github.com/cihub/seelog
Seelog is a native Go logging library that provides flexible asynchronous dispatching, filtering, and formatting. - cihub/seelog
- 933software package for automation
https://github.com/e154/smart-home
software package for automation. Contribute to e154/smart-home development by creating an account on GitHub.
- 934Simple logger for Go programs. Allows custom formats for messages.
https://github.com/apsdehal/go-logger
Simple logger for Go programs. Allows custom formats for messages. - apsdehal/go-logger
- 935Golang ticker that works with Cron scheduling.
https://github.com/krayzpipes/cronticker
Golang ticker that works with Cron scheduling. Contribute to krayzpipes/cronticker development by creating an account on GitHub.
- 936Selected Machine Learning algorithms for natural language processing and semantic analysis in Golang
https://github.com/james-bowman/nlp
Selected Machine Learning algorithms for natural language processing and semantic analysis in Golang - james-bowman/nlp
- 937Avatar generation library for GO language
https://github.com/o1egl/govatar
Avatar generation library for GO language. Contribute to o1egl/govatar development by creating an account on GitHub.
- 938Go implementation of systemd Journal's native API for logging
https://github.com/ssgreg/journald
Go implementation of systemd Journal's native API for logging - ssgreg/journald
- 939Gatt is a Go package for building Bluetooth Low Energy peripherals
https://github.com/paypal/gatt
Gatt is a Go package for building Bluetooth Low Energy peripherals - paypal/gatt
- 940xorm
https://gitea.com/xorm/xorm
Simple and Powerful ORM for Go, support mysql,postgres,tidb,sqlite3,sqlite,mssql,oracle,cockroach
- 941On-line Machine Learning in Go (and so much more)
https://github.com/cdipaolo/goml
On-line Machine Learning in Go (and so much more). Contribute to cdipaolo/goml development by creating an account on GitHub.
- 942The simple JSON parser with validation by condition
https://github.com/dedalqq/omg.jsonparser
The simple JSON parser with validation by condition - dedalqq/omg.jsonparser
- 943ODE system solver made simple. For IVPs (initial value problems).
https://github.com/soypat/godesim
ODE system solver made simple. For IVPs (initial value problems). - soypat/godesim
- 944A CLI tool for building Go applications.
https://github.com/go-nunu/nunu
A CLI tool for building Go applications. Contribute to go-nunu/nunu development by creating an account on GitHub.
- 945Golang logger to different configurable writers.
https://github.com/mbndr/logo
Golang logger to different configurable writers. Contribute to mbndr/logo development by creating an account on GitHub.
- 946汉字转拼音
https://github.com/mozillazg/go-pinyin
汉字转拼音. Contribute to mozillazg/go-pinyin development by creating an account on GitHub.
- 947Go Stream, like Java 8 Stream.
https://github.com/youthlin/stream
Go Stream, like Java 8 Stream. Contribute to youthlin/stream development by creating an account on GitHub.
- 948A tiny wrapper over amqp exchanges and queues 🚌 ✨
https://github.com/rafaeljesus/rabbus
A tiny wrapper over amqp exchanges and queues 🚌 ✨. Contribute to rafaeljesus/rabbus development by creating an account on GitHub.
- 949A collection of Go packages for creating robust GraphQL APIs
https://github.com/ccbrown/api-fu
A collection of Go packages for creating robust GraphQL APIs - ccbrown/api-fu
- 950io.Writer implementation using logrus logger [managed by soy-programador]
https://github.com/cabify/logrusiowriter
io.Writer implementation using logrus logger [managed by soy-programador] - cabify/logrusiowriter
- 951Artificial Neural Network
https://github.com/patrikeh/go-deep
Artificial Neural Network. Contribute to patrikeh/go-deep development by creating an account on GitHub.
- 952Cameradar hacks its way into RTSP videosurveillance cameras
https://github.com/Ullaakut/cameradar
Cameradar hacks its way into RTSP videosurveillance cameras - Ullaakut/cameradar
- 953Lightweight stream processing engine for IoT
https://github.com/sensorbee/sensorbee
Lightweight stream processing engine for IoT. Contribute to sensorbee/sensorbee development by creating an account on GitHub.
- 9542D triangulation library. Allows translating lines and polygons (both based on points) to the language of GPUs.
https://github.com/tchayen/triangolatte
2D triangulation library. Allows translating lines and polygons (both based on points) to the language of GPUs. - tchayen/triangolatte
- 955Go package for reading from continously updated files (tail -f)
https://github.com/hpcloud/tail
Go package for reading from continously updated files (tail -f) - hpcloud/tail
- 956Zap logger with context
https://github.com/yuseferi/zax
Zap logger with context. Contribute to yuseferi/zax development by creating an account on GitHub.
- 957Rolling writer is an IO util for auto rolling write in go.
https://github.com/arthurkiller/rollingWriter
Rolling writer is an IO util for auto rolling write in go. - arthurkiller/rollingwriter
- 958Package for multi-level logging
https://github.com/ewwwwwqm/logdump
Package for multi-level logging. Contribute to ewwwwwqm/logdump development by creating an account on GitHub.
- 959A Go client for Google IoT Core
https://github.com/vaelen/iot/
A Go client for Google IoT Core. Contribute to vaelen/iot development by creating an account on GitHub.
- 960Closure Tools | Google for Developers
https://developers.google.com/closure/templates/.
The Closure Compiler, Library, Templates, and Stylesheets help developers create powerful and efficient JavaScript.
- 961Apache htpasswd Parser for Go.
https://github.com/tg123/go-htpasswd
Apache htpasswd Parser for Go. Contribute to tg123/go-htpasswd development by creating an account on GitHub.
- 962get simple struct from complex json
https://github.com/limiu82214/gojmapr
get simple struct from complex json. Contribute to limiu82214/gojmapr development by creating an account on GitHub.
- 963Light Object-Relational Environment (LORE) provides a simple and lightweight pseudo-ORM/pseudo-struct-mapping environment for Go
https://github.com/abrahambotros/lore
Light Object-Relational Environment (LORE) provides a simple and lightweight pseudo-ORM/pseudo-struct-mapping environment for Go - abrahambotros/lore
- 964Quick Solution with Unstructured JSON data
https://github.com/Andrew-M-C/go.jsonvalue
Quick Solution with Unstructured JSON data. Contribute to Andrew-M-C/go.jsonvalue development by creating an account on GitHub.
- 965A simple Cron library for go that can execute closures or functions at varying intervals, from once a second to once a year on a specific date and time. Primarily for web applications and long running daemons.
https://github.com/rk/go-cron
A simple Cron library for go that can execute closures or functions at varying intervals, from once a second to once a year on a specific date and time. Primarily for web applications and long runn...
- 966An AMQP 0-9-1 Go client maintained by the RabbitMQ team. Originally by @streadway: `streadway/amqp`
https://github.com/rabbitmq/amqp091-go
An AMQP 0-9-1 Go client maintained by the RabbitMQ team. Originally by @streadway: `streadway/amqp` - rabbitmq/amqp091-go
- 967Machine Learning for Go
https://github.com/sjwhitworth/golearn
Machine Learning for Go. Contribute to sjwhitworth/golearn development by creating an account on GitHub.
- 968Database access layer for golang
https://github.com/Fs02/grimoire
Database access layer for golang. Contribute to Fs02/grimoire development by creating an account on GitHub.
- 969Pure Go implementation of the NaCL set of API's
https://github.com/kevinburke/nacl
Pure Go implementation of the NaCL set of API's. Contribute to kevinburke/nacl development by creating an account on GitHub.
- 970Resource Query Language for REST
https://github.com/a8m/rql
Resource Query Language for REST. Contribute to a8m/rql development by creating an account on GitHub.
- 971Scout is a standalone open source software solution for DIY video security.
https://github.com/jonoton/scout
Scout is a standalone open source software solution for DIY video security. - jonoton/scout
- 972Loggly Hooks for GO Logrus logger
https://github.com/sebest/logrusly
Loggly Hooks for GO Logrus logger. Contribute to sebest/logrusly development by creating an account on GitHub.
- 973A simplistic Neural Network Library in Go
https://github.com/surenderthakran/gomind
A simplistic Neural Network Library in Go. Contribute to surenderthakran/gomind development by creating an account on GitHub.
- 974A hyperparameter optimization framework, inspired by Optuna.
https://github.com/c-bata/goptuna
A hyperparameter optimization framework, inspired by Optuna. - c-bata/goptuna
- 975Small utility to create JSON objects
https://github.com/skanehira/gjo
Small utility to create JSON objects. Contribute to skanehira/gjo development by creating an account on GitHub.
- 976cdule (pronounce as Schedule) Golang based scheduler library with database support.
https://github.com/deepaksinghvi/cdule
cdule (pronounce as Schedule) Golang based scheduler library with database support. - deepaksinghvi/cdule
- 977Neural Networks written in go
https://github.com/goml/gobrain
Neural Networks written in go. Contribute to goml/gobrain development by creating an account on GitHub.
- 978Colored pretty printer for Go language
https://github.com/k0kubun/pp
Colored pretty printer for Go language. Contribute to k0kubun/pp development by creating an account on GitHub.
- 979Write and read JSON from different sources in one line
https://github.com/lucassscaravelli/ej
Write and read JSON from different sources in one line - lucassscaravelli/ej
- 980Gaurun Client written in Go
https://github.com/osamingo/gaurun-client
Gaurun Client written in Go. Contribute to osamingo/gaurun-client development by creating an account on GitHub.
- 981Utilities for slightly better logging in Go (Golang).
https://github.com/hashicorp/logutils
Utilities for slightly better logging in Go (Golang). - hashicorp/logutils
- 982🔍 gowitness - a golang, web screenshot utility using Chrome Headless
https://github.com/sensepost/gowitness
🔍 gowitness - a golang, web screenshot utility using Chrome Headless - sensepost/gowitness
- 983A Grid based 2D Graphics library
https://github.com/shomali11/gridder
A Grid based 2D Graphics library. Contribute to shomali11/gridder development by creating an account on GitHub.
- 984A persistent and flexible background jobs library for go.
https://github.com/albrow/jobs
A persistent and flexible background jobs library for go. - albrow/jobs
- 985plugin architecture and flexible log system for golang
https://github.com/xfxdev/xlog
plugin architecture and flexible log system for golang - xfxdev/xlog
- 986OpenAPI Initiative golang toolkit
https://github.com/go-openapi
OpenAPI Initiative golang toolkit has 16 repositories available. Follow their code on GitHub.
- 987Golang Neural Network
https://github.com/Xamber/Varis
Golang Neural Network . Contribute to Xamber/Varis development by creating an account on GitHub.
- 988Recommendation engine for Go
https://github.com/muesli/regommend
Recommendation engine for Go. Contribute to muesli/regommend development by creating an account on GitHub.
- 989Minimalistic logging library for Go.
https://github.com/azer/logger
Minimalistic logging library for Go. Contribute to azer/logger development by creating an account on GitHub.
- 990Machinery is an asynchronous task queue/job queue based on distributed message passing.
https://github.com/RichardKnop/machinery
Machinery is an asynchronous task queue/job queue based on distributed message passing. - RichardKnop/machinery
- 991Validate the Strength of a Password in Go
https://github.com/lane-c-wagner/go-password-validator
Validate the Strength of a Password in Go. Contribute to wagslane/go-password-validator development by creating an account on GitHub.
- 992Minimalist and zero-dependency scheduling library for Go
https://github.com/reugn/go-quartz
Minimalist and zero-dependency scheduling library for Go - reugn/go-quartz
- 993A library for storing unit vectors in a representation that lends itself to saving space on disk.
https://github.com/recolude/unitpacking
A library for storing unit vectors in a representation that lends itself to saving space on disk. - recolude/unitpacking
- 994The official Go package for NSQ
https://github.com/nsqio/go-nsq
The official Go package for NSQ. Contribute to nsqio/go-nsq development by creating an account on GitHub.
- 995Transform ML models into a native code (Java, C, Python, Go, JavaScript, Visual Basic, C#, R, PowerShell, PHP, Dart, Haskell, Ruby, F#, Rust) with zero dependencies
https://github.com/BayesWitnesses/m2cgen
Transform ML models into a native code (Java, C, Python, Go, JavaScript, Visual Basic, C#, R, PowerShell, PHP, Dart, Haskell, Ruby, F#, Rust) with zero dependencies - BayesWitnesses/m2cgen
- 996💨 A real time messaging system to build a scalable in-app notifications, multiplayer games, chat apps in web and mobile apps.
https://github.com/Clivern/Beaver
💨 A real time messaging system to build a scalable in-app notifications, multiplayer games, chat apps in web and mobile apps. - Clivern/Beaver
- 997Package for downloading things from a string URL using a variety of protocols.
https://github.com/hashicorp/go-getter
Package for downloading things from a string URL using a variety of protocols. - hashicorp/go-getter
- 998JSON query in Golang
https://github.com/elgs/gojq
JSON query in Golang. Contribute to elgs/gojq development by creating an account on GitHub.
- 999Neural Network for Go.
https://github.com/dathoangnd/gonet
Neural Network for Go. Contribute to dathoangnd/gonet development by creating an account on GitHub.
- 10003D line art engine.
https://github.com/fogleman/ln
3D line art engine. Contribute to fogleman/ln development by creating an account on GitHub.
- 1001Collaborative Filtering (CF) Algorithms in Go!
https://github.com/timkaye11/goRecommend
Collaborative Filtering (CF) Algorithms in Go! . Contribute to timkaye11/goRecommend development by creating an account on GitHub.
- 1002go websocket, a better way to buid your IM server
https://github.com/fanux/lhttp
go websocket, a better way to buid your IM server. Contribute to fanux/lhttp development by creating an account on GitHub.
- 1003Confluent's Apache Kafka Golang client
https://github.com/confluentinc/confluent-kafka-go
Confluent's Apache Kafka Golang client. Contribute to confluentinc/confluent-kafka-go development by creating an account on GitHub.
- 1004Get JSON values quickly - JSON parser for Go
https://github.com/tidwall/gjson
Get JSON values quickly - JSON parser for Go. Contribute to tidwall/gjson development by creating an account on GitHub.
- 1005Glue - Robust Go and Javascript Socket Library (Alternative to Socket.io)
https://github.com/desertbit/glue
Glue - Robust Go and Javascript Socket Library (Alternative to Socket.io) - desertbit/glue
- 1006Optimized JSON for Go
https://github.com/ohler55/ojg
Optimized JSON for Go. Contribute to ohler55/ojg development by creating an account on GitHub.
- 1007Logging packages for Go
https://github.com/alexcesaro/log
Logging packages for Go. Contribute to alexcesaro/log development by creating an account on GitHub.
- 1008Naive Bayesian Classification for Golang.
https://github.com/jbrukh/bayesian
Naive Bayesian Classification for Golang. Contribute to jbrukh/bayesian development by creating an account on GitHub.
- 1009Library for interacting with LLVM IR in pure Go.
https://github.com/llir/llvm
Library for interacting with LLVM IR in pure Go. Contribute to llir/llvm development by creating an account on GitHub.
- 1010Nudity detection with Go.
https://github.com/koyachi/go-nude
Nudity detection with Go. Contribute to koyachi/go-nude development by creating an account on GitHub.
- 1011onnx-go gives the ability to import a pre-trained neural network within Go without being linked to a framework or library.
https://github.com/owulveryck/onnx-go
onnx-go gives the ability to import a pre-trained neural network within Go without being linked to a framework or library. - owulveryck/onnx-go
- 1012Lightweight, fast and dependency-free Cron expression parser (due checker, next/prev due date finder), task runner, job scheduler and/or daemon for Golang (tested on v1.13+) and standalone usage. If you are bold, use it to replace crontab entirely.
https://github.com/adhocore/gronx
Lightweight, fast and dependency-free Cron expression parser (due checker, next/prev due date finder), task runner, job scheduler and/or daemon for Golang (tested on v1.13+) and standalone usage. I...
- 1013Console JSON formatter with query feature
https://github.com/miolini/jsonf
Console JSON formatter with query feature. Contribute to miolini/jsonf development by creating an account on GitHub.
- 1014A lightweight stream processing library for Go
https://github.com/reugn/go-streams
A lightweight stream processing library for Go. Contribute to reugn/go-streams development by creating an account on GitHub.
- 1015Chanify is a safe and simple notification tools. This repository is command line tools for Chanify.
https://github.com/chanify/chanify
Chanify is a safe and simple notification tools. This repository is command line tools for Chanify. - chanify/chanify
- 1016Pattern recognition package in Go lang.
https://github.com/daviddengcn/go-pr
Pattern recognition package in Go lang. Contribute to daviddengcn/go-pr development by creating an account on GitHub.
- 1017fonet is a deep neural network package for Go.
https://github.com/Fontinalis/fonet
fonet is a deep neural network package for Go. Contribute to Fontinalis/fonet development by creating an account on GitHub.
- 1018Kubernetes-native IoT gateway
https://github.com/Edgenesis/shifu
Kubernetes-native IoT gateway. Contribute to Edgenesis/shifu development by creating an account on GitHub.
- 1019Open source image CDN.
https://github.com/Pixboost/transformimgs
Open source image CDN. Contribute to Pixboost/transformimgs development by creating an account on GitHub.
- 1020A path tracer written in Go.
https://github.com/fogleman/pt
A path tracer written in Go. Contribute to fogleman/pt development by creating an account on GitHub.
- 1021Package notify provides an implementation of the Gnome DBus Notifications Specification.
https://github.com/TheCreeper/go-notify
Package notify provides an implementation of the Gnome DBus Notifications Specification. - TheCreeper/go-notify
- 1022Go Graphics - 2D rendering in Go with a simple API.
https://github.com/fogleman/gg
Go Graphics - 2D rendering in Go with a simple API. - fogleman/gg
- 1023JSON library to expose simple handlers that lets you easily read and write json from various sources.
https://github.com/abusomani/jsonhandlers
JSON library to expose simple handlers that lets you easily read and write json from various sources. - abusomani/jsonhandlers
- 1024A powerful zero-dependency json logger.
https://github.com/edoger/zkits-logger
A powerful zero-dependency json logger. Contribute to edoger/zkits-logger development by creating an account on GitHub.
- 1025Automatically generate Go (golang) struct definitions from example JSON
https://github.com/ChimeraCoder/gojson
Automatically generate Go (golang) struct definitions from example JSON - ChimeraCoder/gojson
- 1026k-modes and k-prototypes clustering algorithms implementation in Go
https://github.com/e-XpertSolutions/go-cluster
k-modes and k-prototypes clustering algorithms implementation in Go - e-XpertSolutions/go-cluster
- 1027LogVoyage - logging SaaS written in GoLang
https://github.com/firstrow/logvoyage
LogVoyage - logging SaaS written in GoLang. Contribute to firstrow/logvoyage development by creating an account on GitHub.
- 1028Framework for authoring workflows and activities running on top of the Cadence orchestration engine.
https://github.com/uber-go/cadence-client
Framework for authoring workflows and activities running on top of the Cadence orchestration engine. - uber-go/cadence-client
- 1029Automatically exported from code.google.com/p/probab
https://github.com/ThePaw/probab
Automatically exported from code.google.com/p/probab - ThePaw/probab
- 1030Go package for decoding and encoding TARGA image format
https://github.com/ftrvxmtrx/tga
Go package for decoding and encoding TARGA image format - ftrvxmtrx/tga
- 1031The fantastic ORM library for Golang, aims to be developer friendly
https://github.com/go-gorm/gorm
The fantastic ORM library for Golang, aims to be developer friendly - go-gorm/gorm
- 1032Lightweight data stream processing engine for IoT edge
https://github.com/lf-edge/ekuiper
Lightweight data stream processing engine for IoT edge - lf-edge/ekuiper
- 1033:triangular_ruler: Create beautiful generative image patterns from a string in golang.
https://github.com/pravj/geopattern
:triangular_ruler: Create beautiful generative image patterns from a string in golang. - pravj/geopattern
- 1034gron, Cron Jobs in Go.
https://github.com/roylee0704/gron
gron, Cron Jobs in Go. Contribute to roylee0704/gron development by creating an account on GitHub.
- 1035Passive DNS Capture and Monitoring Toolkit
https://github.com/mosajjal/dnsmonster
Passive DNS Capture and Monitoring Toolkit. Contribute to mosajjal/dnsmonster development by creating an account on GitHub.
- 1036Emits events in Go way, with wildcard, predicates, cancellation possibilities and many other good wins
https://github.com/olebedev/emitter
Emits events in Go way, with wildcard, predicates, cancellation possibilities and many other good wins - olebedev/emitter
- 1037🚨 Design workflows of slog handlers: pipeline, middleware, fanout, routing, failover, load balancing...
https://github.com/samber/slog-multi
🚨 Design workflows of slog handlers: pipeline, middleware, fanout, routing, failover, load balancing... - samber/slog-multi
- 1038Abstraction layer for simple rabbitMQ connection, messaging and administration
https://github.com/socifi/jazz
Abstraction layer for simple rabbitMQ connection, messaging and administration - socifi/jazz
- 1039A Go interface to ZeroMQ version 3
https://github.com/pebbe/zmq3
A Go interface to ZeroMQ version 3. Contribute to pebbe/zmq3 development by creating an account on GitHub.
- 1040Generate scaffold project layout for Go.
https://github.com/catchplay/scaffold
Generate scaffold project layout for Go. Contribute to catchplay/scaffold development by creating an account on GitHub.
- 1041🍜 Parametrized JSON logging library in Golang which lets you obfuscate sensitive data and marshal any kind of content.
https://github.com/gyozatech/noodlog
🍜 Parametrized JSON logging library in Golang which lets you obfuscate sensitive data and marshal any kind of content. - gyozatech/noodlog
- 1042RapidMQ is a pure, extremely productive, lightweight and reliable library for managing of the local messages queue
https://github.com/sybrexsys/RapidMQ
RapidMQ is a pure, extremely productive, lightweight and reliable library for managing of the local messages queue - sybrexsys/RapidMQ
- 1043GoLang Library for Browser Capabilities Project
https://github.com/digitalcrab/browscap_go
GoLang Library for Browser Capabilities Project. Contribute to digitalcrab/browscap_go development by creating an account on GitHub.
- 1044A simple logging framework out of the box
https://github.com/no-src/log
A simple logging framework out of the box. Contribute to no-src/log development by creating an account on GitHub.
- 1045Go Scoring API for PMML
https://github.com/asafschers/goscore
Go Scoring API for PMML. Contribute to asafschers/goscore development by creating an account on GitHub.
- 1046:mailbox_closed: Your own local SMS gateway in Go
https://github.com/haxpax/gosms
:mailbox_closed: Your own local SMS gateway in Go. Contribute to haxpax/gosms development by creating an account on GitHub.
- 1047An additive dependency injection container for Golang.
https://github.com/magic003/alice
An additive dependency injection container for Golang. - magic003/alice
- 1048Unicode transliterator for #golang
https://github.com/fiam/gounidecode
Unicode transliterator for #golang. Contribute to fiam/gounidecode development by creating an account on GitHub.
- 1049Go Todo Backend example using modular project layout for product microservice.
https://github.com/Fs02/go-todo-backend
Go Todo Backend example using modular project layout for product microservice. - Fs02/go-todo-backend
- 1050High performance JSON iterator & validator for Go
https://github.com/romshark/jscan
High performance JSON iterator & validator for Go. Contribute to romshark/jscan development by creating an account on GitHub.
- 1051cross-platform, normalized battery information library
https://github.com/distatus/battery
cross-platform, normalized battery information library - distatus/battery
- 1052Implements string functions widely used in other languages but absent in Go.
https://github.com/huandu/xstrings
Implements string functions widely used in other languages but absent in Go. - huandu/xstrings
- 1053Go bindings based on the JSON API errors reference
https://github.com/AmuzaTkts/jsonapi-errors
Go bindings based on the JSON API errors reference - AmuzaTkts/jsonapi-errors
- 1054Minimal cgo bindings for libenca
https://github.com/endeveit/enca
Minimal cgo bindings for libenca. Contribute to endeveit/enca development by creating an account on GitHub.
- 1055Minimalistic, pluggable Golang evloop/timer handler with dependency-injection
https://github.com/mudler/anagent
Minimalistic, pluggable Golang evloop/timer handler with dependency-injection - mudler/anagent
- 1056Strict Runtime Dependency Injection for Golang
https://github.com/Fs02/wire
Strict Runtime Dependency Injection for Golang. Contribute to Fs02/wire development by creating an account on GitHub.
- 1057xlog is a logger for net/context aware HTTP applications
https://github.com/rs/xlog
xlog is a logger for net/context aware HTTP applications - rs/xlog
- 1058Package dhcp6 implements a DHCPv6 server, as described in RFC 3315. MIT Licensed.
https://github.com/mdlayher/dhcp6
Package dhcp6 implements a DHCPv6 server, as described in RFC 3315. MIT Licensed. - mdlayher/dhcp6
- 1059Marshmallow provides a flexible and performant JSON unmarshalling in Go. It specializes in dealing with unstructured struct - when some fields are known and some aren't, with zero performance overhead nor extra coding needed.
https://github.com/PerimeterX/marshmallow
Marshmallow provides a flexible and performant JSON unmarshalling in Go. It specializes in dealing with unstructured struct - when some fields are known and some aren't, with zero performance o...
- 1060Go package for OCR (Optical Character Recognition), by using Tesseract C++ library
https://github.com/otiai10/gosseract
Go package for OCR (Optical Character Recognition), by using Tesseract C++ library - otiai10/gosseract
- 1061Golang bindings for libxlsxwriter for writing XLSX files
https://github.com/fterrag/goxlsxwriter
Golang bindings for libxlsxwriter for writing XLSX files - fterrag/goxlsxwriter
- 1062Simple command bus for GO
https://github.com/lana/go-commandbus
Simple command bus for GO. Contribute to lana/go-commandbus development by creating an account on GitHub.
- 1063🚃 A library for handling mediator patterns and simplified CQRS patterns within an event-driven architecture, inspired by csharp MediatR library.
https://github.com/mehdihadeli/Go-MediatR
🚃 A library for handling mediator patterns and simplified CQRS patterns within an event-driven architecture, inspired by csharp MediatR library. - mehdihadeli/Go-MediatR
- 1064Extremely flexible golang deep comparison, extends the go testing package, tests HTTP APIs and provides tests suite
https://github.com/maxatome/go-testdeep
Extremely flexible golang deep comparison, extends the go testing package, tests HTTP APIs and provides tests suite - maxatome/go-testdeep
- 1065Native Go bindings for D-Bus
https://github.com/godbus/dbus
Native Go bindings for D-Bus. Contribute to godbus/dbus development by creating an account on GitHub.
- 1066YAML-based Dependency Injection container for GO
https://github.com/gontainer/gontainer
YAML-based Dependency Injection container for GO. Contribute to gontainer/gontainer development by creating an account on GitHub.
- 1067A lightning fast image processing and resizing library for Go
https://github.com/davidbyttow/govips
A lightning fast image processing and resizing library for Go - davidbyttow/govips
- 1068Convert digit strings between arbitrary bases.
https://github.com/bobg/basexx
Convert digit strings between arbitrary bases. Contribute to bobg/basexx development by creating an account on GitHub.
- 1069Image processing algorithms in pure Go
https://github.com/anthonynsimon/bild
Image processing algorithms in pure Go. Contribute to anthonynsimon/bild development by creating an account on GitHub.
- 1070:sunglasses:Package captcha provides an easy to use, unopinionated API for captcha generation
https://github.com/steambap/captcha
:sunglasses:Package captcha provides an easy to use, unopinionated API for captcha generation - steambap/captcha
- 1071lumberjack is a log rolling package for Go
https://github.com/natefinch/lumberjack
lumberjack is a log rolling package for Go. Contribute to natefinch/lumberjack development by creating an account on GitHub.
- 1072[Go] Lightweight eventbus with async compatibility for Go
https://github.com/asaskevich/EventBus
[Go] Lightweight eventbus with async compatibility for Go - asaskevich/EventBus
- 1073This is a simple skeleton for golang applications
https://github.com/wajox/gobase
This is a simple skeleton for golang applications. Contribute to wajox/gobase development by creating an account on GitHub.
- 1074Fake data generator
https://github.com/neotoolkit/faker
Fake data generator. Contribute to neotoolkit/faker development by creating an account on GitHub.
- 1075Sending line notifications using a binary, docker or Drone CI.
https://github.com/appleboy/drone-line
Sending line notifications using a binary, docker or Drone CI. - appleboy/drone-line
- 1076Go bindings for unarr (decompression library for RAR, TAR, ZIP and 7z archives)
https://github.com/gen2brain/go-unarr
Go bindings for unarr (decompression library for RAR, TAR, ZIP and 7z archives) - gen2brain/go-unarr
- 1077Golang project standard layout generator
https://github.com/insidieux/inizio
Golang project standard layout generator. Contribute to insidieux/inizio development by creating an account on GitHub.
- 1078Simple Logging Facade for Golang
https://github.com/echocat/slf4g
Simple Logging Facade for Golang. Contribute to echocat/slf4g development by creating an account on GitHub.
- 1079Calculate average score and rating based on Wilson Score Equation
https://github.com/kirillDanshin/avgRating
Calculate average score and rating based on Wilson Score Equation - kirillDanshin/avgRating
- 1080Go package for computer vision using OpenCV 4 and beyond. Includes support for DNN, CUDA, and OpenCV Contrib.
https://github.com/hybridgroup/gocv
Go package for computer vision using OpenCV 4 and beyond. Includes support for DNN, CUDA, and OpenCV Contrib. - hybridgroup/gocv
- 1081A logger for Go SQL database driver without modifying existing *sql.DB stdlib usage.
https://github.com/simukti/sqldb-logger
A logger for Go SQL database driver without modifying existing *sql.DB stdlib usage. - simukti/sqldb-logger
- 1082Lightweight library that handles RabbitMQ auto-reconnect and publishing retry routine for you.
https://github.com/furdarius/rabbitroutine
Lightweight library that handles RabbitMQ auto-reconnect and publishing retry routine for you. - furdarius/rabbitroutine
- 1083Golang beautify data display for Humans
https://github.com/go-ffmt/ffmt
Golang beautify data display for Humans. Contribute to go-ffmt/ffmt development by creating an account on GitHub.
- 1084Golang counters for readers/writers
https://github.com/miolini/datacounter
Golang counters for readers/writers. Contribute to miolini/datacounter development by creating an account on GitHub.
- 1085Go Image Filtering Toolkit
https://github.com/disintegration/gift
Go Image Filtering Toolkit. Contribute to disintegration/gift development by creating an account on GitHub.
- 1086:notes: Minimalist websocket framework for Go
https://github.com/olahol/melody
:notes: Minimalist websocket framework for Go. Contribute to olahol/melody development by creating an account on GitHub.
- 1087Go-blueprint allows users to spin up a quick Go project using a popular framework
https://github.com/Melkeydev/go-blueprint
Go-blueprint allows users to spin up a quick Go project using a popular framework - Melkeydev/go-blueprint
- 1088Blazing fast, structured, leveled logging in Go.
https://github.com/uber-go/zap
Blazing fast, structured, leveled logging in Go. Contribute to uber-go/zap development by creating an account on GitHub.
- 1089⚡ HTTP/2 Apple Push Notification Service (APNs) push provider for Go — Send push notifications to iOS, tvOS, Safari and OSX apps, using the APNs HTTP/2 protocol.
https://github.com/sideshow/apns2
⚡ HTTP/2 Apple Push Notification Service (APNs) push provider for Go — Send push notifications to iOS, tvOS, Safari and OSX apps, using the APNs HTTP/2 protocol. - sideshow/apns2
- 1090Dependency Injection and Inversion of Control package
https://github.com/logrange/linker
Dependency Injection and Inversion of Control package - logrange/linker
- 1091mangos is a pure Golang implementation of nanomsg's "Scalablilty Protocols"
https://github.com/nanomsg/mangos
mangos is a pure Golang implementation of nanomsg's "Scalablilty Protocols" - nanomsg/mangos
- 1092Structured, pluggable logging for Go.
https://github.com/Sirupsen/logrus
Structured, pluggable logging for Go. Contribute to sirupsen/logrus development by creating an account on GitHub.
- 1093Golang implementation of the Paice/Husk Stemming Algorithm
https://github.com/rookii/paicehusk
Golang implementation of the Paice/Husk Stemming Algorithm - rookii/paicehusk
- 1094Configuration and dependency injection
http://github.com/boot-go/boot
Configuration and dependency injection. Contribute to boot-go/boot development by creating an account on GitHub.
- 1095A selection of image manipulation tools
https://github.com/hawx/img
A selection of image manipulation tools. Contribute to hawx/img development by creating an account on GitHub.
- 1096Go Project Sample Layout
https://github.com/zitryss/go-sample
Go Project Sample Layout. Contribute to zitryss/go-sample development by creating an account on GitHub.
- 1097a golang log lib supports level and multi handlers
https://github.com/siddontang/go-log
a golang log lib supports level and multi handlers - siddontang/go-log
- 1098A library for scheduling when to dispatch a message to a channel
https://github.com/alexsniffin/gosd
A library for scheduling when to dispatch a message to a channel - alexsniffin/gosd
- 1099A Go project template
https://github.com/lacion/cookiecutter-golang
A Go project template. Contribute to lacion/cookiecutter-golang development by creating an account on GitHub.
- 1100Build event-driven and event streaming applications with ease
https://github.com/jeroenrinzema/commander
Build event-driven and event streaming applications with ease - jeroenrinzema/commander
- 1101Package raw enables reading and writing data at the device driver level for a network interface. MIT Licensed.
https://github.com/mdlayher/raw
Package raw enables reading and writing data at the device driver level for a network interface. MIT Licensed. - mdlayher/raw
- 1102Full-featured test framework for Go! Assertions, fuzzing, input testing, output capturing, and much more! 🍕
https://github.com/MarvinJWendt/testza
Full-featured test framework for Go! Assertions, fuzzing, input testing, output capturing, and much more! 🍕 - MarvinJWendt/testza
- 1103bobg/encid
https://github.com/bobg/encid
Contribute to bobg/encid development by creating an account on GitHub.
- 1104Provide Go Statistics Handler, Struct, Measure Method
https://github.com/osamingo/gosh
Provide Go Statistics Handler, Struct, Measure Method - osamingo/gosh
- 1105A tiny debug logging tool. Ideal for CLI tools and command applications. Inspired by https://github.com/visionmedia/debug
https://github.com/clok/kemba
A tiny debug logging tool. Ideal for CLI tools and command applications. Inspired by https://github.com/visionmedia/debug - clok/kemba
- 1106A multilingual command line sentence tokenizer in Golang
https://github.com/neurosnap/sentences
A multilingual command line sentence tokenizer in Golang - neurosnap/sentences
- 1107PowerDNS-Auth API client for Go (community project)
https://github.com/joeig/go-powerdns
PowerDNS-Auth API client for Go (community project) - joeig/go-powerdns
- 1108porter stemmer
https://github.com/a2800276/porter
porter stemmer. Contribute to a2800276/porter development by creating an account on GitHub.
- 1109String utilities for Go
https://github.com/ozgio/strutil
String utilities for Go. Contribute to ozgio/strutil development by creating an account on GitHub.
- 1110Unfancy resources embedding for Go with out of box http.FileSystem support.
https://github.com/omeid/go-resources
Unfancy resources embedding for Go with out of box http.FileSystem support. - omeid/go-resources
- 1111A collection of packages to augment the go testing package and support common patterns.
https://github.com/gotestyourself/gotest.tools
A collection of packages to augment the go testing package and support common patterns. - gotestyourself/gotest.tools
- 1112A toolkit with common assertions and mocks that plays nicely with the standard library
https://github.com/stretchr/testify
A toolkit with common assertions and mocks that plays nicely with the standard library - stretchr/testify
- 1113Reverse proxy with automatically obtains TLS certificates from Let's Encrypt
https://github.com/rekby/lets-proxy2
Reverse proxy with automatically obtains TLS certificates from Let's Encrypt - rekby/lets-proxy2
- 1114RES Service protocol library for Go
https://github.com/jirenius/go-res
RES Service protocol library for Go. Contribute to jirenius/go-res development by creating an account on GitHub.
- 1115An image resizing server written in Go
https://github.com/thoas/picfit
An image resizing server written in Go. Contribute to thoas/picfit development by creating an account on GitHub.
- 1116Asn.1 BER and DER encoding library for golang.
https://github.com/PromonLogicalis/asn1
Asn.1 BER and DER encoding library for golang. Contribute to Logicalis/asn1 development by creating an account on GitHub.
- 1117Simple mDNS client/server library in Golang
https://github.com/hashicorp/mdns
Simple mDNS client/server library in Golang. Contribute to hashicorp/mdns development by creating an account on GitHub.
- 1118"结巴"中文分词的Golang版本
https://github.com/yanyiwu/gojieba
"结巴"中文分词的Golang版本. Contribute to yanyiwu/gojieba development by creating an account on GitHub.
- 1119Populate go command line app flags from config struct
https://github.com/artyom/autoflags
Populate go command line app flags from config struct - artyom/autoflags
- 1120embed.FS wrapper providing additional functionality
https://github.com/leaanthony/debme
embed.FS wrapper providing additional functionality - leaanthony/debme
- 1121🧩 Template for a typical module written on Go.
https://github.com/octomation/go-module
🧩 Template for a typical module written on Go. Contribute to octomation/go-module development by creating an account on GitHub.
- 1122A repository for plotting and visualizing data
https://github.com/gonum/plot
A repository for plotting and visualizing data. Contribute to gonum/plot development by creating an account on GitHub.
- 1123Recreates directory and files from embedded filesystem using Go 1.16 embed.FS type.
https://github.com/soypat/rebed
Recreates directory and files from embedded filesystem using Go 1.16 embed.FS type. - soypat/rebed
- 1124Query Parser for REST
https://github.com/timsolov/rest-query-parser
Query Parser for REST. Contribute to timsolov/rest-query-parser development by creating an account on GitHub.
- 1125Cgo binding for libtextcat C library
https://github.com/goodsign/libtextcat
Cgo binding for libtextcat C library. Contribute to goodsign/libtextcat development by creating an account on GitHub.
- 1126Small package which wraps error responses to follow jsonapi.org
https://github.com/ddymko/go-jsonerror
Small package which wraps error responses to follow jsonapi.org - ddymko/go-jsonerror
- 1127Fastest structured logging
https://github.com/phuslu/log
Fastest structured logging. Contribute to phuslu/log development by creating an account on GitHub.
- 1128Prevent your secrets from leaking into logs, std* etc.
https://github.com/rsjethani/secret
Prevent your secrets from leaking into logs, std* etc. - rsjethani/secret
- 1129🌰 encrypt/decrypt using ssh keys
https://github.com/ssh-vault/ssh-vault
🌰 encrypt/decrypt using ssh keys. Contribute to ssh-vault/ssh-vault development by creating an account on GitHub.
- 1130Professional lightweight testing mini-framework for Go.
https://github.com/matryer/is
Professional lightweight testing mini-framework for Go. - matryer/is
- 1131golang client library to Viessmann Vitotrol web service
https://github.com/maxatome/go-vitotrol
golang client library to Viessmann Vitotrol web service - maxatome/go-vitotrol
- 1132Package assocentity returns the mean distance from tokens to an entity and its synonyms
https://github.com/ndabAP/assocentity
Package assocentity returns the mean distance from tokens to an entity and its synonyms - ndabAP/assocentity
- 1133tools for working with streams of data
https://github.com/nytlabs/streamtools
tools for working with streams of data. Contribute to nytlabs/streamtools development by creating an account on GitHub.
- 1134Go testing in the browser. Integrates with `go test`. Write behavioral tests in Go.
https://github.com/smartystreets/goconvey/
Go testing in the browser. Integrates with `go test`. Write behavioral tests in Go. - smartystreets/goconvey
- 1135[Deprecated] Protocol Buffers for Go with Gadgets
https://github.com/gogo/protobuf
[Deprecated] Protocol Buffers for Go with Gadgets. Contribute to gogo/protobuf development by creating an account on GitHub.
- 1136dependency tool for go
https://github.com/tools/godep
dependency tool for go. Contribute to tools/godep development by creating an account on GitHub.
- 1137Minimal and Beautiful Go testing framework
https://github.com/franela/goblin
Minimal and Beautiful Go testing framework. Contribute to franela/goblin development by creating an account on GitHub.
- 1138Vendor Go dependencies
https://github.com/jingweno/nut
Vendor Go dependencies. Contribute to owenthereal/nut development by creating an account on GitHub.
- 1139A Go library help testing your RESTful API application
https://github.com/yookoala/restit
A Go library help testing your RESTful API application - go-restit/restit
- 1140:lock: acmetool, an automatic certificate acquisition tool for ACME (Let's Encrypt)
https://github.com/hlandau/acme
:lock: acmetool, an automatic certificate acquisition tool for ACME (Let's Encrypt) - hlandau/acmetool
- 1141Simple Dependency Injection Container
https://github.com/vardius/gocontainer
Simple Dependency Injection Container. Contribute to vardius/gocontainer development by creating an account on GitHub.
- 1142A Go-based framework has been developed to oversee the execution of workflows delineated by directed acyclic graphs (DAGs).
https://github.com/rhosocial/go-dag
A Go-based framework has been developed to oversee the execution of workflows delineated by directed acyclic graphs (DAGs). - rhosocial/go-dag
- 1143A go implementation of the STUN client (RFC 3489 and RFC 5389)
https://github.com/ccding/go-stun
A go implementation of the STUN client (RFC 3489 and RFC 5389) - ccding/go-stun
- 1144Declare AMQP entities like queues, producers, and consumers in a declarative way. Can be used to work with RabbitMQ.
https://github.com/cheshir/go-mq
Declare AMQP entities like queues, producers, and consumers in a declarative way. Can be used to work with RabbitMQ. - cheshir/go-mq
- 1145Antch, a fast, powerful and extensible web crawling & scraping framework for Go
https://github.com/antchfx/antch
Antch, a fast, powerful and extensible web crawling & scraping framework for Go - antchfx/antch
- 1146a generic object pool for golang
https://github.com/jolestar/go-commons-pool
a generic object pool for golang. Contribute to jolestar/go-commons-pool development by creating an account on GitHub.
- 1147A 12-factor app logger built for performance and happy development
https://github.com/mgutz/logxi
A 12-factor app logger built for performance and happy development - mgutz/logxi
- 1148Make IoT a lot more fun with data.
https://github.com/xcodersun/eywa
Make IoT a lot more fun with data. . Contribute to xcodersun/eywa development by creating an account on GitHub.
- 1149Argon2 password hashing package for go with constant time hash comparison
https://github.com/raja/argon2pw
Argon2 password hashing package for go with constant time hash comparison - raja/argon2pw
- 1150kirillDanshin/llb
https://github.com/kirillDanshin/llb
Contribute to kirillDanshin/llb development by creating an account on GitHub.
- 1151An ordinary differential equation solving library in golang.
https://github.com/ChristopherRabotin/ode
An ordinary differential equation solving library in golang. - ChristopherRabotin/ode
- 1152golang common library
https://github.com/kubeservice-stack/common
golang common library. Contribute to kubeservice-stack/common development by creating an account on GitHub.
- 1153Read and use word2vec vectors in Go
https://github.com/danieldk/go2vec
Read and use word2vec vectors in Go. Contribute to danieldk/go2vec development by creating an account on GitHub.
- 1154ID hashing and Obfuscation using Knuth's Algorithm
https://github.com/pjebs/optimus-go
ID hashing and Obfuscation using Knuth's Algorithm - pjebs/optimus-go
- 1155Gorgonia is a library that helps facilitate machine learning in Go.
https://github.com/gorgonia/gorgonia
Gorgonia is a library that helps facilitate machine learning in Go. - gorgonia/gorgonia
- 1156go generate based graphql server library
https://github.com/99designs/gqlgen
go generate based graphql server library. Contribute to 99designs/gqlgen development by creating an account on GitHub.
- 1157This tool can be useful for writing a tests. If you want change private field in struct from imported libraries than it can help you.
https://github.com/dedalqq/omg.testingtools
This tool can be useful for writing a tests. If you want change private field in struct from imported libraries than it can help you. - dedalqq/omg.testingtools
- 1158go-glmatrix is a golang version of glMatrix, which is "designed to perform vector and matrix operations stupidly fast".
https://github.com/technohippy/go-glmatrix
go-glmatrix is a golang version of glMatrix, which is "designed to perform vector and matrix operations stupidly fast". - technohippy/go-glmatrix
- 1159Structured, pluggable logging for Go.
https://github.com/sirupsen/logrus
Structured, pluggable logging for Go. Contribute to sirupsen/logrus development by creating an account on GitHub.
- 1160Some utilities for Persian language in Go (Golang)
https://github.com/mavihq/persian
Some utilities for Persian language in Go (Golang) - mavihq/persian
- 1161:book: A Golang library for text processing, including tokenization, part-of-speech tagging, and named-entity extraction.
https://github.com/jdkato/prose
:book: A Golang library for text processing, including tokenization, part-of-speech tagging, and named-entity extraction. - jdkato/prose
- 1162:sparkles: EasyTCP is a light-weight TCP framework written in Go (Golang), built with message router. EasyTCP helps you build a TCP server easily fast and less painful.
https://github.com/DarthPestilane/easytcp
:sparkles: :rocket: EasyTCP is a light-weight TCP framework written in Go (Golang), built with message router. EasyTCP helps you build a TCP server easily fast and less painful. - GitHub - DarthPes...
- 1163Architecture checks for Go projects
https://github.com/fdaines/arch-go
Architecture checks for Go projects. Contribute to arch-go/arch-go development by creating an account on GitHub.
- 1164Pure Go implementation of the WebRTC API
https://github.com/pions/webrtc
Pure Go implementation of the WebRTC API. Contribute to pion/webrtc development by creating an account on GitHub.
- 1165Test your command line interfaces on windows, linux and osx and nodes viá ssh and docker
https://github.com/SimonBaeumer/commander
Test your command line interfaces on windows, linux and osx and nodes viá ssh and docker - commander-cli/commander
- 1166Streaming approximate histograms in Go
https://github.com/VividCortex/gohistogram
Streaming approximate histograms in Go. Contribute to VividCortex/gohistogram development by creating an account on GitHub.
- 1167A logging library with strack traces, object dumping and optional timestamps
https://github.com/pieterclaerhout/go-log
A logging library with strack traces, object dumping and optional timestamps - pieterclaerhout/go-log
- 1168A simple to use log system, minimalist but with features for debugging and differentiation of messages
https://github.com/structy/log
A simple to use log system, minimalist but with features for debugging and differentiation of messages - structy/log
- 1169🪽 An open, easy, fast, reliable and battery-efficient solution for real-time communications
https://github.com/dunglas/mercure
🪽 An open, easy, fast, reliable and battery-efficient solution for real-time communications - dunglas/mercure
- 1170Jazigo is a tool written in Go for retrieving configuration for multiple devices, similar to rancid, fetchconfig, oxidized, Sweet.
https://github.com/udhos/jazigo
Jazigo is a tool written in Go for retrieving configuration for multiple devices, similar to rancid, fetchconfig, oxidized, Sweet. - udhos/jazigo
- 1171A Go port of the Rapid Automatic Keyword Extraction algorithm (RAKE)
https://github.com/afjoseph/RAKE.Go
A Go port of the Rapid Automatic Keyword Extraction algorithm (RAKE) - afjoseph/RAKE.Go
- 1172Uniqush is a free and open source software system which provides a unified push service for server side notification to apps on mobile devices.
https://github.com/uniqush/uniqush-push
Uniqush is a free and open source software system which provides a unified push service for server side notification to apps on mobile devices. - uniqush/uniqush-push
- 1173📑 Lightweight, configurable, extensible logging library written in Go. Support multi level, multi outputs and built-in multi file logger, buffers, clean, rotate-file handling.一个易于使用的,轻量级、可配置、可扩展的日志库。支持多个级别,输出到多文件;内置文件日志处理、自动切割、清理、压缩等增强功能
https://github.com/gookit/slog
📑 Lightweight, configurable, extensible logging library written in Go. Support multi level, multi outputs and built-in multi file logger, buffers, clean, rotate-file handling.一个易于使用的,轻量级、可配置、可扩展的日志...
- 1174RabbitMQ Reconnection client
https://github.com/sbabiv/rmqconn
RabbitMQ Reconnection client. Contribute to sbabiv/rmqconn development by creating an account on GitHub.
- 1175Go support for Google's protocol buffers
https://github.com/golang/protobuf
Go support for Google's protocol buffers. Contribute to golang/protobuf development by creating an account on GitHub.
- 1176:incoming_envelope: A fast Message/Event Hub using publish/subscribe pattern with support for topics like* rabbitMQ exchanges for Go applications
https://github.com/leandro-lugaresi/hub
:incoming_envelope: A fast Message/Event Hub using publish/subscribe pattern with support for topics like* rabbitMQ exchanges for Go applications - leandro-lugaresi/hub
- 1177Barebones dependency manager for Go.
https://github.com/pote/gpm
Barebones dependency manager for Go. Contribute to pote/gpm development by creating an account on GitHub.
- 1178Contains primitives for marshaling/unmarshaling Unix timestamp/epoch to/from built-in time.Time type in JSON
https://github.com/vtopc/epoch
Contains primitives for marshaling/unmarshaling Unix timestamp/epoch to/from built-in time.Time type in JSON - vtopc/epoch
- 1179persistence layer code generation for golang
https://github.com/marlow/marlow
persistence layer code generation for golang. Contribute to marlow/marlow development by creating an account on GitHub.
- 1180Gonum is a set of numeric libraries for the Go programming language. It contains libraries for matrices, statistics, optimization, and more
https://github.com/gonum/gonum
Gonum is a set of numeric libraries for the Go programming language. It contains libraries for matrices, statistics, optimization, and more - gonum/gonum
- 1181Golang text Transliterator (i.e München -> Muenchen)
https://github.com/alexsergivan/transliterator
Golang text Transliterator (i.e München -> Muenchen) - alexsergivan/transliterator
- 1182Turn Nginx logs into Prometheus metrics
https://github.com/blind-oracle/nginx-prometheus
Turn Nginx logs into Prometheus metrics. Contribute to blind-oracle/nginx-prometheus development by creating an account on GitHub.
- 1183Build LDAP services w/ Go
https://github.com/jimlambrt/gldap
Build LDAP services w/ Go. Contribute to jimlambrt/gldap development by creating an account on GitHub.
- 1184Select, put and delete data from JSON, TOML, YAML, XML and CSV files with a single tool. Supports conversion between formats and can be used as a Go package.
https://github.com/tomwright/dasel
Select, put and delete data from JSON, TOML, YAML, XML and CSV files with a single tool. Supports conversion between formats and can be used as a Go package. - TomWright/dasel
- 1185An aggregator for personal metrics, and an extensible analysis engine
https://github.com/connectordb/connectordb
An aggregator for personal metrics, and an extensible analysis engine - heedy/heedy
- 1186🤯 High-performance PHP application server, process manager written in Go and powered with plugins
https://github.com/spiral/roadrunner
🤯 High-performance PHP application server, process manager written in Go and powered with plugins - roadrunner-server/roadrunner
- 1187Enterprise-ready, GitOps enabled, CloudNative feature management solution
https://github.com/markphelps/flipt
Enterprise-ready, GitOps enabled, CloudNative feature management solution - flipt-io/flipt
- 1188Go binding for the cairo graphics library
https://github.com/ungerik/go-cairo
Go binding for the cairo graphics library. Contribute to ungerik/go-cairo development by creating an account on GitHub.
- 1189Jest-like snapshot testing in Go 📸
http://github.com/gkampitakis/go-snaps
Jest-like snapshot testing in Go 📸. Contribute to gkampitakis/go-snaps development by creating an account on GitHub.
- 1190A simple and light excel file reader to read a standard excel as a table faster | 一个轻量级的Excel数据读取库,用一种更`关系数据库`的方式解析Excel。
https://github.com/szyhf/go-excel
A simple and light excel file reader to read a standard excel as a table faster | 一个轻量级的Excel数据读取库,用一种更`关系数据库`的方式解析Excel。 - szyhf/go-excel
- 1191redisqueue provides a producer and consumer of a queue that uses Redis streams
https://github.com/robinjoseph08/redisqueue
redisqueue provides a producer and consumer of a queue that uses Redis streams - robinjoseph08/redisqueue
- 1192OpenAPI 3.x parser
https://github.com/neotoolkit/openapi
OpenAPI 3.x parser. Contribute to neotoolkit/openapi development by creating an account on GitHub.
- 1193A simple and flexible health check library for Go.
https://github.com/alexliesenfeld/health
A simple and flexible health check library for Go. - alexliesenfeld/health
- 1194Notification library for gophers and their furry friends.
https://github.com/containrrr/shoutrrr
Notification library for gophers and their furry friends. - containrrr/shoutrrr
- 1195A probably paranoid Golang utility library for securely hashing and encrypting passwords based on the Dropbox method. This implementation uses Blake2b, Scrypt and XSalsa20-Poly1305 (via NaCl SecretBox) to create secure password hashes that are also encrypted using a master passphrase.
https://github.com/dwin/goSecretBoxPassword
A probably paranoid Golang utility library for securely hashing and encrypting passwords based on the Dropbox method. This implementation uses Blake2b, Scrypt and XSalsa20-Poly1305 (via NaCl Secret...
- 1196Moved to https://gitea.com/lunny/gop
https://github.com/lunny/gop
Moved to https://gitea.com/lunny/gop. Contribute to lunny/gop development by creating an account on GitHub.
- 1197Time based rotating file writer
https://github.com/utahta/go-cronowriter
Time based rotating file writer. Contribute to utahta/go-cronowriter development by creating an account on GitHub.
- 1198Golang framework for robotics, drones, and the Internet of Things (IoT)
https://github.com/hybridgroup/gobot/
Golang framework for robotics, drones, and the Internet of Things (IoT) - hybridgroup/gobot
- 1199A simple SHOUTcast server.
https://github.com/krotik/dudeldu
A simple SHOUTcast server. Contribute to krotik/dudeldu development by creating an account on GitHub.
- 1200A Go library for performing Unicode Text Segmentation as described in Unicode Standard Annex #29
https://github.com/blevesearch/segment
A Go library for performing Unicode Text Segmentation as described in Unicode Standard Annex #29 - blevesearch/segment
- 1201Go library for reading and writing XLSX files.
https://github.com/tealeg/xlsx
Go library for reading and writing XLSX files. . Contribute to tealeg/xlsx development by creating an account on GitHub.
- 1202Gorse open source recommender system engine
https://github.com/zhenghaoz/gorse
Gorse open source recommender system engine. Contribute to gorse-io/gorse development by creating an account on GitHub.
- 1203A high-performance non-blocking I/O networking framework focusing on RPC scenarios.
https://github.com/cloudwego/netpoll
A high-performance non-blocking I/O networking framework focusing on RPC scenarios. - cloudwego/netpoll
- 1204Make SSH apps, just like that! 💫
https://github.com/charmbracelet/wish
Make SSH apps, just like that! 💫. Contribute to charmbracelet/wish development by creating an account on GitHub.
- 1205Simple, reliable, and efficient distributed task queue in Go
https://github.com/hibiken/asynq
Simple, reliable, and efficient distributed task queue in Go - hibiken/asynq
- 1206Random Forest implementation in golang
https://github.com/malaschitz/randomForest
Random Forest implementation in golang. Contribute to malaschitz/randomForest development by creating an account on GitHub.
- 1207Stemmer packages for Go programming language. Includes English, German and Dutch stemmers.
https://github.com/dchest/stemmer
Stemmer packages for Go programming language. Includes English, German and Dutch stemmers. - dchest/stemmer
- 1208CGo bindings to Yandex.Mystem
https://github.com/dveselov/mystem
CGo bindings to Yandex.Mystem. Contribute to dveselov/mystem development by creating an account on GitHub.
- 1209A Go package for sending and receiving ethernet frames. Currently supporting Linux, Freebsd, and OS X.
https://github.com/songgao/ether
A Go package for sending and receiving ethernet frames. Currently supporting Linux, Freebsd, and OS X. - songgao/ether
- 1210My understanding of how to structure a golang project.
https://github.com/wangyoucao577/go-project-layout
My understanding of how to structure a golang project. - GitHub - wangyoucao577/go-project-layout: My understanding of how to structure a golang project.
- 1211gNXI Tools - gRPC Network Management/Operations Interface Tools
https://github.com/google/gnxi
gNXI Tools - gRPC Network Management/Operations Interface Tools - google/gnxi
- 1212Industrial IoT Messaging and Device Management Platform
https://github.com/Mainflux/mainflux
Industrial IoT Messaging and Device Management Platform - mainflux/mainflux
- 1213DI container library that is focused on clean API and flexibility.
https://github.com/HnH/di
DI container library that is focused on clean API and flexibility. - HnH/di
- 1214⚙️ A dependency injection toolkit based on Go 1.18+ Generics.
https://github.com/samber/do
⚙️ A dependency injection toolkit based on Go 1.18+ Generics. - samber/do
- 1215Linear algebra, eigenvalues, FFT, Bessel, elliptic, orthogonal polys, geometry, NURBS, numerical quadrature, 3D transfinite interpolation, random numbers, Mersenne twister, probability distributions, optimisation, differential equations.
https://github.com/cpmech/gosl
Linear algebra, eigenvalues, FFT, Bessel, elliptic, orthogonal polys, geometry, NURBS, numerical quadrature, 3D transfinite interpolation, random numbers, Mersenne twister, probability distribution...
- 1216Embed files into a Go executable
https://github.com/rakyll/statik
Embed files into a Go executable. Contribute to rakyll/statik development by creating an account on GitHub.
- 1217Zero Allocation JSON Logger
https://github.com/rs/zerolog
Zero Allocation JSON Logger. Contribute to rs/zerolog development by creating an account on GitHub.
- 1218BadActor.org An in-memory application driven jailer written in Go
https://github.com/jaredfolkins/badactor
BadActor.org An in-memory application driven jailer written in Go - jaredfolkins/badactor
- 1219Go HTTP client w/ HTTPS and SOCKS5 Proxy Load-balancing, Failover, Retries, Authentication, and RoundTripper
https://github.com/presbrey/go-multiproxy
Go HTTP client w/ HTTPS and SOCKS5 Proxy Load-balancing, Failover, Retries, Authentication, and RoundTripper - presbrey/go-multiproxy
- 1220Dynamically generate self-signed certificates and certificate authorities for Go tests
https://github.com/madflojo/testcerts
Dynamically generate self-signed certificates and certificate authorities for Go tests - madflojo/testcerts
- 1221Gmqtt is a flexible, high-performance MQTT broker library that fully implements the MQTT protocol V3.x and V5 in golang
https://github.com/DrmagicE/gmqtt
Gmqtt is a flexible, high-performance MQTT broker library that fully implements the MQTT protocol V3.x and V5 in golang - DrmagicE/gmqtt
- 1222t/cmd/xtemplate at main · youthlin/t
https://github.com/youthlin/t/blob/main/cmd/xtemplate
t: translation util for go, using GNU gettext. Contribute to youthlin/t development by creating an account on GitHub.
- 1223Genetic Algorithms library written in Go / golang
https://github.com/thoj/go-galib
Genetic Algorithms library written in Go / golang. Contribute to thoj/go-galib development by creating an account on GitHub.
- 1224An golang log lib, supports tracking and level, wrap by standard log lib
https://github.com/chzyer/logex
An golang log lib, supports tracking and level, wrap by standard log lib - chzyer/logex
- 1225State estimation and filtering algorithms in Go
https://github.com/milosgajdos/go-estimate
State estimation and filtering algorithms in Go. Contribute to milosgajdos/go-estimate development by creating an account on GitHub.
- 1226HTTP proxy handler and dialer
https://github.com/wzshiming/httpproxy
HTTP proxy handler and dialer. Contribute to wzshiming/httpproxy development by creating an account on GitHub.
- 1227Dependency Injection container for Golang projects.
https://github.com/NVIDIA/gontainer
Dependency Injection container for Golang projects. - NVIDIA/gontainer
- 1228Go efficient multilingual NLP and text segmentation; support English, Chinese, Japanese and others.
https://github.com/go-ego/gse
Go efficient multilingual NLP and text segmentation; support English, Chinese, Japanese and others. - go-ego/gse
- 1229GO Dependency Injection
https://github.com/go-kata/kinit
GO Dependency Injection. Contribute to go-kata/kinit development by creating an account on GitHub.
- 1230A BDD library for Go
https://github.com/stesla/gospecify
A BDD library for Go. Contribute to stesla/gospecify development by creating an account on GitHub.
- 1231User level X Keyboard Grabber
https://github.com/go-xkg/xkg
User level X Keyboard Grabber. Contribute to go-xkg/xkg development by creating an account on GitHub.
- 1232🚀Gev is a lightweight, fast non-blocking TCP network library / websocket server based on Reactor mode. Support custom protocols to quickly and easily build high-performance servers.
https://github.com/Allenxuxu/gev
🚀Gev is a lightweight, fast non-blocking TCP network library / websocket server based on Reactor mode. Support custom protocols to quickly and easily build high-performance servers. - GitHub - All...
- 1233Pure golang package for reading and writing xz-compressed files
https://github.com/ulikunitz/xz
Pure golang package for reading and writing xz-compressed files - ulikunitz/xz
- 1234Hit your API targets with rapid-fire precision using Go's fastest and simple HTTP Client.
https://github.com/opus-domini/fast-shot
Hit your API targets with rapid-fire precision using Go's fastest and simple HTTP Client. - opus-domini/fast-shot
- 1235A golang json library inspired by jsoniter
https://github.com/zerosnake0/jzon
A golang json library inspired by jsoniter. Contribute to zerosnake0/jzon development by creating an account on GitHub.
- 1236A Go testing framework for distributed applications
https://github.com/stv0g/gont
A Go testing framework for distributed applications - cunicu/gont
- 1237Simulate network link speed
https://github.com/ian-kent/linkio
Simulate network link speed. Contribute to ian-kent/linkio development by creating an account on GitHub.
- 1238Package goraph implements graph data structure and algorithms.
https://github.com/gyuho/goraph
Package goraph implements graph data structure and algorithms. - gyuho/goraph
- 1239Self-contained Japanese Morphological Analyzer written in pure Go
https://github.com/ikawaha/kagome
Self-contained Japanese Morphological Analyzer written in pure Go - ikawaha/kagome
- 1240🧑💻 High Developer Experience, zap based logger. It offers rich functionality but is easy to configure and allows the user to choose the best output format for their purposes.
https://github.com/nkmr-jp/zl
🧑💻 High Developer Experience, zap based logger. It offers rich functionality but is easy to configure and allows the user to choose the best output format for their purposes. - nkmr-jp/zl
- 1241A simple and fast Redis backed key-value store library for Go
https://github.com/gosuri/go-store
A simple and fast Redis backed key-value store library for Go - gosuri/go-store
- 1242Fast conversions across various Go types with a simple API.
https://github.com/cstockton/go-conv
Fast conversions across various Go types with a simple API. - cstockton/go-conv
- 1243NUMA is a utility library, which is written in go. It help us to write some NUMA-AWARED code.
https://github.com/lrita/numa
NUMA is a utility library, which is written in go. It help us to write some NUMA-AWARED code. - lrita/numa
- 1244Convert string to camel case, snake case, kebab case / slugify, custom delimiter, pad string, tease string and many other functionalities with help of by Stringy package.
https://github.com/gobeam/Stringy
Convert string to camel case, snake case, kebab case / slugify, custom delimiter, pad string, tease string and many other functionalities with help of by Stringy package. - gobeam/stringy
- 1245Simple and configurable Logging in Go, with level, formatters and writers
https://github.com/subchen/go-log
Simple and configurable Logging in Go, with level, formatters and writers - subchen/go-log
- 1246socket.io library for golang, a realtime application framework.
https://github.com/googollee/go-socket.io
socket.io library for golang, a realtime application framework. - googollee/go-socket.io
- 1247Cap'n Proto library and parser for go. This is go-capnproto-1.0, and does not have rpc. See https://github.com/zombiezen/go-capnproto2 for 2.0 which has rpc and capabilities.
https://github.com/glycerine/go-capnproto
Cap'n Proto library and parser for go. This is go-capnproto-1.0, and does not have rpc. See https://github.com/zombiezen/go-capnproto2 for 2.0 which has rpc and capabilities. - glycerine/go-cap...
- 1248Lightweight, generic & simple dependency injection (DI) container for Go
https://github.com/firasdarwish/ore
Lightweight, generic & simple dependency injection (DI) container for Go - firasdarwish/ore
- 1249Every grain of sand on Earth has its own ID.
https://github.com/aofei/sandid
Every grain of sand on Earth has its own ID. Contribute to aofei/sandid development by creating an account on GitHub.
- 1250An ERB-style templating language for Go.
https://github.com/benbjohnson/ego
An ERB-style templating language for Go. Contribute to benbjohnson/ego development by creating an account on GitHub.
- 1251Test suites support for standard Go1.7 "testing" by leveraging Subtests feature
https://github.com/pavlo/gosuite
Test suites support for standard Go1.7 "testing" by leveraging Subtests feature - pavlo/gosuite
- 1252A tokenizer based on the dictionary and Bigram language models for Go. (Now only support chinese segmentation)
https://github.com/xujiajun/gotokenizer
A tokenizer based on the dictionary and Bigram language models for Go. (Now only support chinese segmentation) - xujiajun/gotokenizer
- 1253Go MapSlice for ordered marshal/ unmarshal of maps in JSON
https://github.com/mickep76/mapslice-json
Go MapSlice for ordered marshal/ unmarshal of maps in JSON - ake-persson/mapslice-json
- 1254An entity framework for Go
https://github.com/facebook/ent
An entity framework for Go. Contribute to ent/ent development by creating an account on GitHub.
- 1255Optimized bit-level Reader and Writer for Go.
https://github.com/icza/bitio
Optimized bit-level Reader and Writer for Go. Contribute to icza/bitio development by creating an account on GitHub.
- 1256A tiny wrapper around NSQ topic and channel :rocket:
https://github.com/rafaeljesus/nsq-event-bus
A tiny wrapper around NSQ topic and channel :rocket: - rafaeljesus/nsq-event-bus
- 1257Golang Genetic Algorithm
https://github.com/tomcraven/goga
Golang Genetic Algorithm. Contribute to tomcraven/goga development by creating an account on GitHub.
- 1258JSON query expression library in Golang.
https://github.com/elgs/jsonql
JSON query expression library in Golang. Contribute to elgs/jsonql development by creating an account on GitHub.
- 1259Use Go Modules.
https://github.com/kardianos/govendor
Use Go Modules. Contribute to kardianos/govendor development by creating an account on GitHub.
- 1260BGP implemented in the Go Programming Language
https://github.com/osrg/gobgp
BGP implemented in the Go Programming Language. Contribute to osrg/gobgp development by creating an account on GitHub.
- 1261Sparse matrix formats for linear algebra supporting scientific and machine learning applications
https://github.com/james-bowman/sparse
Sparse matrix formats for linear algebra supporting scientific and machine learning applications - james-bowman/sparse
- 1262Project Flogo is an open source ecosystem of opinionated event-driven capabilities to simplify building efficient & modern serverless functions, microservices & edge apps.
https://github.com/tibcosoftware/flogo
Project Flogo is an open source ecosystem of opinionated event-driven capabilities to simplify building efficient & modern serverless functions, microservices & edge apps. - TIBCOSoftware/...
- 1263A dead-simple, extensible MQTT implementation well suited for embedded systems.
https://github.com/soypat/natiu-mqtt
A dead-simple, extensible MQTT implementation well suited for embedded systems. - GitHub - soypat/natiu-mqtt: A dead-simple, extensible MQTT implementation well suited for embedded systems.
- 1264Logging library for Golang
https://github.com/lajosbencz/glo
Logging library for Golang. Contribute to lajosbencz/glo development by creating an account on GitHub.
- 1265Self-contained Machine Learning and Natural Language Processing library in Go
https://github.com/nlpodyssey/spago
Self-contained Machine Learning and Natural Language Processing library in Go - nlpodyssey/spago
- 1266Pure Go 1000k+ connections solution, support tls/http1.x/websocket and basically compatible with net/http, with high-performance and low memory cost, non-blocking, event-driven, easy-to-use.
https://github.com/lesismal/nbio
Pure Go 1000k+ connections solution, support tls/http1.x/websocket and basically compatible with net/http, with high-performance and low memory cost, non-blocking, event-driven, easy-to-use. - lesi...
- 1267Client-customizable JSON formats for dynamic APIs
https://github.com/cocoonspace/dynjson
Client-customizable JSON formats for dynamic APIs. Contribute to cocoonspace/dynjson development by creating an account on GitHub.
- 1268golang long polling library. Makes web pub-sub easy via HTTP long-poll servers and clients :coffee: :computer:
https://github.com/jcuga/golongpoll
golang long polling library. Makes web pub-sub easy via HTTP long-poll servers and clients :smiley: :coffee: :computer: - GitHub - jcuga/golongpoll: golang long polling library. Makes web pub-sub...
- 1269:file_folder: Embeds static resources into go files for single binary compilation + works with http.FileSystem + symlinks
https://github.com/go-playground/statics
:file_folder: Embeds static resources into go files for single binary compilation + works with http.FileSystem + symlinks - go-playground/statics
- 1270A Crypto-Secure Reliable-UDP Library for golang with FEC
https://github.com/xtaci/kcp-go
A Crypto-Secure Reliable-UDP Library for golang with FEC - GitHub - xtaci/kcp-go: A Crypto-Secure Reliable-UDP Library for golang with FEC
- 1271Sentimental Analysis Microservice
https://github.com/PIMPfiction/govader_backend
Sentimental Analysis Microservice. Contribute to PIMPfiction/govader_backend development by creating an account on GitHub.
- 1272Use anacrolix/go-libutp instead
https://github.com/anacrolix/utp
Use anacrolix/go-libutp instead. Contribute to anacrolix/utp development by creating an account on GitHub.
- 1273database to golang struct
https://github.com/xxjwxc/gormt
database to golang struct. Contribute to xxjwxc/gormt development by creating an account on GitHub.
- 1274Sarama is a Go library for Apache Kafka.
https://github.com/Shopify/sarama
Sarama is a Go library for Apache Kafka. Contribute to IBM/sarama development by creating an account on GitHub.
- 1275Easily and dynamically generate maps from Go static structures
https://github.com/tuvistavie/structomap
Easily and dynamically generate maps from Go static structures - danhper/structomap
- 1276Super short, fully unique, non-sequential and URL friendly Ids
https://github.com/teris-io/shortid
Super short, fully unique, non-sequential and URL friendly Ids - teris-io/shortid
- 1277Easy and fluent Go cron scheduling. This is a fork from https://github.com/jasonlvhit/gocron
https://github.com/go-co-op/gocron
Easy and fluent Go cron scheduling. This is a fork from https://github.com/jasonlvhit/gocron - go-co-op/gocron
- 1278NEAT (NeuroEvolution of Augmenting Topologies) implemented in Go
https://github.com/jinyeom/neat
NEAT (NeuroEvolution of Augmenting Topologies) implemented in Go - jinyeom/neat
- 1279Fast IP to CIDR lookup in Golang
https://github.com/yl2chen/cidranger
Fast IP to CIDR lookup in Golang. Contribute to yl2chen/cidranger development by creating an account on GitHub.
- 1280liblinear bindings for Go
https://github.com/danieldk/golinear
liblinear bindings for Go. Contribute to danieldk/golinear development by creating an account on GitHub.
- 1281Event Bus package for Go
https://github.com/stanipetrosyan/go-eventbus
Event Bus package for Go. Contribute to stanipetrosyan/go-eventbus development by creating an account on GitHub.
- 1282Excel binding to struct written in Go.(Only supports Go1.18+)
https://github.com/go-the-way/exl
Excel binding to struct written in Go.(Only supports Go1.18+) - go-the-way/exl
- 1283An easy way to add useful startup banners into your Go applications
https://github.com/dimiro1/banner
An easy way to add useful startup banners into your Go applications - dimiro1/banner
- 1284GoStats is a go library for math statistics mostly used in ML domains, it covers most of the statistical measures functions.
https://github.com/OGFris/GoStats
GoStats is a go library for math statistics mostly used in ML domains, it covers most of the statistical measures functions. - OGFris/GoStats
- 1285An easy to use, extensible health check library for Go applications.
https://github.com/dimiro1/health
An easy to use, extensible health check library for Go applications. - dimiro1/health
- 1286Starter-kit for writing services in Go using Kubernetes.
https://github.com/ardanlabs/service
Starter-kit for writing services in Go using Kubernetes. - ardanlabs/service
- 1287gojek/darkroom
https://github.com/gojek/darkroom
Contribute to gojek/darkroom development by creating an account on GitHub.
- 1288Go simple async message bus
https://github.com/vardius/message-bus
Go simple async message bus. Contribute to vardius/message-bus development by creating an account on GitHub.
- 1289Digital Signal Processing for Go
https://github.com/mjibson/go-dsp
Digital Signal Processing for Go. Contribute to maddyblue/go-dsp development by creating an account on GitHub.
- 1290A library for working with IP addresses and networks in Go
https://github.com/c-robinson/iplib
A library for working with IP addresses and networks in Go - c-robinson/iplib
- 1291Graph algorithms and data structures
https://github.com/yourbasic/graph
Graph algorithms and data structures. Contribute to yourbasic/graph development by creating an account on GitHub.
- 1292A golang library for packing and unpacking hosts list
https://github.com/Wing924/hostutils
A golang library for packing and unpacking hosts list - Wing924/hostutils
- 1293:key: Idiotproof golang password validation library inspired by Python's passlib
https://github.com/hlandau/passlib
:key: Idiotproof golang password validation library inspired by Python's passlib - hlandau/passlib
- 1294Logur is an opinionated collection of logging best practices
https://github.com/logur/logur
Logur is an opinionated collection of logging best practices - logur/logur
- 1295A high-performance, recursive DNS resolver server with DNSSEC support, focused on preserving privacy.
https://github.com/semihalev/sdns
A high-performance, recursive DNS resolver server with DNSSEC support, focused on preserving privacy. - semihalev/sdns
- 1296websocket based messaging server written in golang
https://github.com/smancke/guble
websocket based messaging server written in golang - smancke/guble
- 1297Ratus is a RESTful asynchronous task queue server. It translated concepts of distributed task queues into a set of resources that conform to REST principles and provides a consistent HTTP API for various backends.
https://github.com/hyperonym/ratus
Ratus is a RESTful asynchronous task queue server. It translated concepts of distributed task queues into a set of resources that conform to REST principles and provides a consistent HTTP API for v...
- 1298Logging, distilled
https://github.com/amoghe/distillog
Logging, distilled. Contribute to amoghe/distillog development by creating an account on GitHub.
- 1299Generate test data from SQL files before testing and clear it after finished.
https://github.com/zhulongcheng/testsql
Generate test data from SQL files before testing and clear it after finished. - zhulongcheng/testsql
- 1300Go bindings for GLFW 3
https://github.com/go-gl/glfw
Go bindings for GLFW 3. Contribute to go-gl/glfw development by creating an account on GitHub.
- 1301A Go (golang) package providing high-performance asynchronous logging, message filtering by severity and category, and multiple message targets.
https://github.com/go-ozzo/ozzo-log
A Go (golang) package providing high-performance asynchronous logging, message filtering by severity and category, and multiple message targets. - go-ozzo/ozzo-log
- 1302🌈 slog.Handler that writes tinted (colorized) logs
https://github.com/lmittmann/tint
🌈 slog.Handler that writes tinted (colorized) logs - lmittmann/tint
- 1303Machine is a workflow/pipeline library for processing data
https://github.com/whitaker-io/machine
Machine is a workflow/pipeline library for processing data - whitaker-io/machine
- 1304An avatar generator for Go.
https://github.com/aofei/cameron
An avatar generator for Go. Contribute to aofei/cameron development by creating an account on GitHub.
- 1305Go package for fast high-level image processing powered by libvips C library
https://github.com/h2non/bimg
Go package for fast high-level image processing powered by libvips C library - h2non/bimg
- 1306Fluent API to make it easier to create Json objects.
https://github.com/ricardolonga/jsongo
Fluent API to make it easier to create Json objects. - ricardolonga/jsongo
- 1307:ledger: Yet another minimalist logging library
https://github.com/jfcg/yell
:ledger: Yet another minimalist logging library. Contribute to jfcg/yell development by creating an account on GitHub.
- 1308Go bindings for FANN, library for artificial neural networks
https://github.com/white-pony/go-fann
Go bindings for FANN, library for artificial neural networks - vksnk/go-fann
- 1309A reflection based dependency injection toolkit for Go.
https://github.com/uber-go/dig
A reflection based dependency injection toolkit for Go. - uber-go/dig
- 1310🚨 slog: Attribute formatting
https://github.com/samber/slog-formatter
🚨 slog: Attribute formatting. Contribute to samber/slog-formatter development by creating an account on GitHub.
- 1311:chart_with_upwards_trend: Monitors Go MemStats + System stats such as Memory, Swap and CPU and sends via UDP anywhere you want for logging etc...
https://github.com/go-playground/stats
:chart_with_upwards_trend: Monitors Go MemStats + System stats such as Memory, Swap and CPU and sends via UDP anywhere you want for logging etc... - go-playground/stats
- 1312Chinese word splitting algorithm MMSEG in GO
https://github.com/awsong/MMSEGO
Chinese word splitting algorithm MMSEG in GO. Contribute to awsong/MMSEGO development by creating an account on GitHub.
- 1313Simple sitemap formatting, with a little syntactic sugar
https://github.com/mingard/sitemap-format
Simple sitemap formatting, with a little syntactic sugar - mingard/sitemap-format
- 1314Go bindings for YARA
https://github.com/hillu/go-yara
Go bindings for YARA. Contribute to hillu/go-yara development by creating an account on GitHub.
- 1315Go pkg for returning your public facing IP address.
https://github.com/polera/publicip
Go pkg for returning your public facing IP address. - polera/publicip
- 1316High performance minimalism async-io(proactor) networking for Golang.
https://github.com/xtaci/gaio
High performance minimalism async-io(proactor) networking for Golang. - xtaci/gaio
- 1317A convenience library for generating, comparing and inspecting password hashes using the scrypt KDF in Go 🔑
https://github.com/elithrar/simple-scrypt
A convenience library for generating, comparing and inspecting password hashes using the scrypt KDF in Go 🔑 - elithrar/simple-scrypt
- 1318Simple and blazing fast lockfree logging library for golang
https://github.com/kpango/glg
Simple and blazing fast lockfree logging library for golang - kpango/glg
- 1319cheek: a pico-sized declarative job scheduler
https://github.com/datarootsio/cheek
cheek: a pico-sized declarative job scheduler. Contribute to datarootsio/cheek development by creating an account on GitHub.
- 1320Simple and fast template engine for Go
https://github.com/valyala/fasttemplate
Simple and fast template engine for Go. Contribute to valyala/fasttemplate development by creating an account on GitHub.
- 1321Huggingface transformer pipelines in Golang
https://github.com/knights-analytics/hugot
Huggingface transformer pipelines in Golang. Contribute to knights-analytics/hugot development by creating an account on GitHub.
- 1322Golang type-safe dependency injection
https://github.com/muir/nject
Golang type-safe dependency injection. Contribute to muir/nject development by creating an account on GitHub.
- 1323LINEヤフー for Business|LINEヤフーが提供する法人向けサービス
https://at.line.me/en
LINEヤフーが提供する法人向けサービス(広告・コミュニケーション・販促・データソリューション)の概要、導入事例、媒体資料、イベント・セミナー情報などを紹介。各種サービスの管理画面ログイン、申し込みや問い合わせもこちらから。
- 1324A Cloud Native traffic orchestration system
https://github.com/megaease/easegress
A Cloud Native traffic orchestration system. Contribute to easegress-io/easegress development by creating an account on GitHub.
- 1325Random fake data generator written in go
https://github.com/brianvoe/gofakeit
Random fake data generator written in go. Contribute to brianvoe/gofakeit development by creating an account on GitHub.
- 1326Gountries provides: Countries (ISO-3166-1), Country Subdivisions(ISO-3166-2), Currencies (ISO 4217), Geo Coordinates(ISO-6709) as well as translations, country borders and other stuff exposed as struct data.
https://github.com/pariz/gountries
Gountries provides: Countries (ISO-3166-1), Country Subdivisions(ISO-3166-2), Currencies (ISO 4217), Geo Coordinates(ISO-6709) as well as translations, country borders and other stuff exposed as st...
- 1327Golang Super Simple Load Balance
https://github.com/eduardonunesp/sslb
Golang Super Simple Load Balance. Contribute to eduardonunesp/sslb development by creating an account on GitHub.
- 1328Simple and yet powerful Dependency Injection for Go
https://github.com/goioc/di
Simple and yet powerful Dependency Injection for Go - goioc/di
- 1329Dead simple, super fast, zero allocation logger for Golang
https://github.com/francoispqt/onelog
Dead simple, super fast, zero allocation logger for Golang - francoispqt/onelog
- 1330Go package for sending and receiving SSE (server-sent events)
https://github.com/lampctl/go-sse
Go package for sending and receiving SSE (server-sent events) - lampctl/go-sse
- 1331GO Implementation of Entropy Measures
https://github.com/kzahedi/goent
GO Implementation of Entropy Measures. Contribute to kzahedi/goent development by creating an account on GitHub.
- 1332goArgonPass is a Argon2 Password utility package for Go using the crypto library package Argon2 designed to be compatible with Passlib for Python and Argon2 PHP. Argon2 was the winner of the most recent Password Hashing Competition. This is designed for use anywhere password hashing and verification might be needed and is intended to replace implementations using bcrypt or Scrypt.
https://github.com/dwin/goArgonPass
goArgonPass is a Argon2 Password utility package for Go using the crypto library package Argon2 designed to be compatible with Passlib for Python and Argon2 PHP. Argon2 was the winner of the most r...
- 1333t: translation util for go, using GNU gettext
https://github.com/youthlin/t
t: translation util for go, using GNU gettext. Contribute to youthlin/t development by creating an account on GitHub.
- 1334A Go slugify application that handles string
https://github.com/avelino/slugify
A Go slugify application that handles string. Contribute to avelino/slugify development by creating an account on GitHub.
- 1335A Comprehensive Coverage Testing System for The Go Programming Language
https://github.com/qiniu/goc
A Comprehensive Coverage Testing System for The Go Programming Language - qiniu/goc
- 1336Storage and image processing server written in Go
https://github.com/aldor007/mort
Storage and image processing server written in Go. Contribute to aldor007/mort development by creating an account on GitHub.
- 1337Morse Code Library in Go
https://github.com/alwindoss/morse
Morse Code Library in Go. Contribute to alwindoss/morse development by creating an account on GitHub.
- 1338Gomol is a library for structured, multiple-output logging for Go with extensible logging outputs
https://github.com/aphistic/gomol
Gomol is a library for structured, multiple-output logging for Go with extensible logging outputs - aphistic/gomol
- 1339An enjoyable golang test framework.
https://github.com/ysmood/got
An enjoyable golang test framework. Contribute to ysmood/got development by creating an account on GitHub.
- 1340Simple and colorful test tools
https://github.com/vcaesar/tt
Simple and colorful test tools. Contribute to vcaesar/tt development by creating an account on GitHub.
- 1341A golang generic graph library that provides mathematical graph-theory and algorithms.
https://github.com/hmdsefi/gograph
A golang generic graph library that provides mathematical graph-theory and algorithms. - hmdsefi/gograph
- 1342Go (golang) http calls with retries and backoff
https://github.com/sethgrid/pester
Go (golang) http calls with retries and backoff . Contribute to sethgrid/pester development by creating an account on GitHub.
- 1343Convert Golang Struct To GraphQL Object On The Fly
https://github.com/SonicRoshan/straf
Convert Golang Struct To GraphQL Object On The Fly - ThundR67/straf
- 1344A simple dependency manager for Go (golang), inspired by Bundler.
https://github.com/nitrous-io/goop
A simple dependency manager for Go (golang), inspired by Bundler. - petejkim/goop
- 1345teler-waf is a Go HTTP middleware that protects local web services from OWASP Top 10 threats, known vulnerabilities, malicious actors, botnets, unwanted crawlers, and brute force attacks.
https://github.com/kitabisa/teler-waf
teler-waf is a Go HTTP middleware that protects local web services from OWASP Top 10 threats, known vulnerabilities, malicious actors, botnets, unwanted crawlers, and brute force attacks. - teler-s...
- 1346A Go package for n-gram based text categorization, with support for utf-8 and raw text
https://github.com/pebbe/textcat
A Go package for n-gram based text categorization, with support for utf-8 and raw text - pebbe/textcat
- 1347Simple Email Parser
https://github.com/sanbornm/mp
Simple Email Parser. Contribute to sanbornm/mp development by creating an account on GitHub.
- 1348vader sentiment analysis in go
https://github.com/jonreiter/govader
vader sentiment analysis in go. Contribute to jonreiter/govader development by creating an account on GitHub.
- 1349Compile-time Dependency Injection for Go
https://github.com/google/wire
Compile-time Dependency Injection for Go. Contribute to google/wire development by creating an account on GitHub.
- 1350Package arp implements the ARP protocol, as described in RFC 826. MIT Licensed.
https://github.com/mdlayher/arp
Package arp implements the ARP protocol, as described in RFC 826. MIT Licensed. - mdlayher/arp
- 1351A Go package for handling common HTTP JSON responses.
https://github.com/nicklaw5/go-respond
A Go package for handling common HTTP JSON responses. - nicklaw5/go-respond
- 1352Package httpretty prints the HTTP requests you make with Go pretty on your terminal.
https://github.com/henvic/httpretty
Package httpretty prints the HTTP requests you make with Go pretty on your terminal. - henvic/httpretty
- 1353:package: Send network packets over a TCP or UDP connection.
https://github.com/aerogo/packet
:package: Send network packets over a TCP or UDP connection. - aerogo/packet
- 1354Pure-Go library for cross-platform local peer discovery using UDP multicast :repeat: :woman:
https://github.com/schollz/peerdiscovery
Pure-Go library for cross-platform local peer discovery using UDP multicast :woman: :repeat: :woman: - GitHub - schollz/peerdiscovery: Pure-Go library for cross-platform local peer discovery using ...
- 1355📅 Calendar heatmap inspired by GitHub contribution activity
https://github.com/nikolaydubina/calendarheatmap
📅 Calendar heatmap inspired by GitHub contribution activity - GitHub - nikolaydubina/calendarheatmap: 📅 Calendar heatmap inspired by GitHub contribution activity
- 1356Building event-driven applications the easy way in Go.
https://github.com/ThreeDotsLabs/watermill
Building event-driven applications the easy way in Go. - ThreeDotsLabs/watermill
- 1357Random fake data and struct generator for Go.
https://github.com/pioz/faker
Random fake data and struct generator for Go. Contribute to pioz/faker development by creating an account on GitHub.
- 1358Provide basic charts in go
https://github.com/vdobler/chart
Provide basic charts in go. Contribute to vdobler/chart development by creating an account on GitHub.
- 1359Emojis for Go 😄🐢🚀
https://github.com/hackebrot/turtle
Emojis for Go 😄🐢🚀. Contribute to hackebrot/turtle development by creating an account on GitHub.
- 1360Automatically exported from code.google.com/p/go-gt
https://github.com/ThePaw/go-gt
Automatically exported from code.google.com/p/go-gt - ThePaw/go-gt
- 1361Provides packet processing capabilities for Go
https://github.com/google/gopacket
Provides packet processing capabilities for Go. Contribute to google/gopacket development by creating an account on GitHub.
- 1362Simple I/O event notification library wirtten in Golang
https://github.com/cheng-zhongliang/event
Simple I/O event notification library wirtten in Golang - cheng-zhongliang/event
- 1363tcpack is an application protocol based on TCP to Pack and Unpack bytes stream in go program.
https://github.com/lim-yoona/tcpack
tcpack is an application protocol based on TCP to Pack and Unpack bytes stream in go program. - lim-yoona/tcpack
- 1364Retryable HTTP client in Go
https://github.com/hashicorp/go-retryablehttp
Retryable HTTP client in Go. Contribute to hashicorp/go-retryablehttp development by creating an account on GitHub.
- 1365Package for comparing Go values in tests
https://github.com/google/go-cmp
Package for comparing Go values in tests. Contribute to google/go-cmp development by creating an account on GitHub.
- 1366The Go test helper for minimalists
https://github.com/carlmjohnson/be
The Go test helper for minimalists. Contribute to earthboundkid/be development by creating an account on GitHub.
- 1367Flagr is a feature flagging, A/B testing and dynamic configuration microservice
https://github.com/checkr/flagr
Flagr is a feature flagging, A/B testing and dynamic configuration microservice - openflagr/flagr
- 1368Enforce git message commit consistency
https://github.com/antham/gommit
Enforce git message commit consistency. Contribute to antham/gommit development by creating an account on GitHub.
- 1369HTTP service to generate PDF from Json requests
https://github.com/hyperboloide/pdfgen
HTTP service to generate PDF from Json requests. Contribute to hyperboloide/pdfgen development by creating an account on GitHub.
- 1370Converts 'go mod graph' output into Graphviz's DOT language
https://github.com/lucasepe/modgv
Converts 'go mod graph' output into Graphviz's DOT language - lucasepe/modgv
- 1371happy/pkg/strings/bexp at main · happy-sdk/happy
https://github.com/happy-sdk/happy/tree/main/pkg/strings/bexp
Accelerate your development with Happy SDK written in Go. Rapidly prototype and bring your ideas to life with its comprehensive resources and modular design, even with limited technical knowledge. ...
- 1372Fast JSON parser and validator for Go. No custom structs, no code generation, no reflection
https://github.com/valyala/fastjson
Fast JSON parser and validator for Go. No custom structs, no code generation, no reflection - valyala/fastjson
- 1373A layer of abstraction the around acme/autocert certificate manager (Golang)
https://github.com/adrianosela/sslmgr
A layer of abstraction the around acme/autocert certificate manager (Golang) - adrianosela/sslmgr
- 1374Natural language detection package in pure Go
https://github.com/rylans/getlang
Natural language detection package in pure Go. Contribute to rylans/getlang development by creating an account on GitHub.
- 1375Exponentially Weighted Moving Average algorithms for Go.
https://github.com/VividCortex/ewma
Exponentially Weighted Moving Average algorithms for Go. - VividCortex/ewma
- 1376Implements a deep pretty printer for Go data structures to aid in debugging
https://github.com/davecgh/go-spew
Implements a deep pretty printer for Go data structures to aid in debugging - davecgh/go-spew
- 1377Easy SSH servers in Golang
https://github.com/gliderlabs/ssh
Easy SSH servers in Golang. Contribute to gliderlabs/ssh development by creating an account on GitHub.
- 1378Type-safe Prometheus metrics builder library for golang [managed by soy-programador]
https://github.com/cabify/gotoprom
Type-safe Prometheus metrics builder library for golang [managed by soy-programador] - cabify/gotoprom
- 1379graphql parser + utilities
https://github.com/tmc/graphql
graphql parser + utilities. Contribute to tmc/graphql development by creating an account on GitHub.
- 1380Jet template engine
https://github.com/CloudyKit/jet
Jet template engine. Contribute to CloudyKit/jet development by creating an account on GitHub.
- 1381An enhanced HTTP client for Go
https://github.com/gojektech/heimdall
An enhanced HTTP client for Go. Contribute to gojek/heimdall development by creating an account on GitHub.
- 1382Ensembles of decision trees in go/golang.
https://github.com/ryanbressler/CloudForest
Ensembles of decision trees in go/golang. Contribute to ryanbressler/CloudForest development by creating an account on GitHub.
- 1383Spelling corrector for Spanish language
https://github.com/jorelosorio/spellingcorrector
Spelling corrector for Spanish language . Contribute to jorelosorio/spellingcorrector development by creating an account on GitHub.
- 1384🪡 Dead simple, lightweight tracing.
https://github.com/kamilsk/tracer
🪡 Dead simple, lightweight tracing. Contribute to kamilsk/tracer development by creating an account on GitHub.
- 1385Stream database events from PostgreSQL to Kafka
https://github.com/blind-oracle/psql-streamer
Stream database events from PostgreSQL to Kafka. Contribute to blind-oracle/psql-streamer development by creating an account on GitHub.
- 1386A simple file embedder for Go
https://github.com/mjibson/esc
A simple file embedder for Go. Contribute to maddyblue/esc development by creating an account on GitHub.
- 1387Go bindings for the snowball libstemmer library including porter 2
https://github.com/rjohnsondev/golibstemmer
Go bindings for the snowball libstemmer library including porter 2 - rjohnsondev/golibstemmer
- 1388Golang Dynamic Decision Tree
https://github.com/sgrodriguez/ddt
Golang Dynamic Decision Tree. Contribute to sgrodriguez/ddt development by creating an account on GitHub.
- 1389A lightweight yet powerful IoC dependency injection container for the Go programming language
https://github.com/golobby/container
A lightweight yet powerful IoC dependency injection container for the Go programming language - golobby/container
- 1390Translate your Go program into multiple languages.
https://github.com/nicksnyder/go-i18n/
Translate your Go program into multiple languages. - nicksnyder/go-i18n
- 1391Tensorflow + Go, the gopher way
https://github.com/galeone/tfgo
Tensorflow + Go, the gopher way. Contribute to galeone/tfgo development by creating an account on GitHub.
- 1392Go Dependency Injection Framework
https://github.com/i-love-flamingo/dingo
Go Dependency Injection Framework. Contribute to i-love-flamingo/dingo development by creating an account on GitHub.
- 1393Pretty Slug.
https://github.com/mozillazg/go-slugify
Pretty Slug. Contribute to mozillazg/go-slugify development by creating an account on GitHub.
- 1394A Go package that provides a simple way of accessing nested properties in maps and slices.
https://github.com/simonnilsson/ask
A Go package that provides a simple way of accessing nested properties in maps and slices. - simonnilsson/ask
- 1395tcp server pool
https://github.com/two/tspool
tcp server pool. Contribute to two/tspool development by creating an account on GitHub.
- 1396Easily create & extract archives, and compress & decompress files of various formats
https://github.com/mholt/archiver
Easily create & extract archives, and compress & decompress files of various formats - mholt/archiver
- 1397🚀 gnet is a high-performance, lightweight, non-blocking, event-driven networking framework written in pure Go.
https://github.com/panjf2000/gnet
🚀 gnet is a high-performance, lightweight, non-blocking, event-driven networking framework written in pure Go. - panjf2000/gnet
- 1398sunwxg/goshark
https://github.com/sunwxg/goshark
Contribute to sunwxg/goshark development by creating an account on GitHub.
- 1399Open Source HTTP Reverse Proxy Cache and Time Series Dashboard Accelerator
https://github.com/tricksterproxy/trickster
Open Source HTTP Reverse Proxy Cache and Time Series Dashboard Accelerator - trickstercache/trickster
- 1400A TCP Server Framework with graceful shutdown, custom protocol.
https://github.com/xfxdev/xtcp
A TCP Server Framework with graceful shutdown, custom protocol. - xfxdev/xtcp
- 1401Tool for show test coverage in terminal for Go source files
https://github.com/msoap/go-carpet
Tool for show test coverage in terminal for Go source files - msoap/go-carpet
- 1402Article spinning and spintax/spinning syntax engine written in Go, useful for A/B, testing pieces of text/articles and creating more natural conversations
https://github.com/m1/gospin
Article spinning and spintax/spinning syntax engine written in Go, useful for A/B, testing pieces of text/articles and creating more natural conversations - m1/gospin
- 1403Golang binary decoder for mapping data into the structure
https://github.com/ghostiam/binstruct
Golang binary decoder for mapping data into the structure - ghostiam/binstruct
- 1404Golang HTTP client testing framework
https://github.com/suzuki-shunsuke/flute
Golang HTTP client testing framework. Contribute to suzuki-shunsuke/flute development by creating an account on GitHub.
- 1405Model Query Language (mql) is a query language for your database models.
https://github.com/hashicorp/mql
Model Query Language (mql) is a query language for your database models. - hashicorp/mql
- 1406Datastore Testibility
https://github.com/viant/dsunit
Datastore Testibility. Contribute to viant/dsunit development by creating an account on GitHub.
- 1407Prisma Client Go is an auto-generated and fully type-safe database client
https://github.com/prisma/prisma-client-go
Prisma Client Go is an auto-generated and fully type-safe database client - steebchen/prisma-client-go
- 1408Genetic Algorithm and Particle Swarm Optimization
https://github.com/khezen/evoli
Genetic Algorithm and Particle Swarm Optimization. Contribute to khezen/evoli development by creating an account on GitHub.
- 1409Go application GitHub repository template.
https://github.com/golang-templates/seed
Go application GitHub repository template. Contribute to golang-templates/seed development by creating an account on GitHub.
- 1410An extensive Philips Hue client library for Go with an emphasis on simplicity
https://github.com/amimof/huego
An extensive Philips Hue client library for Go with an emphasis on simplicity - amimof/huego
- 1411generators/varhandler at master · azr/generators
https://github.com/azr/generators/tree/master/varhandler
#golang generator. Contribute to azr/generators development by creating an account on GitHub.
- 1412URL-friendly slugify with multiple languages support.
https://github.com/gosimple/slug
URL-friendly slugify with multiple languages support. - gosimple/slug
- 1413Generate TypeScript interfaces from Go structs/interfaces - useful for JSON RPC
https://github.com/32leaves/bel
Generate TypeScript interfaces from Go structs/interfaces - useful for JSON RPC - csweichel/bel
- 1414A generic oplog/replication system for microservices
https://github.com/dailymotion/oplog
A generic oplog/replication system for microservices - dailymotion/oplog
- 1415Pure go library for creating and processing Office Word (.docx), Excel (.xlsx) and Powerpoint (.pptx) documents
https://github.com/unidoc/unioffice
Pure go library for creating and processing Office Word (.docx), Excel (.xlsx) and Powerpoint (.pptx) documents - unidoc/unioffice
- 1416Package tasks is an easy to use in-process scheduler for recurring tasks in Go
https://github.com/madflojo/tasks
Package tasks is an easy to use in-process scheduler for recurring tasks in Go - madflojo/tasks
- 1417go-lctree provides a CLI and Go primitives to serialize and deserialize LeetCode binary trees (e.g. "[5,4,7,3,null,2,null,-1,null,9]").
https://github.com/sbourlon/go-lctree
go-lctree provides a CLI and Go primitives to serialize and deserialize LeetCode binary trees (e.g. "[5,4,7,3,null,2,null,-1,null,9]"). - sbourlon/go-lctree
- 1418Takes an input http.FileSystem (likely at go generate time) and generates Go code that statically implements it.
https://github.com/shurcooL/vfsgen
Takes an input http.FileSystem (likely at go generate time) and generates Go code that statically implements it. - shurcooL/vfsgen
- 1419go websocket client for unit testing of a websocket handler
https://github.com/posener/wstest
go websocket client for unit testing of a websocket handler - posener/wstest
- 1420Home
https://github.com/ardanlabs/service/wiki
Starter-kit for writing services in Go using Kubernetes. - ardanlabs/service
- 1421Colorized JSON output for Go
https://github.com/neilotoole/jsoncolor
Colorized JSON output for Go. Contribute to neilotoole/jsoncolor development by creating an account on GitHub.
- 1422🐇 CLI tool for websockets and Go package
https://github.com/leozz37/hare
🐇 CLI tool for websockets and Go package. Contribute to leozz37/hare development by creating an account on GitHub.
- 1423An experimental go FTP server framework
https://github.com/koofr/graval
An experimental go FTP server framework. Contribute to koofr/graval development by creating an account on GitHub.
- 1424atomic measures + Prometheus exposition library
https://github.com/pascaldekloe/metrics
atomic measures + Prometheus exposition library. Contribute to pascaldekloe/metrics development by creating an account on GitHub.
- 1425auto-generate capnproto schema from your golang source files. Depends on go-capnproto-1.0 at https://github.com/glycerine/go-capnproto
https://github.com/glycerine/bambam
auto-generate capnproto schema from your golang source files. Depends on go-capnproto-1.0 at https://github.com/glycerine/go-capnproto - glycerine/bambam
- 1426A words table and text resource library for Golang projects.
https://github.com/saleh-rahimzadeh/go-words
A words table and text resource library for Golang projects. - saleh-rahimzadeh/go-words
- 1427Go Library to Execute Commands Over SSH at Scale
https://github.com/yahoo/vssh
Go Library to Execute Commands Over SSH at Scale. Contribute to yahoo/vssh development by creating an account on GitHub.
- 1428API testing framework inspired by frisby-js
https://github.com/verdverm/frisby
API testing framework inspired by frisby-js. Contribute to hofstadter-io/frisby development by creating an account on GitHub.
- 1429HTTP requests for Gophers
https://github.com/carlmjohnson/requests
HTTP requests for Gophers. Contribute to earthboundkid/requests development by creating an account on GitHub.
- 1430IP Geolocation API | IP2Location.io
https://www.ip2location.io/.
An IP Geolocation API tool that provides geolocation information like country, city, ISP, proxy and so on in JSON and XML formats by using users' IP addresses.
- 1431A Go library to parse and format vCard
https://github.com/emersion/go-vcard
A Go library to parse and format vCard. Contribute to emersion/go-vcard development by creating an account on GitHub.
- 1432Labeled Tab-separated Values (LTSV)
http://ltsv.org/
Labeled Tab-separated Values (LTSV) format is a variant of Tab-separated Values (TSV). Each record in a LTSV file is represented as a single line. Each field is separated by TAB and has a label and a value. The label and the value have been separated by ':'. With the LTSV format, you can parse each line by spliting with TAB (like original TSV format) easily, and extend any fields with unique labels in no particular order.
- 1433TOML parser for Golang with reflection.
https://github.com/BurntSushi/toml
TOML parser for Golang with reflection. Contribute to BurntSushi/toml development by creating an account on GitHub.
- 1434⏳ Provide filtering, sanitizing, and conversion of Golang data. 提供对Golang数据的过滤,净化,转换。
https://github.com/gookit/filter
⏳ Provide filtering, sanitizing, and conversion of Golang data. 提供对Golang数据的过滤,净化,转换。 - gookit/filter
- 1435wcwidth for golang
https://github.com/mattn/go-runewidth
wcwidth for golang. Contribute to mattn/go-runewidth development by creating an account on GitHub.
- 1436Daniil Literate / genmock · GitLab
https://gitlab.com/so_literate/genmock
Golang mock system with code generator
- 1437Go library for interacting with CircleCI
https://github.com/jszwedko/go-circleci
Go library for interacting with CircleCI. Contribute to jszwedko/go-circleci development by creating an account on GitHub.
- 1438:fishing_pole_and_fish: Webhook receiver for GitHub, Bitbucket, GitLab, Gogs
https://github.com/go-playground/webhooks
:fishing_pole_and_fish: Webhook receiver for GitHub, Bitbucket, GitLab, Gogs - go-playground/webhooks
- 1439GoMock is a mocking framework for the Go programming language.
https://github.com/golang/mock
GoMock is a mocking framework for the Go programming language. - golang/mock
- 1440ok, run your gofile
https://github.com/xta/okrun
ok, run your gofile. Contribute to xta/okrun development by creating an account on GitHub.
- 1441Tokenizer (lexer) for golang
https://github.com/bzick/tokenizer
Tokenizer (lexer) for golang. Contribute to bzick/tokenizer development by creating an account on GitHub.
- 1442Go(lang) client library for Cachet (open source status page system).
https://github.com/andygrunwald/cachet
Go(lang) client library for Cachet (open source status page system). - andygrunwald/cachet
- 1443Parse TODOs in your GO code
https://github.com/asticode/go-astitodo
Parse TODOs in your GO code. Contribute to asticode/go-astitodo development by creating an account on GitHub.
- 1444htmlquery is golang XPath package for HTML query.
https://github.com/antchfx/htmlquery
htmlquery is golang XPath package for HTML query. Contribute to antchfx/htmlquery development by creating an account on GitHub.
- 1445An implementation of failpoints for Golang.
https://github.com/pingcap/failpoint
An implementation of failpoints for Golang. Contribute to pingcap/failpoint development by creating an account on GitHub.
- 1446A Chrome DevTools Protocol driver for web automation and scraping.
https://github.com/go-rod/rod
A Chrome DevTools Protocol driver for web automation and scraping. - go-rod/rod
- 1447#1 Password Manager & Vault App with Single-Sign On & MFA Solutions - LastPass
https://www.lastpass.com/
Go beyond saving passwords with the best password manager! Generate strong passwords and store them in a secure vault. Now with single-sign on (SSO) and adaptive MFA solutions that integrate with over 1,200 apps.
- 1448The Best API Documentation Tool
https://redocly.com/.
OpenAPI-generated documentation tool with 17,000+ stars on Github - for API docs you can be proud of.
- 1449Apache Mesos
https://mesos.apache.org/
Apache Mesos abstracts resources away from machines, enabling fault-tolerant and elastic distributed systems to easily be built and run effectively.
- 1450bluemonday: a fast golang HTML sanitizer (inspired by the OWASP Java HTML Sanitizer) to scrub user generated content of XSS
https://github.com/microcosm-cc/bluemonday
bluemonday: a fast golang HTML sanitizer (inspired by the OWASP Java HTML Sanitizer) to scrub user generated content of XSS - microcosm-cc/bluemonday
- 1451Common case conversions covering common initialisms.
https://github.com/codemodus/kace
Common case conversions covering common initialisms. - codemodus/kace
- 1452EditorConfig Core written in Go
https://github.com/editorconfig/editorconfig-core-go
EditorConfig Core written in Go. Contribute to editorconfig/editorconfig-core-go development by creating an account on GitHub.
- 1453A NMEA parser library in pure Go
https://github.com/adrianmo/go-nmea
A NMEA parser library in pure Go. Contribute to adrianmo/go-nmea development by creating an account on GitHub.
- 1454Parse placeholder and wildcard text commands
https://github.com/sbstjn/allot
Parse placeholder and wildcard text commands. Contribute to sbstjn/allot development by creating an account on GitHub.
- 1455Simple | Fast tool to manage MinIO clusters :cloud:
https://github.com/minio/mc
Simple | Fast tool to manage MinIO clusters :cloud: - minio/mc
- 1456Parse and check doi objects in go.
https://github.com/hscells/doi
Parse and check doi objects in go. Contribute to hscells/doi development by creating an account on GitHub.
- 1457A mock code autogenerator for Go
https://github.com/vektra/mockery
A mock code autogenerator for Go. Contribute to vektra/mockery development by creating an account on GitHub.
- 1458Port of Python's "textwrap" module to Go
https://github.com/isbm/textwrap
Port of Python's "textwrap" module to Go. Contribute to isbm/textwrap development by creating an account on GitHub.
- 1459JokeAPI
https://sv443.net/jokeapi/v2/.
JokeAPI is a RESTful API that serves jokes from many categories while also offering a lot of filtering methods
- 1460Minimalistic REST client for Go applications
https://github.com/zpatrick/rclient
Minimalistic REST client for Go applications. Contribute to zpatrick/rclient development by creating an account on GitHub.
- 1461gommon/bytes at master · labstack/gommon
https://github.com/labstack/gommon/tree/master/bytes
Common packages for Go. Contribute to labstack/gommon development by creating an account on GitHub.
- 1462Golang SDK for Dusupay payment gateway API (Unofficial)
https://github.com/Kachit/dusupay-sdk-go
Golang SDK for Dusupay payment gateway API (Unofficial) - Kachit/dusupay-sdk-go
- 1463A natural language date/time parser with pluggable rules
https://github.com/olebedev/when
A natural language date/time parser with pluggable rules - olebedev/when
- 1464🍫 A collection of common regular expressions for Go
https://github.com/mingrammer/commonregex
🍫 A collection of common regular expressions for Go - mingrammer/commonregex
- 1465Simple and expressive toolbox written in Go
https://github.com/alxrm/ugo
Simple and expressive toolbox written in Go. Contribute to alxrm/ugo development by creating an account on GitHub.
- 1466Golang port of Petrovich - an inflector for Russian anthroponyms.
https://github.com/striker2000/petrovich
Golang port of Petrovich - an inflector for Russian anthroponyms. - striker2000/petrovich
- 1467Lightweight service virtualization/ API simulation / API mocking tool for developers and testers
https://github.com/SpectoLabs/hoverfly
Lightweight service virtualization/ API simulation / API mocking tool for developers and testers - SpectoLabs/hoverfly
- 1468:100: JSend's implementation writen in Go(golang)
https://github.com/clevergo/jsend
:100: JSend's implementation writen in Go(golang). Contribute to clevergo/jsend development by creating an account on GitHub.
- 1469DeviceCheck | Apple Developer Documentation
https://developer.apple.com/documentation/devicecheck
Reduce fraudulent use of your services by managing device state and asserting app integrity.
- 1470A pretty simple library to ensure your work to be done
https://github.com/shafreeck/retry
A pretty simple library to ensure your work to be done - shafreeck/retry
- 1471A terminal application to watch crypto prices!
https://github.com/Gituser143/cryptgo
A terminal application to watch crypto prices! Contribute to Gituser143/cryptgo development by creating an account on GitHub.
- 1472GitLab.org / cli · GitLab
https://gitlab.com/gitlab-org/cli
A GitLab CLI tool bringing GitLab to your command line
- 1473Maxim Lebedev / Telegraph · GitLab
https://gitlab.com/toby3d/telegraph
📚 Official unofficial Golang bindings for Telegraph API https://source.toby3d.me/toby3d/telegraph
- 1474Imgur
https://imgur.com
Imgur: The magic of the Internet
- 1475avito-tech/normalize
https://github.com/avito-tech/normalize
Contribute to avito-tech/normalize development by creating an account on GitHub.
- 1476Takes a full name and splits it into individual name parts
https://github.com/polera/gonameparts
Takes a full name and splits it into individual name parts - polera/gonameparts
- 1477Immutable transaction isolated sql driver for golang
https://github.com/DATA-DOG/go-txdb
Immutable transaction isolated sql driver for golang - DATA-DOG/go-txdb
- 1478Interface mocking tool for go generate
https://github.com/matryer/moq
Interface mocking tool for go generate. Contribute to matryer/moq development by creating an account on GitHub.
- 1479OpenCage Geocoding API Documentation
https://opencagedata.com/api
Full documentation and reference for the OpenCage Geocoding API for forward and reverse geocoding: formats, parameters, response codes, best practices, etc.
- 1480utils for Go context
https://github.com/posener/ctxutil
utils for Go context. Contribute to posener/ctxutil development by creating an account on GitHub.
- 1481:moon_cake: A simple way to generate mocks for multiple purposes
https://github.com/GuilhermeCaruso/mooncake
:moon_cake: A simple way to generate mocks for multiple purposes - GuilhermeCaruso/mooncake
- 1482SDP: Session Description Protocol
https://tools.ietf.org/html/rfc4566
This memo defines the Session Description Protocol (SDP). SDP is intended for describing multimedia sessions for the purposes of session announcement, session invitation, and other forms of multimedia session initiation. [STANDARDS-TRACK]
- 1483The Biggest Video Game Database on RAWG - Video Game Discovery Service
https://rawg.io/
RAWG.IO ♛ Keep all games in one profile ✔ See what friends are playing, and find your next great game.
- 1484Go implementation of OpenSKY Network API
https://github.com/navidys/gopensky
Go implementation of OpenSKY Network API. Contribute to navidys/gopensky development by creating an account on GitHub.
- 1485Powerful mock generation tool for Go programming language
https://github.com/gojuno/minimock
Powerful mock generation tool for Go programming language - gojuno/minimock
- 1486Address handling for Go.
https://github.com/bojanz/address
Address handling for Go. Contribute to bojanz/address development by creating an account on GitHub.
- 1487Rob Muhlestein / Unique Identifiers · GitLab
https://gitlab.com/skilstak/code/go/uniq
No-hassle unique identifiers.
- 1488Go library for accessing the Codeship API v2
https://github.com/codeship/codeship-go
Go library for accessing the Codeship API v2. Contribute to codeship/codeship-go development by creating an account on GitHub.
- 1489Mock object for Go http.ResponseWriter
https://github.com/tv42/mockhttp
Mock object for Go http.ResponseWriter. Contribute to tv42/mockhttp development by creating an account on GitHub.
- 1490Seamlessly fetch paginated data from any source. Simple and high performance API scraping included!
https://github.com/cyucelen/walker
Seamlessly fetch paginated data from any source. Simple and high performance API scraping included! - cyucelen/walker
- 1491All you need when you are working with countries in Go.
https://github.com/pioz/countries
All you need when you are working with countries in Go. - pioz/countries
- 1492Tools for working with trees of html.Nodes.
https://github.com/bobg/htree
Tools for working with trees of html.Nodes. Contribute to bobg/htree development by creating an account on GitHub.
- 1493:dancers: Go Chronos 3.x REST API Client
https://github.com/axelspringer/go-chronos
:dancers: Go Chronos 3.x REST API Client. Contribute to axelspringer/go-chronos development by creating an account on GitHub.
- 1494Delve is a debugger for the Go programming language.
https://github.com/derekparker/delve
Delve is a debugger for the Go programming language. - derekparker/delve
- 1495Go Wrapper for using localstack
https://github.com/elgohr/go-localstack
Go Wrapper for using localstack. Contribute to elgohr/go-localstack development by creating an account on GitHub.
- 1496A test-friendly replacement for golang's time package [managed by soy-programador]
https://github.com/cabify/timex
A test-friendly replacement for golang's time package [managed by soy-programador] - cabify/timex
- 1497Converter from BBCode to HTML
https://github.com/CalebQ42/bbConvert
Converter from BBCode to HTML. Contribute to CalebQ42/bbConvert development by creating an account on GitHub.
- 1498GO - Asterisk AMI Interface
https://github.com/bit4bit/gami
GO - Asterisk AMI Interface. Contribute to bit4bit/gami development by creating an account on GitHub.
- 1499hierarchical timing wheel
https://github.com/andy2046/tik
hierarchical timing wheel. Contribute to andy2046/tik development by creating an account on GitHub.
- 1500Zero-width character detection and removal for Go
https://github.com/trubitsyn/go-zero-width
Zero-width character detection and removal for Go. Contribute to trubitsyn/go-zero-width development by creating an account on GitHub.
- 1501Managing go application shutdown with signals.
https://github.com/vrecan/death
Managing go application shutdown with signals. Contribute to vrecan/death development by creating an account on GitHub.
- 1502:evergreen_tree: Parses indented code and returns a tree structure.
https://github.com/aerogo/codetree
:evergreen_tree: Parses indented code and returns a tree structure. - aerogo/codetree
- 1503Universal JSON, BSON, YAML, CSV, XML, mt940 converter with templates
https://github.com/mmalcek/bafi
Universal JSON, BSON, YAML, CSV, XML, mt940 converter with templates - mmalcek/bafi
- 1504Create a QR code with your Wi-Fi login details
https://github.com/reugn/wifiqr
Create a QR code with your Wi-Fi login details. Contribute to reugn/wifiqr development by creating an account on GitHub.
- 1505NFDump File Reader
https://github.com/chrispassas/nfdump
NFDump File Reader. Contribute to chrispassas/nfdump development by creating an account on GitHub.
- 1506Manipulate subtitles in GO (.srt, .ssa/.ass, .stl, .ttml, .vtt (webvtt), teletext, etc.)
https://github.com/asticode/go-astisub
Manipulate subtitles in GO (.srt, .ssa/.ass, .stl, .ttml, .vtt (webvtt), teletext, etc.) - asticode/go-astisub
- 1507Go library for querying Source servers
https://github.com/sostronk/go-steam
Go library for querying Source servers. Contribute to sostronk/go-steam development by creating an account on GitHub.
- 1508Go package provides a generic interface to encoders and decoders
https://github.com/mickep76/encdec
Go package provides a generic interface to encoders and decoders - ake-persson/encoding
- 1509Struct validation using tags
https://github.com/twharmon/govalid
Struct validation using tags. Contribute to twharmon/govalid development by creating an account on GitHub.
- 1510Xferspdy provides binary diff and patch library in golang. [Mentioned in Awesome Go, https://github.com/avelino/awesome-go]
https://github.com/monmohan/xferspdy
Xferspdy provides binary diff and patch library in golang. [Mentioned in Awesome Go, https://github.com/avelino/awesome-go] - monmohan/xferspdy
- 1511Slice conversion between primitive types
https://github.com/Henry-Sarabia/sliceconv
Slice conversion between primitive types. Contribute to Henry-Sarabia/sliceconv development by creating an account on GitHub.
- 1512APM is a process manager for Golang applications.
https://github.com/topfreegames/apm
APM is a process manager for Golang applications. Contribute to topfreegames/apm development by creating an account on GitHub.
- 1513Easy and simple CLI time tracker for your tasks
https://github.com/mlimaloureiro/golog
Easy and simple CLI time tracker for your tasks. Contribute to mlimaloureiro/golog development by creating an account on GitHub.
- 1514A complete Golang client for SeaweedFS
https://github.com/linxGnu/goseaweedfs
A complete Golang client for SeaweedFS. Contribute to linxGnu/goseaweedfs development by creating an account on GitHub.
- 1515Official golang wrapper for Sv443's jokeapi.
https://github.com/icelain/jokeapi
Official golang wrapper for Sv443's jokeapi. Contribute to Icelain/jokeapi development by creating an account on GitHub.
- 1516A Global event triggerer for golang. Defines functions as event with id string. Trigger the event anywhere from your project.
https://github.com/sadlil/go-trigger
A Global event triggerer for golang. Defines functions as event with id string. Trigger the event anywhere from your project. - sadlil/go-trigger
- 1517All-in-one go testing library
https://github.com/xhd2015/xgo
All-in-one go testing library. Contribute to xhd2015/xgo development by creating an account on GitHub.
- 1518Parses the Graphviz DOT language in golang
https://github.com/awalterschulze/gographviz
Parses the Graphviz DOT language in golang. Contribute to awalterschulze/gographviz development by creating an account on GitHub.
- 1519⚙️ Convert HTML to Markdown. Even works with entire websites and can be extended through rules.
https://github.com/JohannesKaufmann/html-to-markdown
⚙️ Convert HTML to Markdown. Even works with entire websites and can be extended through rules. - JohannesKaufmann/html-to-markdown
- 1520Fast, dependable universally unique ids
https://github.com/twharmon/gouid
Fast, dependable universally unique ids. Contribute to twharmon/gouid development by creating an account on GitHub.
- 1521SmiteGo is an API wrapper for the Smite game from HiRez. It is written in Go!
https://github.com/sergiotapia/smitego
SmiteGo is an API wrapper for the Smite game from HiRez. It is written in Go! - sergiotapia/smitego
- 1522Fuzz testing for go.
https://github.com/google/gofuzz
Fuzz testing for go. Contribute to google/gofuzz development by creating an account on GitHub.
- 1523Match regex group into go struct using struct tags and automatic parsing
https://github.com/oriser/regroup
Match regex group into go struct using struct tags and automatic parsing - oriser/regroup
- 1524mikekonan/go-countries
https://github.com/mikekonan/go-countries
Contribute to mikekonan/go-countries development by creating an account on GitHub.
- 1525Package cdp provides type-safe bindings for the Chrome DevTools Protocol (CDP), written in the Go programming language.
https://github.com/mafredri/cdp
Package cdp provides type-safe bindings for the Chrome DevTools Protocol (CDP), written in the Go programming language. - mafredri/cdp
- 1526Fast implementation for uuid
https://github.com/rekby/fastuuid
Fast implementation for uuid. Contribute to rekby/fastuuid development by creating an account on GitHub.
- 1527xid is a globally unique id generator thought for the web
https://github.com/rs/xid
xid is a globally unique id generator thought for the web - rs/xid
- 1528manipulate and inspect VCS repositories in Go
https://github.com/sourcegraph/go-vcs
manipulate and inspect VCS repositories in Go. Contribute to sourcegraph/go-vcs development by creating an account on GitHub.
- 1529Validate Golang request data with simple rules. Highly inspired by Laravel's request validation.
https://github.com/thedevsaddam/govalidator
Validate Golang request data with simple rules. Highly inspired by Laravel's request validation. - thedevsaddam/govalidator
- 1530:runner:runs go generate recursively on a specified path or environment variable and can filter by regex
https://github.com/go-playground/generate
:runner:runs go generate recursively on a specified path or environment variable and can filter by regex - go-playground/generate
- 1531hvalid is a lightweight validation library written in Go language.(一个用Go语言编写的轻量级验证库)
https://github.com/lyonnee/hvalid
hvalid is a lightweight validation library written in Go language.(一个用Go语言编写的轻量级验证库) - lyonnee/hvalid
- 1532lodash throttle like Go library
https://github.com/yudppp/throttle
lodash throttle like Go library. Contribute to yudppp/throttle development by creating an account on GitHub.
- 1533Go library for the Spotify Web API
https://github.com/rapito/go-spotify
Go library for the Spotify Web API. Contribute to rapito/go-spotify development by creating an account on GitHub.
- 1534randexp for Go.
https://github.com/zach-klippenstein/goregen
randexp for Go. Contribute to zach-klippenstein/goregen development by creating an account on GitHub.
- 1535Go Client Library for Amazon Product Advertising API
https://github.com/ngs/go-amazon-product-advertising-api
Go Client Library for Amazon Product Advertising API - ngs/go-amazon-product-advertising-api
- 1536a fake clock for golang
https://github.com/jonboulle/clockwork
a fake clock for golang. Contribute to jonboulle/clockwork development by creating an account on GitHub.
- 1537A tiny and fast Go unique string generator
https://github.com/aidarkhanov/nanoid
A tiny and fast Go unique string generator. Contribute to aidarkhanov/nanoid development by creating an account on GitHub.
- 1538A general purpose application and library for aligning text.
https://github.com/Guitarbum722/align
A general purpose application and library for aligning text. - Guitarbum722/align
- 1539Locations API - Bing Maps
https://msdn.microsoft.com/en-us/library/ff701715.aspx
Learn how to use the Locations API to get location information for Bing Maps.
- 1540DRY (don't repeat yourself) package for Go
https://github.com/ungerik/go-dry
DRY (don't repeat yourself) package for Go. Contribute to ungerik/go-dry development by creating an account on GitHub.
- 1541A lightweight load balancer used to create big Selenium clusters
https://github.com/aerokube/ggr
A lightweight load balancer used to create big Selenium clusters - aerokube/ggr
- 1542go-querystring is Go library for encoding structs into URL query strings.
https://github.com/google/go-querystring
go-querystring is Go library for encoding structs into URL query strings. - google/go-querystring
- 1543Golang package which provides http Handlers to serve the swagger ui
https://github.com/esurdam/go-swagger-ui
Golang package which provides http Handlers to serve the swagger ui - esurdam/go-swagger-ui
- 1544fill struct data easily with fill tags
https://github.com/yaronsumel/filler
fill struct data easily with fill tags. Contribute to yaronsumel/filler development by creating an account on GitHub.
- 1545A Golang SDK for Medium's OAuth2 API
https://github.com/Medium/medium-sdk-go
A Golang SDK for Medium's OAuth2 API. Contribute to Medium/medium-sdk-go development by creating an account on GitHub.
- 1546Golang struct operations.
https://github.com/PumpkinSeed/structs
Golang struct operations. Contribute to PumpkinSeed/structs development by creating an account on GitHub.
- 1547GO Golang Utilities and helpers like validators and string formatters
https://github.com/miguelpragier/handy
GO Golang Utilities and helpers like validators and string formatters - miguelpragier/handy
- 1548Easily consume REST APIs with Go (golang)
https://github.com/bndr/gopencils
Easily consume REST APIs with Go (golang). Contribute to bndr/gopencils development by creating an account on GitHub.
- 1549Simple Reporting for Google Analytics
https://github.com/chonthu/go-google-analytics
Simple Reporting for Google Analytics. Contribute to chonthu/go-google-analytics development by creating an account on GitHub.
- 1550simple struct copying for golang
https://github.com/ulule/deepcopier
simple struct copying for golang. Contribute to ulule/deepcopier development by creating an account on GitHub.
- 1551Extract urls from text
https://github.com/mvdan/xurls
Extract urls from text. Contribute to mvdan/xurls development by creating an account on GitHub.
- 1552Go Client Library for Amazon's Product Advertising API 5.0
https://github.com/utekaravinash/gopaapi5
Go Client Library for Amazon's Product Advertising API 5.0 - utekaravinash/gopaapi5
- 1553🚀 Fast and light wildcard pattern matching.
https://github.com/IGLOU-EU/go-wildcard
🚀 Fast and light wildcard pattern matching. Contribute to IGLOU-EU/go-wildcard development by creating an account on GitHub.
- 1554a small golang lib to generate placeholder images
https://github.com/michiwend/goplaceholder
a small golang lib to generate placeholder images. Contribute to michiwend/goplaceholder development by creating an account on GitHub.
- 1555A fast string sorting algorithm (MSD radix sort)
https://github.com/yourbasic/radix
A fast string sorting algorithm (MSD radix sort). Contribute to yourbasic/radix development by creating an account on GitHub.
- 1556Go bindings for RRDA https://github.com/fcambus/rrda
https://github.com/Omie/rrdaclient
Go bindings for RRDA https://github.com/fcambus/rrda - Omie/rrdaclient
- 1557Simple Asterisk Manager Interface (AMI) library fo golang
https://github.com/staskobzar/goami2
Simple Asterisk Manager Interface (AMI) library fo golang - staskobzar/goami2
- 1558Changelog generator : use a git repository and various data sources and publish the result on external services
https://github.com/antham/chyle
Changelog generator : use a git repository and various data sources and publish the result on external services - antham/chyle
- 1559HTTP mocking for Golang
https://github.com/jarcoal/httpmock
HTTP mocking for Golang. Contribute to jarcoal/httpmock development by creating an account on GitHub.
- 1560A tool for generating self-contained, type-safe test doubles in go
https://github.com/maxbrunsfeld/counterfeiter
A tool for generating self-contained, type-safe test doubles in go - maxbrunsfeld/counterfeiter
- 1561Google Adwords API for Go
https://github.com/emiddleton/gads
Google Adwords API for Go. Contribute to emiddleton/gads development by creating an account on GitHub.
- 1562This project implements a Go client library for the Hipchat API.
https://github.com/andybons/hipchat
This project implements a Go client library for the Hipchat API. - andybons/hipchat
- 1563A sanitization-based swear filter for Go.
https://github.com/JoshuaDoes/gofuckyourself
A sanitization-based swear filter for Go. Contribute to JoshuaDoes/gofuckyourself development by creating an account on GitHub.
- 1564This package provides a framework for writing validations for Go applications.
https://github.com/gobuffalo/validate
This package provides a framework for writing validations for Go applications. - gobuffalo/validate
- 1565Instant Terminal Sharing
https://github.com/owenthereal/upterm
Instant Terminal Sharing. Contribute to owenthereal/upterm development by creating an account on GitHub.
- 1566A shell parser, formatter, and interpreter with bash support; includes shfmt
https://github.com/mvdan/sh
A shell parser, formatter, and interpreter with bash support; includes shfmt - mvdan/sh
- 1567One caching API, Multiple backends
https://github.com/adelowo/onecache
One caching API, Multiple backends. Contribute to adelowo/onecache development by creating an account on GitHub.
- 1568Provide check digit algorithms and calculators written in Go
https://github.com/osamingo/checkdigit
Provide check digit algorithms and calculators written in Go - osamingo/checkdigit
- 1569Load environment variables from `.env` or `io.Reader` in Go.
https://github.com/subosito/gotenv
Load environment variables from `.env` or `io.Reader` in Go. - subosito/gotenv
- 1570Golang client for LastPass
https://github.com/ansd/lastpass-go
Golang client for LastPass. Contribute to ansd/lastpass-go development by creating an account on GitHub.
- 1571:balloon: A lightweight struct validator for Go
https://github.com/guiferpa/gody
:balloon: A lightweight struct validator for Go. Contribute to guiferpa/gody development by creating an account on GitHub.
- 1572A scanner similar to bufio.Scanner, but it reads and returns lines in reverse order, starting at a given position and going backward.
https://github.com/icza/backscanner
A scanner similar to bufio.Scanner, but it reads and returns lines in reverse order, starting at a given position and going backward. - icza/backscanner
- 1573codemodus/parth
https://github.com/codemodus/parth
Contribute to codemodus/parth development by creating an account on GitHub.
- 1574Go client for NewsAPI
https://github.com/jellydator/newsapi-go
Go client for NewsAPI. Contribute to jellydator/newsapi-go development by creating an account on GitHub.
- 1575Simple Shopify API for the Go Programming Language
https://github.com/rapito/go-shopify
Simple Shopify API for the Go Programming Language - rapito/go-shopify
- 1576Parse and generate m3u8 playlists for Apple HTTP Live Streaming (HLS) in Golang (ported from gem https://github.com/sethdeckard/m3u8)
https://github.com/etherlabsio/go-m3u8
Parse and generate m3u8 playlists for Apple HTTP Live Streaming (HLS) in Golang (ported from gem https://github.com/sethdeckard/m3u8) - etherlabsio/go-m3u8
- 1577An extremely fast globally unique number generator.
https://github.com/edwingeng/wuid
An extremely fast globally unique number generator. - edwingeng/wuid
- 1578Simplest HTTP GET requester for Go with timeout support
https://github.com/inancgumus/myhttp
Simplest HTTP GET requester for Go with timeout support - inancgumus/myhttp
- 1579Command pattern for Go with thread safe serial and parallel dispatcher
https://github.com/txgruppi/command
Command pattern for Go with thread safe serial and parallel dispatcher - txgruppi/command
- 1580♻️ The most advanced interruptible mechanism to perform actions repetitively until successful.
https://github.com/kamilsk/retry
♻️ The most advanced interruptible mechanism to perform actions repetitively until successful. - kamilsk/retry
- 1581Golang scraper to get data from Google Play Store
https://github.com/n0madic/google-play-scraper
Golang scraper to get data from Google Play Store. Contribute to n0madic/google-play-scraper development by creating an account on GitHub.
- 1582Flexible regular expressions constructor for Golang.
https://github.com/hedhyw/rex
Flexible regular expressions constructor for Golang. - hedhyw/rex
- 1583Golang app shutdown hooks.
https://github.com/ztrue/shutdown
Golang app shutdown hooks. Contribute to ztrue/shutdown development by creating an account on GitHub.
- 1584Fast, zero-configuration, static HTTP filer server.
https://github.com/janiltonmaciel/statiks
Fast, zero-configuration, static HTTP filer server. - janiltonmaciel/statiks
- 1585A declarative struct-tag-based HTML unmarshaling or scraping package for Go built on top of the goquery library
https://github.com/andrewstuart/goq
A declarative struct-tag-based HTML unmarshaling or scraping package for Go built on top of the goquery library - andrewstuart/goq
- 1586Go client library for interacting with Coinpaprika's API
https://github.com/coinpaprika/coinpaprika-api-go-client
Go client library for interacting with Coinpaprika's API - coinpaprika/coinpaprika-api-go-client
- 1587Run functions resiliently in Go, catching and restarting panics
https://github.com/VividCortex/robustly
Run functions resiliently in Go, catching and restarting panics - VividCortex/robustly
- 1588Retrying made simple and easy for golang
https://github.com/rafaeljesus/retry-go
Retrying made simple and easy for golang :repeat: - GitHub - rafaeljesus/retry-go: Retrying made simple and easy for golang
- 1589go-redoc is an embedded OpenAPI/Swagger documentation ui for Go using ReDoc
https://github.com/mvrilo/go-redoc
go-redoc is an embedded OpenAPI/Swagger documentation ui for Go using ReDoc - mvrilo/go-redoc
- 1590OS Signal Handlers in Go
https://github.com/sinhashubham95/bleep
OS Signal Handlers in Go. Contribute to sinhashubham95/bleep development by creating an account on GitHub.
- 1591IP2Location.io Go SDK allows user to query for an enriched data set based on IP address and provides WHOIS lookup api that helps users to obtain domain information.
https://github.com/ip2location/ip2location-io-go
IP2Location.io Go SDK allows user to query for an enriched data set based on IP address and provides WHOIS lookup api that helps users to obtain domain information. - ip2location/ip2location-io-go
- 1592Go library MalShare API
https://github.com/MonaxGT/gomalshare
Go library MalShare API. Contribute to MonaxGT/gomalshare development by creating an account on GitHub.
- 1593Go Media Framework
https://github.com/3d0c/gmf
Go Media Framework. Contribute to 3d0c/gmf development by creating an account on GitHub.
- 1594Decode / encode XML to/from map[string]interface{} (or JSON); extract values with dot-notation paths and wildcards. Replaces x2j and j2x packages.
https://github.com/clbanning/mxj
Decode / encode XML to/from map[string]interface{} (or JSON); extract values with dot-notation paths and wildcards. Replaces x2j and j2x packages. - clbanning/mxj
- 1595A Go web framework and Headless CMS
https://github.com/fastschema/fastschema
A Go web framework and Headless CMS. Contribute to fastschema/fastschema development by creating an account on GitHub.
- 1596Benchmarks of Go serialization methods
https://github.com/alecthomas/go_serialization_benchmarks
Benchmarks of Go serialization methods. Contribute to alecthomas/go_serialization_benchmarks development by creating an account on GitHub.
- 1597opennota / re2dfa · GitLab
https://gitlab.com/opennota/re2dfa
Transform regular expressions into finite state machines and output Go source code
- 1598Go - IntelliJ IDEs Plugin | Marketplace
https://plugins.jetbrains.com/plugin/9568-go
This plugin extends IntelliJ IDEA Ultimate with Go-specific coding assistance and tool integrations, and has everything you could find in GoLand. Please note that the...
- 1599API framework written in Golang.
https://github.com/abemedia/go-don
API framework written in Golang. Contribute to abemedia/go-don development by creating an account on GitHub.
- 1600A tiny http middleware for Golang with added handlers for common needs.
https://github.com/InVisionApp/rye
A tiny http middleware for Golang with added handlers for common needs. - InVisionApp/rye
- 1601Go net/http handler to transparently manage posted JSON
https://github.com/rs/formjson
Go net/http handler to transparently manage posted JSON - rs/formjson
- 1602Use Go for AWS Lambda & API Gateway HttpApi
https://github.com/twharmon/golamb
Use Go for AWS Lambda & API Gateway HttpApi. Contribute to twharmon/golamb development by creating an account on GitHub.
- 1603common creational, behavioural and structural patterns implemented in go 🤩
https://github.com/shubhamzanwar/design-patterns
common creational, behavioural and structural patterns implemented in go 🤩 - shubhamzanwar/design-patterns
- 1604Super simple deployment tool
https://github.com/chrismckenzie/dropship
Super simple deployment tool. Contribute to ChrisMcKenzie/dropship development by creating an account on GitHub.
- 1605Go HTTP router
https://github.com/nbari/violetear
Go HTTP router. Contribute to nbari/violetear development by creating an account on GitHub.
- 1606beego is an open-source, high-performance web framework for the Go programming language.
https://github.com/beego/beego
beego is an open-source, high-performance web framework for the Go programming language. - beego/beego
- 1607Idiomatic HTTP Middleware for Golang
https://github.com/urfave/negroni
Idiomatic HTTP Middleware for Golang. Contribute to urfave/negroni development by creating an account on GitHub.
- 1608Goji is a minimalistic and flexible HTTP request multiplexer for Go (golang)
https://github.com/goji/goji
Goji is a minimalistic and flexible HTTP request multiplexer for Go (golang) - goji/goji
- 1609Gin is a HTTP web framework written in Go (Golang). It features a Martini-like API with much better performance -- up to 40 times faster. If you need smashing performance, get yourself some Gin.
https://github.com/gin-gonic/gin
Gin is a HTTP web framework written in Go (Golang). It features a Martini-like API with much better performance -- up to 40 times faster. If you need smashing performance, get yourself some Gin. - ...
- 1610Manage local application configuration files using templates and data from etcd or consul
https://github.com/kelseyhightower/confd
Manage local application configuration files using templates and data from etcd or consul - kelseyhightower/confd
- 1611GoTuna a lightweight web framework for Go with mux router, middlewares, user sessions, templates, embedded views, and static file server.
https://github.com/gotuna/gotuna
GoTuna a lightweight web framework for Go with mux router, middlewares, user sessions, templates, embedded views, and static file server. - gotuna/gotuna
- 1612Fruitygo / Pnutmux · GitLab
https://gitlab.com/fruitygo/pnutmux
🥜 Fabriktor's custom routing solution.
- 1613Lightning Fast HTTP Multiplexer
https://github.com/go-zoo/bone
Lightning Fast HTTP Multiplexer. Contribute to go-zoo/bone development by creating an account on GitHub.
- 1614Composable chains of nested http.Handler instances.
https://github.com/codemodus/chain
Composable chains of nested http.Handler instances. - codemodus/chain
- 1615A small and evil REST framework for Go
https://github.com/ungerik/go-rest
A small and evil REST framework for Go. Contribute to ungerik/go-rest development by creating an account on GitHub.
- 1616Flexible E-Commerce Framework on top of Flamingo. Used to build E-Commerce "Portals" and connect it with the help of individual Adapters to other services.
https://github.com/i-love-flamingo/flamingo-commerce
Flexible E-Commerce Framework on top of Flamingo. Used to build E-Commerce "Portals" and connect it with the help of individual Adapters to other services. - GitHub - i-love-flamingo/fla...
- 1617A microframework to build web apps; with handler chaining, middleware support, and most of all; standard library compliant HTTP handlers(i.e. http.HandlerFunc).
https://github.com/bnkamalesh/webgo
A microframework to build web apps; with handler chaining, middleware support, and most of all; standard library compliant HTTP handlers(i.e. http.HandlerFunc). - bnkamalesh/webgo
- 1618gin auto binding,grpc, and annotated route,gin 注解路由, grpc,自动参数绑定工具
https://github.com/xxjwxc/ginrpc
gin auto binding,grpc, and annotated route,gin 注解路由, grpc,自动参数绑定工具 - xxjwxc/ginrpc
- 1619:zap: Go web framework benchmark
https://github.com/smallnest/go-web-framework-benchmark
:zap: Go web framework benchmark. Contribute to smallnest/go-web-framework-benchmark development by creating an account on GitHub.
- 1620Library to use HTML5 Canvas from Go-WASM, with all drawing within go code
https://github.com/markfarnan/go-canvas
Library to use HTML5 Canvas from Go-WASM, with all drawing within go code - markfarnan/go-canvas
- 1621Go Server/API micro framework, HTTP request router, multiplexer, mux
https://github.com/vardius/gorouter
Go Server/API micro framework, HTTP request router, multiplexer, mux - vardius/gorouter
- 1622FastRouter is a fast, flexible HTTP router written in Go.
https://github.com/razonyang/fastrouter
FastRouter is a fast, flexible HTTP router written in Go. - razonyang/fastrouter
- 1623A high productivity, full-stack web framework for the Go language.
https://github.com/revel/revel
A high productivity, full-stack web framework for the Go language. - revel/revel
- 1624Dead simple rate limit middleware for Go.
https://github.com/ulule/limiter
Dead simple rate limit middleware for Go. Contribute to ulule/limiter development by creating an account on GitHub.
- 1625GoFrame is a modular, powerful, high-performance and enterprise-class application development framework of Golang.
https://github.com/gogf/gf
GoFrame is a modular, powerful, high-performance and enterprise-class application development framework of Golang. - GitHub - gogf/gf: GoFrame is a modular, powerful, high-performance and enterpri...
- 1626Lightweight Middleware for net/http
https://github.com/stephens2424/muxchain
Lightweight Middleware for net/http. Contribute to stephens2424/muxchain development by creating an account on GitHub.
- 1627A lightweight and fast http router from outer space
https://github.com/gernest/alien
A lightweight and fast http router from outer space - gernest/alien
- 1628High performance, minimalist Go web framework
https://github.com/labstack/echo
High performance, minimalist Go web framework. Contribute to labstack/echo development by creating an account on GitHub.
- 1629Welcome to aah Go web framework
https://aahframework.org
aah makes it easy to build secure, scalable, high performance Web and RESTful application with Go. Enterprise grade features OOTB. Code less, achieve goals and aah is free, open source.
- 1630Package gorilla/mux is a powerful HTTP router and URL matcher for building Go web servers with 🦍
https://github.com/gorilla/mux
Package gorilla/mux is a powerful HTTP router and URL matcher for building Go web servers with 🦍 - gorilla/mux
- 1631An opinionated GoLang framework for accelerated microservice development. Built in support for databases and observability.
https://github.com/gofr-dev/gofr
An opinionated GoLang framework for accelerated microservice development. Built in support for databases and observability. - gofr-dev/gofr
- 1632S3 Reverse Proxy with GET, PUT and DELETE methods and authentication (OpenID Connect and Basic Auth)
https://github.com/oxyno-zeta/s3-proxy
S3 Reverse Proxy with GET, PUT and DELETE methods and authentication (OpenID Connect and Basic Auth) - oxyno-zeta/s3-proxy
- 1633Integrates Spiffe and Vault to have secretless authentication
https://github.com/philips-labs/spiffe-vault
Integrates Spiffe and Vault to have secretless authentication - philips-labs/spiffe-vault
- 1634Simple and lightweight Go web framework inspired by koa
https://github.com/aisk/vox
Simple and lightweight Go web framework inspired by koa - aisk/vox
- 1635Jeffrey D. / blanket · GitLab
https://gitlab.com/verygoodsoftwarenotvirus/blanket
a coverage helper tool
- 1636A golang http router based on trie tree.
https://github.com/bmf-san/goblin
A golang http router based on trie tree. Contribute to bmf-san/goblin development by creating an account on GitHub.
- 1637Package macaron is a high productive and modular web framework in Go.
https://github.com/go-macaron/macaron
Package macaron is a high productive and modular web framework in Go. - go-macaron/macaron
- 1638Go Profiling - Visual Studio Marketplace
https://marketplace.visualstudio.com/items?itemName=MaxMedia.go-prof
Extension for Visual Studio Code - Go language profiling
- 1639DOM library for Go and WASM
https://github.com/dennwc/dom
DOM library for Go and WASM. Contribute to dennwc/dom development by creating an account on GitHub.
- 1640A high performance HTTP request router that scales well
https://github.com/julienschmidt/httprouter
A high performance HTTP request router that scales well - julienschmidt/httprouter
- 1641Yet Another REST Framework
https://github.com/yarf-framework/yarf
Yet Another REST Framework. Contribute to yarf-framework/yarf development by creating an account on GitHub.
- 1642Go compiler for small places. Microcontrollers, WebAssembly (WASM/WASI), and command-line tools. Based on LLVM.
https://github.com/tinygo-org/tinygo
Go compiler for small places. Microcontrollers, WebAssembly (WASM/WASI), and command-line tools. Based on LLVM. - tinygo-org/tinygo
- 1643xmux is a httprouter fork on top of xhandler (net/context aware)
https://github.com/rs/xmux
xmux is a httprouter fork on top of xhandler (net/context aware) - rs/xmux
- 1644⚡️ Express inspired web framework written in Go
https://github.com/gofiber/fiber
⚡️ Express inspired web framework written in Go. Contribute to gofiber/fiber development by creating an account on GitHub.
- 1645This library provides a simple microservice framework based on clean architecture principles with a working example implemented.
https://github.com/claygod/microservice
This library provides a simple microservice framework based on clean architecture principles with a working example implemented. - claygod/microservice
- 1646The web framework for writing faster sites, faster
https://github.com/rainycape/gondola
The web framework for writing faster sites, faster - rainycape/gondola
- 1647A lightweight yet powerful HTTP router for the Go programming language
https://github.com/golobby/router
A lightweight yet powerful HTTP router for the Go programming language - golobby/router
- 1648Middleware for HTTP services in Go
https://github.com/bobg/mid
Middleware for HTTP services in Go. Contribute to bobg/mid development by creating an account on GitHub.
- 1649Echo Inspired Stand Alone URL Router
https://github.com/husobee/vestigo
Echo Inspired Stand Alone URL Router. Contribute to husobee/vestigo development by creating an account on GitHub.
- 1650A Go middleware that stores various information about your web application (response time, status code count, etc.)
https://github.com/thoas/stats
A Go middleware that stores various information about your web application (response time, status code count, etc.) - thoas/stats
- 1651Simple middleware to rate-limit HTTP requests.
https://github.com/didip/tollbooth
Simple middleware to rate-limit HTTP requests. Contribute to didip/tollbooth development by creating an account on GitHub.
- 1652Painless middleware chaining for Go
https://github.com/justinas/alice
Painless middleware chaining for Go. Contribute to justinas/alice development by creating an account on GitHub.
- 1653Flamingo Framework and Core Library. Flamingo is a go based framework to build pluggable applications. Focus is on clean architecture, maintainability and operation readiness.
https://github.com/i-love-flamingo/flamingo
Flamingo Framework and Core Library. Flamingo is a go based framework to build pluggable applications. Focus is on clean architecture, maintainability and operation readiness. - i-love-flamingo/fla...
- 1654Huma REST/HTTP API Framework for Golang with OpenAPI 3.1
https://github.com/danielgtaylor/huma/
Huma REST/HTTP API Framework for Golang with OpenAPI 3.1 - danielgtaylor/huma
- 1655The world’s fastest framework for building websites
https://gohugo.io/
The world’s fastest framework for building websites
- 165634 gopher images for Go developers community
https://github.com/shalakhin/gophericons
34 gopher images for Go developers community. Contribute to shalakhin/gophericons development by creating an account on GitHub.
- 1657GopherCon Israel 2024
https://www.gophercon.org.il/
Best Go Conference in Israel
- 1658A Microservice Toolkit from The New York Times
https://github.com/NYTimes/gizmo
A Microservice Toolkit from The New York Times. Contribute to nytimes/gizmo development by creating an account on GitHub.
- 1659⏱ Benchmarks of machine learning inference for Go
https://github.com/nikolaydubina/go-ml-benchmarks
⏱ Benchmarks of machine learning inference for Go. Contribute to nikolaydubina/go-ml-benchmarks development by creating an account on GitHub.
- 1660Simple, modular, and observable Go framework for backend applications.
https://github.com/ankorstore/yokai
Simple, modular, and observable Go framework for backend applications. - ankorstore/yokai
- 1661Let’s Go Further!
https://lets-go-further.alexedwards.net
A guide to advanced patterns for developing, managing and deploying APIs and web applications with Go
- 1662a tool for code clone detection
https://github.com/mibk/dupl
a tool for code clone detection. Contribute to mibk/dupl development by creating an account on GitHub.
- 1663Run WASM tests inside your browser
https://github.com/agnivade/wasmbrowsertest
Run WASM tests inside your browser. Contribute to agnivade/wasmbrowsertest development by creating an account on GitHub.
- 1664A Golang Middleware to handle X-Forwarded-For Header
https://github.com/sebest/xff
A Golang Middleware to handle X-Forwarded-For Header - sebest/xff
- 1665A REST framework for quickly writing resource based services in Golang.
https://github.com/resoursea/api
A REST framework for quickly writing resource based services in Golang. - resoursea/api
- 1666🍐 The enterprise REST API framework
https://github.com/go-goyave/goyave
🍐 The enterprise REST API framework. Contribute to go-goyave/goyave development by creating an account on GitHub.
- 1667:bullettrain_side: High-performance web server for Go (2016). New alpha (2024) with even better performance is currently in development at https://git.akyoto.dev/go/web
https://github.com/aerogo/aero
:bullettrain_side: High-performance web server for Go (2016). New alpha (2024) with even better performance is currently in development at https://git.akyoto.dev/go/web - aerogo/aero
- 1668An opinionated guideline to structure & develop a Go web application/service
https://github.com/bnkamalesh/goapp
An opinionated guideline to structure & develop a Go web application/service - bnkamalesh/goapp
- 1669Simple HTTP router for Go
https://github.com/ngamux/ngamux
Simple HTTP router for Go. Contribute to ngamux/ngamux development by creating an account on GitHub.
- 1670Gophers Japan
https://gocon.connpass.com/
Goの集まり https://github.com/GoCon/GoCon
- 1671hiboot is a high performance web and cli application framework with dependency injection support
https://github.com/hidevopsio/hiboot
hiboot is a high performance web and cli application framework with dependency injection support - hidevopsio/hiboot
- 1672A CLI tool for Kafka
https://github.com/xitonix/trubka
A CLI tool for Kafka. Contribute to xitonix/trubka development by creating an account on GitHub.
- 1673Spriting that sass has been missing
https://github.com/wellington/wellington
Spriting that sass has been missing. Contribute to wellington/wellington development by creating an account on GitHub.
- 1674Codegen for functional options in go projects
https://github.com/kazhuravlev/options-gen
Codegen for functional options in go projects. Contribute to kazhuravlev/options-gen development by creating an account on GitHub.
- 1675Lightweight web framework based on net/http.
https://github.com/twharmon/goweb
Lightweight web framework based on net/http. Contribute to twharmon/goweb development by creating an account on GitHub.
- 1676Microservice framework following best cloud practices with a focus on productivity.
https://github.com/beatlabs/patron
Microservice framework following best cloud practices with a focus on productivity. - beatlabs/patron
- 1677A live-updating version of the UNIX wc command.
https://github.com/timdp/lwc
A live-updating version of the UNIX wc command. Contribute to timdp/lwc development by creating an account on GitHub.
- 1678A modern load testing tool, using Go and JavaScript - https://k6.io
https://github.com/grafana/k6
A modern load testing tool, using Go and JavaScript - https://k6.io - grafana/k6
- 1679The lightweight and powerful web framework using the new way for Go.Another go the way.
https://github.com/go-the-way/anoweb
The lightweight and powerful web framework using the new way for Go.Another go the way. - go-the-way/anoweb
- 1680Go package that provides multiple middlewares for Echo Framework.
https://github.com/faabiosr/echo-middleware
Go package that provides multiple middlewares for Echo Framework. - faabiosr/echo-middleware
- 1681go-tools/cmd/staticcheck at master · dominikh/go-tools
https://github.com/dominikh/go-tools/tree/master/cmd/staticcheck
Staticcheck - The advanced Go linter. Contribute to dominikh/go-tools development by creating an account on GitHub.
- 1682🌟 Goa: Elevate Go API development! 🚀 Streamlined design, automatic code generation, and seamless HTTP/gRPC support. ✨
https://github.com/goadesign/goa
🌟 Goa: Elevate Go API development! 🚀 Streamlined design, automatic code generation, and seamless HTTP/gRPC support. ✨ - goadesign/goa
- 1683KubeVPN offers a Cloud Native Dev Environment that connects to kubernetes cluster network. Gain access to k8s cluster network using service names or Pod IP/Service IP. Intercept k8s cluster service inbound traffic to local PC through a service mesh. Run your k8s pod within a local Docker container with identical env、volume and network setup.
https://github.com/kubenetworks/kubevpn
KubeVPN offers a Cloud Native Dev Environment that connects to kubernetes cluster network. Gain access to k8s cluster network using service names or Pod IP/Service IP. Intercept k8s cluster service...
- 1684Shell script to download and set GO environmental paths to allow multiple versions.
https://github.com/cryptojuice/gobrew
Shell script to download and set GO environmental paths to allow multiple versions. - cryptojuice/gobrew
- 1685Extism Go SDK
https://github.com/extism/go-sdk
Extism Go SDK. Contribute to extism/go-sdk development by creating an account on GitHub.
- 1686Simple, lightweight and faster response (JSON, JSONP, XML, YAML, HTML, File) rendering package for Go
https://github.com/thedevsaddam/renderer
Simple, lightweight and faster response (JSON, JSONP, XML, YAML, HTML, File) rendering package for Go - thedevsaddam/renderer
- 1687golang http router with elegance, speed, and flexibility
https://github.com/muir/nchi
golang http router with elegance, speed, and flexibility - muir/nchi
- 1688:rocket: Modern cross-platform HTTP load-testing tool written in Go
https://github.com/rogerwelin/cassowary
:rocket: Modern cross-platform HTTP load-testing tool written in Go - rogerwelin/cassowary
- 1689Goa is a web framework based on middleware, like koa.js.
https://github.com/goa-go/goa
Goa is a web framework based on middleware, like koa.js. - goa-go/goa
- 1690⚡ Rux is an simple and fast web framework. support route group, param route binding, middleware, compatible http.Handler interface. 简单且快速的 Go api/web 框架,支持路由分组,路由参数绑定,中间件,兼容 http.Handler 接口
https://github.com/gookit/rux
⚡ Rux is an simple and fast web framework. support route group, param route binding, middleware, compatible http.Handler interface. 简单且快速的 Go api/web 框架,支持路由分组,路由参数绑定,中间件,兼容 http.Handler 接口 - gooki...
- 1691xujiajun/gorouter is a simple and fast HTTP router for Go. It is easy to build RESTful APIs and your web framework.
https://github.com/xujiajun/gorouter
xujiajun/gorouter is a simple and fast HTTP router for Go. It is easy to build RESTful APIs and your web framework. - xujiajun/gorouter
- 1692Go Faster
https://leanpub.com/gofaster
Go Faster is book on the Golang programming language which focuses on the harder to learn bits. Available now at Leanpub.com
- 1693:golf: The Golf web framework
https://github.com/dinever/golf
:golf: The Golf web framework. Contribute to dinever/golf development by creating an account on GitHub.
- 1694lightweight, idiomatic and composable router for building Go HTTP services
https://github.com/go-chi/chi
lightweight, idiomatic and composable router for building Go HTTP services - go-chi/chi
- 1695:satellite: A cross-platform task runner for executing commands and generating files from templates
https://github.com/gulien/orbit
:satellite: A cross-platform task runner for executing commands and generating files from templates - gulien/orbit
- 1696gitea-github-migrator
https://git.jonasfranz.software/JonasFranzDEV/gitea-github-migrator
A tool to migrate GitHub Repositories to Gitea including all issues
- 1697Go middleware for monetizing your API on a per-request basis with Bitcoin and Lightning ⚡️
https://github.com/philippgille/ln-paywall
Go middleware for monetizing your API on a per-request basis with Bitcoin and Lightning ⚡️ - philippgille/ln-paywall
- 1698Go (golang) library for creating and consuming HTTP Server-Timing headers
https://github.com/mitchellh/go-server-timing
Go (golang) library for creating and consuming HTTP Server-Timing headers - mitchellh/go-server-timing
- 1699The web framework for Golang
https://github.com/uadmin/uadmin
The web framework for Golang. Contribute to uadmin/uadmin development by creating an account on GitHub.
- 1700Go microservice template for Kubernetes
https://github.com/stefanprodan/podinfo
Go microservice template for Kubernetes. Contribute to stefanprodan/podinfo development by creating an account on GitHub.
- 1701GopherCon Europe 2024
https://gophercon.eu/
GopherCon Europe 2024
- 1702Go micro-benchmarks for calculating the speed of language constructs
https://github.com/feyeleanor/GoSpeed
Go micro-benchmarks for calculating the speed of language constructs - feyeleanor/gospeed
- 1703A lightweight MVC framework for Go(Golang)
https://github.com/gernest/utron
A lightweight MVC framework for Go(Golang). Contribute to gernest/utron development by creating an account on GitHub.
- 1704gRPC interceptor catenation.
https://github.com/codemodus/catena
gRPC interceptor catenation. Contribute to codemodus/catena development by creating an account on GitHub.
- 1705An HTTP client for go-server-timing middleware. Enables automatic timing propagation through HTTP calls between servers.
https://github.com/posener/client-timing
An HTTP client for go-server-timing middleware. Enables automatic timing propagation through HTTP calls between servers. - posener/client-timing
- 1706Copy files and artifacts via SSH using a binary, docker or Drone CI.
https://github.com/appleboy/drone-scp
Copy files and artifacts via SSH using a binary, docker or Drone CI. - appleboy/drone-scp
- 1707From local development to the cloud: web apps development with containers made easy.
https://github.com/kool-dev/kool
From local development to the cloud: web apps development with containers made easy. - kool-dev/kool
- 1708Go HTTP framework with high-performance and strong-extensibility for building micro-services.
https://github.com/cloudwego/hertz
Go HTTP framework with high-performance and strong-extensibility for building micro-services. - cloudwego/hertz
- 1709Let’s Go! Learn to build web apps with Go
https://lets-go.alexedwards.net
A clear and concise guide to practical code patterns, project organization, best practices and more.
- 1710HTTP load testing tool and library. It's over 9000!
https://github.com/tsenart/vegeta
HTTP load testing tool and library. It's over 9000! - tsenart/vegeta
- 1711Go Route - Simple yet powerful HTTP request multiplexer
https://github.com/goroute/route
Go Route - Simple yet powerful HTTP request multiplexer - goroute/route
- 1712:bell: A simple Go router
https://github.com/GuilhermeCaruso/bellt
:bell: A simple Go router. Contribute to GuilhermeCaruso/bellt development by creating an account on GitHub.
- 1713Go Web Framework
https://github.com/ivpusic/neo
Go Web Framework. Contribute to ivpusic/neo development by creating an account on GitHub.
- 1714Go With The Domain
https://threedots.tech/go-with-the-domain/
Go With The Domain Three Dots Labs Sign up to our newsletter and get your copy of Go with the Domain e-book. Subscribe You write business software Okay, we can’t know that for sure. But if you work on any web application or user-facing product, chances are you work with complex business domains. Go 2023 Survey shows that 74% of respondents write API/RPC services, and 42% use Go for web programming.
- 1715Golang Fuego - web framework generating OpenAPI 3 spec from source code
https://github.com/go-fuego/fuego
Golang Fuego - web framework generating OpenAPI 3 spec from source code - go-fuego/fuego
- 1716WebAssembly interop between Go and JS values.
https://github.com/norunners/vert
WebAssembly interop between Go and JS values. Contribute to norunners/vert development by creating an account on GitHub.
- 1717Composable framework for writing HTTP handlers in Go.
https://github.com/VividCortex/siesta
Composable framework for writing HTTP handlers in Go. - VividCortex/siesta
- 1718Bxog is a simple and fast HTTP router for Go (HTTP request multiplexer).
https://github.com/claygod/Bxog
Bxog is a simple and fast HTTP router for Go (HTTP request multiplexer). - claygod/Bxog
- 1719A curated list of awesome awesomeness
https://github.com/bayandin/awesome-awesomeness
A curated list of awesome awesomeness. Contribute to bayandin/awesome-awesomeness development by creating an account on GitHub.
- 1720:non-potable_water: Is a lightweight HTTP router that sticks to the std "net/http" implementation
https://github.com/go-playground/pure
:non-potable_water: Is a lightweight HTTP router that sticks to the std "net/http" implementation - go-playground/pure
- 1721Add interceptors to GO http.Client
https://github.com/HereMobilityDevelopers/mediary
Add interceptors to GO http.Client. Contribute to HereMobilityDevelopers/mediary development by creating an account on GitHub.
- 1722Go Lang Web Assembly bindings for DOM, HTML etc
https://github.com/gowebapi/webapi
Go Lang Web Assembly bindings for DOM, HTML etc. Contribute to gowebapi/webapi development by creating an account on GitHub.
- 1723Go in Practice, Second Edition
https://www.manning.com/books/go-in-practice-second-edition
Practical techniques for building concurrent, cloud-native, and high performance Go applications—all accelerated with productivity-boosting AI tools.</b> Go in Practice, Second Edition</i> is a cookbook-style collection of the techniques and best practices you need to build production-quality Go applications. It builds on your existing knowledge of the Go language, introducing specific strategies you can use to maximize your productivity in day-to-day dev work. In Go in Practice, Second Edition</i>, you’ll learn: Concurrency with goroutines and channels</li> Web servers and microservices with event streams and websockets</li> Logging, caching, and data access from environment variables and files</li> Cloud-native Go applications</li> AI tools to accelerate your development workflow</li> </ul> Go in Practice, Second Edition</i> has been extensively revised by author Nathan Kozyra to cover the latest version of Go, along with new dev techniques, including productivity-boosting AI tools. It follows an instantly-familiar cookbook-style Problem/Solution/Discussion format, building on what you already know about Go with advanced or little-known techniques for concurrency, logging and caching, microservices, testing, deployment, and more.
- 1724Golang Bangalore | Meetup
https://www.meetup.com/Golang-Bangalore/
A meetup group to discuss the Go Programming Language. The Go programming language is an open source project to make programmers more productive. Go is expressive, concise, clean, and efficient. Its concurrency mechanisms make it easy to write programs that get the most out of multi-core and n
- 1725Golang Amsterdam | Meetup
https://www.meetup.com/golang-amsterdam/
This group is for everybody who is interested in the Go programming language (https://go.dev/). We organize meetups with technical talks and social events.If you're interested in hosting a meetup or giving a talk, please contact one of us at a meetup, through the forms:* [I want to be a speaker](htt
- 1726tools/gopls/README.md at master · golang/tools
https://github.com/golang/tools/blob/master/gopls/README.md
[mirror] Go Tools. Contribute to golang/tools development by creating an account on GitHub.
- 1727Parallel S3 and local filesystem execution tool.
https://github.com/peak/s5cmd
Parallel S3 and local filesystem execution tool. Contribute to peak/s5cmd development by creating an account on GitHub.
- 1728Golang DC | Meetup
https://www.meetup.com/Golang-DC/
A group focused on the Go programming language(golang). We want to learn about Golang's role in highly concurrent network services, concurrency, language design, and other related topics.Organized and sponsored by hatchpad - https://dmv.myhatchpad.com
- 1729GoLab | The International Conference on Go in Florence
https://golab.io/
GoLab is a conference for developers made by developers
- 1730Golang Argentina | Meetup
https://www.meetup.com/Golang-Argentina/
**¡Gophers en Argentina! 👋** Queremos impulsar el uso y el conocimiento de este hermoso, simple y eficiente lenguaje en Argentina. Juntarse a compartir conocimiento, experiencias y pasar un buen momento.
- 1731Utah Go User Group | Meetup
https://www.meetup.com/utahgophers/
The Utah Go User Group (#UTGO) is a community of people interested in software development and Google's Go programming language (Golang). Everyone is welcome. We currently meet in Lehi. To discuss and vote on topic ideas, go to [https://github.com/forgeutah/utah-go](https://github.com/forgeutah/uta
- 1732404 Page Not Found - codewithmukesh
https://codewithmukesh.com/blog/category/golang
Page Not Found.
- 1733Go Stockholm | Meetup
https://www.meetup.com/Go-Stockholm/
A group intended to enable golang nuts from Stockholm to meet up and share experiences. Join if you are interested in meeting other golang nuts for informal presentations and possibly even beer and pizza.To email the group about relevant topics such as Go or recruiting Go developers, send to Go
- 1734Golax, a go implementation for the Lax framework.
https://github.com/fulldump/golax
Golax, a go implementation for the Lax framework. Contribute to fulldump/golax development by creating an account on GitHub.
- 1735Awesome Go | LibHunt
https://go.libhunt.com
Your go-to Go Toolbox. A curated list of awesome Go packages, frameworks and resources. 3017 projects organized into 129 categories.
- 1736dropbox based blog engine, written in go.
https://github.com/tejo/boxed
dropbox based blog engine, written in go. Contribute to tejo/boxed development by creating an account on GitHub.
- 1737This is only a mirror and Moved to https://gitea.com/lunny/tango
https://github.com/lunny/tango
This is only a mirror and Moved to https://gitea.com/lunny/tango - lunny/tango
- 1738Golang Dorset | Meetup
https://www.meetup.com/golang-dorset/
Join our discord server: [https://discord.gg/UZuTbrRyur](https://discord.gg/UZuTbrRyur)**Golang Dorset** is a user group for people interested in the [Go programming language](https://golang.org/) and assorted related technologies and methodologies (kubernetes / docker / DevOps etc)We aim to meet ev
- 1739Atlanta Go User Group | Meetup
https://www.meetup.com/Go-Users-Group-Atlanta/
Go Users Group Atlanta is a meeting place for people interested in developing and promoting the local Go development community.We meet monthly at the Fullstory office at [1745 Peachtree Rd NW Suite G](https://goo.gl/maps/onq9DV3uj2vY2hSv6?coh=178572&entry=tt). Typically we have pizza/beer and social
- 1740London Gophers | Meetup
https://www.meetup.com/Go-London-User-Group/
London Gophers ([https://gophers.london](https://gophers.london)), aka Go London User Group (GLUG), is a London-based community for anyone interested in the Go programming language.London Gophers provides opportunities to:\- Discuss Go and related topics\- Socialise with friendly people who are inte
- 1741Athens Gophers | Meetup
https://www.meetup.com/Athens-Gophers/
Welcome to Athens Gophers.This is a group for all those interested in learning about and working with the Go programming language (Golang). Anyone interested in Co-organizing or Presenting in future meetups, please get in contact.About Us: As gophers we follow the Code of Conduct to make this a plea
- 1742Golang Estonia | Meetup
https://www.meetup.com/Golang-Estonia/
This is a group for people interested in the Go programming language. All aspiring, current and past Gophers are welcome. The goals of this Meetup are: * Give professional content - talks, workshops, lightning talks * Share ideas and experiences, spark interest * Build a community * Foster professio
- 1743Belfast Gophers | Meetup
https://www.meetup.com/Belfast-Gophers/
Belfast Gophers, aka The Go Belfast User Group (GBUG), are a Belfast-based community for anyone interested in the Go programming language. Belfast Gophers held their first Meet-up 2016, and since then the group has grown to over 300 gophers. Membership of Belfast Gophers is managed via our Meet
- 1744Go Cph | Meetup
https://www.meetup.com/Go-Cph/
(English below)Denne gruppe er for folk interesseret i Go i København og omegn.Vi mødes ca hver anden måned et sted i København og vi tilstræber både at have et fagligt indhold i form af tech talks og så naturligvis hygge og netværking.Gruppen er både for de nysgerrige, dem der allerede kender Go og
- 1745Basel Go Meetup | Meetup
https://www.meetup.com/Basel-Go-Meetup/
This is a group for everyone interested in the Go programming language. If you are a user of the language, a consumer of software written in Go, or if you are just learning how Go works and you want to chat with like-minded people, this group is for you.
- 1746Golang Rotterdam | Meetup
https://www.meetup.com/golang-rotterdam/
This group is for everybody who is interested in the Go programming language ([https://golang.org/](https://golang.org/)). We organize meetups with technical talks and social events. Contact us if you're interested in hosting a meetup or giving a talk!
- 1747Boston Golang | Meetup
https://www.meetup.com/bostongo/
A forum for people working with Go to discuss ideas, issues and share solutions. We will start to put together meetings as the group grows and topics are suggested.Submit a talk proposal, or come join us in #boston on Slack at https://invite.slack.golangbridge.orgBoston Golang officially adopts
- 1748golang-syd | Meetup
https://www.meetup.com/golang-syd/
Welcome to the GolangSyd Meetup Group, where Sydney's Gophers converge!GolangSyd is the ultimate gathering spot for anyone passionate about the Go programming language in Sydney. Whether you're a professional developer, a hobbyist, or just curious about Go, this community is for you!Our dedicated or
- 1749Ukrainian Golang User Groups | Meetup
https://www.meetup.com/uagolang/
Kyiv meetups for gophers. Let's share our experience!
- 1750Pleasures for Web in Golang
https://github.com/goanywhere/rex
Pleasures for Web in Golang. Contribute to goanywhere/rex development by creating an account on GitHub.
- 1751Go package for easily rendering JSON, XML, binary data, and HTML templates responses.
https://github.com/unrolled/render
Go package for easily rendering JSON, XML, binary data, and HTML templates responses. - unrolled/render
- 1752A simple, fast and expressive HTTP framework for Go.
https://github.com/jvcoutinho/lit
A simple, fast and expressive HTTP framework for Go. - jvcoutinho/lit
- 1753An ideally refined web framework for Go.
https://github.com/aofei/air
An ideally refined web framework for Go. Contribute to aofei/air development by creating an account on GitHub.
- 1754Bärner Go Meetup | Meetup
https://www.meetup.com/berner-go-meetup/
Das **Bärner Go Meetup** ist eine Gruppe für Go Programmierer aus der Region Bern. Wir bieten Euch die Plattform für spannende Präsentationen und angeregten Austausch rund um die Programmiersprache Go (Golang).\-\-\-**Bärner Go Meetup** is a group for Go Developers in and around Berne. We encourage
- 1755A high performance fasthttp request router that scales well
https://github.com/buaazp/fasthttprouter
A high performance fasthttp request router that scales well - buaazp/fasthttprouter
- 1756Cardiff Go Meetup | Meetup
https://www.meetup.com/Cardiff-Go-Meetup/
Hey there, if you're in the Cardiff area and interested in the Go programming language, this is for you. We run a mix of hack nights, training sessions, guest speakers and social evening. Speaker? Event ideas? Topic requests? Venue offer? Drinks? Food? Co-organiser? Do get in touch!You can find us o
- 1757Golang Kathmandu | Meetup
https://www.meetup.com/Golang-Kathmandu/
Golang Kathmandu is a meetup group, started for collaborating, sharing experience, and learning as a community.We will be organizing regular meetups as well as workshops on Golang. You can message me on meetup.com if you have any topic to share or hear about.#GolangKathmandu
- 1758Go net/http configurable handler to handle CORS requests
https://github.com/rs/cors
Go net/http configurable handler to handle CORS requests - rs/cors
- 1759GoJakarta | Meetup
https://www.meetup.com/GoJakarta/
A meetup group to discuss the Go Programming Language in Jakarta. (from golang.org) The Go programming language is an open source project to make programmers more productive. Go is expressive, concise, clean, and efficient. Its concurrency mechanisms make it easy to write programs that get
- 1760GopherCon Russia 2021
https://www.gophercon-russia.ru
Конференция разработчиков на Go, 23-25 апреля 2021 года, Online.
- 1761Golang Aix Marseille | Meetup
https://www.meetup.com/fr-FR/Golang-Marseille/
Ce groupe est ouvert à tous ceux qui s'intéressent de près ou de loin au langage Go : des curieux aux utilisateurs expérimentés.
- 1762GoSG | Meetup
https://www.meetup.com/golangsg/
About Go language The Go programming language is an open source project to make programmers more productive. Go is expressive, concise, clean, and efficient. Its concurrency mechanisms make it easy to write programs that get the most out of multicore and networked machines, while its novel typ
- 1763XML to MAP converter written Golang
https://github.com/sbabiv/xml2map
XML to MAP converter written Golang. Contribute to sbabiv/xml2map development by creating an account on GitHub.
- 1764GO Belo Horizonte | Meetup
https://www.meetup.com/go-belo-horizonte/
Ponto de encontro dos Gophpers de Belo Horizonte e região. O objetivo desse meetup é proporcionar encontros periódicos da comunidade Go de BH.Estamos também no canal #belohorizonte no gophers.slack.com
- 1765win32 ole implementation for golang
https://github.com/go-ole/go-ole
win32 ole implementation for golang. Contribute to go-ole/go-ole development by creating an account on GitHub.
- 1766Golang Serbia | Meetup
https://www.meetup.com/golang-serbia/
This is initial group of golang enthusiast and professionals
- 1767High performance and extensible micro web framework. Zero memory allocations in hot paths.
https://github.com/savsgio/atreugo
High performance and extensible micro web framework. Zero memory allocations in hot paths. - savsgio/atreugo
- 1768Brisbane Gophers | Meetup
https://www.meetup.com/Brisbane-Golang-Meetup/
Meet and share your Golang projects and experiences with other Gophers in Brisbane.Follow us on Twitter at https://twitter.com/golangbris
- 1769Production-Grade Container Scheduling and Management
https://github.com/kubernetes/kubernetes
Production-Grade Container Scheduling and Management - kubernetes/kubernetes
- 1770GoSDDL converter
https://github.com/MonaxGT/gosddl
GoSDDL converter. Contribute to MonaxGT/gosddl development by creating an account on GitHub.
- 1771Go(lang) benchmarks - (measure the speed of golang)
https://github.com/SimonWaldherr/golang-benchmarks
Go(lang) benchmarks - (measure the speed of golang) - SimonWaldherr/golang-benchmarks
- 1772⚡️ A lightning fast HTTP router
https://github.com/gowww/router
⚡️ A lightning fast HTTP router. Contribute to gowww/router development by creating an account on GitHub.
- 1773Pulse: A Golang framework for web development
https://github.com/gopulse/pulse
Pulse: A Golang framework for web development. Contribute to gopulse/pulse development by creating an account on GitHub.
- 1774Istanbul Golang | Meetup
https://www.meetup.com/Istanbul-Golang/
This is a meetup group for Go Programming Language for interested Gophers living in Istanbul. Interest in the language is all you need, no experience is necessary.
- 1775Golang Bulgaria | Meetup
https://www.meetup.com/Golang-Bulgaria/
An open meetup for everybody and anybody interested in Golang, CSP, software development. Experienced & aspiring software developers as well as actual gophers are all welcome!
- 1776The golang fuzzy-find cli jira interface 🚀
https://github.com/mk-5/fjira
The golang fuzzy-find cli jira interface 🚀. Contribute to mk-5/fjira development by creating an account on GitHub.
- 1777Golang Москва | Meetup
https://www.meetup.com/Golang-Moscow/
Встречи русскоязычного сообщества разработчиков на Go. Мы рады видеть всех – от любознательных новичков до маститых профессионалов.Google-группа: golang-ru.
- 1778GolangCWB | Meetup
https://www.meetup.com/GolangCWB/
"Go is an open source programming language that makes it easy to build simple, reliable, and efficient software." O objetivo deste grupo é organizar encontros para pessoas interessadas em Golang, independente do nível de experiência com a linguagem. Encontre-nos em outras redes:• Telegram
- 1779Golang SP | Meetup
https://www.meetup.com/golangbr/
**Somos a comunidade Go de São Paulo 🤟💙**Somos uma comunidade para pessoas interessada em Go (www.golangbr.org) e temos como objetivo principal organizar encontros mensais em São Paulo/Brasil e disseminar cada vez mais a linguagem Go junto a comunidade.**Quer palestrar na comunidade?**Preencha o f
- 1780GoBandung | Meetup
https://www.meetup.com/GoBandung/
A meetup group to discuss all about Go Programming Language in Bandung - West Java. All topics and skill levels are welcome
- 1781Mango is a modular web-application framework for Go, inspired by Rack, and PEP333.
https://github.com/paulbellamy/mango
Mango is a modular web-application framework for Go, inspired by Rack, and PEP333. - paulbellamy/mango
- 1782Gurgaon Go Meetup | Meetup
https://www.meetup.com/Gurgaon-Go-Meetup/
This group is for people interested in learning both the programming language Go(lang), and how to build interesting things with it. We will aim the first few sessions at beginner-intermediate levels and maybe later introduce some high-level talks. WE ARE ACTIVELY LOOKING FOR MEETUP VENUES.Want to h
- 1783GDG Go & Cloud User Group Hamburg | Meetup
https://www.meetup.com/Go-User-Group-Hamburg/
A meetup for users of the Go programming language and anything about cloud technologies in the Hamburg area.
- 1784Zürich Gophers | Meetup
https://www.meetup.com/zurich-gophers/
Meetup group for Go programmers in and around Greater Zürich Area.GitHub Discussions: https://gophers.ch/
- 1785Go Remote Meetup | Meetup
https://www.meetup.com/Go-Remote-Meetup/
Welcome Gophers! Go Remote Meetup is a remote-first group for Go developers worldwide. We're part of the Go Developer Network, and we're excited to connect with you all, no matter where you're located.About Go:Go is an open source programming language that makes it easy to build simple, reliable, an
- 1786Golang Melbourne | Meetup
https://www.meetup.com/golang-mel/
Calling all Melbourne Gophers. Any skill level, even those that have just heard about go and are interested
- 1787Swagger 2.0 implementation for go
https://github.com/go-swagger/go-swagger
Swagger 2.0 implementation for go. Contribute to go-swagger/go-swagger development by creating an account on GitHub.
- 1788vienna.go (Golang) | Meetup
https://www.meetup.com/viennago/
Go (golang) programming language user group for Vienna, Austria.Official Go website: https://golang.org/
- 1789Golang Taipei | Meetup
https://www.meetup.com/golang-taipei-meetup/
At Golang Taipei, we promote Golang development and sharing the latest information about Golang. Join our Facebook group to connect with other 6,138 members. Chat with us on Telegram channel with other 880 chatty members. Find all past meetup presentation fil
- 1790GolangVan | Meetup
https://www.meetup.com/golangvan/
We are a group of Go lovers (a.k.a. Gophers) in the Vancouver area. This is a semi regular group hoping to get Gophers in the Vancouver area together to discuss their experiences using and promoting Go.Code of Conduct: https://golang.org/conductTalk Submissions: https://forms.gle/C7NwhmJD8
- 1791apicompat checks recent changes to a Go project for backwards incompatible changes
https://github.com/bradleyfalzon/apicompat
apicompat checks recent changes to a Go project for backwards incompatible changes - bradleyfalzon/apicompat
- 1792Golang Ifood API SDK
https://github.com/arxdsilva/golang-ifood-sdk
Golang Ifood API SDK . Contribute to arxdsilva/golang-ifood-sdk development by creating an account on GitHub.
- 1793errcheck checks that you checked errors.
https://github.com/kisielk/errcheck
errcheck checks that you checked errors. Contribute to kisielk/errcheck development by creating an account on GitHub.
- 1794Clean architecture validator for go, like a The Dependency Rule and interaction between packages in your Go projects.
https://github.com/roblaszczak/go-cleanarch
Clean architecture validator for go, like a The Dependency Rule and interaction between packages in your Go projects. - roblaszczak/go-cleanarch
- 1795Go (Golang) Tutorials & Examples | gosamples.dev
https://gosamples.dev/
Learn Go programming by example. **GOSAMPLES** is a library of Go tutorials and examples that helps you solve everyday code problems.
- 1796GoCracow | Meetup
https://www.meetup.com/GoCracow/
It's a meetup located in Cracow for those who are interested in Go programming language, its ecosystem and related technologies.YouTube: [https://www.youtube.com/@gocracow](https://www.youtube.com/@gocracow)Talk proposal form: [https://docs.google.com/forms/d/e/1FAIpQLSeTAkTCdU9buxtpvTHWe8c31n6K-RKi
- 1797Women Who Go | Meetup
https://www.meetup.com/Women-Who-Go/
I had been going to GoSF meetups for about 2 years before I started this group. When the events were small (~50 people), I was usually the only woman. Now the events are larger (~250 people), and I am often still the only woman. This makes me sad.I am hoping that this group will provide a better ent
- 1798An Microsoft Visual Code extension for Golang to print symbol definition to output
https://github.com/msyrus/vscode-go-doc
An Microsoft Visual Code extension for Golang to print symbol definition to output - msyrus/vscode-go-doc
- 1799Visualise Go program GC trace data in real time
https://github.com/davecheney/gcvis
Visualise Go program GC trace data in real time. Contribute to davecheney/gcvis development by creating an account on GitHub.
- 1800xmlwriter is a pure-Go library providing procedural XML generation based on libxml2's xmlwriter module
https://github.com/shabbyrobe/xmlwriter
xmlwriter is a pure-Go library providing procedural XML generation based on libxml2's xmlwriter module - shabbyrobe/xmlwriter
- 1801Go TO | Meetup
https://www.meetup.com/go-toronto/
[https://golang.to/](https://golang.to/)This is GoTO, meant to bring Toronto and GTA Gophers together so we can learn and collaborate, regardless of skill level. This group is intended to provide:• avenues for continuous improvement and growth• a platform for developers to present their ideas and e
- 1802Thanos
https://thanos.io/tip/contributing/coding-style-guide.md/
Thanos - Highly available Prometheus setup with long term storage capabilities
- 1803Golang Lyon | Meetup
https://www.meetup.com/Golang-Lyon/
Ce groupe s'adresse à toute personne s'intéressant de près ou de loin au langage Go : curieux, débutants ou personnes expérimentées !Plus d'informations sur le langage Go : https://golang.org/ Crédits : la mascotte utilisée en image de profil du groupe a été dessinée par Renee French (htt
- 1804A different approach to Go web frameworks
https://github.com/mustafaakin/gongular
A different approach to Go web frameworks. Contribute to mustafaakin/gongular development by creating an account on GitHub.
- 1805High-speed, flexible tree-based HTTP router for Go.
https://github.com/dimfeld/httptreemux
High-speed, flexible tree-based HTTP router for Go. - dimfeld/httptreemux
- 1806Sourcegraph docs
https://docs.sourcegraph.com/dev/background-information/languages/go
Documentation for Sourcegraph, the code intelligence platform.
- 1807Go Forum
https://forum.golangbridge.org
The community-driven Go discussion site that keeps on giving
- 1808Golang AST visualizer
https://github.com/yuroyoro/goast-viewer
Golang AST visualizer. Contribute to yuroyoro/goast-viewer development by creating an account on GitHub.
- 1809Find which of your direct GitHub dependencies is susceptible to RepoJacking attacks
https://github.com/Checkmarx/chainjacking
Find which of your direct GitHub dependencies is susceptible to RepoJacking attacks - Checkmarx/chainjacking
- 1810Gophercises
https://gophercises.com/
Coding exercises for budding gophers
- 1811:rotating_light: Is a lightweight, fast and extensible zero allocation HTTP router for Go used to create customizable frameworks.
https://github.com/go-playground/lars
:rotating_light: Is a lightweight, fast and extensible zero allocation HTTP router for Go used to create customizable frameworks. - go-playground/lars
- 1812An extremely fast Go (golang) HTTP router that supports regular expression route matching. Comes with full support for building RESTful APIs.
https://github.com/go-ozzo/ozzo-routing
An extremely fast Go (golang) HTTP router that supports regular expression route matching. Comes with full support for building RESTful APIs. - go-ozzo/ozzo-routing
- 1813Go Router + Middleware. Your Contexts.
https://github.com/gocraft/web
Go Router + Middleware. Your Contexts. Contribute to gocraft/web development by creating an account on GitHub.
- 1814Go Tutorial
https://www.tutorialspoint.com/go/index.htm
Go Tutorial - Go language is a programming language initially developed at Google in the year 2007 by Robert Griesemer, Rob Pike, and Ken Thompson. It is a statically-typed language having syntax similar to that of C. It provides garbage collection, type safety, dynamic-typing capability, many advanced built-in t
- 1815Golang Pune | Meetup
https://www.meetup.com/Golang-Pune/
This is a group for anyone that is interested in the Go programming language. We invite novice developers to experienced developers to attend. We will make sure we have appropriate topics and presentations for all groups.
- 1816A Go package to automatically validate fields with tags
https://github.com/mccoyst/validate
A Go package to automatically validate fields with tags - mccoyst/validate
- 1817Accelerated Container Application Development
https://www.docker.com/
Docker is a platform designed to help developers build, share, and run container applications. We handle the tedious setup, so you can focus on the code.
- 1818Golang tutorial series
https://golangbot.com/learn-golang-series/
Tutorials to learn go from the ground up with a hands approach. Learning is made easier with simple examples. Run the code online using go playground.
- 1819Golang North East | Meetup
https://www.meetup.com/en-AU/Golang-North-East/
This is a group for anyone in the North East of England who's interested in the Go programming language. We meet every other month, on the fourth Tuesday, we will confirm the venue before each meetup.
- 1820Baltimore Metro Area Go User Group | Meetup
https://www.meetup.com/BaltimoreGolang/
If you're interested in the Go Programming Language (aka Golang), newbie, experienced or just curious, this is the meetup for you. We're an inclusive group that aims for diversity in our membership, the talks we select every month and your ideas for making this group thrive. Let's build an awesome G
- 1821Build microservice with rk-boot and let the team take over clean and tidy code.
https://github.com/rookie-ninja/rk-boot
Build microservice with rk-boot and let the team take over clean and tidy code. - rookie-ninja/rk-boot
- 1822GopherCon 2024 - Register Now
https://www.gophercon.com/
Rumor has it that all the cool Gophers will be in Chicago July 7th - 10th to celebrate the 10th anniversary of GopherCon! See you there?
- 1823Seattle Go Programmers | Meetup
https://www.meetup.com/golang/
The Seattle Go User Group is a community for anyone interested in the Go programming language. We provide opportunities to:• Discuss Go and related topics • Socialize with people who are interested in Go• Find or fill Go-related jobs If you want to chat all things Go, f
- 1824🔥 ~6x faster, stricter, configurable, extensible, and beautiful drop-in replacement for golint
https://github.com/mgechev/revive
🔥 ~6x faster, stricter, configurable, extensible, and beautiful drop-in replacement for golint - mgechev/revive
- 1825TOML-to-Go
https://xuri.me/toml-to-go
Convert TOML to Go instantly
- 1826XPath package for Golang, supports HTML, XML, JSON document query.
https://github.com/antchfx/xpath
XPath package for Golang, supports HTML, XML, JSON document query. - antchfx/xpath
- 1827Golang Paris | Meetup
https://www.meetup.com/Golang-Paris/
Go a fêté ses 12 ans le 10 novembre 2021 dernier !Golang Paris est un groupe d'intérêt ouvert à tous celles et ceux qui s'intéressent au langage Go et ses applications. Du curieux à l'experte, lors de nos rencontres nous nous efforçons de varier les contenus et les prérequis.Go, aussi connu sou
- 1828Remove unnecessary type conversions from Go source
https://github.com/mdempsky/unconvert
Remove unnecessary type conversions from Go source - mdempsky/unconvert
- 1829GolangLima | Meetup
https://www.meetup.com/Golang-Peru/
Come for drinks, share stories, and create awesome things using golang!Go is an open source project developed by a team at [Google](https://google.com/) and many [contributors](https://golang.org/CONTRIBUTORS) from the open source community.If you would like to give a presentation at GolangLima plea
- 1830Compare ANY markup documents.
https://github.com/xml-comp/xml-comp
Compare ANY markup documents. Contribute to XML-Comp/XML-Comp development by creating an account on GitHub.
- 1831Lagos Gophers | Meetup
https://www.meetup.com/GolangNigeria/
Calling all Gophers in and around Nigeria. Meet other gophers, learn and share ideas with other gophers, lets grow the golang community in Africa.
- 1832Direct3D9 wrapper for Go.
https://github.com/gonutz/d3d9
Direct3D9 wrapper for Go. Contribute to gonutz/d3d9 development by creating an account on GitHub.
- 1833GDG Berlin Golang | Meetup
https://www.meetup.com/golang-users-berlin/
We are a group of Golang users. Our aim is to meet like minded people, share our experience with others and to promote the use of Go.As Gophers and as Berliners, even if just visiting, we follow the Code of Conduct to make this a pleasant and inclusive environment for everyone: https://golang.org/co
- 1834Golang Tutorial Guide – A List of Free Courses to Learn the Go Programming Language
https://www.freecodecamp.org/news/golang-tutorial-list-free-courses-learn-go-programming-language/
Why choose Go? Before I get into my list of Go learning resources, let me tell you about one of the most famous defections in all of programming history. There was a developer in the Node.js who was so prolific that many people believed he was not a real person.
- 1835Chronos - A static race detector for the go language
https://github.com/amit-davidson/Chronos
Chronos - A static race detector for the go language - amit-davidson/Chronos
- 1836GolangNYC | Meetup
https://www.meetup.com/golanguagenewyork/
Go Language meetups and more in the greatest city in the world! <br>Our purpose is to help build the Go community within NYC. We are here to support individuals by providing a safe platform for them to speak, listen, and learn. When not hosting a meetup, we will help promote and support our pa
- 1837Go Israel | Meetup
https://www.meetup.com/Go-Israel/
This is the group for programmers who are interested in the High Performance, Scalable, lightweight, cross platform development tool that also promises ease of programming, agility and the fun you don't usually get using a low-level language. Welcome to the Israeli Go language Group.If you'd like to
- 1838A Go framework for building JSON web services inspired by Dropwizard
https://github.com/rcrowley/go-tigertonic
A Go framework for building JSON web services inspired by Dropwizard - rcrowley/go-tigertonic
- 1839Thessaloniki Gophers Meetup | Meetup
https://www.meetup.com/thessaloniki-golang-meetup/
The SKG Gophers meetup is just starting up and looking forward to meeting anyone interested in working with the Go programming language.SKG Gophers, is a meetup group based in Thessaloniki, Greece.Our aim is to:- Provide a friendly and inclusive place for Gophers to socialize- Discuss Go-related and
- 1840Find outdated golang packages
https://github.com/firstrow/go-outdated
Find outdated golang packages. Contribute to firstrow/go-outdated development by creating an account on GitHub.
- 1841GoBridge | Meetup
https://www.meetup.com/gobridge/
GoBridge is dedicated to building bridges that educate and empower underrepresented communities to teach and learn technical skills and foster diversity in Go. If you are new to GoBridge, please visit our website to learn of ways to get involved: http://golangbridge.org. All our events follow a code
- 1842Manage your repository's TODOs, tickets and checklists as config in your codebase.
https://github.com/augmentable-dev/tickgit
Manage your repository's TODOs, tickets and checklists as config in your codebase. - augmentable-dev/tickgit
- 1843Gone is a lightweight golang dependency injection framework; a series of Goners components are built in for rapid development of micro services.
https://github.com/gone-io/gone
Gone is a lightweight golang dependency injection framework; a series of Goners components are built in for rapid development of micro services. - gone-io/gone
- 1844Go WebAssembly Tutorial - Building a Calculator Tutorial
https://tutorialedge.net/golang/go-webassembly-tutorial/
In this tutorial, we are going to be looking at how you can compile your Go programs into WebAssembly
- 1845A command line tool that shows the status of Go repositories.
https://github.com/shurcooL/gostatus
A command line tool that shows the status of Go repositories. - shurcooL/gostatus
- 1846A linter that detect the possibility to use variables/constants from the Go standard library.
https://github.com/sashamelentyev/usestdlibvars
A linter that detect the possibility to use variables/constants from the Go standard library. - sashamelentyev/usestdlibvars
- 1847An opinionated productive web framework that helps scaling business easier.
https://github.com/appist/appy
An opinionated productive web framework that helps scaling business easier. - appist/appy
- 1848Extract data or evaluate value from HTML/XML documents using XPath
https://github.com/antchfx/xquery
Extract data or evaluate value from HTML/XML documents using XPath - antchfx/xquery
- 1849checkstyle for go
https://github.com/qiniu/checkstyle
checkstyle for go. Contribute to qiniu/checkstyle development by creating an account on GitHub.
- 1850A Go implementation of in-toto. in-toto is a framework to protect software supply chain integrity.
https://github.com/in-toto/in-toto-golang
A Go implementation of in-toto. in-toto is a framework to protect software supply chain integrity. - in-toto/in-toto-golang
- 1851Converts golang AST to JSON and JSON to AST
https://github.com/asty-org/asty
Converts golang AST to JSON and JSON to AST. Contribute to asty-org/asty development by creating an account on GitHub.
- 1852Learn Go - Best Go Tutorials | Hackr.io
https://hackr.io/tutorials/learn-golang
Learning Go? Check out these best online Go courses and tutorials recommended by the programming community. Pick the tutorial as per your learning style: video tutorials or a book. Free course or paid. Tutorials for beginners or advanced learners. Check Go community's reviews & comments.
- 1853Intro, Tools & Live Demo
https://snipcart.com/blog/golang-ecommerce-ponzu-cms-demo?utm_term=golang-ecommerce-ponzu-cms-demo
Explore the Golang ecosystem and its e-commerce capabilities. Then, try our demo on Go-powered CMS Ponzu, and learn to craft a great shopping experience yourself!
- 1854Run linters from Go code -
https://github.com/surullabs/lint
Run linters from Go code - . Contribute to surullabs/lint development by creating an account on GitHub.
- 1855Vim plugin for https://github.com/hexdigest/gounit
https://github.com/hexdigest/gounit-vim
Vim plugin for https://github.com/hexdigest/gounit - hexdigest/gounit-vim
- 1856Vim compiler plugin for Go (golang)
https://github.com/rjohnsondev/vim-compiler-go
Vim compiler plugin for Go (golang). Contribute to rjohnsondev/vim-compiler-go development by creating an account on GitHub.
- 1857Go language server extension using gopls for coc.nvim.
https://github.com/josa42/coc-go
Go language server extension using gopls for coc.nvim. - josa42/coc-go
- 1858A Go language server.
https://github.com/theia-ide/go-language-server
A Go language server. Contribute to theia-ide/go-language-server development by creating an account on GitHub.
- 1859100 Go Mistakes and How to Avoid Them
https://www.manning.com/books/100-go-mistakes-how-to-avoid-them
Spot errors in your Go code you didn’t even know you were making and boost your productivity by avoiding common mistakes and pitfalls.</b> 100 Go Mistakes and How to Avoid Them</i> shows you how to: Dodge the most common mistakes made by Go developers</li> Structure and organize your Go application</li> Handle data and control structures efficiently</li> Deal with errors in an idiomatic manner</li> Improve your concurrency skills</li> Optimize your code</li> Make your application production-ready and improve testing quality</li> </ul> 100 Go Mistakes and How to Avoid Them</i> puts a spotlight on common errors in Go code you might not even know you’re making. You’ll explore key areas of the language such as concurrency, testing, data structures, and more—and learn how to avoid and fix mistakes in your own projects. As you go, you’ll navigate the tricky bits of handling JSON data and HTTP services, discover best practices for Go code organization, and learn how to use slices efficiently.
- 1860An autocompletion daemon for the Go programming language
https://github.com/nsf/gocode
An autocompletion daemon for the Go programming language - nsf/gocode
- 1861gopher stickers
https://github.com/tenntenn/gopher-stickers
gopher stickers. Contribute to tenntenn/gopher-stickers development by creating an account on GitHub.
- 1862Elegant generics for Go
https://github.com/cheekybits/genny
Elegant generics for Go. Contribute to cheekybits/genny development by creating an account on GitHub.
- 1863A static code analyser for annotated TODO comments
https://github.com/preslavmihaylov/todocheck
A static code analyser for annotated TODO comments - preslavmihaylov/todocheck
- 1864Golang
https://tutorialedge.net/course/golang/
Learn the basics of Go and get started writing your own, highly performant Go programs.
- 1865Go by Example
https://www.manning.com/books/effective-go
Unlock Go’s unique perspective on program design, and start writing simple, maintainable, and testable Go code.</b> In Go by Example</i> you will learn how to: Write and test idiomatic and easy-to-maintain Go programs from scratch</li> Design and structure maintainable and testable command-line applications, concurrent programs, and web servers</li> Test Go according to best practices</li> Spot common Go anti-patterns</li> Understand what makes Go different from other languages</li> </ul> Go by Example</i> is a practical guide to writing high-quality code that’s easy to test and maintain. The book is full of best practices to adopt and anti-patterns to dodge. It explores what makes Go so dramatically different from other languages and how you can still leverage your existing skills into writing excellent Go code. Aimed at Go beginners looking to graduate to serious Go development, this book will help you write and test command line applications, web API clients and servers, concurrent programs, and more.
- 1866The SPIFFE Runtime Environment
https://github.com/spiffe/spire
The SPIFFE Runtime Environment. Contribute to spiffe/spire development by creating an account on GitHub.
- 1867Colorize (highlight) `go build` command output
https://github.com/songgao/colorgo
Colorize (highlight) `go build` command output. Contribute to songgao/colorgo development by creating an account on GitHub.
- 1868Golang Jobs - OnSite and Remote Golang Jobs - August 2022
https://golangjob.xyz
Search Golang jobs and apply for the right Golang position. OnSite and Remote Golang Jobs
- 1869The Go Gopher Amigurumi Pattern
https://github.com/sillecelik/go-gopher
The Go Gopher Amigurumi Pattern. Contribute to sillecelik/go-gopher development by creating an account on GitHub.
- 1870More than just a Go IDE
https://jetbrains.com/go
The complete Go IDE
- 1871janosdebugs/go-patterns
https://github.com/haveyoudebuggedit/go-patterns
Contribute to janosdebugs/go-patterns development by creating an account on GitHub.
- 1872Shipping Go
https://www.manning.com/books/continuous-delivery-in-go
Build and upgrade an automated software delivery pipeline that supports containerization, integration testing, semantic versioning, automated deployment, and more.</b> In Shipping Go</i> you will learn how to: Develop better software based on feedback from customers</li> Create a development pipeline that turns feedback into features</li> Reduce bugs with pipeline automation that validates code before it is deployed</li> Establish continuous testing for exceptional code quality</li> Serverless, container-based, and server-based deployments</li> Scale your deployment in a cost-effective way</li> Deliver a culture of continuous improvement</li> </ul> Shipping Go</i> is a hands-on guide to shipping Go-based software. Author Joel Holmes shows you the easy way to set up development pipelines, fully illustrated with practical examples in the powerful Go language. You’ll put continuous delivery and continuous integration into action, and discover instantly useful guidance on automating your team’s build and reacting with agility to customer demands. Your new pipelines will ferry your projects through production and deployment, and also improve your testing, code quality, and production applications.
- 1873Go Peerflix
https://github.com/Sioro-Neoku/go-peerflix
Go Peerflix. Contribute to Sioro-Neoku/go-peerflix development by creating an account on GitHub.
- 1874Go tool to generate documentation for environment variables
https://github.com/g4s8/envdoc
Go tool to generate documentation for environment variables - g4s8/envdoc
- 1875✨ This pack of 100+ gopher pictures and elements will help you to build own design of almost anything related to Go Programming Language: presentations, posts in blogs or social media, courses, videos and many, many more.
https://github.com/MariaLetta/free-gophers-pack
✨ This pack of 100+ gopher pictures and elements will help you to build own design of almost anything related to Go Programming Language: presentations, posts in blogs or social media, courses, vid...
- 1876A quick and easy way to setup a RESTful JSON API
https://github.com/ant0ine/go-json-rest
A quick and easy way to setup a RESTful JSON API. Contribute to ant0ine/go-json-rest development by creating an account on GitHub.
- 1877Start gRPC microservice from YAML, plugin of rk-boot
https://github.com/rookie-ninja/rk-grpc
Start gRPC microservice from YAML, plugin of rk-boot - rookie-ninja/rk-grpc
- 1878Understanding Go in sketchnotes Series' Articles
https://dev.to/aurelievache/series/26234
View Understanding Go in sketchnotes Series' Articles on DEV Community
- 1879PlantUML Class Diagram Generator for golang projects
https://github.com/jfeliu007/goplantuml
PlantUML Class Diagram Generator for golang projects - jfeliu007/goplantuml
- 1880Watches for changes in a directory tree and reruns a command in an acme win or just on the terminal.
https://github.com/eaburns/Watch
Watches for changes in a directory tree and reruns a command in an acme win or just on the terminal. - eaburns/Watch
- 1881Unit tests generator for Go programming language
https://github.com/hexdigest/gounit
Unit tests generator for Go programming language. Contribute to hexdigest/gounit development by creating an account on GitHub.
- 1882godal provides the ability to generate specific golang code. The godal is to enable developers to write fast code in an expressive way.
https://github.com/mafulong/godal
godal provides the ability to generate specific golang code. The godal is to enable developers to write fast code in an expressive way. - mafulong/godal
- 1883Scaling Go Applications | Better Stack Community
https://betterstack.com/community/guides/scaling-go/
Learn everything you need to know about building, deploying and scaling Go applications in production.
- 1884random gopher graphics
https://github.com/rogeralsing/gophers
random gopher graphics. Contribute to rogeralsing/gophers development by creating an account on GitHub.
- 1885Find outdated dependencies of your Go projects. go-mod-outdated provides a table view of the go list -u -m -json all command which lists all dependencies of a Go project and their available minor and patch updates. It also provides a way to filter indirect dependencies and dependencies without updates.
https://github.com/psampaz/go-mod-outdated
Find outdated dependencies of your Go projects. go-mod-outdated provides a table view of the go list -u -m -json all command which lists all dependencies of a Go project and their available minor a...
- 1886Simulating shitty network connections so you can build better systems.
https://github.com/tylertreat/Comcast
Simulating shitty network connections so you can build better systems. - tylertreat/comcast
- 1887Theia Go Extension
https://github.com/theia-ide/theia-go-extension
Theia Go Extension. Contribute to theia-ide/theia-go-extension development by creating an account on GitHub.
- 1888#go on Hashnode
https://hashnode.com/n/go
Go Language (50.0K followers · 4.4K articles) - Go is an open source programming language that makes it easy to build simple, reliable, and efficient software.
- 1889flexible data type for Go
https://github.com/usk81/generic
flexible data type for Go. Contribute to usk81/generic development by creating an account on GitHub.
- 1890List of opensource projects looking for help
https://github.com/ninedraft/gocryforhelp
List of opensource projects looking for help. Contribute to ninedraft/gocryforhelp development by creating an account on GitHub.
- 1891adorable gopher logos
https://github.com/GolangUA/gopher-logos
adorable gopher logos. Contribute to GolangUA/gopher-logos development by creating an account on GitHub.
- 1892Go implementation of the Rust `dbg` macro
https://github.com/tylerwince/godbg
Go implementation of the Rust `dbg` macro. Contribute to tylerwince/godbg development by creating an account on GitHub.
- 1893LiteIDE is a simple, open source, cross-platform Go IDE.
https://github.com/visualfc/liteide
LiteIDE is a simple, open source, cross-platform Go IDE. - GitHub - visualfc/liteide: LiteIDE is a simple, open source, cross-platform Go IDE.
- 1894The most opinionated Go source code linter for code audit.
https://github.com/go-critic/go-critic
The most opinionated Go source code linter for code audit. - go-critic/go-critic
- 1895An Enhanced Go Experience For The Atom Editor
https://github.com/joefitzgerald/go-plus
An Enhanced Go Experience For The Atom Editor. Contribute to joefitzgerald/go-plus development by creating an account on GitHub.
- 1896Build an Orchestrator in Go (From Scratch)
https://www.manning.com/books/build-an-orchestrator-in-go
Develop a deep understanding of Kubernetes and other orchestration systems by building your own with Go and the Docker API.</b> Orchestration systems like Kubernetes can seem like a black box: you deploy to the cloud and it magically handles everything you need. That might seem perfect—until something goes wrong and you don’t know how to find and fix your problems. Build an Orchestrator in Go (From Scratch)</i> reveals the inner workings of orchestration frameworks by guiding you through creating your own. In Build an Orchestrator in Go (From Scratch)</i> you will learn how to: Identify the components that make up any orchestration system</li> Schedule containers on to worker nodes</li> Start and stop containers using the Docker API</li> Manage a cluster of worker nodes using a simple API</li> Work with algorithms pioneered by Google’s Borg</li> Demystify orchestration systems like Kubernetes and Nomad</li> </ul> Build an Orchestrator in Go (From Scratch)</i> explains each stage of creating an orchestrator with diagrams, step-by-step instructions, and detailed Go code samples. Don’t worry if you’re not a Go expert. The book’s code is optimized for simplicity and readability, and its key concepts are easy to implement in any language. You’ll learn the foundational principles of these frameworks, and even how to manage your orchestrator with a command line interface.
- 1897A golang formatter that fixes long lines
https://github.com/segmentio/golines
A golang formatter that fixes long lines. Contribute to segmentio/golines development by creating an account on GitHub.
- 1898Start gin microservice from YAML, plugin of rk-boot
https://github.com/rookie-ninja/rk-gin
Start gin microservice from YAML, plugin of rk-boot - rookie-ninja/rk-gin
- 1899Go development plugin for Vim
https://github.com/fatih/vim-go
Go development plugin for Vim. Contribute to fatih/vim-go development by creating an account on GitHub.
- 1900Learn how to write webapps without a framework in Go.
https://github.com/thewhitetulip/web-dev-golang-anti-textbook/
Learn how to write webapps without a framework in Go. - thewhitetulip/web-dev-golang-anti-textbook
- 1901Minimalist net/http middleware for golang
https://github.com/carbocation/interpose
Minimalist net/http middleware for golang. Contribute to carbocation/interpose development by creating an account on GitHub.
- 1902Generics for go
https://github.com/bouk/gonerics
Generics for go. Contribute to bouk/gonerics development by creating an account on GitHub.
- 1903PHP parser written in Go
https://github.com/z7zmey/php-parser
PHP parser written in Go. Contribute to z7zmey/php-parser development by creating an account on GitHub.
- 1904How to Use Godog for Behavior-driven Development in Go - Semaphore
https://semaphoreci.com/community/tutorials/how-to-use-godog-for-behavior-driven-development-in-go
Get started with Godog — a Behavior-driven development framework for building and testing Go applications.
- 1905Learn everything about Go in 7 days (from a Nodejs developer).
https://github.com/harrytran103/7_days_of_go
Learn everything about Go in 7 days (from a Nodejs developer). - cuongndc/7_days_of_go
- 1906GoDays 2020 in Berlin - Connect with hundreds of like-minded people
https://www.godays.io/
GoDays is a three day conference focused on connecting the Go community. In numerous technical talks and hands-on workshops, Go experts, beginners, and everyone in between will exchange ideas and learn from each other.
- 1907Emacs mode for the Go programming language
https://github.com/dominikh/go-mode.el
Emacs mode for the Go programming language. Contribute to dominikh/go-mode.el development by creating an account on GitHub.
- 1908Generate a Go struct from XML.
https://github.com/miku/zek
Generate a Go struct from XML. Contribute to miku/zek development by creating an account on GitHub.
- 1909Go go-to guide
https://yourbasic.org/golang
Become a better Go programmer with these tutorials and code examples.
- 1910Go gopher Vector Data [.ai, .svg]
https://github.com/keygx/Go-gopher-Vector
Go gopher Vector Data [.ai, .svg]. Contribute to keygx/Go-gopher-Vector development by creating an account on GitHub.
- 1911Go extension for Visual Studio Code
https://github.com/golang/vscode-go
Go extension for Visual Studio Code. Contribute to golang/vscode-go development by creating an account on GitHub.
- 1912Key/Value database benchmark
https://github.com/jimrobinson/kvbench
Key/Value database benchmark. Contribute to jimrobinson/kvbench development by creating an account on GitHub.
- 1913The Open Operator Collection
https://jujucharms.com/
Universal operators for application lifecycle management on Linux, Windows and Kubernetes
- 1914Gopherize.me app
https://github.com/matryer/gopherize.me
Gopherize.me app. Contribute to matryer/gopherize.me development by creating an account on GitHub.
- 1915A benchmarking shootout of various db/SQL utilities for Go
https://github.com/tyler-smith/golang-sql-benchmark
A benchmarking shootout of various db/SQL utilities for Go - tyler-smith/golang-sql-benchmark
- 1916A pair programming service using operational transforms
https://github.com/jeffail/leaps
A pair programming service using operational transforms - Jeffail/leaps
- 1917:robot: sake is a task runner for local and remote hosts
https://github.com/alajmo/sake
:robot: sake is a task runner for local and remote hosts - alajmo/sake
- 1918The unix-way web crawler
https://github.com/s0rg/crawley
The unix-way web crawler. Contribute to s0rg/crawley development by creating an account on GitHub.
- 1919An app that displays updates for the Go packages in your GOPATH.
https://github.com/shurcooL/Go-Package-Store
An app that displays updates for the Go packages in your GOPATH. - shurcooL/Go-Package-Store
- 1920REST Layer, Go (golang) REST API framework
https://github.com/rs/rest-layer
REST Layer, Go (golang) REST API framework. Contribute to rs/rest-layer development by creating an account on GitHub.
- 1921Automatically generate Go test boilerplate from your source code.
https://github.com/cweill/gotests
Automatically generate Go test boilerplate from your source code. - cweill/gotests
- 1922Search and save shell snippets without leaving your terminal
https://github.com/crufter/borg
Search and save shell snippets without leaving your terminal - ok-borg/borg
- 1923A set of example golang code to start learning Go
https://github.com/mkaz/working-with-go
A set of example golang code to start learning Go. Contribute to mkaz/working-with-go development by creating an account on GitHub.
- 1924vacuum is the worlds fastest OpenAPI 3, OpenAPI 2 / Swagger linter and quality analysis tool. Built in go, it tears through API specs faster than you can think. vacuum is compatible with Spectral rulesets and generates compatible reports.
https://github.com/daveshanley/vacuum
vacuum is the worlds fastest OpenAPI 3, OpenAPI 2 / Swagger linter and quality analysis tool. Built in go, it tears through API specs faster than you can think. vacuum is compatible with Spectral r...
- 1925Building and Testing a REST API in Go with Gorilla Mux and PostgreSQL - Semaphore
https://semaphoreci.com/community/tutorials/building-and-testing-a-rest-api-in-go-with-gorilla-mux-and-postgresql
Learn how to build simple and well-tested REST APIs backed by PostgreSQL in Go, using Gorilla Mux — a highly stable and versatile router.
- 1926Build and deploy Go applications
https://github.com/google/ko
Build and deploy Go applications. Contribute to ko-build/ko development by creating an account on GitHub.
- 1927Haskell-flavoured functions for Go :smiley:
https://github.com/DylanMeeus/hasgo
Haskell-flavoured functions for Go :smiley:. Contribute to DylanMeeus/hasgo development by creating an account on GitHub.
- 1928Wave Terminal
https://waveterm.dev
An open-source, AI-native, terminal built for seamless workflows
- 1929GoReplay is an open-source tool for capturing and replaying live HTTP traffic into a test environment in order to continuously test your system with real data. It can be used to increase confidence in code deployments, configuration changes and infrastructure changes.
https://github.com/buger/gor
GoReplay is an open-source tool for capturing and replaying live HTTP traffic into a test environment in order to continuously test your system with real data. It can be used to increase confidence...
- 1930Gearbox is a web framework written in Go with a focus on high performance
https://github.com/abahmed/gearbox
Gearbox :gear: is a web framework written in Go with a focus on high performance - GitHub - gogearbox/gearbox: Gearbox is a web framework written in Go with a focus on high performance
- 1931Go benchmark harness.
https://github.com/davecheney/autobench
Go benchmark harness. . Contribute to davecheney/autobench development by creating an account on GitHub.
- 1932OctoLinker — Links together, what belongs together
https://github.com/OctoLinker/browser-extension
OctoLinker — Links together, what belongs together - OctoLinker/OctoLinker
- 1933Anteon (formerly Ddosify) - Effortless Kubernetes Monitoring and Performance Testing. Available on CLI, Self-Hosted, and Cloud
https://github.com/ddosify/ddosify
Anteon (formerly Ddosify) - Effortless Kubernetes Monitoring and Performance Testing. Available on CLI, Self-Hosted, and Cloud - getanteon/anteon
- 1934Free gophers
https://github.com/egonelbre/gophers
Free gophers. Contribute to egonelbre/gophers development by creating an account on GitHub.
- 1935Visualize Go Dependency Trees
https://github.com/KyleBanks/depth
Visualize Go Dependency Trees. Contribute to KyleBanks/depth development by creating an account on GitHub.
- 1936Fast and lightweight DNS proxy as ad-blocker for local network with many features
https://github.com/0xERR0R/blocky
Fast and lightweight DNS proxy as ad-blocker for local network with many features - 0xERR0R/blocky
- 1937New community features for Google Chat and an update on Currents
https://plus.google.com/communities/114112804251407510571
Note: This blog post outlines upcoming changes to Google Currents for Workspace users. For information on the previous deprecation of Googl...
- 1938Go package that handles HTML, JSON, XML and etc. responses
https://github.com/alioygur/gores
Go package that handles HTML, JSON, XML and etc. responses - aliuygur/gores
- 1939fault injection library in go using standard http middleware
https://github.com/github/go-fault
fault injection library in go using standard http middleware - lingrino/go-fault
- 1940dbq vs sqlx vs GORM
https://medium.com/@rocketlaunchr.cloud/how-to-benchmark-dbq-vs-sqlx-vs-gorm-e814caacecb5
Benchmark 3 competing libraries to see which is fastest.
- 1941🥘 An independent package manager for compiled binaries.
https://github.com/marwanhawari/stew
🥘 An independent package manager for compiled binaries. - marwanhawari/stew
- 1942An overview of Go syntax and features.
https://github.com/a8m/go-lang-cheat-sheet
An overview of Go syntax and features. Contribute to a8m/golang-cheat-sheet development by creating an account on GitHub.
- 1943SeaweedFS is a fast distributed storage system for blobs, objects, files, and data lake, for billions of files! Blob store has O(1) disk seek, cloud tiering. Filer supports Cloud Drive, cross-DC active-active replication, Kubernetes, POSIX FUSE mount, S3 API, S3 Gateway, Hadoop, WebDAV, encryption, Erasure Coding.
https://github.com/chrislusf/seaweedfs
SeaweedFS is a fast distributed storage system for blobs, objects, files, and data lake, for billions of files! Blob store has O(1) disk seek, cloud tiering. Filer supports Cloud Drive, cross-DC ac...
- 1944Dockerize Go Applications
https://semaphoreci.com/community/tutorials/how-to-deploy-a-go-web-application-with-docker
Get familiar with how Docker can improve the way you build, test and deploy Go web applications, and see how you can use Semaphore for continuous deployment.
- 1945Programming with Google Go
https://www.coursera.org/specializations/google-golang
Offered by University of California, Irvine. Advance Your Computer Programming Career. Develop efficient applications with Google's ... Enroll for free.
- 1946Packer is a tool for creating identical machine images for multiple platforms from a single source configuration.
https://github.com/mitchellh/packer
Packer is a tool for creating identical machine images for multiple platforms from a single source configuration. - hashicorp/packer
- 1947GoSF | Meetup
https://www.meetup.com/golangsf
A meetup group to discuss the Go Programming Language.**About Go** (from golang.org)The Go programming language is an open source project to make programmers more productive. Go is expressive, concise, clean, and efficient. Its concurrency mechanisms make it easy to write programs that get the most
- 1948Enterprise Network Flow Collector (IPFIX, sFlow, Netflow)
https://github.com/VerizonDigital/vflow
Enterprise Network Flow Collector (IPFIX, sFlow, Netflow) - GitHub - Edgio/vflow: Enterprise Network Flow Collector (IPFIX, sFlow, Netflow)
- 1949BANjO is a simple web framework written in Go (golang)
https://github.com/nsheremet/banjo
BANjO is a simple web framework written in Go (golang) - gdwrd/banjo
- 1950A tool to generate bun, gorm, sql, sqlx and xorm sql code.
https://github.com/anqiansong/sqlgen
A tool to generate bun, gorm, sql, sqlx and xorm sql code. - kesonan/sqlgen
- 1951Building Microservices with Go
https://www.youtube.com/playlist?list=PLmD8u-IFdreyh6EUfevBcbiuCKzFk0EW_
Week by week Building Microservices builds on the previous weeks code teaching you how to build a multi-tier microservice system. The code structure for the ...
- 1952Building a Go Microservice with CI/CD
https://semaphoreci.com/community/tutorials/building-go-web-applications-and-microservices-using-gin
Get familiar with Gin and find out how it can help you reduce boilerplate code and build a request handling pipeline.
- 1953The Software Pro's Best Kept Secret.
https://app.codecrafters.io/tracks/go
Real-world proficiency projects designed for experienced engineers. Develop software craftsmanship by recreating popular devtools from scratch.
- 1954Learn Go Programming
https://blog.learngoprogramming.com
Visual, concise and detailed tutorials, tips and tricks about Go (aka Golang).
- 1955Right imports sorting & code formatting tool (goimports alternative)
https://github.com/incu6us/goimports-reviser
Right imports sorting & code formatting tool (goimports alternative) - incu6us/goimports-reviser
- 1956A tiny webchat server in Go.
https://github.com/rafael-santiago/cherry
A tiny webchat server in Go. Contribute to rafael-santiago/cherry development by creating an account on GitHub.
- 1957Parser / Scanner Generator
https://github.com/goccmack/gocc
Parser / Scanner Generator. Contribute to goccmack/gocc development by creating an account on GitHub.
- 1958The Ultimate Guide | Better Stack Community
https://betterstack.com/community/guides/logging/logging-in-go/
Learn everything about the new Slog package which brings high-performance structured, and leveled logging to the Go standard library
- 1959Application for HTTP benchmarking via different rules and configs
https://github.com/mrLSD/go-benchmark-app
Application for HTTP benchmarking via different rules and configs - mrLSD/go-benchmark-app
- 1960Benchmarks of common basic operations for the Go language.
https://github.com/PuerkitoBio/gocostmodel
Benchmarks of common basic operations for the Go language. - mna/gocostmodel
- 1961Benchmark of Golang JSON Libraries
https://github.com/zerosnake0/go-json-benchmark
Benchmark of Golang JSON Libraries. Contribute to zerosnake0/go-json-benchmark development by creating an account on GitHub.
- 1962Direct file transfer over WebRTC
https://github.com/Antonito/gfile
Direct file transfer over WebRTC. Contribute to Antonito/gfile development by creating an account on GitHub.
- 1963Lightweight selfhosted Firefox Send alternative without public upload. AWS S3 supported.
https://github.com/Forceu/gokapi
Lightweight selfhosted Firefox Send alternative without public upload. AWS S3 supported. - Forceu/Gokapi
- 1964Go web framework with a natural feel
https://github.com/zpatrick/fireball
Go web framework with a natural feel. Contribute to ridgelines/fireball development by creating an account on GitHub.
- 1965A Golang plugin collection for SublimeText 3, providing code completion and other IDE-like features.
https://github.com/DisposaBoy/GoSublime
A Golang plugin collection for SublimeText 3, providing code completion and other IDE-like features. - DisposaBoy/GoSublime
- 1966bobg/decouple
https://github.com/bobg/decouple
Contribute to bobg/decouple development by creating an account on GitHub.
- 1967This is the companion repo for Go Succinctly by Amir Irani.
https://github.com/thedevsir/gosuccinctly
This is the companion repo for Go Succinctly by Amir Irani. - thedevsir/gosuccinctly
- 1968part 1 - Introduction & Installation
https://dev.to/aurelievache/learning-go-by-examples-introduction-448n
Serie of article in order to learn Golang language by concrete applications as example
- 1969Visualize call graph of a Go program using Graphviz
https://github.com/TrueFurby/go-callvis
Visualize call graph of a Go program using Graphviz - ondrajz/go-callvis
- 1970bobg/modver
https://github.com/bobg/modver
Contribute to bobg/modver development by creating an account on GitHub.
- 1971XSD (XML Schema Definition) parser and Go/C/Java/Rust/TypeScript code generator
https://github.com/xuri/xgen
XSD (XML Schema Definition) parser and Go/C/Java/Rust/TypeScript code generator - xuri/xgen
- 1972"go build" wrapper to add version info to Golang applications
https://github.com/ahmetalpbalkan/govvv
"go build" wrapper to add version info to Golang applications - ahmetb/govvv
- 1973package main
https://www.youtube.com/packagemain
The Backend is the backbone of any modern application. It handles data storage, user interactions, complex calculations, and much more. Mastering this side of development opens doors to exciting opportunities, but it can be hard. The "package main" channel is here to help!
- 1974A tool for formatting Go test results as readable documentation
https://github.com/bitfield/gotestdox
A tool for formatting Go test results as readable documentation - bitfield/gotestdox
- 1975The world’s easiest introduction to WebAssembly🕹
https://medium.com/@martinolsansky/webassembly-with-golang-is-fun-b243c0e34f02
WASM in Golang for JavaScript developers.
- 1976Coding Games and Programming Challenges to Code Better
https://www.codingame.com/
CodinGame is a challenge-based training platform for programmers where you can play with the hottest programming topics. Solve games, code AI bots, learn from your peers, have fun.
- 1977NES emulator written in Go.
https://github.com/fogleman/nes
NES emulator written in Go. Contribute to fogleman/nes development by creating an account on GitHub.
- 1978A few miscellaneous Go microbenchmarks.
https://github.com/tylertreat/go-benchmarks
A few miscellaneous Go microbenchmarks. Contribute to tylertreat/go-benchmarks development by creating an account on GitHub.
- 1979A tool for design-by-contract in Go
https://github.com/Parquery/gocontracts
A tool for design-by-contract in Go. Contribute to Parquery/gocontracts development by creating an account on GitHub.
- 1980Automatically generate RESTful API documentation for GO projects - aligned with Open API Specification standard
https://github.com/go-oas/docs
Automatically generate RESTful API documentation for GO projects - aligned with Open API Specification standard - go-oas/docs
- 1981:alarm_clock: A TCP proxy to simulate network and system conditions for chaos and resiliency testing
https://github.com/shopify/toxiproxy
:alarm_clock: :fire: A TCP proxy to simulate network and system conditions for chaos and resiliency testing - GitHub - Shopify/toxiproxy: :alarm_clock: A TCP proxy to simulate network and system co...
- 1982The Cloud Native Application Proxy
https://github.com/containous/traefik
The Cloud Native Application Proxy. Contribute to traefik/traefik development by creating an account on GitHub.
- 1983Multi-platform Nintendo Game Boy Color emulator written in Go
https://github.com/Humpheh/goboy
Multi-platform Nintendo Game Boy Color emulator written in Go - Humpheh/goboy
- 1984Projects
https://github.com/golang/go/wiki/Projects
The Go programming language. Contribute to golang/go development by creating an account on GitHub.
- 1985aptly - Debian repository management tool (fork of aptly-dev/aptly)
https://github.com/smira/aptly
aptly - Debian repository management tool (fork of aptly-dev/aptly) - smira/aptly-fork
FAQ'sto know more about the topic.
mail [email protected] to add your project or resources here 🔥.
- 1Why should I choose Golang for backend development?
- 2Why is concurrency important in Golang?
- 3Why is Golang considered efficient in memory management?
- 4Why is the Go community growing?
- 5Why is Go preferred for cloud services?
- 6Why is Golang's syntax considered simple?
- 7Why do companies adopt Golang for DevOps?
- 8Why is error handling different in Golang?
- 9Why is Golang suitable for microservices architecture?
- 10Why do developers appreciate Golang's standard library?
- 11Why is Golang preferred for cloud-native applications?
- 12Why is Golang popular for building web applications?
- 13Why do startups choose Golang for their tech stack?
- 14Why is Golang a great choice for data processing tasks?
- 15How does Golang handle errors?
- 16What are the benefits of using Go modules?
- 17How does Golang support concurrency?
- 18Why should I choose Golang for web development?
- 19Why is Golang considered a good language for microservices?
- 20Why is Golang popular for cloud-native applications?
- 21Why is Golang suitable for DevOps practices?
- 22Why use Golang for developing RESTful APIs?
- 23Why choose Golang for data processing tasks?
- 24Why is Golang favored for developing command-line tools?
- 25Why is Golang a good choice for building blockchain applications?
- 26Why should you consider Golang for developing web applications?
- 27Why is Golang popular for microservices architecture?
- 28Why is Golang ideal for building RESTful APIs?
- 29Why is Golang beneficial for cloud-native development?
- 30How does Golang's memory management work?
- 31What are the benefits of using Goroutines in Golang?
- 32How does error handling in Golang differ from other languages?
- 33What are the differences between Go and other programming languages?
- 34How does Golang handle data structures?
- 35What are the main features of Golang?
- 36How does Golang compare to Java?
- 37What is the significance of goroutines in Golang?
- 38What is a channel in Golang, and how is it used?
- 39What is the difference between a slice and an array in Golang?
- 40How do you handle errors in Golang?
- 41What are interfaces in Golang?
- 42What is a struct in Golang?
- 43How do you create a goroutine in Golang?
- 44Why is Golang considered a good choice for microservices?
- 45Why should I use interfaces in Golang?
- 46Why is error handling important in Golang?
- 47Why is Go a good language for building command-line tools?
- 48What are the best practices for structuring a Go project?
- 49What are the main differences between Go and other programming languages like Python or Java?
- 50How can I get started with Go for web development?
- 51Why should I use Go for building microservices?
- 52Why is error handling important in Go?
- 53Why is Go a good choice for cloud-native applications?
- 54Why do companies choose Go for backend development?
- 55Why should I learn Go as a programming language?
- 56How does Go handle errors?
- 57What are Go's strengths compared to other programming languages?
- 58Why should I learn Golang for backend development?
- 59What are the best practices for writing Go code?
- 60Why is Go popular for microservices architecture?
- 61Why choose Golang for cloud-native applications?
- 62Why is Golang a good choice for DevOps?
- 63What makes Golang suitable for data processing?
- 64What are the benefits of using Golang for web development?
- 65What are the best practices for writing clean Go code?
- 66How does Golang handle concurrency?
- 67What are some common mistakes in Golang programming?
- 68How can Golang be used in microservices architecture?
- 69What tools can help with Golang development?
- 70What is the difference between pointers and values in Golang?
- 71How do interfaces work in Golang?
- 72How does error handling work in Golang?
- 73What are Goroutines and Channels?
- 74How to set up a Golang development environment?
- 75How to create a simple REST API with Golang?
- 76How to use Goroutines for concurrency in Golang?
- 77How to read and write files in Golang?
- 78How to connect to a database using Golang?
- 79How to use struct and methods in Golang?
- 80How to handle JSON in Golang?
- 81How to create a simple web server in Golang?
- 82What is concurrency in Golang?
- 83How do I read a file in Golang?
- 84How do I create a slice in Golang?
- 85How do I handle errors in Golang?
- 86How do I work with JSON in Golang?
- 87How do I create a web server in Golang?
- 88How do I manage dependencies in Golang?
- 89How do I handle files in Golang?
- 90How do I work with goroutines in Golang?
- 91What are channels in Golang?
- 92What are the common errors in Golang?
- 93How do I create a web server in Golang?
- 94How do I connect to a database in Golang?
- 95How do I write tests in Golang?
- 96How do I handle errors in Golang?
- 97How do I create a REST API with Golang?
- 98How do I create a command-line application in Golang?
- 99How do I perform concurrency in Golang?
- 100How do I manage dependencies in Golang?
Queriesor most google FAQ's about GoLang.
mail [email protected] to add more queries here 🔍.
- 1
why golang is bad for smart programmers
- 2
does golang have sets
- 3
what does make do golang
- 4
will golang replace python
- 5
will golang die
- 6
what is golang developer
- 7
is golang hard to learn
- 8
where golang is used
- 9
can golang be used for web development
- 10
how golang is different from java
- 11
is golang open source
- 12
when did golang become popular
- 13
are golang developers in demand
- 14
is golang easy to learn
- 15
how do golang channels work
- 16
where golang is used mostly
- 17
who uses golang
- 18
what are golang channels
- 19
are golang maps ordered
- 20
how golang compiler works
- 21
why golang is popular
- 22
what is struct in golang
- 23
which golang web framework
- 24
does golang have ternary operator
- 25
what are golang interfaces
- 26
should i learn golang
- 27
can golang be used for embedded systems
- 28
can golang replace python
- 29
does golang have inheritance
- 30
what can golang be used for
- 31
what is interface in golang
- 32
what is slice in golang
- 33
will rust replace golang
- 34
do golang tests run in parallel
- 35
what are golang modules
- 36
are golang maps thread safe
- 37
why golang is not popular
- 38
how golang handles concurrency
- 39
does golang have objects
- 40
how golang garbage collection works
- 41
who invented golang
- 42
why did google create golang
- 43
why golang does not have classes
- 44
why golang over java
- 45
what is context in golang
- 46
why golang reddit
- 47
who owns golang
- 48
does golang have garbage collection
- 49
how golang runtime works
- 50
is golang strongly typed
- 51
how golang select works
- 52
is golang a functional language
- 53
does golang have pointers
- 54
are golang maps passed by reference
- 55
what golang is good for
- 56
what golang is used for
- 57
why golang is fast
- 58
which ide for golang
- 59
why golang is used
- 60
is golang in demand
- 61
is golang a compiled language
- 62
why golang was created
- 63
does golang switch fall through
- 64
is golang worth learning
- 65
what is defer in golang
- 66
are golang slices ordered
- 67
is golang object oriented
- 68
does golang have a future
- 69
how long will it take to learn golang
- 70
is golang memory safe
- 71
does golang have classes
- 72
who wrote golang
- 73
what are golang packages
- 74
should i learn golang reddit
- 75
which ide is best for golang
- 76
where to practice golang
- 77
what is golang mostly used for
- 78
does golang have generics
- 79
what does log.fatal in golang
- 80
is golang statically typed
- 81
why golang is better than java
- 82
who is golang developer
- 83
what golang can do
- 84
should i learn golang in 2024
- 85
should i learn python or golang
- 86
should i learn java or golang
- 87
how golang scheduler works
- 88
how golang gc works
- 89
how golang works
- 90
are golang channels thread safe
- 91
why golang is better
- 92
can golang be used for ai
- 93
how golang is better than java
- 94
where to learn golang
- 95
can golang be used for frontend
- 96
when did golang come out
- 97
can golang replace c++
- 98
what is golang best used for
- 99
can golang be used for game development
- 100
when golang was created
- 101
will golang replace java
- 102
will golang replace c++
- 103
will carbon replace golang
- 104
can golang be used for machine learning
- 105
who maintains golang
- 106
can golang replace java
- 107
does golang have enums
More Sitesto check out once you're finished browsing here.
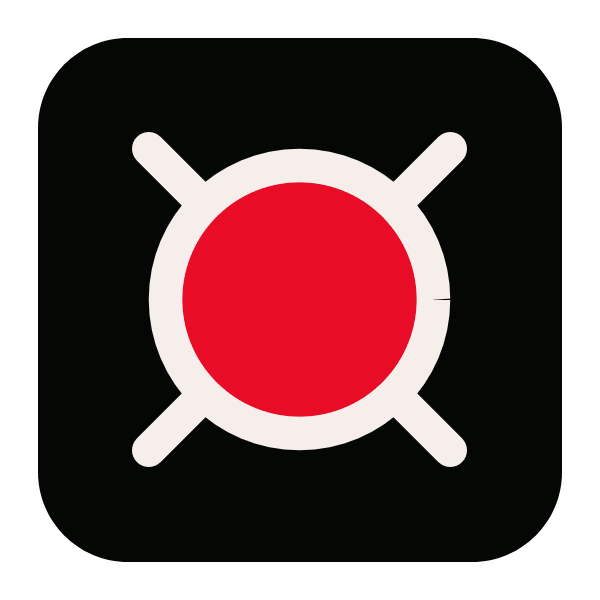
0x3d
https://www.0x3d.site/
0x3d is designed for aggregating information.
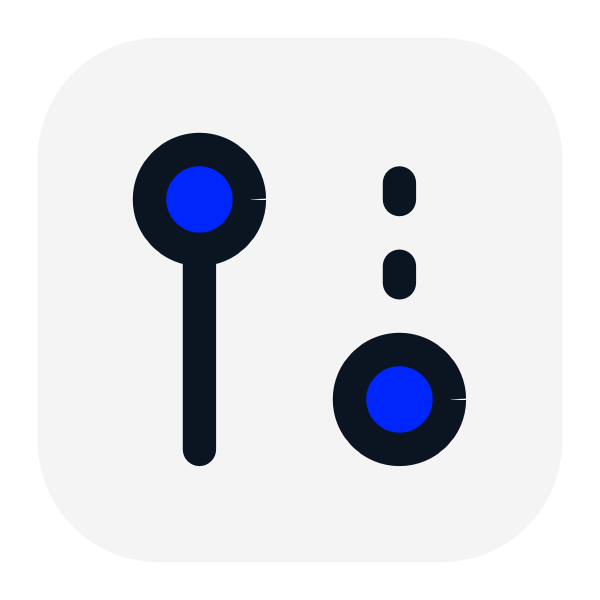
NodeJS
https://nodejs.0x3d.site/
NodeJS Online Directory

Cross Platform
https://cross-platform.0x3d.site/
Cross Platform Online Directory
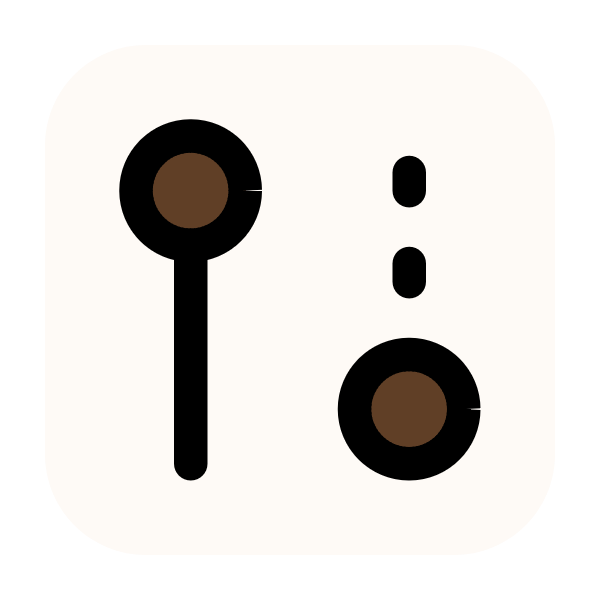
Open Source
https://open-source.0x3d.site/
Open Source Online Directory
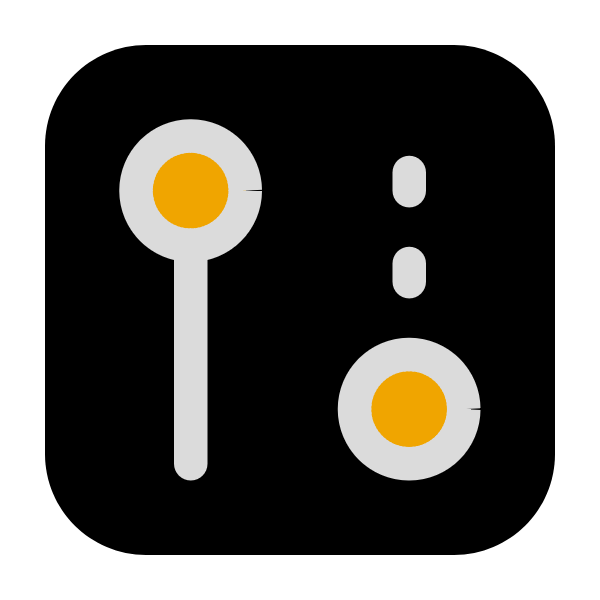
Analytics
https://analytics.0x3d.site/
Analytics Online Directory
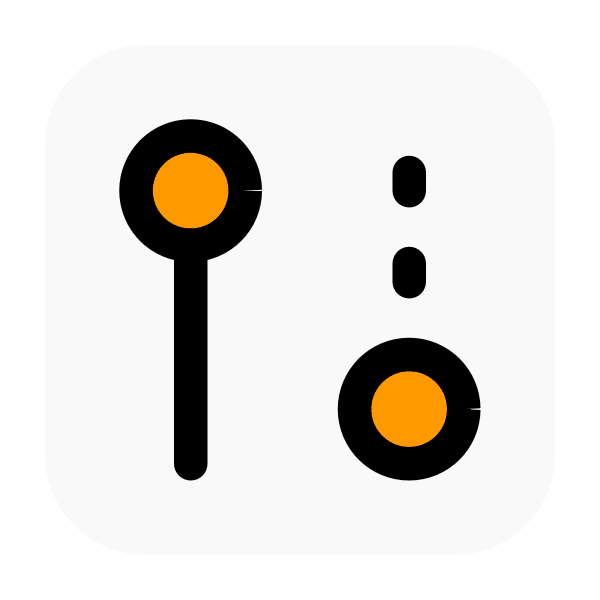
JavaScript
https://javascript.0x3d.site/
JavaScript Online Directory

GoLang
https://golang.0x3d.site/
GoLang Online Directory
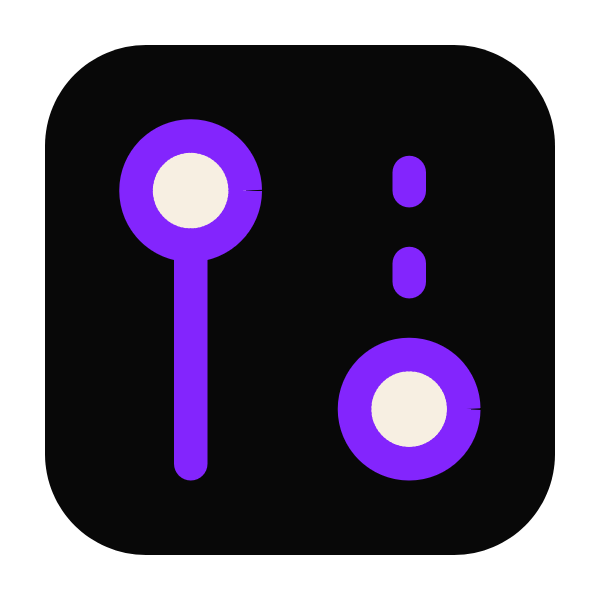
Python
https://python.0x3d.site/
Python Online Directory

Swift
https://swift.0x3d.site/
Swift Online Directory

Rust
https://rust.0x3d.site/
Rust Online Directory
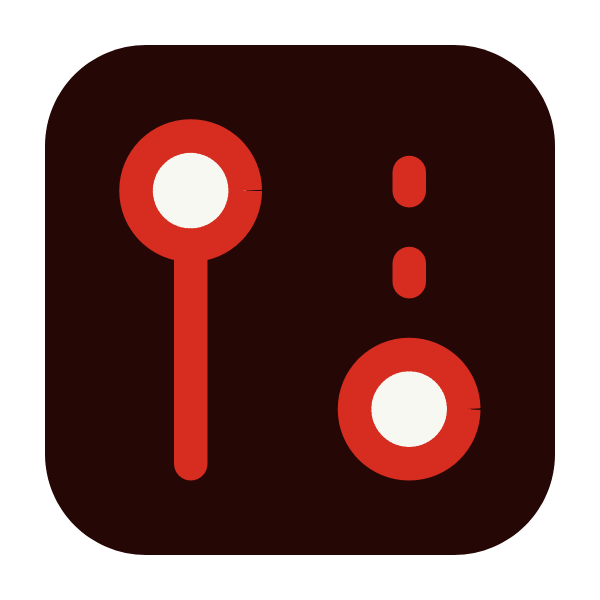
Scala
https://scala.0x3d.site/
Scala Online Directory

Ruby
https://ruby.0x3d.site/
Ruby Online Directory

Clojure
https://clojure.0x3d.site/
Clojure Online Directory
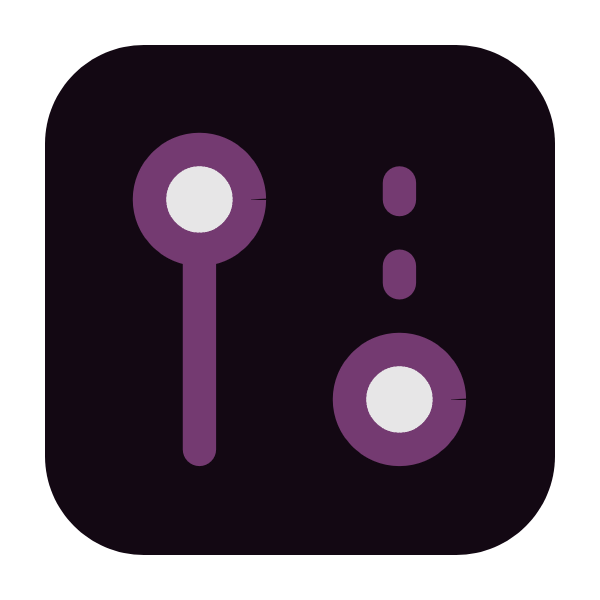
Elixir
https://elixir.0x3d.site/
Elixir Online Directory
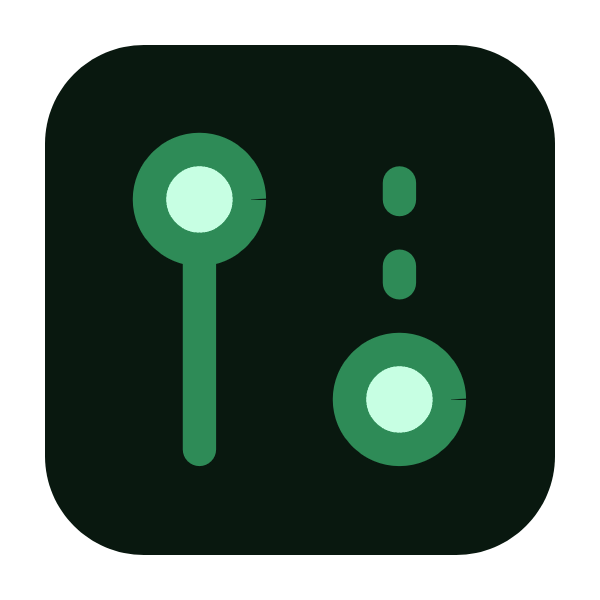
Elm
https://elm.0x3d.site/
Elm Online Directory

Lua
https://lua.0x3d.site/
Lua Online Directory
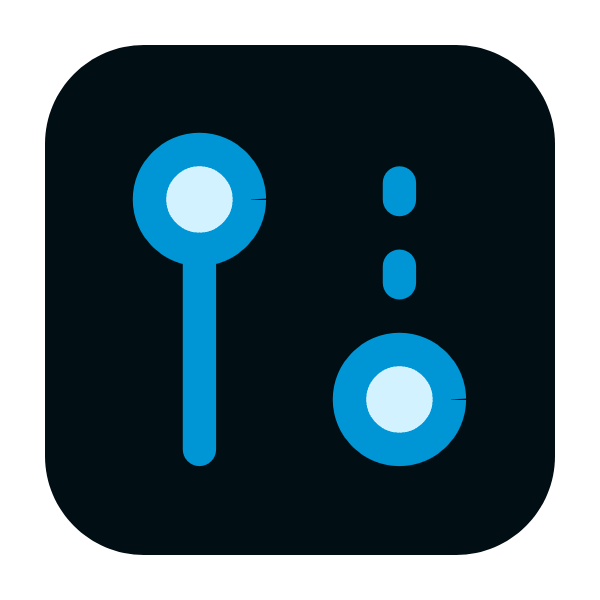
C Programming
https://c-programming.0x3d.site/
C Programming Online Directory
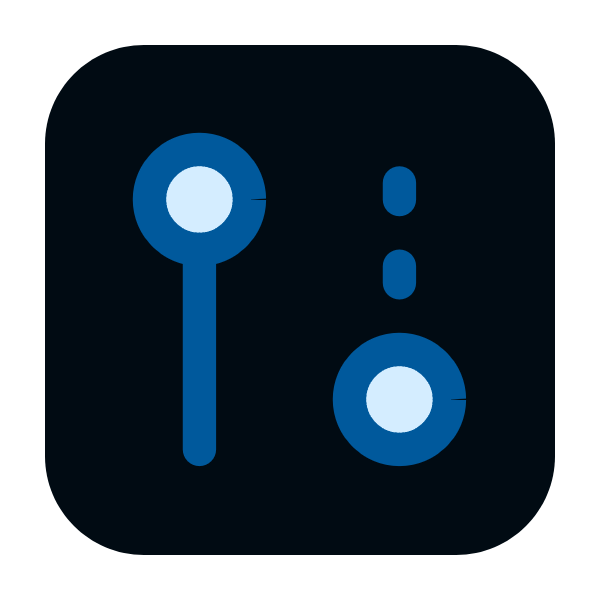
C++ Programming
https://cpp-programming.0x3d.site/
C++ Programming Online Directory

R Programming
https://r-programming.0x3d.site/
R Programming Online Directory

Perl
https://perl.0x3d.site/
Perl Online Directory
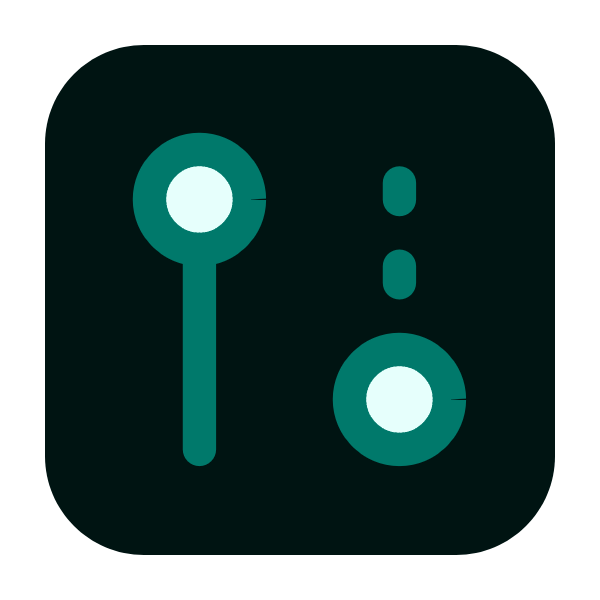
Java
https://java.0x3d.site/
Java Online Directory

Kotlin
https://kotlin.0x3d.site/
Kotlin Online Directory

PHP
https://php.0x3d.site/
PHP Online Directory

React JS
https://react.0x3d.site/
React JS Online Directory
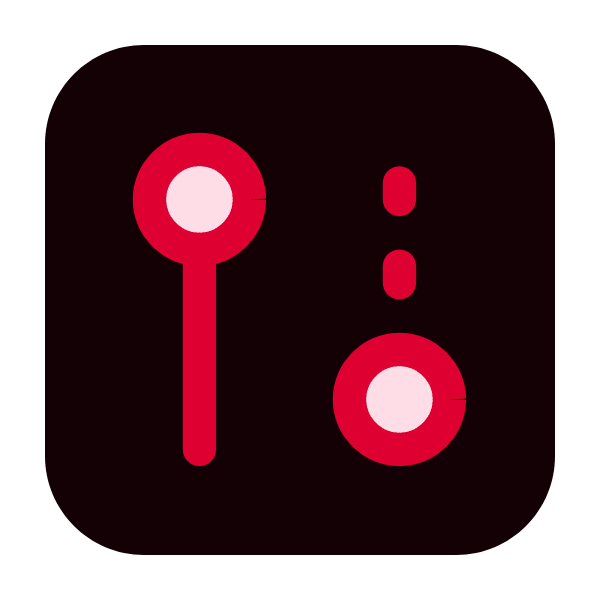
Angular
https://angular.0x3d.site/
Angular JS Online Directory